2023-03-01 10:19:01 +00:00
|
|
|
/**
|
|
|
|
*
|
|
|
|
* OverlayPanel is a container component positioned as connected to its target.
|
|
|
|
*
|
|
|
|
* - [Live Demo](https://www.primefaces.org/primevue/overlaypanel)
|
|
|
|
*
|
|
|
|
* @module overlaypanel
|
|
|
|
*
|
|
|
|
*/
|
2022-09-06 12:03:37 +00:00
|
|
|
import { VNode } from 'vue';
|
|
|
|
import { ClassComponent, GlobalComponentConstructor } from '../ts-helpers';
|
|
|
|
|
2023-03-01 10:19:01 +00:00
|
|
|
/**
|
|
|
|
* OverlayPanel breakpoint metadata.
|
|
|
|
*/
|
2022-09-06 12:03:37 +00:00
|
|
|
export interface OverlayPanelBreakpoints {
|
|
|
|
/**
|
|
|
|
* Breakpoint for responsive mode.
|
|
|
|
*
|
|
|
|
* Example:
|
|
|
|
*
|
|
|
|
* <OverlayPanel :breakpoints="{'960px': '75vw', '640px': '100vw'}" ... />
|
|
|
|
*
|
|
|
|
* Result:
|
|
|
|
*
|
|
|
|
* @media screen and (max-width: ${breakpoint[key]}) {
|
|
|
|
* .p-overlaypanel[attributeSelector] {
|
|
|
|
* width: ${breakpoint[value]} !important;
|
|
|
|
* }
|
|
|
|
* }
|
|
|
|
*/
|
|
|
|
[key: string]: string;
|
|
|
|
}
|
|
|
|
|
2023-03-01 10:19:01 +00:00
|
|
|
/**
|
|
|
|
* Defines valid properties in OverlayPanel component.
|
|
|
|
*/
|
2022-09-06 12:03:37 +00:00
|
|
|
export interface OverlayPanelProps {
|
|
|
|
/**
|
|
|
|
* Enables to hide the overlay when outside is clicked.
|
2023-03-01 10:19:01 +00:00
|
|
|
* @defaultValue true
|
2022-09-06 12:03:37 +00:00
|
|
|
*/
|
|
|
|
dismissable?: boolean;
|
|
|
|
/**
|
|
|
|
* When enabled, displays a close icon at top right corner.
|
2023-03-01 10:19:01 +00:00
|
|
|
* @defaultValue false
|
2022-09-06 12:03:37 +00:00
|
|
|
*/
|
|
|
|
showCloseIcon?: boolean;
|
|
|
|
/**
|
|
|
|
* A valid query selector or an HTMLElement to specify where the overlay gets attached.
|
2023-03-01 10:19:01 +00:00
|
|
|
* @defaultValue body
|
2022-09-06 12:03:37 +00:00
|
|
|
*/
|
2023-03-01 10:19:01 +00:00
|
|
|
appendTo?: 'body' | 'self' | string | undefined | HTMLElement;
|
2022-09-06 12:03:37 +00:00
|
|
|
/**
|
|
|
|
* Base zIndex value to use in layering.
|
2023-03-01 10:19:01 +00:00
|
|
|
* @defaultValue 0
|
2022-09-06 12:03:37 +00:00
|
|
|
*/
|
|
|
|
baseZIndex?: number;
|
|
|
|
/**
|
|
|
|
* Whether to automatically manage layering.
|
2023-03-01 10:19:01 +00:00
|
|
|
* @defaultValue true
|
2022-09-06 12:03:37 +00:00
|
|
|
*/
|
|
|
|
autoZIndex?: boolean;
|
|
|
|
/**
|
|
|
|
* Object literal to define widths per screen size.
|
|
|
|
*/
|
|
|
|
breakpoints?: OverlayPanelBreakpoints;
|
|
|
|
}
|
|
|
|
|
2023-03-01 10:19:01 +00:00
|
|
|
/**
|
|
|
|
* Defines valid slots in OverlayPanel component.
|
|
|
|
*/
|
2022-09-06 12:03:37 +00:00
|
|
|
export interface OverlayPanelSlots {
|
|
|
|
/**
|
|
|
|
* Custom content template.
|
|
|
|
*/
|
2023-03-01 10:19:01 +00:00
|
|
|
default(): VNode[];
|
2022-09-06 12:03:37 +00:00
|
|
|
}
|
|
|
|
|
2023-03-01 10:19:01 +00:00
|
|
|
/**
|
|
|
|
* Defines valid emits in OverlayPanel component.
|
|
|
|
*/
|
|
|
|
export interface OverlayPanelEmits {
|
2022-09-06 12:03:37 +00:00
|
|
|
/**
|
|
|
|
* Callback to invoke when the overlay is shown.
|
|
|
|
*/
|
2023-03-01 10:19:01 +00:00
|
|
|
show(): void;
|
2022-09-06 12:03:37 +00:00
|
|
|
/**
|
|
|
|
* Callback to invoke when the overlay is hidden.
|
|
|
|
*/
|
2023-03-01 10:19:01 +00:00
|
|
|
hide(): void;
|
|
|
|
}
|
2022-09-06 12:03:37 +00:00
|
|
|
|
2023-03-01 10:19:01 +00:00
|
|
|
/**
|
|
|
|
* **PrimeVue - OverlayPanel**
|
|
|
|
*
|
|
|
|
* _OverlayPanel, also known as Popover, is a container component that can overlay other components on page._
|
|
|
|
*
|
|
|
|
* [Live Demo](https://www.primevue.org/overlaypanel/)
|
|
|
|
* --- ---
|
|
|
|
* 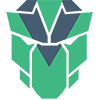
|
|
|
|
*
|
|
|
|
* @group Component
|
|
|
|
*/
|
2023-03-01 14:48:23 +00:00
|
|
|
declare class OverlayPanel extends ClassComponent<OverlayPanelProps, OverlayPanelSlots, OverlayPanelEmits> {
|
2022-09-06 12:03:37 +00:00
|
|
|
/**
|
|
|
|
* Toggles the visibility of the overlay.
|
|
|
|
* @param {Event} event - Browser event.
|
2022-12-08 11:04:25 +00:00
|
|
|
* @param {*} [target] - Optional target if event.currentTarget should not be used.
|
2022-09-06 12:03:37 +00:00
|
|
|
*
|
|
|
|
* @memberof OverlayPanel
|
|
|
|
*/
|
2023-03-01 10:19:01 +00:00
|
|
|
toggle(event: Event, target?: any): void;
|
2022-09-06 12:03:37 +00:00
|
|
|
/**
|
|
|
|
* Shows the overlay.
|
|
|
|
* @param {Event} event - Browser event.
|
|
|
|
* @param {*} [target] - Optional target if event.currentTarget should not be used.
|
|
|
|
*
|
|
|
|
* @memberof OverlayPanel
|
|
|
|
*/
|
2023-03-01 10:19:01 +00:00
|
|
|
show(event: Event, target?: any): void;
|
2022-09-06 12:03:37 +00:00
|
|
|
/**
|
|
|
|
* Hides the overlay.
|
|
|
|
*
|
|
|
|
* @memberof OverlayPanel
|
|
|
|
*/
|
2023-03-01 10:19:01 +00:00
|
|
|
hide(): void;
|
2022-09-06 12:03:37 +00:00
|
|
|
}
|
|
|
|
|
|
|
|
declare module '@vue/runtime-core' {
|
|
|
|
interface GlobalComponents {
|
2022-09-14 11:26:01 +00:00
|
|
|
OverlayPanel: GlobalComponentConstructor<OverlayPanel>;
|
2022-09-06 12:03:37 +00:00
|
|
|
}
|
|
|
|
}
|
|
|
|
|
|
|
|
export default OverlayPanel;
|