2023-03-01 13:12:09 +00:00
|
|
|
/**
|
|
|
|
*
|
|
|
|
* Chip represents people using icons, labels and images.
|
|
|
|
*
|
|
|
|
* [Live Demo](https://www.primevue.org/chip)
|
|
|
|
*
|
|
|
|
* @module chip
|
|
|
|
*
|
|
|
|
*/
|
2022-09-06 12:03:37 +00:00
|
|
|
import { VNode } from 'vue';
|
|
|
|
import { ClassComponent, GlobalComponentConstructor } from '../ts-helpers';
|
|
|
|
|
2023-03-01 13:12:09 +00:00
|
|
|
/**
|
|
|
|
* Defines valid properties in Chip component.
|
|
|
|
*/
|
2022-09-06 12:03:37 +00:00
|
|
|
export interface ChipProps {
|
|
|
|
/**
|
|
|
|
* Defines the text to display.
|
|
|
|
*/
|
|
|
|
label?: string;
|
|
|
|
/**
|
|
|
|
* Defines the icon to display.
|
|
|
|
*/
|
|
|
|
icon?: string;
|
|
|
|
/**
|
|
|
|
* Defines the image to display.
|
|
|
|
*/
|
|
|
|
image?: string;
|
|
|
|
/**
|
|
|
|
* Whether to display a remove icon.
|
2023-03-01 13:12:09 +00:00
|
|
|
* @defaultValue false
|
2022-09-06 12:03:37 +00:00
|
|
|
*/
|
|
|
|
removable?: boolean;
|
|
|
|
/**
|
|
|
|
* Icon of the remove element.
|
2023-03-01 13:12:09 +00:00
|
|
|
* @defaultValue pi pi-times-circle
|
2022-09-06 12:03:37 +00:00
|
|
|
*/
|
|
|
|
removeIcon?: string;
|
|
|
|
}
|
|
|
|
|
2023-03-01 13:12:09 +00:00
|
|
|
/**
|
|
|
|
* Defines valid slots in Chip component.
|
|
|
|
*/
|
2022-09-06 12:03:37 +00:00
|
|
|
export interface ChipSlots {
|
|
|
|
/**
|
|
|
|
* Content can easily be customized with the default slot instead of using the built-in modes.
|
|
|
|
*/
|
2023-03-01 13:12:09 +00:00
|
|
|
default(): VNode[];
|
2022-09-06 12:03:37 +00:00
|
|
|
}
|
|
|
|
|
2023-03-01 13:12:09 +00:00
|
|
|
/**
|
|
|
|
* Defines valid properties in Chip component.
|
|
|
|
*/
|
|
|
|
export interface ChipEmits {
|
2022-09-06 12:03:37 +00:00
|
|
|
/**
|
|
|
|
* Callback to invoke when a chip is removed.
|
|
|
|
* @param {Event} event - Browser event.
|
|
|
|
*/
|
2023-03-01 13:12:09 +00:00
|
|
|
remove(event: Event): void;
|
|
|
|
}
|
2022-09-06 12:03:37 +00:00
|
|
|
|
2023-03-01 13:12:09 +00:00
|
|
|
/**
|
|
|
|
* **PrimeVue - Chip**
|
|
|
|
*
|
|
|
|
* _Chip represents people using icons, labels and images._
|
|
|
|
*
|
|
|
|
* [Live Demo](https://www.primevue.org/chip/)
|
|
|
|
* --- ---
|
|
|
|
* 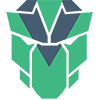
|
|
|
|
*
|
|
|
|
* @group Component
|
|
|
|
*/
|
2022-09-14 11:26:01 +00:00
|
|
|
declare class Chip extends ClassComponent<ChipProps, ChipSlots, ChipEmits> {}
|
2022-09-06 12:03:37 +00:00
|
|
|
|
|
|
|
declare module '@vue/runtime-core' {
|
|
|
|
interface GlobalComponents {
|
2022-09-14 11:26:01 +00:00
|
|
|
Chip: GlobalComponentConstructor<Chip>;
|
2022-09-06 12:03:37 +00:00
|
|
|
}
|
|
|
|
}
|
|
|
|
|
|
|
|
export default Chip;
|