2023-03-01 12:59:47 +00:00
|
|
|
/**
|
|
|
|
*
|
|
|
|
* Message groups a collection of contents in tabs.
|
|
|
|
*
|
|
|
|
* [Live Demo](https://www.primevue.org/message/)
|
|
|
|
*
|
|
|
|
* @module message
|
|
|
|
*
|
|
|
|
*/
|
2023-08-02 14:07:22 +00:00
|
|
|
import { ButtonHTMLAttributes, TransitionProps, VNode } from 'vue';
|
2023-07-06 11:17:08 +00:00
|
|
|
import { ComponentHooks } from '../basecomponent';
|
2023-09-05 08:50:46 +00:00
|
|
|
import { PassThroughOptions } from '../passthrough';
|
2024-05-16 10:50:43 +00:00
|
|
|
import { DefineComponent, DesignToken, EmitFn, GlobalComponentConstructor, HintedString, PassThrough } from '../ts-helpers';
|
2022-09-06 12:03:37 +00:00
|
|
|
|
2023-12-05 07:06:55 +00:00
|
|
|
export declare type MessagePassThroughOptionType<T = any> = MessagePassThroughAttributes | ((options: MessagePassThroughMethodOptions<T>) => MessagePassThroughAttributes | string) | string | null | undefined;
|
2023-04-28 11:56:49 +00:00
|
|
|
|
2023-12-05 07:06:55 +00:00
|
|
|
export declare type MessagePassThroughTransitionType<T = any> = TransitionProps | ((options: MessagePassThroughMethodOptions<T>) => TransitionProps) | undefined;
|
2023-08-02 14:07:22 +00:00
|
|
|
|
2023-04-28 11:56:49 +00:00
|
|
|
/**
|
|
|
|
* Custom passthrough(pt) option method.
|
|
|
|
*/
|
2023-12-05 07:06:55 +00:00
|
|
|
export interface MessagePassThroughMethodOptions<T = any> {
|
2023-09-05 06:50:04 +00:00
|
|
|
/**
|
|
|
|
* Defines instance.
|
|
|
|
*/
|
2023-07-06 12:01:33 +00:00
|
|
|
instance: any;
|
2023-09-05 06:50:04 +00:00
|
|
|
/**
|
|
|
|
* Defines valid properties.
|
|
|
|
*/
|
2023-04-28 11:56:49 +00:00
|
|
|
props: MessageProps;
|
2023-09-05 06:50:04 +00:00
|
|
|
/**
|
|
|
|
* Defines current inline state.
|
|
|
|
*/
|
2023-04-28 11:56:49 +00:00
|
|
|
state: MessageState;
|
2023-12-05 07:06:55 +00:00
|
|
|
/**
|
|
|
|
* Defines parent instance.
|
|
|
|
*/
|
|
|
|
parent: T;
|
2023-09-05 08:50:46 +00:00
|
|
|
/**
|
|
|
|
* Defines passthrough(pt) options in global config.
|
|
|
|
*/
|
|
|
|
global: object | undefined;
|
2023-04-28 11:56:49 +00:00
|
|
|
}
|
|
|
|
|
|
|
|
/**
|
|
|
|
* Custom passthrough(pt) options.
|
|
|
|
* @see {@link MessageProps.pt}
|
|
|
|
*/
|
2023-12-05 07:06:55 +00:00
|
|
|
export interface MessagePassThroughOptions<T = any> {
|
2023-04-28 11:56:49 +00:00
|
|
|
/**
|
2024-05-15 09:24:26 +00:00
|
|
|
* Used to pass attributes to the root's DOM element.
|
2023-04-28 11:56:49 +00:00
|
|
|
*/
|
2023-12-05 07:06:55 +00:00
|
|
|
root?: MessagePassThroughOptionType<T>;
|
2023-04-28 11:56:49 +00:00
|
|
|
/**
|
2024-05-15 09:24:26 +00:00
|
|
|
* Used to pass attributes to the content's DOM element.
|
2023-04-28 11:56:49 +00:00
|
|
|
*/
|
2024-04-30 08:15:34 +00:00
|
|
|
content?: MessagePassThroughOptionType<T>;
|
2023-04-28 11:56:49 +00:00
|
|
|
/**
|
2024-05-15 09:24:26 +00:00
|
|
|
* Used to pass attributes to the icon's DOM element.
|
2023-04-28 11:56:49 +00:00
|
|
|
*/
|
2023-12-05 07:06:55 +00:00
|
|
|
icon?: MessagePassThroughOptionType<T>;
|
2023-04-28 11:56:49 +00:00
|
|
|
/**
|
2024-05-15 09:24:26 +00:00
|
|
|
* Used to pass attributes to the text's DOM element.
|
2023-04-28 11:56:49 +00:00
|
|
|
*/
|
2023-12-05 07:06:55 +00:00
|
|
|
text?: MessagePassThroughOptionType<T>;
|
2023-04-28 11:56:49 +00:00
|
|
|
/**
|
2024-05-15 09:24:26 +00:00
|
|
|
* Used to pass attributes to the button's DOM element.
|
2023-07-19 08:21:09 +00:00
|
|
|
* @deprecated since v3.30.2. Use 'closeButton' option.
|
2023-04-28 11:56:49 +00:00
|
|
|
*/
|
2023-12-05 07:06:55 +00:00
|
|
|
button?: MessagePassThroughOptionType<T>;
|
2023-07-19 08:21:09 +00:00
|
|
|
/**
|
2024-05-15 09:24:26 +00:00
|
|
|
* Used to pass attributes to the button's DOM element.
|
2023-07-19 08:21:09 +00:00
|
|
|
*/
|
2023-12-05 07:06:55 +00:00
|
|
|
closeButton?: MessagePassThroughOptionType<T>;
|
2023-04-28 11:56:49 +00:00
|
|
|
/**
|
2024-05-15 09:24:26 +00:00
|
|
|
* Used to pass attributes to the button icon's DOM element.
|
2023-07-19 08:21:09 +00:00
|
|
|
* @deprecated since v3.30.2. Use 'closeIcon' option.
|
2023-04-28 11:56:49 +00:00
|
|
|
*/
|
2023-12-05 07:06:55 +00:00
|
|
|
buttonIcon?: MessagePassThroughOptionType<T>;
|
2023-07-19 08:21:09 +00:00
|
|
|
/**
|
2024-05-15 09:24:26 +00:00
|
|
|
* Used to pass attributes to the button icon's DOM element.
|
2023-07-19 08:21:09 +00:00
|
|
|
*/
|
2023-12-05 07:06:55 +00:00
|
|
|
closeIcon?: MessagePassThroughOptionType<T>;
|
2023-07-06 11:09:01 +00:00
|
|
|
/**
|
2023-11-07 06:16:39 +00:00
|
|
|
* Used to manage all lifecycle hooks.
|
2023-07-06 11:09:01 +00:00
|
|
|
* @see {@link BaseComponent.ComponentHooks}
|
|
|
|
*/
|
|
|
|
hooks?: ComponentHooks;
|
2023-08-02 12:01:26 +00:00
|
|
|
/**
|
|
|
|
* Used to control Vue Transition API.
|
|
|
|
*/
|
2023-12-05 07:06:55 +00:00
|
|
|
transition?: MessagePassThroughTransitionType<T>;
|
2023-04-28 11:56:49 +00:00
|
|
|
}
|
|
|
|
|
|
|
|
/**
|
|
|
|
* Custom passthrough attributes for each DOM elements
|
|
|
|
*/
|
|
|
|
export interface MessagePassThroughAttributes {
|
|
|
|
[key: string]: any;
|
|
|
|
}
|
|
|
|
|
|
|
|
/**
|
|
|
|
* Defines current inline state in Message component.
|
|
|
|
*/
|
|
|
|
export interface MessageState {
|
|
|
|
/**
|
|
|
|
* Current visible state as a boolean.
|
|
|
|
* @defaultValue false
|
|
|
|
*/
|
|
|
|
visible: boolean;
|
|
|
|
}
|
|
|
|
|
2023-03-01 12:59:47 +00:00
|
|
|
/**
|
|
|
|
* Defines valid properties in Message component.
|
|
|
|
*/
|
2022-09-06 12:03:37 +00:00
|
|
|
export interface MessageProps {
|
|
|
|
/**
|
|
|
|
* Severity level of the message.
|
2023-03-08 10:51:52 +00:00
|
|
|
* @defaultValue info
|
2022-09-06 12:03:37 +00:00
|
|
|
*/
|
2024-02-23 08:38:50 +00:00
|
|
|
severity?: HintedString<'success' | 'info' | 'warn' | 'error' | 'secondary' | 'contrast'> | undefined;
|
2022-09-06 12:03:37 +00:00
|
|
|
/**
|
|
|
|
* Whether the message can be closed manually using the close icon.
|
2023-03-01 12:59:47 +00:00
|
|
|
* @defaultValue true
|
2022-09-06 12:03:37 +00:00
|
|
|
*/
|
|
|
|
closable?: boolean | undefined;
|
|
|
|
/**
|
|
|
|
* When enabled, message is not removed automatically.
|
2023-03-01 12:59:47 +00:00
|
|
|
* @defaultValue true
|
2022-09-06 12:03:37 +00:00
|
|
|
*/
|
|
|
|
sticky?: boolean | undefined;
|
|
|
|
/**
|
|
|
|
* Delay in milliseconds to close the message automatically.
|
2023-03-01 12:59:47 +00:00
|
|
|
* @defaultValue 3000
|
2022-09-06 12:03:37 +00:00
|
|
|
*/
|
|
|
|
life?: number | undefined;
|
|
|
|
/**
|
|
|
|
* Display a custom icon for the message.
|
|
|
|
*/
|
|
|
|
icon?: string | undefined;
|
2022-12-08 11:04:25 +00:00
|
|
|
/**
|
|
|
|
* Icon to display in the message close button.
|
2023-04-18 10:51:10 +00:00
|
|
|
* @deprecated since v3.27.0. Use 'closeicon' slot.
|
2022-12-08 11:04:25 +00:00
|
|
|
*/
|
|
|
|
closeIcon?: string | undefined;
|
|
|
|
/**
|
2023-08-01 14:01:12 +00:00
|
|
|
* Used to pass all properties of the HTMLButtonElement to the close button.
|
2022-12-08 11:04:25 +00:00
|
|
|
*/
|
|
|
|
closeButtonProps?: ButtonHTMLAttributes | undefined;
|
2024-04-02 08:24:31 +00:00
|
|
|
/**
|
|
|
|
* It generates scoped CSS variables using design tokens for the component.
|
|
|
|
*/
|
|
|
|
dt?: DesignToken<any>;
|
2023-04-28 13:40:36 +00:00
|
|
|
/**
|
2023-08-01 14:01:12 +00:00
|
|
|
* Used to pass attributes to DOM elements inside the component.
|
2023-04-28 13:40:36 +00:00
|
|
|
* @type {MessagePassThroughOptions}
|
|
|
|
*/
|
2023-09-05 11:28:04 +00:00
|
|
|
pt?: PassThrough<MessagePassThroughOptions>;
|
2023-09-05 08:50:46 +00:00
|
|
|
/**
|
|
|
|
* Used to configure passthrough(pt) options of the component.
|
|
|
|
* @type {PassThroughOptions}
|
|
|
|
*/
|
|
|
|
ptOptions?: PassThroughOptions;
|
2023-05-25 13:22:28 +00:00
|
|
|
/**
|
|
|
|
* When enabled, it removes component related styles in the core.
|
|
|
|
* @defaultValue false
|
|
|
|
*/
|
|
|
|
unstyled?: boolean;
|
2022-09-06 12:03:37 +00:00
|
|
|
}
|
|
|
|
|
2023-03-01 12:59:47 +00:00
|
|
|
/**
|
|
|
|
* Defines valid slots in Message slots.
|
|
|
|
*/
|
2022-09-06 12:03:37 +00:00
|
|
|
export interface MessageSlots {
|
|
|
|
/**
|
|
|
|
* Default custom slot.
|
|
|
|
*/
|
2023-03-01 12:59:47 +00:00
|
|
|
default(): VNode[];
|
2023-04-07 14:15:21 +00:00
|
|
|
/**
|
|
|
|
* Custom message icon template.
|
2023-08-17 07:16:25 +00:00
|
|
|
* @param {Object} scope - messageicon slot's params.
|
2023-04-07 14:15:21 +00:00
|
|
|
*/
|
2023-04-18 10:51:10 +00:00
|
|
|
messageicon(scope: {
|
|
|
|
/**
|
|
|
|
* Style class of the item icon element.
|
|
|
|
*/
|
|
|
|
class: any;
|
|
|
|
}): VNode[];
|
2023-04-07 14:15:21 +00:00
|
|
|
/**
|
|
|
|
* Custom close icon template.
|
2023-08-17 07:16:25 +00:00
|
|
|
* @param {Object} scope - closeicon slot's params.
|
2023-04-07 14:15:21 +00:00
|
|
|
*/
|
2023-04-18 10:51:10 +00:00
|
|
|
closeicon(scope: {
|
|
|
|
/**
|
|
|
|
* Style class of the item icon element.
|
|
|
|
*/
|
|
|
|
class: any;
|
|
|
|
}): VNode[];
|
2023-09-19 11:22:25 +00:00
|
|
|
/**
|
|
|
|
* Custom container slot.
|
|
|
|
* @param {Object} scope - container slot's params.
|
|
|
|
*/
|
|
|
|
container(scope: {
|
|
|
|
/**
|
|
|
|
* Close message function.
|
2023-10-31 12:52:10 +00:00
|
|
|
* @deprecated since v3.39.0. Use 'closeCallback' property instead.
|
2023-09-19 11:22:25 +00:00
|
|
|
*/
|
|
|
|
onClose: () => void;
|
2023-10-31 12:52:10 +00:00
|
|
|
/**
|
|
|
|
* Close message function.
|
|
|
|
*/
|
|
|
|
closeCallback: () => void;
|
2023-09-19 11:22:25 +00:00
|
|
|
}): VNode[];
|
2022-09-06 12:03:37 +00:00
|
|
|
}
|
|
|
|
|
2023-03-01 12:59:47 +00:00
|
|
|
/**
|
|
|
|
* Defines valid emits in Message component.
|
|
|
|
*/
|
2024-05-16 14:05:43 +00:00
|
|
|
export interface MessageEmitsOptions {
|
2022-09-06 12:03:37 +00:00
|
|
|
/**
|
|
|
|
* Callback to invoke when a message is closed.
|
|
|
|
* @param {Event} event - Browser event.
|
|
|
|
*/
|
2023-03-01 12:59:47 +00:00
|
|
|
close(event: Event): void;
|
2024-01-18 06:44:22 +00:00
|
|
|
/**
|
|
|
|
* Callback to invoke when the message's timeout is over.
|
|
|
|
*/
|
|
|
|
'life-end'(): void;
|
2023-03-01 12:59:47 +00:00
|
|
|
}
|
2022-09-06 12:03:37 +00:00
|
|
|
|
2024-05-16 10:50:43 +00:00
|
|
|
export declare type MessageEmits = EmitFn<MessageEmitsOptions>;
|
|
|
|
|
2023-03-01 12:59:47 +00:00
|
|
|
/**
|
|
|
|
* **PrimeVue - Message**
|
|
|
|
*
|
|
|
|
* _Messages is used to display inline messages with various severities._
|
|
|
|
*
|
|
|
|
* [Live Demo](https://www.primevue.org/message/)
|
|
|
|
* --- ---
|
2023-03-03 10:55:20 +00:00
|
|
|
* 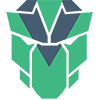
|
2023-03-01 12:59:47 +00:00
|
|
|
*
|
|
|
|
* @group Component
|
|
|
|
*
|
|
|
|
*/
|
2024-05-16 10:50:43 +00:00
|
|
|
declare const Message: DefineComponent<MessageProps, MessageSlots, MessageEmits>;
|
2022-09-06 12:03:37 +00:00
|
|
|
|
2024-03-14 22:58:11 +00:00
|
|
|
declare module 'vue' {
|
|
|
|
export interface GlobalComponents {
|
2024-05-16 10:50:43 +00:00
|
|
|
Message: GlobalComponentConstructor<MessageProps, MessageSlots, MessageEmits>;
|
2022-09-06 12:03:37 +00:00
|
|
|
}
|
|
|
|
}
|
|
|
|
|
|
|
|
export default Message;
|