2023-03-02 11:26:12 +00:00
|
|
|
/**
|
|
|
|
*
|
2024-05-21 12:38:25 +00:00
|
|
|
* StyleClass manages CSS classes declaratively to during enter/leave animations or just to toggle classes on an element.
|
2023-03-02 11:26:12 +00:00
|
|
|
*
|
2023-03-03 14:17:03 +00:00
|
|
|
* [Live Demo](https://primevue.org/styleclass)
|
2023-03-02 14:25:05 +00:00
|
|
|
*
|
|
|
|
* @module styleclass
|
2024-05-08 11:40:44 +00:00
|
|
|
*
|
2023-03-02 11:26:12 +00:00
|
|
|
*/
|
|
|
|
import { DirectiveBinding, ObjectDirective } from 'vue';
|
2023-07-06 11:09:01 +00:00
|
|
|
import { DirectiveHooks } from '../basedirective';
|
2023-09-05 08:50:46 +00:00
|
|
|
import { PassThroughOptions } from '../passthrough';
|
2024-04-02 08:24:31 +00:00
|
|
|
import { DesignToken, HintedString, PassThrough } from '../ts-helpers';
|
2022-09-06 12:03:37 +00:00
|
|
|
|
2023-06-22 13:55:50 +00:00
|
|
|
/**
|
2023-06-23 09:47:31 +00:00
|
|
|
* Defines options of StyleClass.
|
2023-06-22 13:55:50 +00:00
|
|
|
*/
|
2023-06-23 09:47:31 +00:00
|
|
|
export interface StyleClassOptions {
|
2023-06-22 13:55:50 +00:00
|
|
|
/**
|
2023-06-23 09:47:31 +00:00
|
|
|
* Selector to define the target element. Available selectors are '@next', '@prev', '@parent' and '@grandparent'.
|
2023-06-22 13:55:50 +00:00
|
|
|
*/
|
2024-02-23 08:38:50 +00:00
|
|
|
selector?: HintedString<'@next' | '@prev' | '@parent' | '@grandparent'> | undefined;
|
2023-06-22 13:55:50 +00:00
|
|
|
/**
|
2023-06-23 09:47:31 +00:00
|
|
|
* Style class to add when item begins to get displayed.
|
2023-11-22 13:19:12 +00:00
|
|
|
* @deprecated since v3.41.0. Use 'enterFromClass' option instead.
|
2023-06-22 13:55:50 +00:00
|
|
|
*/
|
2023-10-13 10:32:57 +00:00
|
|
|
enterClass?: string | undefined;
|
2023-11-22 13:19:12 +00:00
|
|
|
/**
|
|
|
|
* Style class to add when item begins to get displayed.
|
|
|
|
*/
|
|
|
|
enterFromClass?: string | undefined;
|
2023-06-22 13:55:50 +00:00
|
|
|
/**
|
2023-06-23 09:47:31 +00:00
|
|
|
* Style class to add during enter animation.
|
2023-06-22 13:55:50 +00:00
|
|
|
*/
|
2023-10-13 10:32:57 +00:00
|
|
|
enterActiveClass?: string | undefined;
|
2023-06-22 13:55:50 +00:00
|
|
|
/**
|
2023-06-23 09:47:31 +00:00
|
|
|
* Style class to add when item begins to get displayed.
|
2023-06-22 13:55:50 +00:00
|
|
|
*/
|
2023-10-13 10:32:57 +00:00
|
|
|
enterToClass?: string | undefined;
|
2023-06-22 13:55:50 +00:00
|
|
|
/**
|
2023-06-23 09:47:31 +00:00
|
|
|
* Style class to add when item begins to get hidden.
|
2023-11-22 13:19:12 +00:00
|
|
|
* @deprecated since v3.41.0. Use 'leaveFromClass' option instead.
|
2023-06-22 13:55:50 +00:00
|
|
|
*/
|
2023-10-13 10:32:57 +00:00
|
|
|
leaveClass?: string | undefined;
|
2023-11-22 13:19:12 +00:00
|
|
|
/**
|
|
|
|
* Style class to add when item begins to get hidden.
|
|
|
|
*/
|
|
|
|
leaveFromClass?: string | undefined;
|
2023-06-22 13:55:50 +00:00
|
|
|
/**
|
2023-06-23 09:47:31 +00:00
|
|
|
* Style class to add during leave animation.
|
2023-06-22 13:55:50 +00:00
|
|
|
*/
|
2023-10-13 10:32:57 +00:00
|
|
|
leaveActiveClass?: string | undefined;
|
2023-06-22 13:55:50 +00:00
|
|
|
/**
|
2023-06-23 09:47:31 +00:00
|
|
|
* Style class to add when leave animation is completed.
|
2023-06-22 13:55:50 +00:00
|
|
|
*/
|
2023-10-13 10:32:57 +00:00
|
|
|
leaveToClass?: string | undefined;
|
2023-06-23 09:47:31 +00:00
|
|
|
/**
|
|
|
|
* Whether to trigger leave animation when outside of the element is clicked.
|
|
|
|
* @defaultValue false
|
|
|
|
*/
|
|
|
|
hideOnOutsideClick?: boolean | undefined;
|
|
|
|
/**
|
|
|
|
* Adds or removes a class when no enter-leave animation is required.
|
|
|
|
*/
|
|
|
|
toggleClass?: string | undefined;
|
2024-04-02 08:24:31 +00:00
|
|
|
/**
|
|
|
|
* It generates scoped CSS variables using design tokens for the component.
|
|
|
|
*/
|
|
|
|
dt?: DesignToken<any>;
|
2023-06-23 09:47:31 +00:00
|
|
|
/**
|
2023-08-01 14:01:12 +00:00
|
|
|
* Used to pass attributes to DOM elements inside the component.
|
2023-06-23 14:51:42 +00:00
|
|
|
* @type {StyleClassDirectivePassThroughOptions}
|
2023-06-23 09:47:31 +00:00
|
|
|
*/
|
2023-09-05 11:28:04 +00:00
|
|
|
pt?: PassThrough<StyleClassDirectivePassThroughOptions>;
|
2023-09-05 08:50:46 +00:00
|
|
|
/**
|
|
|
|
* Used to configure passthrough(pt) options of the component.
|
|
|
|
* @type {PassThroughOptions}
|
|
|
|
*/
|
|
|
|
ptOptions?: PassThroughOptions;
|
2023-06-22 13:55:50 +00:00
|
|
|
}
|
|
|
|
|
|
|
|
/**
|
2023-06-23 09:47:31 +00:00
|
|
|
* Custom passthrough(pt) directive options.
|
2023-06-22 13:55:50 +00:00
|
|
|
*/
|
2023-06-23 14:51:42 +00:00
|
|
|
export interface StyleClassDirectivePassThroughOptions {
|
2023-06-22 13:55:50 +00:00
|
|
|
/**
|
2023-11-07 06:16:39 +00:00
|
|
|
* Used to manage all lifecycle hooks.
|
2023-07-06 11:09:01 +00:00
|
|
|
* @see {@link BaseDirective.DirectiveHooks}
|
2023-06-22 13:55:50 +00:00
|
|
|
*/
|
2023-07-06 11:09:01 +00:00
|
|
|
hooks?: DirectiveHooks;
|
2023-03-02 11:26:12 +00:00
|
|
|
}
|
|
|
|
|
|
|
|
/**
|
|
|
|
* Binding of StyleClass directive.
|
|
|
|
*/
|
|
|
|
export interface StyleClassDirectiveBinding extends Omit<DirectiveBinding, 'modifiers' | 'value'> {
|
|
|
|
/**
|
|
|
|
* Value of the StyleClass.
|
|
|
|
*/
|
|
|
|
value?: StyleClassOptions | undefined;
|
|
|
|
}
|
|
|
|
|
|
|
|
/**
|
|
|
|
* **PrimeVue - StyleClass**
|
|
|
|
*
|
|
|
|
* _StyleClass manages css classes declaratively to during enter/leave animations or just to toggle classes on an element._
|
|
|
|
*
|
|
|
|
* [Live Demo](https://www.primevue.org/styleclass/)
|
|
|
|
* --- ---
|
2023-03-03 10:55:20 +00:00
|
|
|
* 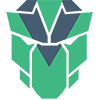
|
2023-03-02 11:26:12 +00:00
|
|
|
*
|
|
|
|
*/
|
2022-09-06 12:03:37 +00:00
|
|
|
declare const StyleClass: ObjectDirective;
|
|
|
|
|
|
|
|
export default StyleClass;
|