2023-03-02 11:03:01 +00:00
|
|
|
/**
|
|
|
|
*
|
|
|
|
* Tooltip directive provides advisory information for a component.
|
|
|
|
*
|
2023-03-03 14:17:03 +00:00
|
|
|
* [Live Demo](https://primevue.org/tooltip)
|
2023-03-02 14:25:05 +00:00
|
|
|
*
|
|
|
|
* @module tooltip
|
|
|
|
*
|
2023-03-02 11:03:01 +00:00
|
|
|
*/
|
2023-03-02 09:31:36 +00:00
|
|
|
import { DirectiveBinding, ObjectDirective } from 'vue';
|
2022-09-06 12:03:37 +00:00
|
|
|
|
2023-06-21 13:58:32 +00:00
|
|
|
/**
|
|
|
|
* Custom passthrough(pt) hooks options.
|
|
|
|
*/
|
|
|
|
export interface TooltipPassThroughHooksOptions {
|
|
|
|
/**
|
|
|
|
* Called before bound element's attributes or event listeners are applied.
|
|
|
|
*/
|
|
|
|
created?: DirectiveBinding;
|
|
|
|
/**
|
|
|
|
* Called right before the element is inserted into the DOM.
|
|
|
|
*/
|
|
|
|
beforeMount?: DirectiveBinding;
|
|
|
|
/**
|
|
|
|
* Called when the bound element's parent component and all its children are mounted.
|
|
|
|
*/
|
|
|
|
mounted?: DirectiveBinding;
|
|
|
|
/**
|
|
|
|
* Called before the parent component is updated.
|
|
|
|
*/
|
|
|
|
beforeUpdate?: DirectiveBinding;
|
|
|
|
/**
|
|
|
|
* Called after the parent component and all of its children have updated all of its children have updated.
|
|
|
|
*/
|
|
|
|
updated?: DirectiveBinding;
|
|
|
|
/**
|
|
|
|
* Called before the parent component is unmounted.
|
|
|
|
*/
|
|
|
|
beforeUnmount?: DirectiveBinding;
|
|
|
|
/**
|
|
|
|
* Called when the parent component is unmounted.
|
|
|
|
*/
|
|
|
|
unmounted?: DirectiveBinding;
|
|
|
|
}
|
|
|
|
|
|
|
|
/**
|
|
|
|
* Custom passthrough(pt) css options.
|
|
|
|
*/
|
|
|
|
export interface TooltipPassThroughCSSOptions {
|
|
|
|
/**
|
|
|
|
* Style class of the element.
|
|
|
|
*/
|
|
|
|
class?: any;
|
|
|
|
/**
|
|
|
|
* Inline style of the element.
|
|
|
|
*/
|
|
|
|
style?: any;
|
|
|
|
}
|
|
|
|
|
|
|
|
export interface TooltipPassThroughDirectiveOptions {
|
|
|
|
/**
|
|
|
|
* Uses to pass attributes to the life cycle hooks.
|
|
|
|
* @see {@link TooltipPassThroughHooksOptions}
|
|
|
|
*/
|
|
|
|
hooks?: TooltipPassThroughHooksOptions;
|
|
|
|
/**
|
|
|
|
* Uses to pass attributes to the styles.
|
|
|
|
* @see {@link TooltipPassThroughCSSOptions}
|
|
|
|
*/
|
|
|
|
css?: TooltipPassThroughCSSOptions;
|
|
|
|
}
|
|
|
|
|
|
|
|
/**
|
|
|
|
* Custom passthrough(pt) options.
|
|
|
|
* @see {@link TooltipOptions.pt}
|
|
|
|
*/
|
|
|
|
export interface TooltipPassThroughOptions {
|
|
|
|
/**
|
|
|
|
* Uses to pass attributes to the root's DOM element.
|
|
|
|
* @see {@link TooltipPassThroughDirectiveOptions}
|
|
|
|
*/
|
|
|
|
root?: TooltipPassThroughDirectiveOptions;
|
|
|
|
/**
|
|
|
|
* Uses to pass attributes to the text's DOM element.
|
|
|
|
* @see {@link TooltipPassThroughDirectiveOptions}
|
|
|
|
*/
|
|
|
|
text?: TooltipPassThroughDirectiveOptions;
|
|
|
|
/**
|
|
|
|
* Uses to pass attributes to the arrow's DOM element.
|
|
|
|
* @see {@link TooltipPassThroughDirectiveOptions}
|
|
|
|
*/
|
|
|
|
arrow?: TooltipPassThroughDirectiveOptions;
|
|
|
|
}
|
|
|
|
|
2023-03-02 10:54:16 +00:00
|
|
|
/**
|
|
|
|
* Defines options of Tooltip.
|
|
|
|
*/
|
2023-03-02 09:53:55 +00:00
|
|
|
export interface TooltipOptions {
|
|
|
|
/**
|
|
|
|
* Text of the tooltip.
|
|
|
|
*/
|
|
|
|
value?: string | undefined;
|
2023-03-01 14:57:18 +00:00
|
|
|
/**
|
|
|
|
* When present, it specifies that the component should be disabled.
|
|
|
|
* @defaultValue false
|
|
|
|
*/
|
|
|
|
disabled?: boolean | undefined;
|
|
|
|
/**
|
|
|
|
* When present, it adds a custom id to the tooltip.
|
|
|
|
*/
|
|
|
|
id?: string | undefined;
|
|
|
|
/**
|
|
|
|
* When present, it adds a custom class to the tooltip.
|
|
|
|
*/
|
|
|
|
class?: string | undefined;
|
|
|
|
/**
|
2023-03-07 13:44:36 +00:00
|
|
|
* By default the tooltip contents are not rendered as text. Set to true to support html tags in the content.
|
2023-03-01 14:57:18 +00:00
|
|
|
* @defaultValue false
|
|
|
|
*/
|
|
|
|
escape?: boolean | undefined;
|
|
|
|
/**
|
2023-03-02 09:31:36 +00:00
|
|
|
* Automatically adjusts the element position when there is not enough space on the selected position.
|
2023-03-01 14:57:18 +00:00
|
|
|
* @defaultValue true
|
|
|
|
*/
|
|
|
|
fitContent?: boolean | undefined;
|
2023-04-09 15:11:41 +00:00
|
|
|
/**
|
|
|
|
* When present, it adds a custom delay to the tooltip's display.
|
|
|
|
* @defaultValue 0
|
|
|
|
*/
|
|
|
|
showDelay?: number | undefined;
|
|
|
|
/**
|
|
|
|
* When present, it adds a custom delay to the tooltip's hiding.
|
|
|
|
* @defaultValue 0
|
|
|
|
*/
|
|
|
|
hideDelay?: number | undefined;
|
2023-06-21 13:58:32 +00:00
|
|
|
/**
|
|
|
|
* Uses to pass attributes to DOM elements inside the component.
|
|
|
|
* @type {TooltipPassThroughOptions}
|
|
|
|
*/
|
|
|
|
pt?: TooltipPassThroughOptions;
|
2023-03-02 09:53:55 +00:00
|
|
|
}
|
|
|
|
|
2023-03-02 10:54:16 +00:00
|
|
|
/**
|
|
|
|
* Defines modifiers of Tooltip.
|
|
|
|
*/
|
2023-03-02 14:25:05 +00:00
|
|
|
export interface TooltipDirectiveModifiers {
|
2023-03-02 10:54:16 +00:00
|
|
|
/**
|
|
|
|
* Right position for Tooltip.
|
2023-03-02 18:53:47 +00:00
|
|
|
* @defaultValue true
|
2023-03-02 10:54:16 +00:00
|
|
|
*/
|
2023-03-02 18:53:47 +00:00
|
|
|
right?: boolean | undefined;
|
2023-03-02 10:54:16 +00:00
|
|
|
/**
|
2023-03-02 18:53:47 +00:00
|
|
|
* Left position for Tooltip.
|
|
|
|
* @defaultValue false
|
2023-03-02 10:54:16 +00:00
|
|
|
*/
|
2023-03-02 18:53:47 +00:00
|
|
|
left?: boolean | undefined;
|
2023-03-02 10:54:16 +00:00
|
|
|
/**
|
|
|
|
* Top position for Tooltip.
|
2023-03-02 18:53:47 +00:00
|
|
|
* @defaultValue false
|
2023-03-02 10:54:16 +00:00
|
|
|
*/
|
2023-03-02 18:53:47 +00:00
|
|
|
top?: boolean | undefined;
|
2023-03-02 10:54:16 +00:00
|
|
|
/**
|
|
|
|
* Bottom position for Tooltip.
|
2023-03-02 18:53:47 +00:00
|
|
|
* @defaultValue false
|
2023-03-02 10:54:16 +00:00
|
|
|
*/
|
2023-03-02 18:53:47 +00:00
|
|
|
bottom?: boolean | undefined;
|
2023-03-02 10:54:16 +00:00
|
|
|
/**
|
|
|
|
* Focus event for Tooltip.
|
2023-03-02 18:53:47 +00:00
|
|
|
* @defaultValue true
|
2023-03-02 10:54:16 +00:00
|
|
|
*/
|
2023-03-02 18:53:47 +00:00
|
|
|
focus?: boolean | undefined;
|
2023-03-02 14:25:05 +00:00
|
|
|
}
|
2023-03-02 09:31:36 +00:00
|
|
|
|
2023-03-02 10:54:16 +00:00
|
|
|
/**
|
|
|
|
* Binding of Tooltip directive.
|
|
|
|
*/
|
2023-03-02 09:31:36 +00:00
|
|
|
export interface TooltipDirectiveBinding extends Omit<DirectiveBinding, 'modifiers' | 'value'> {
|
|
|
|
/**
|
2023-03-02 09:53:55 +00:00
|
|
|
* Value of the tooltip.
|
2023-03-02 09:31:36 +00:00
|
|
|
*/
|
2023-03-02 09:53:55 +00:00
|
|
|
value?: string | TooltipOptions | undefined;
|
2023-03-02 09:31:36 +00:00
|
|
|
/**
|
2023-03-02 09:53:55 +00:00
|
|
|
* Modifiers of the tooltip.
|
2023-03-02 09:31:36 +00:00
|
|
|
* @type {TooltipDirectiveModifiers}
|
|
|
|
*/
|
|
|
|
modifiers?: TooltipDirectiveModifiers | undefined;
|
2023-03-01 14:57:18 +00:00
|
|
|
}
|
|
|
|
|
2023-03-02 10:54:16 +00:00
|
|
|
/**
|
|
|
|
* **PrimeVue - Tooltip**
|
|
|
|
*
|
|
|
|
* _Tooltip directive provides advisory information for a component._
|
|
|
|
*
|
|
|
|
* [Live Demo](https://www.primevue.org/tooltip/)
|
|
|
|
* --- ---
|
2023-03-03 10:55:20 +00:00
|
|
|
* 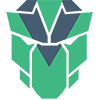
|
2023-03-02 10:54:16 +00:00
|
|
|
*
|
|
|
|
*/
|
2023-03-02 09:31:36 +00:00
|
|
|
declare const Tooltip: ObjectDirective;
|
|
|
|
|
2022-09-06 12:03:37 +00:00
|
|
|
export default Tooltip;
|