2023-03-01 13:12:23 +00:00
|
|
|
/**
|
|
|
|
*
|
|
|
|
* Inplace provides an easy to do editing and display at the same time where clicking the output displays the actual content.
|
|
|
|
*
|
|
|
|
* [Live Demo](https://www.primevue.org/inplace)
|
|
|
|
*
|
|
|
|
* @module inplace
|
|
|
|
*
|
|
|
|
*/
|
|
|
|
import { ButtonHTMLAttributes, HTMLAttributes, VNode } from 'vue';
|
2022-09-06 12:03:37 +00:00
|
|
|
import { ClassComponent, GlobalComponentConstructor } from '../ts-helpers';
|
|
|
|
|
2023-03-01 13:12:23 +00:00
|
|
|
/**
|
|
|
|
* Defines valid properties in Inplace component.
|
|
|
|
*/
|
2022-09-06 12:03:37 +00:00
|
|
|
export interface InplaceProps {
|
|
|
|
/**
|
|
|
|
* Displays a button to switch back to display mode.
|
2023-03-01 13:12:23 +00:00
|
|
|
* @defaultValue false
|
2022-09-06 12:03:37 +00:00
|
|
|
*/
|
|
|
|
closable?: boolean | undefined;
|
|
|
|
/**
|
|
|
|
* Whether the content is displayed or not.
|
2023-03-01 13:12:23 +00:00
|
|
|
* @defaultValue false
|
2022-09-06 12:03:37 +00:00
|
|
|
*/
|
|
|
|
active?: boolean | undefined;
|
|
|
|
/**
|
|
|
|
* When present, it specifies that the element should be disabled.
|
2023-03-01 13:12:23 +00:00
|
|
|
* @defaultValue false
|
2022-09-06 12:03:37 +00:00
|
|
|
*/
|
|
|
|
disabled?: boolean | undefined;
|
2022-12-08 11:04:25 +00:00
|
|
|
/**
|
|
|
|
* Icon to display in the close button.
|
2023-03-01 13:12:23 +00:00
|
|
|
* @defaultValue pi pi-times
|
2022-12-08 11:04:25 +00:00
|
|
|
*/
|
|
|
|
closeIcon?: string | undefined;
|
|
|
|
/**
|
|
|
|
* Uses to pass all properties of the HTMLDivElement to display container.
|
|
|
|
*/
|
|
|
|
displayProps?: HTMLAttributes | undefined;
|
|
|
|
/**
|
|
|
|
* Uses to pass all properties of the HTMLButtonElement to the close button.
|
|
|
|
*/
|
|
|
|
closeButtonProps?: ButtonHTMLAttributes | undefined;
|
2022-09-06 12:03:37 +00:00
|
|
|
}
|
|
|
|
|
2023-03-01 13:12:23 +00:00
|
|
|
/**
|
|
|
|
* Defines valid slots in Inplace component.
|
|
|
|
*/
|
2022-09-06 12:03:37 +00:00
|
|
|
export interface InplaceSlots {
|
|
|
|
/**
|
|
|
|
* Custom display template.
|
|
|
|
*/
|
2023-03-01 13:12:23 +00:00
|
|
|
display(): VNode[];
|
2022-09-06 12:03:37 +00:00
|
|
|
/**
|
|
|
|
* Custom content template.
|
|
|
|
*/
|
2023-03-01 13:12:23 +00:00
|
|
|
content(): VNode[];
|
2022-09-06 12:03:37 +00:00
|
|
|
}
|
|
|
|
|
2023-03-01 13:12:23 +00:00
|
|
|
/**
|
|
|
|
* Defines valid emits in Inplace component.
|
|
|
|
*/
|
|
|
|
export interface InplaceEmits {
|
2022-09-06 12:03:37 +00:00
|
|
|
/**
|
|
|
|
* Emitted when the active changes.
|
|
|
|
* @param {boolean} value - New value.
|
|
|
|
*/
|
2023-03-01 13:12:23 +00:00
|
|
|
'update:active'(value: boolean): void;
|
2022-09-06 12:03:37 +00:00
|
|
|
/**
|
|
|
|
* Callback to invoke when inplace is opened.
|
|
|
|
* @param {Event} event - Browser event.
|
|
|
|
*/
|
2023-03-01 13:12:23 +00:00
|
|
|
open(event: Event): void;
|
2022-09-06 12:03:37 +00:00
|
|
|
/**
|
|
|
|
* Callback to invoke when inplace is closed.
|
|
|
|
* @param {Event} event - Browser event.
|
|
|
|
*/
|
2023-03-01 13:12:23 +00:00
|
|
|
close(event: Event): void;
|
|
|
|
}
|
2022-09-06 12:03:37 +00:00
|
|
|
|
2023-03-01 13:12:23 +00:00
|
|
|
/**
|
|
|
|
* **PrimeVue - Inplace**
|
|
|
|
*
|
|
|
|
* _Inplace provides an easy to do editing and display at the same time where clicking the output displays the actual content._
|
|
|
|
*
|
|
|
|
* [Live Demo](https://www.primevue.org/inplace/)
|
|
|
|
* --- ---
|
|
|
|
* 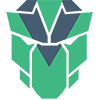
|
|
|
|
*
|
|
|
|
* @group Component
|
|
|
|
*/
|
2022-09-14 11:26:01 +00:00
|
|
|
declare class Inplace extends ClassComponent<InplaceProps, InplaceSlots, InplaceEmits> {}
|
2022-09-06 12:03:37 +00:00
|
|
|
|
|
|
|
declare module '@vue/runtime-core' {
|
|
|
|
interface GlobalComponents {
|
2022-09-14 11:26:01 +00:00
|
|
|
Inplace: GlobalComponentConstructor<Inplace>;
|
2022-09-06 12:03:37 +00:00
|
|
|
}
|
|
|
|
}
|
|
|
|
|
|
|
|
export default Inplace;
|