2023-03-01 10:19:16 +00:00
|
|
|
/**
|
|
|
|
*
|
|
|
|
* Sidebar is a panel component displayed as an overlay at the edges of the screen.
|
|
|
|
*
|
|
|
|
* - [Live Demo](https://www.primefaces.org/primevue/sidebar)
|
|
|
|
*
|
|
|
|
* @module sidebar
|
|
|
|
*
|
|
|
|
*/
|
2022-09-06 12:03:37 +00:00
|
|
|
import { VNode } from 'vue';
|
|
|
|
import { ClassComponent, GlobalComponentConstructor } from '../ts-helpers';
|
|
|
|
|
2023-03-01 10:19:16 +00:00
|
|
|
/**
|
|
|
|
* Defines valid properties in Sidebar component.
|
|
|
|
*/
|
2022-09-06 12:03:37 +00:00
|
|
|
export interface SidebarProps {
|
|
|
|
/**
|
|
|
|
* Specifies the visibility of the dialog.
|
2023-03-01 10:19:16 +00:00
|
|
|
* @defaultValue false
|
2022-09-06 12:03:37 +00:00
|
|
|
*/
|
|
|
|
visible?: boolean | undefined;
|
|
|
|
/**
|
|
|
|
* Specifies the position of the sidebar.
|
2023-03-01 10:19:16 +00:00
|
|
|
* @defaultValue left
|
2022-09-06 12:03:37 +00:00
|
|
|
*/
|
2023-03-01 10:19:16 +00:00
|
|
|
position?: 'left' | 'right' | 'top' | 'bottom' | 'full' | undefined;
|
2022-09-06 12:03:37 +00:00
|
|
|
/**
|
|
|
|
* Base zIndex value to use in layering.
|
2023-03-01 10:19:16 +00:00
|
|
|
* @defaultValue 0
|
2022-09-06 12:03:37 +00:00
|
|
|
*/
|
|
|
|
baseZIndex?: number | undefined;
|
|
|
|
/**
|
|
|
|
* Whether to automatically manage layering.
|
2023-03-01 10:19:16 +00:00
|
|
|
* @defaultValue true
|
2022-09-06 12:03:37 +00:00
|
|
|
*/
|
|
|
|
autoZIndex?: boolean | undefined;
|
|
|
|
/**
|
|
|
|
* Whether clicking outside closes the panel.
|
2023-03-01 10:19:16 +00:00
|
|
|
* @defaultValue true
|
2022-09-06 12:03:37 +00:00
|
|
|
*/
|
|
|
|
dismissable?: boolean | undefined;
|
|
|
|
/**
|
|
|
|
* Whether to display a close icon inside the panel.
|
2023-03-01 10:19:16 +00:00
|
|
|
* @defaultValue true
|
2022-09-06 12:03:37 +00:00
|
|
|
*/
|
|
|
|
showCloseIcon?: boolean | undefined;
|
2022-12-08 11:04:25 +00:00
|
|
|
/**
|
|
|
|
* Icon to display in the sidebar close button.
|
2023-03-01 10:19:16 +00:00
|
|
|
* @defaultValue pi pi-times
|
2022-12-08 11:04:25 +00:00
|
|
|
*/
|
|
|
|
closeIcon?: string | undefined;
|
2022-09-06 12:03:37 +00:00
|
|
|
/**
|
|
|
|
* Whether to a modal layer behind the sidebar.
|
2023-03-01 10:19:16 +00:00
|
|
|
* @defaultValue true
|
2022-09-06 12:03:37 +00:00
|
|
|
*/
|
|
|
|
modal?: boolean | undefined;
|
2023-01-04 10:12:04 +00:00
|
|
|
/**
|
|
|
|
* Whether background scroll should be blocked when sidebar is visible.
|
2023-03-01 10:19:16 +00:00
|
|
|
* @defaultValue false
|
2023-01-04 10:12:04 +00:00
|
|
|
*/
|
|
|
|
blockScroll?: boolean | undefined;
|
2022-09-06 12:03:37 +00:00
|
|
|
}
|
|
|
|
|
2023-03-01 10:19:16 +00:00
|
|
|
/**
|
|
|
|
* Defines valid slots in Sidebar component.
|
|
|
|
*/
|
2022-09-06 12:03:37 +00:00
|
|
|
export interface SidebarSlots {
|
|
|
|
/**
|
|
|
|
* Custom content template.
|
|
|
|
*/
|
2023-03-01 10:19:16 +00:00
|
|
|
default(): VNode[];
|
2022-09-06 12:03:37 +00:00
|
|
|
/**
|
|
|
|
* Custom header template.
|
|
|
|
*/
|
2023-03-01 10:19:16 +00:00
|
|
|
header(): VNode[];
|
2022-09-06 12:03:37 +00:00
|
|
|
}
|
|
|
|
|
2023-03-01 10:19:16 +00:00
|
|
|
/**
|
|
|
|
* Defines valid emits in Sidebar component.
|
|
|
|
*/
|
|
|
|
export interface SidebarEmits {
|
2022-09-06 12:03:37 +00:00
|
|
|
/**
|
|
|
|
* Emitted when the value changes.
|
|
|
|
* @param {boolean} value - New value.
|
|
|
|
*/
|
2023-03-01 10:19:16 +00:00
|
|
|
'update:modelValue'(value: boolean): void;
|
2022-09-06 12:03:37 +00:00
|
|
|
/**
|
|
|
|
* Callback to invoke when sidebar gets shown.
|
|
|
|
*/
|
2023-03-01 10:19:16 +00:00
|
|
|
show(): void;
|
2022-09-06 12:03:37 +00:00
|
|
|
/**
|
|
|
|
* Callback to invoke when sidebar gets hidden.
|
|
|
|
*/
|
2023-03-01 10:19:16 +00:00
|
|
|
hide(): void;
|
|
|
|
}
|
2022-09-06 12:03:37 +00:00
|
|
|
|
2023-03-01 10:19:16 +00:00
|
|
|
/**
|
|
|
|
* **PrimeVue - Sidebar**
|
|
|
|
*
|
|
|
|
* _Sidebar is a panel component displayed as an overlay._
|
|
|
|
*
|
|
|
|
* [Live Demo](https://www.primevue.org/sidebar/)
|
|
|
|
* --- ---
|
|
|
|
* 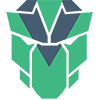
|
|
|
|
*
|
|
|
|
* @group Component
|
|
|
|
*/
|
2023-03-01 14:48:23 +00:00
|
|
|
declare class Sidebar extends ClassComponent<SidebarProps, SidebarSlots, SidebarEmits> {}
|
2022-09-06 12:03:37 +00:00
|
|
|
|
|
|
|
declare module '@vue/runtime-core' {
|
|
|
|
interface GlobalComponents {
|
2022-09-14 11:26:01 +00:00
|
|
|
Sidebar: GlobalComponentConstructor<Sidebar>;
|
2022-09-06 12:03:37 +00:00
|
|
|
}
|
|
|
|
}
|
|
|
|
|
|
|
|
/**
|
|
|
|
*
|
|
|
|
* Sidebar is a panel component displayed as an overlay at the edges of the screen.
|
|
|
|
*
|
|
|
|
* Demos:
|
|
|
|
*
|
2022-09-14 11:26:01 +00:00
|
|
|
* - [Sidebar](https://www.primefaces.org/primevue/sidebar)
|
2022-09-06 12:03:37 +00:00
|
|
|
*
|
|
|
|
*/
|
|
|
|
export default Sidebar;
|