diff --git a/components/lib/step/BaseStep.vue b/components/lib/step/BaseStep.vue
new file mode 100644
index 000000000..313368c79
--- /dev/null
+++ b/components/lib/step/BaseStep.vue
@@ -0,0 +1,34 @@
+
diff --git a/components/lib/step/Step.d.ts b/components/lib/step/Step.d.ts
new file mode 100644
index 000000000..f164f2baa
--- /dev/null
+++ b/components/lib/step/Step.d.ts
@@ -0,0 +1,187 @@
+/**
+ *
+ * Step is a helper component for Stepper component.
+ *
+ * [Live Demo](https://www.primevue.org/stepper/)
+ *
+ * @module step
+ *
+ */
+import { VNode } from 'vue';
+import { ComponentHooks } from '../basecomponent';
+import { PassThroughOptions } from '../passthrough';
+import { DefineComponent, DesignToken, EmitFn, GlobalComponentConstructor, PassThrough } from '../ts-helpers';
+
+export declare type StepPassThroughOptionType = StepPassThroughAttributes | ((options: StepPassThroughMethodOptions) => StepPassThroughAttributes | string) | string | null | undefined;
+
+/**
+ * Custom passthrough(pt) option method.
+ */
+export interface StepPassThroughMethodOptions {
+ /**
+ * Defines instance.
+ */
+ instance: any;
+ /**
+ * Defines valid properties.
+ */
+ props: StepProps;
+ /**
+ * Defines current options.
+ */
+ context: StepContext;
+ /**
+ * Defines valid attributes.
+ */
+ attrs: any;
+ /**
+ * Defines parent options.
+ */
+ parent: any;
+ /**
+ * Defines passthrough(pt) options in global config.
+ */
+ global: object | undefined;
+}
+
+/**
+ * Custom passthrough(pt) options.
+ * @see {@link StepProps.pt}
+ */
+export interface StepPassThroughOptions {
+ /**
+ * Used to pass attributes to the root's DOM element.
+ */
+ root?: StepPassThroughOptionType;
+ /**
+ * Used to pass attributes to the header's DOM element.
+ */
+ header?: StepPassThroughOptionType;
+ /**
+ * Used to pass attributes to the number's DOM element.
+ */
+ number?: StepPassThroughOptionType;
+ /**
+ * Used to pass attributes to the title's DOM element.
+ */
+ title?: StepPassThroughOptionType;
+ /**
+ * Used to manage all lifecycle hooks.
+ * @see {@link BaseComponent.ComponentHooks}
+ */
+ hooks?: ComponentHooks;
+}
+
+export interface StepPassThroughAttributes {
+ [key: string]: any;
+}
+
+/**
+ * Defines valid properties in Step component.
+ */
+export interface StepProps {
+ /**
+ * Value of step.
+ */
+ value?: string | number | undefined;
+ /**
+ * Whether the step is disabled.
+ * @defaultValue false
+ */
+ disabled?: boolean | undefined;
+ /**
+ * Use to change the HTML tag of root element.
+ * @defaultValue BUTTON
+ */
+ as?: string | undefined;
+ /**
+ * When enabled, it changes the default rendered element for the one passed as a child element.
+ * @defaultValue false
+ */
+ asChild?: boolean | undefined;
+ /**
+ * It generates scoped CSS variables using design tokens for the component.
+ */
+ dt?: DesignToken;
+ /**
+ * Used to pass attributes to DOM elements inside the component.
+ * @type {StepPassThroughOptions}
+ */
+ pt?: PassThrough;
+ /**
+ * Used to configure passthrough(pt) options of the component.
+ * @type {PassThroughOptions}
+ */
+ ptOptions?: PassThroughOptions;
+}
+
+/**
+ * Defines current options in Step component.
+ */
+export interface StepContext {
+ /**
+ * Whether the step is active.
+ */
+ active: boolean;
+ /**
+ * Whether the step is disabled.
+ */
+ disabled: boolean;
+}
+
+/**
+ * Defines valid slots in Step slots.
+ */
+export interface StepSlots {
+ /**
+ * Custom content template. Slot attributes can be used when asChild prop is true.
+ */
+ default(scope: {
+ /**
+ * Style class of the loader.
+ */
+ class: string;
+ /**
+ * Whether the step is active.
+ */
+ active: boolean;
+ /**
+ * Value of step.
+ */
+ value: string | number;
+ /**
+ * A11t attributes
+ */
+ a11yAttrs: any;
+ /**
+ * Click function.
+ */
+ activateCallback: () => void;
+ }): VNode[];
+}
+
+export interface StepEmitsOptions {}
+
+export declare type StepEmits = EmitFn;
+
+/**
+ * **PrimeVue - Step**
+ *
+ * _Step is a helper component for Stepper component._
+ *
+ * [Live Demo](https://www.primevue.org/stepper/)
+ * --- ---
+ * 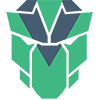
+ *
+ * @group Component
+ *
+ */
+declare const Step: DefineComponent;
+
+declare module 'vue' {
+ export interface GlobalComponents {
+ Step: GlobalComponentConstructor;
+ }
+}
+
+export default Step;
diff --git a/components/lib/step/Step.vue b/components/lib/step/Step.vue
new file mode 100644
index 000000000..1cd87a07a
--- /dev/null
+++ b/components/lib/step/Step.vue
@@ -0,0 +1,98 @@
+
+
+
+
+
+
+
+
+
diff --git a/components/lib/step/package.json b/components/lib/step/package.json
new file mode 100644
index 000000000..889ffe7bd
--- /dev/null
+++ b/components/lib/step/package.json
@@ -0,0 +1,11 @@
+{
+ "main": "./step.mjs",
+ "module": "./step.mjs",
+ "types": "./Step.d.ts",
+ "browser": {
+ "./sfc": "./Step.vue"
+ },
+ "sideEffects": [
+ "*.vue"
+ ]
+}
diff --git a/components/lib/step/style/StepStyle.d.ts b/components/lib/step/style/StepStyle.d.ts
new file mode 100644
index 000000000..36da74349
--- /dev/null
+++ b/components/lib/step/style/StepStyle.d.ts
@@ -0,0 +1,31 @@
+/**
+ *
+ * Step is a helper component for Stepper component.
+ *
+ * [Live Demo](https://www.primevue.org/stepper/)
+ *
+ * @module stepstyle
+ *
+ */
+import { BaseStyle } from '../../base/style';
+
+export enum StepClasses {
+ /**
+ * Class name of the root element
+ */
+ root = 'p-step',
+ /**
+ * Class name of the header element
+ */
+ header = 'p-step-header',
+ /**
+ * Class name of the number element
+ */
+ number = 'p-step-number',
+ /**
+ * Class name of the title element
+ */
+ title = 'p-step-title'
+}
+
+export interface StepStyle extends BaseStyle {}
diff --git a/components/lib/step/style/StepStyle.js b/components/lib/step/style/StepStyle.js
new file mode 100644
index 000000000..7bd4cac18
--- /dev/null
+++ b/components/lib/step/style/StepStyle.js
@@ -0,0 +1,19 @@
+import BaseStyle from 'primevue/base/style';
+
+const classes = {
+ root: ({ instance, props }) => [
+ 'p-step',
+ {
+ 'p-step-active': instance.active,
+ 'p-disabled': instance.isStepDisabled() || props.disabled
+ }
+ ],
+ header: 'p-step-header',
+ number: 'p-step-number',
+ title: 'p-step-title'
+};
+
+export default BaseStyle.extend({
+ name: 'step',
+ classes
+});
diff --git a/components/lib/step/style/package.json b/components/lib/step/style/package.json
new file mode 100644
index 000000000..bb4bf5fcd
--- /dev/null
+++ b/components/lib/step/style/package.json
@@ -0,0 +1,6 @@
+{
+ "main": "./stepstyle.mjs",
+ "module": "./stepstyle.mjs",
+ "types": "./StepStyle.d.ts",
+ "sideEffects": false
+}
diff --git a/components/lib/stepitem/BaseStepItem.vue b/components/lib/stepitem/BaseStepItem.vue
new file mode 100644
index 000000000..5ec215733
--- /dev/null
+++ b/components/lib/stepitem/BaseStepItem.vue
@@ -0,0 +1,22 @@
+
diff --git a/components/lib/stepitem/StepItem.d.ts b/components/lib/stepitem/StepItem.d.ts
new file mode 100644
index 000000000..4c66daeaf
--- /dev/null
+++ b/components/lib/stepitem/StepItem.d.ts
@@ -0,0 +1,121 @@
+/**
+ *
+ * StepItem is a helper component for Stepper component.
+ *
+ * [Live Demo](https://www.primevue.org/stepper/)
+ *
+ * @module stepitem
+ *
+ */
+import { VNode } from 'vue';
+import { ComponentHooks } from '../basecomponent';
+import { PassThroughOptions } from '../passthrough';
+import { DefineComponent, DesignToken, EmitFn, GlobalComponentConstructor, PassThrough } from '../ts-helpers';
+
+export declare type StepItemPassThroughOptionType = StepItemPassThroughAttributes | ((options: StepItemPassThroughMethodOptions) => StepItemPassThroughAttributes | string) | string | null | undefined;
+
+/**
+ * Custom passthrough(pt) option method.
+ */
+export interface StepItemPassThroughMethodOptions {
+ /**
+ * Defines instance.
+ */
+ instance: any;
+ /**
+ * Defines valid properties.
+ */
+ props: StepItemProps;
+ /**
+ * Defines valid attributes.
+ */
+ attrs: any;
+ /**
+ * Defines parent options.
+ */
+ parent: any;
+ /**
+ * Defines passthrough(pt) options in global config.
+ */
+ global: object | undefined;
+}
+
+/**
+ * Custom passthrough(pt) options.
+ * @see {@link StepItemProps.pt}
+ */
+export interface StepItemPassThroughOptions {
+ /**
+ * Used to pass attributes to the root's DOM element.
+ */
+ root?: StepItemPassThroughOptionType;
+ /**
+ * Used to manage all lifecycle hooks.
+ * @see {@link BaseComponent.ComponentHooks}
+ */
+ hooks?: ComponentHooks;
+}
+
+export interface StepItemPassThroughAttributes {
+ [key: string]: any;
+}
+
+/**
+ * Defines valid properties in StepItem component.
+ */
+export interface StepItemProps {
+ /**
+ * Value of step.
+ */
+ value?: string | number | undefined;
+ /**
+ * It generates scoped CSS variables using design tokens for the component.
+ */
+ dt?: DesignToken;
+ /**
+ * Used to pass attributes to DOM elements inside the component.
+ * @type {StepItemPassThroughOptions}
+ */
+ pt?: PassThrough;
+ /**
+ * Used to configure passthrough(pt) options of the component.
+ * @type {PassThroughOptions}
+ */
+ ptOptions?: PassThroughOptions;
+}
+
+/**
+ * Defines valid slots in StepItem slots.
+ */
+export interface StepItemSlots {
+ /**
+ * Custom content template.
+ */
+ default(): VNode[];
+}
+
+export interface StepItemEmitsOptions {}
+
+export declare type StepItemEmits = EmitFn;
+
+/**
+ * **PrimeVue - StepItem**
+ *
+ * _StepItem is a helper component for Stepper component._
+ *
+ * [Live Demo](https://www.primevue.org/stepper/)
+ * --- ---
+ * 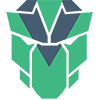
+ *
+ * @group Component
+ *
+ */
+declare const StepItem: DefineComponent;
+
+declare module 'vue' {
+ export interface GlobalComponents {
+ StepItem: GlobalComponentConstructor;
+ }
+}
+
+export default StepItem;
diff --git a/components/lib/stepitem/StepItem.vue b/components/lib/stepitem/StepItem.vue
new file mode 100644
index 000000000..a02dfa1a4
--- /dev/null
+++ b/components/lib/stepitem/StepItem.vue
@@ -0,0 +1,21 @@
+
+
+
+
+
+
+
diff --git a/components/lib/stepitem/package.json b/components/lib/stepitem/package.json
new file mode 100644
index 000000000..13a00c151
--- /dev/null
+++ b/components/lib/stepitem/package.json
@@ -0,0 +1,11 @@
+{
+ "main": "./stepitem.mjs",
+ "module": "./stepitem.mjs",
+ "types": "./StepItem.d.ts",
+ "browser": {
+ "./sfc": "./StepItem.vue"
+ },
+ "sideEffects": [
+ "*.vue"
+ ]
+}
diff --git a/components/lib/stepitem/style/StepItemStyle.d.ts b/components/lib/stepitem/style/StepItemStyle.d.ts
new file mode 100644
index 000000000..a4e476cd5
--- /dev/null
+++ b/components/lib/stepitem/style/StepItemStyle.d.ts
@@ -0,0 +1,19 @@
+/**
+ *
+ * StepItem is a helper component for Stepper component.
+ *
+ * [Live Demo](https://www.primevue.org/stepper/)
+ *
+ * @module stepitemstyle
+ *
+ */
+import { BaseStyle } from '../../base/style';
+
+export enum StepItemClasses {
+ /**
+ * Class name of the root element
+ */
+ root = 'p-stepitem'
+}
+
+export interface StepItemStyle extends BaseStyle {}
diff --git a/components/lib/stepitem/style/StepItemStyle.js b/components/lib/stepitem/style/StepItemStyle.js
new file mode 100644
index 000000000..204913c75
--- /dev/null
+++ b/components/lib/stepitem/style/StepItemStyle.js
@@ -0,0 +1,15 @@
+import BaseStyle from 'primevue/base/style';
+
+const classes = {
+ root: ({ instance }) => [
+ 'p-stepitem',
+ {
+ 'p-stepitem-active': instance.isActive
+ }
+ ]
+};
+
+export default BaseStyle.extend({
+ name: 'stepitem',
+ classes
+});
diff --git a/components/lib/stepitem/style/package.json b/components/lib/stepitem/style/package.json
new file mode 100644
index 000000000..9dc896030
--- /dev/null
+++ b/components/lib/stepitem/style/package.json
@@ -0,0 +1,6 @@
+{
+ "main": "./stepitemstyle.mjs",
+ "module": "./stepitemstyle.mjs",
+ "types": "./StepItemStyle.d.ts",
+ "sideEffects": false
+}
diff --git a/components/lib/steplist/BaseStepList.vue b/components/lib/steplist/BaseStepList.vue
new file mode 100644
index 000000000..8b71a8b56
--- /dev/null
+++ b/components/lib/steplist/BaseStepList.vue
@@ -0,0 +1,22 @@
+
diff --git a/components/lib/steplist/StepList.d.ts b/components/lib/steplist/StepList.d.ts
new file mode 100644
index 000000000..2de48b18f
--- /dev/null
+++ b/components/lib/steplist/StepList.d.ts
@@ -0,0 +1,121 @@
+/**
+ *
+ * StepList is a helper component for Stepper component.
+ *
+ * [Live Demo](https://www.primevue.org/stepper/)
+ *
+ * @module steplist
+ *
+ */
+import { VNode } from 'vue';
+import { ComponentHooks } from '../basecomponent';
+import { PassThroughOptions } from '../passthrough';
+import { DefineComponent, DesignToken, EmitFn, GlobalComponentConstructor, PassThrough } from '../ts-helpers';
+
+export declare type StepListPassThroughOptionType = StepListPassThroughAttributes | ((options: StepListPassThroughMethodOptions) => StepListPassThroughAttributes | string) | string | null | undefined;
+
+/**
+ * Custom passthrough(pt) option method.
+ */
+export interface StepListPassThroughMethodOptions {
+ /**
+ * Defines instance.
+ */
+ instance: any;
+ /**
+ * Defines valid properties.
+ */
+ props: StepListProps;
+ /**
+ * Defines valid attributes.
+ */
+ attrs: any;
+ /**
+ * Defines parent options.
+ */
+ parent: any;
+ /**
+ * Defines passthrough(pt) options in global config.
+ */
+ global: object | undefined;
+}
+
+/**
+ * Custom passthrough(pt) options.
+ * @see {@link StepListProps.pt}
+ */
+export interface StepListPassThroughOptions {
+ /**
+ * Used to pass attributes to the root's DOM element.
+ */
+ root?: StepListPassThroughOptionType;
+ /**
+ * Used to manage all lifecycle hooks.
+ * @see {@link BaseComponent.ComponentHooks}
+ */
+ hooks?: ComponentHooks;
+}
+
+export interface StepListPassThroughAttributes {
+ [key: string]: any;
+}
+
+/**
+ * Defines valid properties in StepList component.
+ */
+export interface StepListProps {
+ /**
+ * Value of step.
+ */
+ value: string;
+ /**
+ * It generates scoped CSS variables using design tokens for the component.
+ */
+ dt?: DesignToken;
+ /**
+ * Used to pass attributes to DOM elements inside the component.
+ * @type {StepListPassThroughOptions}
+ */
+ pt?: PassThrough;
+ /**
+ * Used to configure passthrough(pt) options of the component.
+ * @type {PassThroughOptions}
+ */
+ ptOptions?: PassThroughOptions;
+}
+
+/**
+ * Defines valid slots in StepList slots.
+ */
+export interface StepListSlots {
+ /**
+ * Custom content template.
+ */
+ default(): VNode[];
+}
+
+export interface StepListEmitsOptions {}
+
+export declare type StepListEmits = EmitFn;
+
+/**
+ * **PrimeVue - StepList**
+ *
+ * _StepList is a helper component for Stepper component._
+ *
+ * [Live Demo](https://www.primevue.org/stepper/)
+ * --- ---
+ * 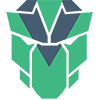
+ *
+ * @group Component
+ *
+ */
+declare const StepList: DefineComponent;
+
+declare module 'vue' {
+ export interface GlobalComponents {
+ StepList: GlobalComponentConstructor;
+ }
+}
+
+export default StepList;
diff --git a/components/lib/steplist/StepList.vue b/components/lib/steplist/StepList.vue
new file mode 100644
index 000000000..e67133da4
--- /dev/null
+++ b/components/lib/steplist/StepList.vue
@@ -0,0 +1,15 @@
+
+
+
+
+
+
+
diff --git a/components/lib/steplist/package.json b/components/lib/steplist/package.json
new file mode 100644
index 000000000..3fd9218d5
--- /dev/null
+++ b/components/lib/steplist/package.json
@@ -0,0 +1,11 @@
+{
+ "main": "./steplist.mjs",
+ "module": "./steplist.mjs",
+ "types": "./StepList.d.ts",
+ "browser": {
+ "./sfc": "./StepList.vue"
+ },
+ "sideEffects": [
+ "*.vue"
+ ]
+}
diff --git a/components/lib/steplist/style/StepListStyle.d.ts b/components/lib/steplist/style/StepListStyle.d.ts
new file mode 100644
index 000000000..4779ff014
--- /dev/null
+++ b/components/lib/steplist/style/StepListStyle.d.ts
@@ -0,0 +1,19 @@
+/**
+ *
+ * StepList is a helper component for Stepper component.
+ *
+ * [Live Demo](https://www.primevue.org/stepper/)
+ *
+ * @module stepliststyle
+ *
+ */
+import { BaseStyle } from '../../base/style';
+
+export enum StepListClasses {
+ /**
+ * Class name of the root element
+ */
+ root = 'p-steplist'
+}
+
+export interface StepListStyle extends BaseStyle {}
diff --git a/components/lib/steplist/style/StepListStyle.js b/components/lib/steplist/style/StepListStyle.js
new file mode 100644
index 000000000..c885f0461
--- /dev/null
+++ b/components/lib/steplist/style/StepListStyle.js
@@ -0,0 +1,10 @@
+import BaseStyle from 'primevue/base/style';
+
+const classes = {
+ root: 'p-steplist'
+};
+
+export default BaseStyle.extend({
+ name: 'steplist',
+ classes
+});
diff --git a/components/lib/steplist/style/package.json b/components/lib/steplist/style/package.json
new file mode 100644
index 000000000..c5e27601f
--- /dev/null
+++ b/components/lib/steplist/style/package.json
@@ -0,0 +1,6 @@
+{
+ "main": "./stepliststyle.mjs",
+ "module": "./stepliststyle.mjs",
+ "types": "./StepListStyle.d.ts",
+ "sideEffects": false
+}
diff --git a/components/lib/steppanel/BaseStepPanel.vue b/components/lib/steppanel/BaseStepPanel.vue
new file mode 100644
index 000000000..6cd065837
--- /dev/null
+++ b/components/lib/steppanel/BaseStepPanel.vue
@@ -0,0 +1,30 @@
+
diff --git a/components/lib/steppanel/StepPanel.d.ts b/components/lib/steppanel/StepPanel.d.ts
new file mode 100644
index 000000000..7a5bf4341
--- /dev/null
+++ b/components/lib/steppanel/StepPanel.d.ts
@@ -0,0 +1,148 @@
+/**
+ *
+ * StepPanel is a helper component for Stepper component.
+ *
+ * [Live Demo](https://www.primevue.org/stepper/)
+ *
+ * @module steppanel
+ *
+ */
+import { VNode } from 'vue';
+import { ComponentHooks } from '../basecomponent';
+import { PassThroughOptions } from '../passthrough';
+import { DefineComponent, DesignToken, EmitFn, GlobalComponentConstructor, PassThrough } from '../ts-helpers';
+
+export declare type StepPanelPassThroughOptionType = StepPanelPassThroughAttributes | ((options: StepPanelPassThroughMethodOptions) => StepPanelPassThroughAttributes | string) | string | null | undefined;
+
+/**
+ * Custom passthrough(pt) option method.
+ */
+export interface StepPanelPassThroughMethodOptions {
+ /**
+ * Defines instance.
+ */
+ instance: any;
+ /**
+ * Defines valid properties.
+ */
+ props: StepPanelProps;
+ /**
+ * Defines valid attributes.
+ */
+ attrs: any;
+ /**
+ * Defines parent options.
+ */
+ parent: any;
+ /**
+ * Defines passthrough(pt) options in global config.
+ */
+ global: object | undefined;
+}
+
+/**
+ * Custom passthrough(pt) options.
+ * @see {@link StepPanelProps.pt}
+ */
+export interface StepPanelPassThroughOptions {
+ /**
+ * Used to pass attributes to the root's DOM element.
+ */
+ root?: StepPanelPassThroughOptionType;
+ /**
+ * Used to manage all lifecycle hooks.
+ * @see {@link BaseComponent.ComponentHooks}
+ */
+ hooks?: ComponentHooks;
+}
+
+export interface StepPanelPassThroughAttributes {
+ [key: string]: any;
+}
+
+/**
+ * Defines valid properties in StepPanel component.
+ */
+export interface StepPanelProps {
+ /**
+ * Value of step.
+ */
+ value?: string | number | undefined;
+ /**
+ * Use to change the HTML tag of root element.
+ * @defaultValue BUTTON
+ */
+ as?: string | undefined;
+ /**
+ * When enabled, it changes the default rendered element for the one passed as a child element.
+ * @defaultValue false
+ */
+ asChild?: boolean | undefined;
+ /**
+ * It generates scoped CSS variables using design tokens for the component.
+ */
+ dt?: DesignToken;
+ /**
+ * Used to pass attributes to DOM elements inside the component.
+ * @type {StepPanelPassThroughOptions}
+ */
+ pt?: PassThrough;
+ /**
+ * Used to configure passthrough(pt) options of the component.
+ * @type {PassThroughOptions}
+ */
+ ptOptions?: PassThroughOptions;
+}
+
+/**
+ * Defines valid slots in StepPanel slots.
+ */
+export interface StepPanelSlots {
+ /**
+ * Custom content template. Slot attributes can be used when asChild prop is true.
+ */
+ default(scope: {
+ /**
+ * Whether the step is active.
+ */
+ active: boolean;
+ /**
+ * Value of step.
+ */
+ value: string | number;
+ /**
+ * A11t attributes
+ */
+ a11yAttrs: any;
+ /**
+ * Click function.
+ */
+ activateCallback: () => void;
+ }): VNode[];
+}
+
+export interface StepPanelEmitsOptions {}
+
+export declare type StepPanelEmits = EmitFn;
+
+/**
+ * **PrimeVue - StepPanel**
+ *
+ * _StepPanel is a helper component for Stepper component._
+ *
+ * [Live Demo](https://www.primevue.org/stepper/)
+ * --- ---
+ * 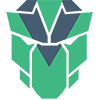
+ *
+ * @group Component
+ *
+ */
+declare const StepPanel: DefineComponent;
+
+declare module 'vue' {
+ export interface GlobalComponents {
+ StepPanel: GlobalComponentConstructor;
+ }
+}
+
+export default StepPanel;
diff --git a/components/lib/steppanel/StepPanel.vue b/components/lib/steppanel/StepPanel.vue
new file mode 100644
index 000000000..312864c98
--- /dev/null
+++ b/components/lib/steppanel/StepPanel.vue
@@ -0,0 +1,99 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/components/lib/steppanel/package.json b/components/lib/steppanel/package.json
new file mode 100644
index 000000000..92a1d1093
--- /dev/null
+++ b/components/lib/steppanel/package.json
@@ -0,0 +1,11 @@
+{
+ "main": "./steppanel.mjs",
+ "module": "./steppanel.mjs",
+ "types": "./StepPanel.d.ts",
+ "browser": {
+ "./sfc": "./StepPanel.vue"
+ },
+ "sideEffects": [
+ "*.vue"
+ ]
+}
diff --git a/components/lib/steppanel/style/StepPanelStyle.d.ts b/components/lib/steppanel/style/StepPanelStyle.d.ts
new file mode 100644
index 000000000..7fecfc1c8
--- /dev/null
+++ b/components/lib/steppanel/style/StepPanelStyle.d.ts
@@ -0,0 +1,19 @@
+/**
+ *
+ * StepPanel is a helper component for Stepper component.
+ *
+ * [Live Demo](https://www.primevue.org/stepper/)
+ *
+ * @module steppanelstyle
+ *
+ */
+import { BaseStyle } from '../../base/style';
+
+export enum StepPanelClasses {
+ /**
+ * Class name of the root element
+ */
+ root = 'p-steppanel'
+}
+
+export interface StepPanelStyle extends BaseStyle {}
diff --git a/components/lib/steppanel/style/StepPanelStyle.js b/components/lib/steppanel/style/StepPanelStyle.js
new file mode 100644
index 000000000..2cddf8384
--- /dev/null
+++ b/components/lib/steppanel/style/StepPanelStyle.js
@@ -0,0 +1,16 @@
+import BaseStyle from 'primevue/base/style';
+
+const classes = {
+ root: ({ instance }) => [
+ 'p-steppanel',
+ {
+ 'p-steppanel-active': instance.isVertical && instance.active
+ }
+ ],
+ content: 'p-steppanel-content'
+};
+
+export default BaseStyle.extend({
+ name: 'steppanel',
+ classes
+});
diff --git a/components/lib/steppanel/style/package.json b/components/lib/steppanel/style/package.json
new file mode 100644
index 000000000..a41306a2e
--- /dev/null
+++ b/components/lib/steppanel/style/package.json
@@ -0,0 +1,6 @@
+{
+ "main": "./steppanelstyle.mjs",
+ "module": "./steppanelstyle.mjs",
+ "types": "./StepPanelStyle.d.ts",
+ "sideEffects": false
+}
diff --git a/components/lib/steppanels/BaseStepPanels.vue b/components/lib/steppanels/BaseStepPanels.vue
new file mode 100644
index 000000000..5e3ff73cc
--- /dev/null
+++ b/components/lib/steppanels/BaseStepPanels.vue
@@ -0,0 +1,22 @@
+
diff --git a/components/lib/steppanels/StepPanels.d.ts b/components/lib/steppanels/StepPanels.d.ts
new file mode 100644
index 000000000..e085fc812
--- /dev/null
+++ b/components/lib/steppanels/StepPanels.d.ts
@@ -0,0 +1,121 @@
+/**
+ *
+ * StepPanels is a helper component for Stepper component.
+ *
+ * [Live Demo](https://www.primevue.org/stepper/)
+ *
+ * @module steppanels
+ *
+ */
+import { VNode } from 'vue';
+import { ComponentHooks } from '../basecomponent';
+import { PassThroughOptions } from '../passthrough';
+import { DefineComponent, DesignToken, EmitFn, GlobalComponentConstructor, PassThrough } from '../ts-helpers';
+
+export declare type StepPanelsPassThroughOptionType = StepPanelsPassThroughAttributes | ((options: StepPanelsPassThroughMethodOptions) => StepPanelsPassThroughAttributes | string) | string | null | undefined;
+
+/**
+ * Custom passthrough(pt) option method.
+ */
+export interface StepPanelsPassThroughMethodOptions {
+ /**
+ * Defines instance.
+ */
+ instance: any;
+ /**
+ * Defines valid properties.
+ */
+ props: StepPanelsProps;
+ /**
+ * Defines valid attributes.
+ */
+ attrs: any;
+ /**
+ * Defines parent options.
+ */
+ parent: any;
+ /**
+ * Defines passthrough(pt) options in global config.
+ */
+ global: object | undefined;
+}
+
+/**
+ * Custom passthrough(pt) options.
+ * @see {@link StepPanelsProps.pt}
+ */
+export interface StepPanelsPassThroughOptions {
+ /**
+ * Used to pass attributes to the root's DOM element.
+ */
+ root?: StepPanelsPassThroughOptionType;
+ /**
+ * Used to manage all lifecycle hooks.
+ * @see {@link BaseComponent.ComponentHooks}
+ */
+ hooks?: ComponentHooks;
+}
+
+export interface StepPanelsPassThroughAttributes {
+ [key: string]: any;
+}
+
+/**
+ * Defines valid properties in StepPanels component.
+ */
+export interface StepPanelsProps {
+ /**
+ * Value of step.
+ */
+ value: string;
+ /**
+ * It generates scoped CSS variables using design tokens for the component.
+ */
+ dt?: DesignToken;
+ /**
+ * Used to pass attributes to DOM elements inside the component.
+ * @type {StepPanelsPassThroughOptions}
+ */
+ pt?: PassThrough;
+ /**
+ * Used to configure passthrough(pt) options of the component.
+ * @type {PassThroughOptions}
+ */
+ ptOptions?: PassThroughOptions;
+}
+
+/**
+ * Defines valid slots in StepPanels slots.
+ */
+export interface StepPanelsSlots {
+ /**
+ * Custom content template.
+ */
+ default(): VNode[];
+}
+
+export interface StepPanelsEmitsOptions {}
+
+export declare type StepPanelsEmits = EmitFn;
+
+/**
+ * **PrimeVue - StepPanels**
+ *
+ * _StepPanels is a helper component for Stepper component._
+ *
+ * [Live Demo](https://www.primevue.org/stepper/)
+ * --- ---
+ * 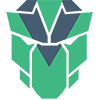
+ *
+ * @group Component
+ *
+ */
+declare const StepPanels: DefineComponent;
+
+declare module 'vue' {
+ export interface GlobalComponents {
+ StepPanels: GlobalComponentConstructor;
+ }
+}
+
+export default StepPanels;
diff --git a/components/lib/steppanels/StepPanels.vue b/components/lib/steppanels/StepPanels.vue
new file mode 100644
index 000000000..0be949db1
--- /dev/null
+++ b/components/lib/steppanels/StepPanels.vue
@@ -0,0 +1,15 @@
+
+
+
+
+
+
+
diff --git a/components/lib/steppanels/package.json b/components/lib/steppanels/package.json
new file mode 100644
index 000000000..2f063f57e
--- /dev/null
+++ b/components/lib/steppanels/package.json
@@ -0,0 +1,11 @@
+{
+ "main": "./steppanels.mjs",
+ "module": "./steppanels.mjs",
+ "types": "./StepPanels.d.ts",
+ "browser": {
+ "./sfc": "./StepPanels.vue"
+ },
+ "sideEffects": [
+ "*.vue"
+ ]
+}
diff --git a/components/lib/steppanels/style/StepPanelsStyle.d.ts b/components/lib/steppanels/style/StepPanelsStyle.d.ts
new file mode 100644
index 000000000..7101d82a3
--- /dev/null
+++ b/components/lib/steppanels/style/StepPanelsStyle.d.ts
@@ -0,0 +1,19 @@
+/**
+ *
+ * StepPanels
+ *
+ * [Live Demo](https://www.primevue.org/stepper/)
+ *
+ * @module steppanelsstyle
+ *
+ */
+import { BaseStyle } from '../../base/style';
+
+export enum StepPanelsClasses {
+ /**
+ * Class name of the root element
+ */
+ root = 'p-steppanels'
+}
+
+export interface StepPanelsStyle extends BaseStyle {}
diff --git a/components/lib/steppanels/style/StepPanelsStyle.js b/components/lib/steppanels/style/StepPanelsStyle.js
new file mode 100644
index 000000000..1a13c986b
--- /dev/null
+++ b/components/lib/steppanels/style/StepPanelsStyle.js
@@ -0,0 +1,10 @@
+import BaseStyle from 'primevue/base/style';
+
+const classes = {
+ root: 'p-steppanels'
+};
+
+export default BaseStyle.extend({
+ name: 'steppanels',
+ classes
+});
diff --git a/components/lib/steppanels/style/package.json b/components/lib/steppanels/style/package.json
new file mode 100644
index 000000000..c327af15f
--- /dev/null
+++ b/components/lib/steppanels/style/package.json
@@ -0,0 +1,6 @@
+{
+ "main": "./steppanelsstyle.mjs",
+ "module": "./steppanelsstyle.mjs",
+ "types": "./StepPanelsStyle.d.ts",
+ "sideEffects": false
+}
diff --git a/components/lib/stepper/BaseStepper.vue b/components/lib/stepper/BaseStepper.vue
index 12968cb3f..4c090a472 100644
--- a/components/lib/stepper/BaseStepper.vue
+++ b/components/lib/stepper/BaseStepper.vue
@@ -6,13 +6,9 @@ export default {
name: 'BaseStepper',
extends: BaseComponent,
props: {
- activeStep: {
- type: Number,
- default: 0
- },
- orientation: {
- type: String,
- default: 'horizontal'
+ value: {
+ type: [String, Number],
+ default: undefined
},
linear: {
type: Boolean,
diff --git a/components/lib/stepper/Stepper.d.ts b/components/lib/stepper/Stepper.d.ts
index 459fdeef6..50ee46c36 100644
--- a/components/lib/stepper/Stepper.d.ts
+++ b/components/lib/stepper/Stepper.d.ts
@@ -10,7 +10,6 @@
import { VNode } from 'vue';
import { ComponentHooks } from '../basecomponent';
import { PassThroughOptions } from '../passthrough';
-import { StepperPanelPassThroughOptionType } from '../stepperpanel';
import { DefineComponent, DesignToken, EmitFn, GlobalComponentConstructor, PassThrough } from '../ts-helpers';
export declare type StepperPassThroughOptionType = StepperPassThroughAttributes | ((options: StepperPassThroughMethodOptions) => StepperPassThroughAttributes | string) | string | null | undefined;
@@ -54,18 +53,6 @@ export interface StepperPassThroughOptions {
* Used to pass attributes to the root's DOM element.
*/
root?: StepperPassThroughOptionType;
- /**
- * Used to pass attributes to the list's DOM element.
- */
- list?: StepperPassThroughOptionType;
- /**
- * Used to pass attributes to the panels' DOM element.
- */
- panels?: StepperPassThroughOptionType;
- /**
- * Used to pass attributes to the end handler's DOM element.
- */
- stepperpanel?: StepperPanelPassThroughOptionType;
/**
* Used to manage all lifecycle hooks.
* @see {@link BaseComponent.ComponentHooks}
@@ -85,44 +72,24 @@ export interface StepperPassThroughAttributes {
*/
export interface StepperState {
/**
- * Current active index state.
+ * Current active value state.
*/
- d_activeStep: number;
+ d_value: any;
/**
* Unique id for the Stepper component.
*/
id: string;
}
-/**
- * Custom tab change event.
- * @see {@link StepperEmitsOptions['step-change']}
- */
-export interface StepperChangeEvent {
- /**
- * Browser event
- */
- originalEvent: Event;
- /**
- * Index of the selected stepper panel
- */
- index: number;
-}
-
/**
* Defines valid properties in Stepper component.
*/
export interface StepperProps {
/**
- * Active step index of stepper.
- * @defaultValue 0
+ * Active value of stepper.
+ * @defaultValue null
*/
- activeStep?: number | undefined;
- /**
- * Orientation of the stepper.
- * @defaultValue horizontal
- */
- orientation?: 'horizontal' | 'vertical' | undefined;
+ value?: string | number | undefined;
/**
* Whether the steps are clickable or not.
* @defaultValue false
@@ -153,6 +120,10 @@ export interface StepperProps {
* Defines valid slots in Stepper component.
*/
export interface StepperSlots {
+ /**
+ * Custom default template.
+ */
+ default(): VNode[];
/**
* Custom start template.
*/
@@ -169,13 +140,9 @@ export interface StepperSlots {
export interface StepperEmitsOptions {
/**
* Emitted when the value changes.
- * @param {number | number[]} value - New value.
+ * @param {any} value - New value.
*/
- 'update:activeStep'(value: number): void;
- /**
- * Callback to invoke when an active panel is changed.
- */
- 'step-change'(event: StepperChangeEvent): void;
+ 'update:value'(value: any): void;
}
export declare type StepperEmits = EmitFn;
diff --git a/components/lib/stepper/Stepper.vue b/components/lib/stepper/Stepper.vue
index e8d50241a..2fd0a64a3 100644
--- a/components/lib/stepper/Stepper.vue
+++ b/components/lib/stepper/Stepper.vue
@@ -1,266 +1,50 @@
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
+
diff --git a/components/lib/stepper/StepperContent.vue b/components/lib/stepper/StepperContent.vue
deleted file mode 100644
index 6ef36c5fe..000000000
--- a/components/lib/stepper/StepperContent.vue
+++ /dev/null
@@ -1,47 +0,0 @@
-
-
-
-
-
-
-
-
diff --git a/components/lib/stepper/StepperHeader.vue b/components/lib/stepper/StepperHeader.vue
deleted file mode 100644
index 39ccd4800..000000000
--- a/components/lib/stepper/StepperHeader.vue
+++ /dev/null
@@ -1,41 +0,0 @@
-
-
-
-
-
-
diff --git a/components/lib/stepper/StepperSeparator.vue b/components/lib/stepper/StepperSeparator.vue
index e071b1bec..bb1ded267 100644
--- a/components/lib/stepper/StepperSeparator.vue
+++ b/components/lib/stepper/StepperSeparator.vue
@@ -1,6 +1,5 @@
-
-
+
diff --git a/components/lib/stepper/style/StepperStyle.d.ts b/components/lib/stepper/style/StepperStyle.d.ts
index a902718a6..342acd555 100644
--- a/components/lib/stepper/style/StepperStyle.d.ts
+++ b/components/lib/stepper/style/StepperStyle.d.ts
@@ -15,45 +15,9 @@ export enum StepperClasses {
*/
root = 'p-stepper',
/**
- * Class name of the list element
+ * Class name of the separator element
*/
- list = 'p-stepper-list',
- /**
- * Class name of the stepper item element
- */
- stepperItem = 'p-stepper-item',
- /**
- * Class name of the stepper item header element
- */
- stepperItemHeader = 'p-stepper-item-header',
- /**
- * Class name of the stepper item number element
- */
- stepperItemNumber = 'p-stepper-item-number',
- /**
- * Class name of the stepper item title element
- */
- stepperItemTitle = 'p-stepper-item-title',
- /**
- * Class name of the stepper separator element
- */
- stepperSeparator = 'p-stepper-separator',
- /**
- * Class name of the stepper panel content container element
- */
- stepperPanelContentContainer = 'p-stepper-panel-content-container',
- /**
- * Class name of the stepper panel content element
- */
- stepperPanelContent = 'p-stepper-panel-content',
- /**
- * Class name of the panels element
- */
- panels = 'p-stepper-panels',
- /**
- * Class name of the panel element
- */
- panel = 'p-stepper-panel'
+ separator = 'p-stepper-separator'
}
export interface StepperStyle extends BaseStyle {}
diff --git a/components/lib/stepper/style/StepperStyle.js b/components/lib/stepper/style/StepperStyle.js
index 113e8c5f8..80d95f6e9 100644
--- a/components/lib/stepper/style/StepperStyle.js
+++ b/components/lib/stepper/style/StepperStyle.js
@@ -1,7 +1,7 @@
import BaseStyle from 'primevue/base/style';
const theme = ({ dt }) => `
-.p-stepper-list {
+.p-steplist {
position: relative;
display: flex;
justify-content: space-between;
@@ -12,20 +12,20 @@ const theme = ({ dt }) => `
overflow-x: auto;
}
-.p-stepper-item {
+.p-step {
position: relative;
display: flex;
flex: 1 1 auto;
align-items: center;
- gap: ${dt('stepper.item.gap')};
- padding: ${dt('stepper.item.padding')};
+ gap: ${dt('stepper.step.gap')};
+ padding: ${dt('stepper.step.padding')};
}
-.p-stepper-item:last-of-type {
+.p-step:last-of-type {
flex: initial;
}
-.p-stepper-item-header {
+.p-step-header {
border: 0 none;
display: inline-flex;
align-items: center;
@@ -34,82 +34,86 @@ const theme = ({ dt }) => `
transition: background ${dt('stepper.transition.duration')}, color ${dt('stepper.transition.duration')}, border-color ${dt('stepper.transition.duration')}, outline-color ${dt('stepper.transition.duration')}, box-shadow ${dt(
'stepper.transition.duration'
)};
- border-radius: ${dt('stepper.item.header.border.radius')};
+ border-radius: ${dt('stepper.step.header.border.radius')};
outline-color: transparent;
background: transparent;
- padding: ${dt('stepper.item.header.padding')};
- gap: ${dt('stepper.item.header.gap')};
+ padding: ${dt('stepper.step.header.padding')};
+ gap: ${dt('stepper.step.header.gap')};
}
-.p-stepper-item-header:focus-visible {
- box-shadow: ${dt('stepper.item.header.focus.ring.shadow')};
- outline: ${dt('stepper.item.header.focus.ring.width')} ${dt('stepper.item.header.focus.ring.style')} ${dt('stepper.item.header.focus.ring.color')};
- outline-offset: ${dt('stepper.item.header.focus.ring.offset')};
+.p-step-header:focus-visible {
+ box-shadow: ${dt('stepper.step.header.focus.ring.shadow')};
+ outline: ${dt('stepper.step.header.focus.ring.width')} ${dt('stepper.step.header.focus.ring.style')} ${dt('stepper.step.header.focus.ring.color')};
+ outline-offset: ${dt('stepper.step.header.focus.ring.offset')};
}
-.p-stepper.p-stepper-readonly .p-stepper-item {
+.p-stepper.p-stepper-readonly .p-step {
cursor: auto;
}
-.p-stepper-item-title {
+.p-step-title {
display: block;
white-space: nowrap;
overflow: hidden;
text-overflow: ellipsis;
max-width: 100%;
- color: ${dt('stepper.item.title.color')};
- font-weight: ${dt('stepper.item.title.font.weight')};
+ color: ${dt('stepper.step.title.color')};
+ font-weight: ${dt('stepper.step.title.font.weight')};
transition: background ${dt('stepper.transition.duration')}, color ${dt('stepper.transition.duration')}, border-color ${dt('stepper.transition.duration')}, box-shadow ${dt('stepper.transition.duration')}, outline-color ${dt(
'stepper.transition.duration'
)};
}
-.p-stepper-item-number {
+.p-step-number {
display: flex;
align-items: center;
justify-content: center;
- color: ${dt('stepper.item.number.color')};
- border: 2px solid ${dt('stepper.item.number.border.color')};
- background: ${dt('stepper.item.number.background')};
- min-width: ${dt('stepper.item.number.size')};
- height: ${dt('stepper.item.number.size')};
- line-height: ${dt('stepper.item.number.size')};
- font-size: ${dt('stepper.item.number.font.size')};
+ color: ${dt('stepper.step.number.color')};
+ border: 2px solid ${dt('stepper.step.number.border.color')};
+ background: ${dt('stepper.step.number.background')};
+ min-width: ${dt('stepper.step.number.size')};
+ height: ${dt('stepper.step.number.size')};
+ line-height: ${dt('stepper.step.number.size')};
+ font-size: ${dt('stepper.step.number.font.size')};
z-index: 1;
- border-radius: ${dt('stepper.item.number.border.radius')};
+ border-radius: ${dt('stepper.step.number.border.radius')};
position: relative;
- font-weight: ${dt('stepper.item.number.font.weight')};
+ font-weight: ${dt('stepper.step.number.font.weight')};
}
-.p-stepper-item-number::after {
+.p-step-number::after {
content: " ";
position: absolute;
width: 100%;
height: 100%;
- border-radius: ${dt('stepper.item.number.border.radius')};
- box-shadow: ${dt('stepper.item.number.shadow')};
+ border-radius: ${dt('stepper.step.number.border.radius')};
+ box-shadow: ${dt('stepper.step.number.shadow')};
}
-.p-stepper-item-active .p-stepper-item-header {
+.p-step-active .p-step-header {
cursor: default;
}
-.p-stepper-item-active .p-stepper-item-number {
- background: ${dt('stepper.item.number.active.background')};
- border-color: ${dt('stepper.item.number.active.border.color')};
- color: ${dt('stepper.item.number.active.color')};
+.p-step-active .p-step-number {
+ background: ${dt('stepper.step.number.active.background')};
+ border-color: ${dt('stepper.step.number.active.border.color')};
+ color: ${dt('stepper.step.number.active.color')};
}
-.p-stepper-item-active .p-stepper-item-title {
- color: ${dt('stepper.item.title.active.color')};
+.p-step-active .p-step-title {
+ color: ${dt('stepper.step.title.active.color')};
}
-.p-stepper-item:not(.p-disabled):focus-visible {
+.p-step:not(.p-disabled):focus-visible {
outline: ${dt('focus.ring.width')} ${dt('focus.ring.style')} ${dt('focus.ring.color')};
outline-offset: ${dt('focus.ring.offset')};
}
-.p-stepper-item:has(~ .p-stepper-item-active) .p-stepper-separator {
+.p-step:has(~ .p-step-active) .p-stepper-separator {
+ background: ${dt('stepper.separator.active.background')};
+}
+
+.p-step:has(~ .p-step-active) .p-stepper-separator {
background: ${dt('stepper.separator.active.background')};
}
@@ -123,63 +127,59 @@ const theme = ({ dt }) => `
)};
}
-.p-stepper-panels {
- padding: ${dt('stepper.panel.content.orientation.horizontal.padding')};
+.p-steppanels {
+ padding: ${dt('stepper.steppanels.padding')};
}
-.p-stepper-panel-content {
- background: ${dt('stepper.panel.content.background')};
- color: ${dt('stepper.panel.content.color')};
+.p-steppanel {
+ background: ${dt('stepper.steppanel.content.background')};
+ color: ${dt('stepper.steppanel.content.color')};
}
-.p-stepper-vertical .p-stepper-list {
- flex-direction: column;
-}
-
-.p-stepper-vertical {
+.p-stepper:has(.p-stepitem) {
display: flex;
flex-direction: column;
}
-.p-stepper-vertical .p-stepper-panel-content-container {
- display: flex;
- flex: 1 1 auto;
-}
-
-.p-stepper-vertical .p-stepper-panel {
+.p-stepitem {
display: flex;
flex-direction: column;
flex: initial;
}
-.p-stepper-vertical .p-stepper-panel.p-stepper-panel-active {
+.p-stepitem.p-stepitem-active {
flex: 1 1 auto;
}
-.p-stepper-vertical .p-stepper-panel .p-stepper-item {
+.p-stepitem .p-step {
flex: initial;
}
-.p-stepper-vertical .p-stepper-panel .p-stepper-panel-content {
+.p-stepitem .p-steppanel-content {
width: 100%;
- padding: ${dt('stepper.panel.content.orientation.vertical.padding')};
+ padding: ${dt('stepper.steppanel.padding')};
}
-.p-stepper-vertical .p-stepper-panel .p-stepper-separator {
+.p-stepitem .p-steppanel {
+ display: flex;
+ flex: 1 1 auto;
+}
+
+.p-stepitem .p-stepper-separator {
flex: 0 0 auto;
width: ${dt('stepper.separator.size')};
height: auto;
- margin: ${dt('stepper.separator.orientation.vertical.margin')};
+ margin: ${dt('stepper.separator.margin')};
position: relative;
- left: calc(-1 * ${dt('stepper.separator.size')} / 2);
+ left: calc(-1 * ${dt('stepper.separator.size')});
}
-.p-stepper-vertical .p-stepper-panel:has(~ .p-stepper-panel-active) .p-stepper-separator {
- background: ${dt('stepper.connector.active.background')};
+.p-stepitem:has(~ .p-stepitem-active) .p-stepper-separator {
+ background: ${dt('stepper.separator.active.background')};
}
-.p-stepper-vertical .p-stepper-panel:last-of-type .p-stepper-panel-content {
- padding: ${dt('stepper.panel.content.orientation.vertical.last.padding')};
+.p-stepitem:last-of-type .p-steppanel {
+ padding: ${dt('stepper.steppanel.last.padding')};
}
`;
@@ -187,34 +187,10 @@ const classes = {
root: ({ props }) => [
'p-stepper p-component',
{
- 'p-stepper-horizontal': props.orientation === 'horizontal',
- 'p-stepper-vertical': props.orientation === 'vertical',
'p-readonly': props.linear
}
],
- list: 'p-stepper-list',
- stepper: {
- item: ({ instance, step, index }) => [
- 'p-stepper-item',
- {
- 'p-stepper-item-active': instance.isStepActive(index),
- 'p-disabled': instance.isItemDisabled(index)
- }
- ],
- itemHeader: 'p-stepper-item-header',
- itemNumber: 'p-stepper-item-number',
- itemTitle: 'p-stepper-item-title',
- separator: 'p-stepper-separator',
- panelContentContainer: 'p-stepper-panel-content-container',
- panelContent: 'p-stepper-panel-content'
- },
- panels: 'p-stepper-panels',
- panel: ({ instance, props, index }) => [
- 'p-stepper-panel',
- {
- 'p-stepper-panel-active': props.orientation === 'vertical' && instance.isStepActive(index)
- }
- ]
+ separator: 'p-stepper-separator'
};
export default BaseStyle.extend({
diff --git a/components/lib/stepperpanel/StepperPanel.d.ts b/components/lib/stepperpanel/StepperPanel.d.ts
index 29f81f603..37bc70166 100644
--- a/components/lib/stepperpanel/StepperPanel.d.ts
+++ b/components/lib/stepperpanel/StepperPanel.d.ts
@@ -266,6 +266,8 @@ export interface StepperPanelEmitsOptions {}
export declare type StepperPanelEmits = EmitFn;
/**
+ * @deprecated since v4. Use the new structure of Stepper instead.
+ *
* **PrimeVue - StepperPanel**
*
* _StepperPanel is a helper component for Stepper component._
diff --git a/components/lib/stepperpanel/StepperPanel.vue b/components/lib/stepperpanel/StepperPanel.vue
index 47865642f..e586bccec 100644
--- a/components/lib/stepperpanel/StepperPanel.vue
+++ b/components/lib/stepperpanel/StepperPanel.vue
@@ -7,6 +7,9 @@ import BaseStepperPanel from './BaseStepperPanel.vue';
export default {
name: 'StepperPanel',
- extends: BaseStepperPanel
+ extends: BaseStepperPanel,
+ mounted() {
+ console.warn('Deprecated since v4. Use the new structure of Stepper instead.');
+ }
};
diff --git a/components/lib/themes/aura/stepper/index.js b/components/lib/themes/aura/stepper/index.js
index a907a45e6..c8af64c00 100644
--- a/components/lib/themes/aura/stepper/index.js
+++ b/components/lib/themes/aura/stepper/index.js
@@ -5,18 +5,14 @@ export default {
separator: {
background: '{content.border.color}',
activeBackground: '{primary.color}',
- orientation: {
- vertical: {
- margin: '0 0 0 1.625rem'
- }
- },
+ margin: '0 0 0 1.625rem',
size: '2px'
},
- item: {
+ step: {
padding: '0.5rem',
gap: '1rem'
},
- itemHeader: {
+ stepHeader: {
padding: '0',
borderRadius: '{content.border.radius}',
focusRing: {
@@ -28,12 +24,12 @@ export default {
},
gap: '0.5rem'
},
- itemTitle: {
+ stepTitle: {
color: '{text.muted.color}',
activeColor: '{primary.color}',
fontWeight: '500'
},
- itemNumber: {
+ stepNumber: {
background: '{content.background}',
activeBackground: '{content.background}',
borderColor: '{content.border.color}',
@@ -46,22 +42,15 @@ export default {
borderRadius: '50%',
shadow: '0px 0.5px 0px 0px rgba(0, 0, 0, 0.06), 0px 1px 1px 0px rgba(0, 0, 0, 0.12)'
},
- verticalPanelContainer: {
- paddingLeft: '1rem'
+ steppanels: {
+ padding: '0.875rem 0.5rem 1.125rem 0.5rem'
},
- panelContent: {
+ steppanel: {
background: '{content.background}',
color: '{content.color}',
- orientation: {
- horizontal: {
- padding: '0.875rem 0.5rem 1.125rem 0.5rem'
- },
- vertical: {
- padding: '0 0 0 1rem',
- last: {
- padding: '0 0 0 3rem'
- }
- }
+ padding: '0 0 0 1rem',
+ last: {
+ padding: '0 0 0 1.625rem'
}
}
};
diff --git a/components/lib/themes/lara/stepper/index.js b/components/lib/themes/lara/stepper/index.js
index 07e53819b..7f5adf697 100644
--- a/components/lib/themes/lara/stepper/index.js
+++ b/components/lib/themes/lara/stepper/index.js
@@ -5,18 +5,14 @@ export default {
separator: {
background: '{content.border.color}',
activeBackground: '{primary.color}',
- orientation: {
- vertical: {
- margin: '0 0 0 1.625rem'
- }
- },
+ margin: '0 0 0 1.625rem',
size: '2px'
},
- item: {
+ step: {
padding: '0.5rem',
gap: '1rem'
},
- itemHeader: {
+ stepHeader: {
padding: '0',
borderRadius: '{content.border.radius}',
focusRing: {
@@ -28,12 +24,12 @@ export default {
},
gap: '0.5rem'
},
- itemTitle: {
+ stepTitle: {
color: '{text.muted.color}',
activeColor: '{primary.color}',
fontWeight: '500'
},
- itemNumber: {
+ stepNumber: {
background: '{content.background}',
activeBackground: '{primary.color}',
borderColor: '{content.border.color}',
@@ -46,22 +42,15 @@ export default {
borderRadius: '50%',
shadow: 'none'
},
- verticalPanelContainer: {
- paddingLeft: '1rem'
+ steppanels: {
+ padding: '0.875rem 0.5rem 1.125rem 0.5rem'
},
- panelContent: {
+ steppanel: {
background: '{content.background}',
color: '{content.color}',
- orientation: {
- horizontal: {
- padding: '0.875rem 0.5rem 1.125rem 0.5rem'
- },
- vertical: {
- padding: '0 0 0 1rem',
- last: {
- padding: '0 0 0 3rem'
- }
- }
+ padding: '0 0 0 1rem',
+ last: {
+ padding: '0 0 0 1.625rem'
}
}
};
diff --git a/components/lib/themes/nora/stepper/index.js b/components/lib/themes/nora/stepper/index.js
index 11e4af71e..bfcffb021 100644
--- a/components/lib/themes/nora/stepper/index.js
+++ b/components/lib/themes/nora/stepper/index.js
@@ -5,18 +5,14 @@ export default {
separator: {
background: '{content.border.color}',
activeBackground: '{primary.color}',
- orientation: {
- vertical: {
- margin: '0 0 0 1.625rem'
- }
- },
+ margin: '0 0 0 1.625rem',
size: '2px'
},
- item: {
+ step: {
padding: '0.5rem',
gap: '1rem'
},
- itemHeader: {
+ stepHeader: {
padding: '0',
borderRadius: '{content.border.radius}',
focusRing: {
@@ -28,12 +24,12 @@ export default {
},
gap: '0.5rem'
},
- itemTitle: {
+ stepTitle: {
color: '{text.muted.color}',
activeColor: '{primary.color}',
fontWeight: '700'
},
- itemNumber: {
+ stepNumber: {
background: '{content.background}',
activeBackground: '{primary.color}',
borderColor: '{content.border.color}',
@@ -46,22 +42,15 @@ export default {
borderRadius: '50%',
shadow: 'none'
},
- verticalPanelContainer: {
- paddingLeft: '1rem'
+ steppanels: {
+ padding: '0.875rem 0.5rem 1.125rem 0.5rem'
},
- panelContent: {
+ steppanel: {
background: '{content.background}',
color: '{content.color}',
- orientation: {
- horizontal: {
- padding: '0.875rem 0.5rem 1.125rem 0.5rem'
- },
- vertical: {
- padding: '0 0 0 1rem',
- last: {
- padding: '0 0 0 3rem'
- }
- }
+ padding: '0 0 0 1rem',
+ last: {
+ padding: '0 0 0 1.625rem'
}
}
};
diff --git a/components/lib/themes/types/stepper/index.d.ts b/components/lib/themes/types/stepper/index.d.ts
index f141475d3..0bf63908a 100644
--- a/components/lib/themes/types/stepper/index.d.ts
+++ b/components/lib/themes/types/stepper/index.d.ts
@@ -39,21 +39,11 @@ export interface StepperDesignTokens extends ColorSchemeDesignToken