Paginator .d.ts updated
parent
c4c39c8c9f
commit
056f644a17
|
@ -1,6 +1,18 @@
|
|||
/**
|
||||
*
|
||||
* Paginator is a generic component to display content in paged format.
|
||||
*
|
||||
* - [Paginator](https://www.primefaces.org/primevue/paginator)
|
||||
*
|
||||
* @module paginator
|
||||
*
|
||||
*/
|
||||
import { VNode } from 'vue';
|
||||
import { ClassComponent, GlobalComponentConstructor } from '../ts-helpers';
|
||||
|
||||
/**
|
||||
* Paginator page state metadata.
|
||||
*/
|
||||
export interface PageState {
|
||||
/**
|
||||
* Index of first record
|
||||
|
@ -20,25 +32,28 @@ export interface PageState {
|
|||
pageCount?: number;
|
||||
}
|
||||
|
||||
/**
|
||||
* Defines valid properties in Paginator component.
|
||||
*/
|
||||
export interface PaginatorProps {
|
||||
/**
|
||||
* Number of total records.
|
||||
* Default value is 0.
|
||||
* @defaultValue 0
|
||||
*/
|
||||
totalRecords?: number | undefined;
|
||||
/**
|
||||
* Data count to display per page.
|
||||
* Default value is 0.
|
||||
* @defaultValue 0
|
||||
*/
|
||||
rows?: number | undefined;
|
||||
/**
|
||||
* Zero-relative number of the first row to be displayed.
|
||||
* Default value is 0.
|
||||
* @defaultValue 0
|
||||
*/
|
||||
first?: number | undefined;
|
||||
/**
|
||||
* Number of page links to display.
|
||||
* Default value is 5.
|
||||
* @defaultValue 5
|
||||
*/
|
||||
pageLinkSize?: number | undefined;
|
||||
/**
|
||||
|
@ -60,7 +75,7 @@ export interface PaginatorProps {
|
|||
*/
|
||||
template?: any | string;
|
||||
/**
|
||||
* Template of the current page report element. It displays information about the pagination state. Default value is ({currentPage} of {totalPages}) whereas available placeholders are the following;
|
||||
* Template of the current page report element. It displays information about the pagination state. Available placeholders are the following;
|
||||
*
|
||||
* - {currentPage}
|
||||
* - {totalPages}
|
||||
|
@ -68,58 +83,77 @@ export interface PaginatorProps {
|
|||
* - {first}
|
||||
* - {last}
|
||||
* - {totalRecords}
|
||||
*
|
||||
* @defaultValue ({currentPage} of {totalPages})
|
||||
*/
|
||||
currentPageReportTemplate?: string | undefined;
|
||||
/**
|
||||
* Whether to show the paginator even there is only one page.
|
||||
* Default value is true.
|
||||
* @defaultValue true
|
||||
*/
|
||||
alwaysShow?: boolean | undefined;
|
||||
}
|
||||
|
||||
/**
|
||||
* Defines valid slots in Paginator component.
|
||||
*/
|
||||
export interface PaginatorSlots {
|
||||
/**
|
||||
* Custom start template.
|
||||
* @param {Object} scope - start slot's params.
|
||||
*/
|
||||
start: (scope: {
|
||||
start(scope: {
|
||||
/**
|
||||
* Current state
|
||||
* @see PageState
|
||||
*/
|
||||
state: PageState;
|
||||
}) => VNode[];
|
||||
}): VNode[];
|
||||
/**
|
||||
* Custom end template.
|
||||
* @param {Object} scope - end slot's params.
|
||||
*/
|
||||
end: (scope: {
|
||||
end(scope: {
|
||||
/**
|
||||
* Current state
|
||||
* @see PageState
|
||||
*/
|
||||
state: PageState;
|
||||
}) => VNode[];
|
||||
}): VNode[];
|
||||
}
|
||||
|
||||
export declare type PaginatorEmits = {
|
||||
/**
|
||||
* Defines valid emits in Paginator component.
|
||||
*/
|
||||
export interface PaginatorEmits {
|
||||
/**
|
||||
* Emitted when the first changes.
|
||||
* @param {number} value - New value.
|
||||
*/
|
||||
'update:first': (value: number) => void;
|
||||
'update:first'(value: number): void;
|
||||
/**
|
||||
* Emitted when the rows changes.
|
||||
* @param {number} value - New value.
|
||||
*/
|
||||
'update:rows': (value: number) => void;
|
||||
'update:rows'(value: number): void;
|
||||
/**
|
||||
* Callback to invoke when page changes, the event object contains information about the new state.
|
||||
* @param {PageState} event - New page state.
|
||||
*/
|
||||
page: (event: PageState) => void;
|
||||
};
|
||||
page(event: PageState): void;
|
||||
}
|
||||
|
||||
/**
|
||||
* **PrimeVue - Paginator**
|
||||
*
|
||||
* _Paginator is a generic widget to display content in paged format._
|
||||
*
|
||||
* [Live Demo](https://www.primevue.org/paginator/)
|
||||
* --- ---
|
||||
* 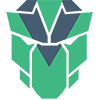
|
||||
*
|
||||
* @group Component
|
||||
*/
|
||||
declare class Paginator extends ClassComponent<PaginatorProps, PaginatorSlots, PaginatorEmits> {}
|
||||
|
||||
declare module '@vue/runtime-core' {
|
||||
|
@ -128,13 +162,4 @@ declare module '@vue/runtime-core' {
|
|||
}
|
||||
}
|
||||
|
||||
/**
|
||||
*
|
||||
* Paginator is a generic component to display content in paged format.
|
||||
*
|
||||
* Demos:
|
||||
*
|
||||
* - [Paginator](https://www.primefaces.org/primevue/paginator)
|
||||
*
|
||||
*/
|
||||
export default Paginator;
|
||||
|
|
Loading…
Reference in New Issue