Initiated InputOtp
parent
c555e258c0
commit
067da063a8
|
@ -0,0 +1,80 @@
|
||||||
|
const InputSwitchProps = [
|
||||||
|
{
|
||||||
|
name: 'modelValue',
|
||||||
|
type: 'boolean',
|
||||||
|
default: 'null',
|
||||||
|
description: 'Specifies whether a inputswitch should be checked or not.'
|
||||||
|
},
|
||||||
|
{
|
||||||
|
name: 'trueValue',
|
||||||
|
type: 'any',
|
||||||
|
default: 'null',
|
||||||
|
description: 'Value in checked state.'
|
||||||
|
},
|
||||||
|
{
|
||||||
|
name: 'falseValue',
|
||||||
|
type: 'any',
|
||||||
|
default: 'null',
|
||||||
|
description: 'Value in unchecked state.'
|
||||||
|
},
|
||||||
|
{
|
||||||
|
name: 'inputId',
|
||||||
|
type: 'string',
|
||||||
|
default: 'null',
|
||||||
|
description: 'Identifier of the underlying input element.'
|
||||||
|
},
|
||||||
|
{
|
||||||
|
name: 'inputStyle',
|
||||||
|
type: 'object',
|
||||||
|
default: 'null',
|
||||||
|
description: 'Inline style of the input field.'
|
||||||
|
},
|
||||||
|
{
|
||||||
|
name: 'inputClass',
|
||||||
|
type: 'string | object',
|
||||||
|
default: 'null',
|
||||||
|
description: 'Style class of the input field.'
|
||||||
|
},
|
||||||
|
{
|
||||||
|
name: 'inputProps',
|
||||||
|
type: 'object',
|
||||||
|
default: 'null',
|
||||||
|
description: 'Used to pass all properties of the HTMLInputElement to the focusable input element inside the component.'
|
||||||
|
},
|
||||||
|
{
|
||||||
|
name: 'pt',
|
||||||
|
type: 'any',
|
||||||
|
default: 'null',
|
||||||
|
description: 'Used to pass attributes to DOM elements inside the component.'
|
||||||
|
},
|
||||||
|
{
|
||||||
|
name: 'unstyled',
|
||||||
|
type: 'boolean',
|
||||||
|
default: 'false',
|
||||||
|
description: 'When enabled, it removes component related styles in the core.'
|
||||||
|
}
|
||||||
|
];
|
||||||
|
|
||||||
|
const InputSwitchEvents = [
|
||||||
|
{
|
||||||
|
name: 'click',
|
||||||
|
description: 'Callback to invoke on click.'
|
||||||
|
},
|
||||||
|
{
|
||||||
|
name: 'change',
|
||||||
|
description: 'Callback to invoke on value change.'
|
||||||
|
},
|
||||||
|
{
|
||||||
|
name: 'input',
|
||||||
|
description: 'Callback to invoke on value change.'
|
||||||
|
}
|
||||||
|
];
|
||||||
|
|
||||||
|
module.exports = {
|
||||||
|
inputswitch: {
|
||||||
|
name: 'InputSwitch',
|
||||||
|
description: 'InputSwitch is used to select a boolean value.',
|
||||||
|
props: InputSwitchProps,
|
||||||
|
events: InputSwitchEvents
|
||||||
|
}
|
||||||
|
};
|
|
@ -115,6 +115,11 @@
|
||||||
"name": "InputNumber",
|
"name": "InputNumber",
|
||||||
"to": "/inputnumber"
|
"to": "/inputnumber"
|
||||||
},
|
},
|
||||||
|
{
|
||||||
|
"name": "InputOtp",
|
||||||
|
"to": "/inputotp",
|
||||||
|
"badge": "NEW"
|
||||||
|
},
|
||||||
{
|
{
|
||||||
"name": "InputSwitch",
|
"name": "InputSwitch",
|
||||||
"to": "/inputswitch"
|
"to": "/inputswitch"
|
||||||
|
|
|
@ -0,0 +1,53 @@
|
||||||
|
<script>
|
||||||
|
import BaseComponent from 'primevue/basecomponent';
|
||||||
|
import InputOtpStyle from 'primevue/inputotp/style';
|
||||||
|
|
||||||
|
export default {
|
||||||
|
name: 'BaseInputOtp',
|
||||||
|
extends: BaseComponent,
|
||||||
|
props: {
|
||||||
|
modelValue: {
|
||||||
|
type: null,
|
||||||
|
default: false
|
||||||
|
},
|
||||||
|
invalid: {
|
||||||
|
type: Boolean,
|
||||||
|
default: false
|
||||||
|
},
|
||||||
|
disabled: {
|
||||||
|
type: Boolean,
|
||||||
|
default: false
|
||||||
|
},
|
||||||
|
readonly: {
|
||||||
|
type: Boolean,
|
||||||
|
default: false
|
||||||
|
},
|
||||||
|
variant: {
|
||||||
|
type: String,
|
||||||
|
default: null
|
||||||
|
},
|
||||||
|
tabindex: {
|
||||||
|
type: Number,
|
||||||
|
default: null
|
||||||
|
},
|
||||||
|
length: {
|
||||||
|
type: Number,
|
||||||
|
default: 4
|
||||||
|
},
|
||||||
|
mask: {
|
||||||
|
type: Boolean,
|
||||||
|
default: false
|
||||||
|
},
|
||||||
|
integerOnly: {
|
||||||
|
type: Boolean,
|
||||||
|
default: false
|
||||||
|
}
|
||||||
|
},
|
||||||
|
style: InputOtpStyle,
|
||||||
|
provide() {
|
||||||
|
return {
|
||||||
|
$parentInstance: this
|
||||||
|
};
|
||||||
|
}
|
||||||
|
};
|
||||||
|
</script>
|
|
@ -0,0 +1,227 @@
|
||||||
|
/**
|
||||||
|
*
|
||||||
|
* InputSwitch is used to select a boolean value.
|
||||||
|
*
|
||||||
|
* [Live Demo](https://www.primevue.org/inputswitch/)
|
||||||
|
*
|
||||||
|
* @module inputotp
|
||||||
|
*
|
||||||
|
*/
|
||||||
|
import { ComponentHooks } from '../basecomponent/BaseComponent';
|
||||||
|
import { PassThroughOptions } from '../passthrough';
|
||||||
|
import { ClassComponent, GlobalComponentConstructor, PassThrough } from '../ts-helpers';
|
||||||
|
|
||||||
|
export declare type InputSwitchPassThroughOptionType = InputSwitchPassThroughAttributes | ((options: InputSwitchPassThroughMethodOptions) => InputSwitchPassThroughAttributes | string) | string | null | undefined;
|
||||||
|
|
||||||
|
/**
|
||||||
|
* Custom passthrough(pt) option method.
|
||||||
|
*/
|
||||||
|
export interface InputSwitchPassThroughMethodOptions {
|
||||||
|
/**
|
||||||
|
* Defines instance.
|
||||||
|
*/
|
||||||
|
instance: any;
|
||||||
|
/**
|
||||||
|
* Defines valid properties.
|
||||||
|
*/
|
||||||
|
props: InputSwitchProps;
|
||||||
|
/**
|
||||||
|
* Defines current inline state.
|
||||||
|
*/
|
||||||
|
state: InputSwitchState;
|
||||||
|
/**
|
||||||
|
* Defines current options.
|
||||||
|
*/
|
||||||
|
context: InputSwitchContext;
|
||||||
|
/**
|
||||||
|
* Defines valid attributes.
|
||||||
|
*/
|
||||||
|
attrs: any;
|
||||||
|
/**
|
||||||
|
* Defines parent options.
|
||||||
|
*/
|
||||||
|
parent: any;
|
||||||
|
/**
|
||||||
|
* Defines passthrough(pt) options in global config.
|
||||||
|
*/
|
||||||
|
global: object | undefined;
|
||||||
|
}
|
||||||
|
|
||||||
|
/**
|
||||||
|
* Custom passthrough(pt) options.
|
||||||
|
* @see {@link InputSwitchProps.pt}
|
||||||
|
*/
|
||||||
|
export interface InputSwitchPassThroughOptions {
|
||||||
|
/**
|
||||||
|
* Used to pass attributes to the root's DOM element.
|
||||||
|
*/
|
||||||
|
root?: InputSwitchPassThroughOptionType;
|
||||||
|
/**
|
||||||
|
* Used to pass attributes to the input's DOM element.
|
||||||
|
*/
|
||||||
|
input?: InputSwitchPassThroughOptionType;
|
||||||
|
/**
|
||||||
|
* Used to pass attributes to the slider's DOM element.
|
||||||
|
*/
|
||||||
|
slider?: InputSwitchPassThroughOptionType;
|
||||||
|
/**
|
||||||
|
* Used to manage all lifecycle hooks.
|
||||||
|
* @see {@link BaseComponent.ComponentHooks}
|
||||||
|
*/
|
||||||
|
hooks?: ComponentHooks;
|
||||||
|
}
|
||||||
|
|
||||||
|
/**
|
||||||
|
* Custom passthrough attributes for each DOM elements
|
||||||
|
*/
|
||||||
|
export interface InputSwitchPassThroughAttributes {
|
||||||
|
[key: string]: any;
|
||||||
|
}
|
||||||
|
|
||||||
|
/**
|
||||||
|
* Defines current inline state in InputSwitch component.
|
||||||
|
*/
|
||||||
|
export interface InputSwitchState {
|
||||||
|
[key: string]: any;
|
||||||
|
}
|
||||||
|
|
||||||
|
/**
|
||||||
|
* Defines valid properties in InputSwitch component.
|
||||||
|
*/
|
||||||
|
export interface InputSwitchProps {
|
||||||
|
/**
|
||||||
|
* Specifies whether a inputswitch should be checked or not.
|
||||||
|
* @defaultValue false
|
||||||
|
*/
|
||||||
|
modelValue?: boolean | string | undefined;
|
||||||
|
/**
|
||||||
|
* Value in checked state.
|
||||||
|
* @defaultValue true
|
||||||
|
*/
|
||||||
|
trueValue?: any;
|
||||||
|
/**
|
||||||
|
* Value in unchecked state.
|
||||||
|
* @defaultValue false
|
||||||
|
*/
|
||||||
|
falseValue?: any;
|
||||||
|
/**
|
||||||
|
* When present, it specifies that the component should have invalid state style.
|
||||||
|
* @defaultValue false
|
||||||
|
*/
|
||||||
|
invalid?: boolean | undefined;
|
||||||
|
/**
|
||||||
|
* When present, it specifies that the component should be disabled.
|
||||||
|
* @defaultValue false
|
||||||
|
*/
|
||||||
|
disabled?: boolean | undefined;
|
||||||
|
/**
|
||||||
|
* When present, it specifies that an input field is read-only.
|
||||||
|
* @default false
|
||||||
|
*/
|
||||||
|
readonly?: boolean | undefined;
|
||||||
|
/**
|
||||||
|
* Index of the element in tabbing order.
|
||||||
|
*/
|
||||||
|
tabindex?: number | undefined;
|
||||||
|
/**
|
||||||
|
* Identifier of the underlying input element.
|
||||||
|
*/
|
||||||
|
inputId?: string | undefined;
|
||||||
|
/**
|
||||||
|
* Style class of the input field.
|
||||||
|
*/
|
||||||
|
inputClass?: string | object | undefined;
|
||||||
|
/**
|
||||||
|
* Inline style of the input field.
|
||||||
|
*/
|
||||||
|
inputStyle?: object | undefined;
|
||||||
|
/**
|
||||||
|
* Establishes relationships between the component and label(s) where its value should be one or more element IDs.
|
||||||
|
*/
|
||||||
|
ariaLabelledby?: string | undefined;
|
||||||
|
/**
|
||||||
|
* Establishes a string value that labels the component.
|
||||||
|
*/
|
||||||
|
ariaLabel?: string | undefined;
|
||||||
|
/**
|
||||||
|
* Used to pass attributes to DOM elements inside the component.
|
||||||
|
* @type {InputSwitchPassThroughOptions}
|
||||||
|
*/
|
||||||
|
pt?: PassThrough<InputSwitchPassThroughOptions>;
|
||||||
|
/**
|
||||||
|
* Used to configure passthrough(pt) options of the component.
|
||||||
|
* @type {PassThroughOptions}
|
||||||
|
*/
|
||||||
|
ptOptions?: PassThroughOptions;
|
||||||
|
/**
|
||||||
|
* When enabled, it removes component related styles in the core.
|
||||||
|
* @defaultValue false
|
||||||
|
*/
|
||||||
|
unstyled?: boolean;
|
||||||
|
}
|
||||||
|
|
||||||
|
/**
|
||||||
|
* Defines current options in InputSwitch component.
|
||||||
|
*/
|
||||||
|
export interface InputSwitchContext {
|
||||||
|
/**
|
||||||
|
* Current checked state of the item as a boolean.
|
||||||
|
* @defaultValue false
|
||||||
|
*/
|
||||||
|
checked: boolean;
|
||||||
|
/**
|
||||||
|
* Current disabled state of the item as a boolean.
|
||||||
|
* @defaultValue false
|
||||||
|
*/
|
||||||
|
disabled: boolean;
|
||||||
|
}
|
||||||
|
|
||||||
|
export interface InputSwitchSlots {}
|
||||||
|
|
||||||
|
/**
|
||||||
|
* Defines valid emits in InputSwitch component.
|
||||||
|
*/
|
||||||
|
export interface InputSwitchEmits {
|
||||||
|
/**
|
||||||
|
* Emitted when the value changes.
|
||||||
|
* @param {boolean} value - New value.
|
||||||
|
*/
|
||||||
|
'update:modelValue'(value: boolean): void;
|
||||||
|
/**
|
||||||
|
* Callback to invoke on value change.
|
||||||
|
* @param {Event} event - Browser event.
|
||||||
|
*/
|
||||||
|
change(event: Event): void;
|
||||||
|
/**
|
||||||
|
* Callback to invoke when the component receives focus.
|
||||||
|
* @param {Event} event - Browser event.
|
||||||
|
*/
|
||||||
|
focus(event: Event): void;
|
||||||
|
/**
|
||||||
|
* Callback to invoke when the component loses focus.
|
||||||
|
* @param {Event} event - Browser event.
|
||||||
|
*/
|
||||||
|
blur(event: Event): void;
|
||||||
|
}
|
||||||
|
|
||||||
|
/**
|
||||||
|
* **PrimeVue - InputSwitch**
|
||||||
|
*
|
||||||
|
* _InputSwitch is used to select a boolean value._
|
||||||
|
*
|
||||||
|
* [Live Demo](https://www.primevue.org/inputswitch/)
|
||||||
|
* --- ---
|
||||||
|
* 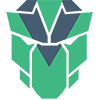
|
||||||
|
*
|
||||||
|
* @group Component
|
||||||
|
*
|
||||||
|
*/
|
||||||
|
declare class InputSwitch extends ClassComponent<InputSwitchProps, InputSwitchSlots, InputSwitchEmits> {}
|
||||||
|
|
||||||
|
declare module '@vue/runtime-core' {
|
||||||
|
interface GlobalComponents {
|
||||||
|
InputSwitch: GlobalComponentConstructor<InputSwitch>;
|
||||||
|
}
|
||||||
|
}
|
||||||
|
|
||||||
|
export default InputSwitch;
|
|
@ -0,0 +1,20 @@
|
||||||
|
import { mount } from '@vue/test-utils';
|
||||||
|
import InputSwitch from './InputSwitch.vue';
|
||||||
|
|
||||||
|
describe('InputSwitch.vue', () => {
|
||||||
|
it('should exist', async () => {
|
||||||
|
const wrapper = mount(InputSwitch);
|
||||||
|
|
||||||
|
expect(wrapper.find('.p-inputswitch.p-component').exists()).toBe(true);
|
||||||
|
expect(wrapper.find('.p-inputswitch-slider').exists()).toBe(true);
|
||||||
|
|
||||||
|
await wrapper.vm.onChange({});
|
||||||
|
|
||||||
|
expect(wrapper.emitted()['update:modelValue'][0]).toEqual([true]);
|
||||||
|
|
||||||
|
await wrapper.setProps({ modelValue: true });
|
||||||
|
|
||||||
|
expect(wrapper.vm.checked).toBe(true);
|
||||||
|
expect(wrapper.find('.p-inputswitch').classes()).toContain('p-highlight');
|
||||||
|
});
|
||||||
|
});
|
|
@ -0,0 +1,155 @@
|
||||||
|
<template>
|
||||||
|
<div :class="cx('root')" v-bind="ptmi('root')">
|
||||||
|
<OtpInputText
|
||||||
|
v-for="i in length"
|
||||||
|
:key="i"
|
||||||
|
v-model="tokens[i - 1]"
|
||||||
|
:type="inputType"
|
||||||
|
:class="cx('input')"
|
||||||
|
maxlength="1"
|
||||||
|
:inputmode="inputMode"
|
||||||
|
@input="onInput"
|
||||||
|
@focus="onFocus"
|
||||||
|
@blur="onBlur"
|
||||||
|
@paste="onPaste"
|
||||||
|
@keydown="onKeyDown($event, i - 1)"
|
||||||
|
:pt="ptm('input')"
|
||||||
|
/>
|
||||||
|
</div>
|
||||||
|
</template>
|
||||||
|
|
||||||
|
<script>
|
||||||
|
import InputText from 'primevue/inputtext';
|
||||||
|
import BaseInputOtp from './BaseInputOtp.vue';
|
||||||
|
|
||||||
|
export default {
|
||||||
|
name: 'InputOtp',
|
||||||
|
extends: BaseInputOtp,
|
||||||
|
inheritAttrs: false,
|
||||||
|
emits: ['update:modelValue', 'change', 'focus', 'blur'],
|
||||||
|
data() {
|
||||||
|
return {
|
||||||
|
tokens: []
|
||||||
|
};
|
||||||
|
},
|
||||||
|
watch: {
|
||||||
|
modelValue: {
|
||||||
|
immediate: true,
|
||||||
|
handler(newValue) {
|
||||||
|
this.tokens = newValue ? newValue.split('') : new Array(this.length);
|
||||||
|
}
|
||||||
|
}
|
||||||
|
},
|
||||||
|
methods: {
|
||||||
|
getPTOptions(key) {
|
||||||
|
const _ptm = key === 'root' ? this.ptmi : this.ptm;
|
||||||
|
|
||||||
|
return _ptm(key, {
|
||||||
|
context: {
|
||||||
|
checked: this.checked,
|
||||||
|
disabled: this.disabled
|
||||||
|
}
|
||||||
|
});
|
||||||
|
},
|
||||||
|
onInput(event) {
|
||||||
|
this.moveToNext(event);
|
||||||
|
this.updateModel(event);
|
||||||
|
},
|
||||||
|
updateModel(event) {
|
||||||
|
const newValue = this.tokens.join('');
|
||||||
|
|
||||||
|
this.$emit('update:modelValue', newValue);
|
||||||
|
this.$emit('change', {
|
||||||
|
originalEvent: event,
|
||||||
|
value: newValue
|
||||||
|
});
|
||||||
|
},
|
||||||
|
moveToPrev(event) {
|
||||||
|
var prevElement = event.target.previousElementSibling;
|
||||||
|
|
||||||
|
if (prevElement) {
|
||||||
|
prevElement.focus();
|
||||||
|
prevElement.select();
|
||||||
|
}
|
||||||
|
},
|
||||||
|
moveToNext(event) {
|
||||||
|
var nextElement = event.target.nextElementSibling;
|
||||||
|
|
||||||
|
if (nextElement) {
|
||||||
|
nextElement.focus();
|
||||||
|
nextElement.select();
|
||||||
|
}
|
||||||
|
},
|
||||||
|
onFocus(event) {
|
||||||
|
event.target.select();
|
||||||
|
this.$emit('focus', event);
|
||||||
|
},
|
||||||
|
onBlur(event) {
|
||||||
|
this.$emit('blur', event);
|
||||||
|
},
|
||||||
|
onKeyDown(event, index) {
|
||||||
|
const keyCode = event.keyCode;
|
||||||
|
|
||||||
|
switch (keyCode) {
|
||||||
|
case 37:
|
||||||
|
this.moveToPrev(event);
|
||||||
|
event.preventDefault();
|
||||||
|
|
||||||
|
break;
|
||||||
|
|
||||||
|
case 38:
|
||||||
|
case 40:
|
||||||
|
event.preventDefault();
|
||||||
|
|
||||||
|
break;
|
||||||
|
|
||||||
|
case 39:
|
||||||
|
this.moveToNext(event);
|
||||||
|
event.preventDefault();
|
||||||
|
|
||||||
|
break;
|
||||||
|
|
||||||
|
case 8:
|
||||||
|
event.preventDefault();
|
||||||
|
this.tokens[index] = '';
|
||||||
|
this.moveToPrev(event);
|
||||||
|
this.updateModel(event);
|
||||||
|
|
||||||
|
break;
|
||||||
|
|
||||||
|
default:
|
||||||
|
if (this.integerOnly && !(event.keyCode >= 48 && event.keyCode <= 57)) {
|
||||||
|
event.preventDefault();
|
||||||
|
}
|
||||||
|
|
||||||
|
break;
|
||||||
|
}
|
||||||
|
},
|
||||||
|
onPaste(event) {
|
||||||
|
let paste = event.clipboardData.getData('text');
|
||||||
|
|
||||||
|
if (paste.length) {
|
||||||
|
let pastedCode = paste.substring(0, this.length + 1);
|
||||||
|
|
||||||
|
if (!this.isIntegerOnly || !isNaN(pastedCode)) {
|
||||||
|
this.tokens = pastedCode.split('');
|
||||||
|
this.updateModel(event);
|
||||||
|
}
|
||||||
|
}
|
||||||
|
|
||||||
|
event.preventDefault();
|
||||||
|
}
|
||||||
|
},
|
||||||
|
computed: {
|
||||||
|
inputMode() {
|
||||||
|
return this.integerOnly ? 'number' : 'text';
|
||||||
|
},
|
||||||
|
inputType() {
|
||||||
|
return this.mask ? 'password' : 'text';
|
||||||
|
}
|
||||||
|
},
|
||||||
|
components: {
|
||||||
|
OtpInputText: InputText
|
||||||
|
}
|
||||||
|
};
|
||||||
|
</script>
|
|
@ -0,0 +1,9 @@
|
||||||
|
{
|
||||||
|
"main": "./inputotp.cjs.js",
|
||||||
|
"module": "./inputotp.esm.js",
|
||||||
|
"unpkg": "./inputotp.min.js",
|
||||||
|
"types": "./inputotp.d.ts",
|
||||||
|
"browser": {
|
||||||
|
"./sfc": "./inputotp.vue"
|
||||||
|
}
|
||||||
|
}
|
|
@ -0,0 +1,3 @@
|
||||||
|
import { BaseStyle } from '../../base/style/BaseStyle';
|
||||||
|
|
||||||
|
export interface InputOtpStyle extends BaseStyle {}
|
|
@ -0,0 +1,11 @@
|
||||||
|
import BaseStyle from 'primevue/base/style';
|
||||||
|
|
||||||
|
const classes = {
|
||||||
|
root: ({ props }) => ['p-inputotp p-component'],
|
||||||
|
input: 'p-inputotp-input'
|
||||||
|
};
|
||||||
|
|
||||||
|
export default BaseStyle.extend({
|
||||||
|
name: 'inputotp',
|
||||||
|
classes
|
||||||
|
});
|
|
@ -0,0 +1,6 @@
|
||||||
|
{
|
||||||
|
"main": "./inputotpstyle.cjs.js",
|
||||||
|
"module": "./inputotpstyle.esm.js",
|
||||||
|
"unpkg": "./inputotpstyle.min.js",
|
||||||
|
"types": "./inputotpstyle.d.ts"
|
||||||
|
}
|
|
@ -5418,7 +5418,7 @@
|
||||||
}
|
}
|
||||||
],
|
],
|
||||||
"methods": [],
|
"methods": [],
|
||||||
"extendedBy": "AccordionStyle,AccordionTabStyle,AnimateOnScrollStyle,AutoCompleteStyle,AvatarStyle,AvatarGroupStyle,BadgeStyle,BadgeDirectiveStyle,BaseComponentStyle,BaseIconStyle,BlockUIStyle,BreadcrumbStyle,ButtonStyle,ButtonGroupStyle,CalendarStyle,CardStyle,CarouselStyle,CascadeSelectStyle,ChartStyle,CheckboxStyle,ChipStyle,ChipsStyle,ColorPickerStyle,ColumnStyle,ColumnGroupStyle,ConfirmDialogStyle,ConfirmPopupStyle,ContextMenuStyle,DataTableStyle,DataViewStyle,DataViewLayoutOptionsStyle,DeferredContentStyle,DialogStyle,DividerStyle,DockStyle,DropdownStyle,DynamicDialogStyle,EditorStyle,FieldsetStyle,AccordionStyle,FloatLabelStyle,FocusTrapStyle,GalleriaStyle,IconFieldStyle,ImageStyle,InlineMessageStyle,InplaceStyle,InputGroupStyle,InputGroupAddonStyle,InputIconStyle,InputMaskStyle,InputNumberStyle,InputSwitchStyle,InputTextStyle,KnobStyle,ListboxStyle,MegaMenuStyle,MenuStyle,MenubarStyle,MessageStyle,MeterGroupStyle,MultiSelectStyle,OrderListStyle,OrganizationChartStyle,OverlayPanelStyle,PaginatorStyle,PanelStyle,PanelMenuStyle,PasswordStyle,PickListStyle,PortalStyle,ProgressBarStyle,ProgressSpinnerStyle,RadioButtonStyle,RatingStyle,AccordionStyle,RowStyle,ScrollPanelStyle,ScrollTopStyle,SelectButtonStyle,SidebarStyle,SkeletonStyle,SliderStyle,SpeedDialStyle,SplitButtonStyle,SplitterStyle,SplitterPanelStyle,StepperStyle,StepperPanelStyle,StepsStyle,StyleClassStyle,TabMenuStyle,TabPanelStyle,TabViewStyle,TagStyle,TerminalStyle,TextareaStyle,TieredMenuStyle,TimelineStyle,ToastStyle,ToggleButtonStyle,ToolbarStyle,TooltipStyle,TreeStyle,TreeSelectStyle,TreeTableStyle,TriStateCheckboxStyle,VirtualScrollerStyle"
|
"extendedBy": "AccordionStyle,AccordionTabStyle,AnimateOnScrollStyle,AutoCompleteStyle,AvatarStyle,AvatarGroupStyle,BadgeStyle,BadgeDirectiveStyle,BaseComponentStyle,BaseIconStyle,BlockUIStyle,BreadcrumbStyle,ButtonStyle,ButtonGroupStyle,CalendarStyle,CardStyle,CarouselStyle,CascadeSelectStyle,ChartStyle,CheckboxStyle,ChipStyle,ChipsStyle,ColorPickerStyle,ColumnStyle,ColumnGroupStyle,ConfirmDialogStyle,ConfirmPopupStyle,ContextMenuStyle,DataTableStyle,DataViewStyle,DataViewLayoutOptionsStyle,DeferredContentStyle,DialogStyle,DividerStyle,DockStyle,DropdownStyle,DynamicDialogStyle,EditorStyle,FieldsetStyle,AccordionStyle,FloatLabelStyle,FocusTrapStyle,GalleriaStyle,IconFieldStyle,ImageStyle,InlineMessageStyle,InplaceStyle,InputGroupStyle,InputGroupAddonStyle,InputIconStyle,InputMaskStyle,InputNumberStyle,InputOtpStyle,InputSwitchStyle,InputTextStyle,KnobStyle,ListboxStyle,MegaMenuStyle,MenuStyle,MenubarStyle,MessageStyle,MeterGroupStyle,MultiSelectStyle,OrderListStyle,OrganizationChartStyle,OverlayPanelStyle,PaginatorStyle,PanelStyle,PanelMenuStyle,PasswordStyle,PickListStyle,PortalStyle,ProgressBarStyle,ProgressSpinnerStyle,RadioButtonStyle,RatingStyle,AccordionStyle,RowStyle,ScrollPanelStyle,ScrollTopStyle,SelectButtonStyle,SidebarStyle,SkeletonStyle,SliderStyle,SpeedDialStyle,SplitButtonStyle,SplitterStyle,SplitterPanelStyle,StepperStyle,StepperPanelStyle,StepsStyle,StyleClassStyle,TabMenuStyle,TabPanelStyle,TabViewStyle,TagStyle,TerminalStyle,TextareaStyle,TieredMenuStyle,TimelineStyle,ToastStyle,ToggleButtonStyle,ToolbarStyle,TooltipStyle,TreeStyle,TreeSelectStyle,TreeTableStyle,TriStateCheckboxStyle,VirtualScrollerStyle"
|
||||||
}
|
}
|
||||||
}
|
}
|
||||||
}
|
}
|
||||||
|
@ -31974,6 +31974,435 @@
|
||||||
}
|
}
|
||||||
}
|
}
|
||||||
},
|
},
|
||||||
|
"inputotp": {
|
||||||
|
"description": "InputSwitch is used to select a boolean value.\n\n[Live Demo](https://www.primevue.org/inputswitch/)",
|
||||||
|
"components": {
|
||||||
|
"default": {
|
||||||
|
"description": "InputSwitch is used to select a boolean value.",
|
||||||
|
"methods": {
|
||||||
|
"description": "Defines methods that can be accessed by the component's reference.",
|
||||||
|
"values": []
|
||||||
|
}
|
||||||
|
}
|
||||||
|
},
|
||||||
|
"interfaces": {
|
||||||
|
"description": "Defines the custom interfaces used by the module.",
|
||||||
|
"eventDescription": "Defines the custom events used by the component's emit.",
|
||||||
|
"methodDescription": "Defines methods that can be accessed by the component's reference.",
|
||||||
|
"typeDescription": "Defines the custom types used by the module.",
|
||||||
|
"values": {
|
||||||
|
"InputSwitchPassThroughMethodOptions": {
|
||||||
|
"description": "Custom passthrough(pt) option method.",
|
||||||
|
"relatedProp": "",
|
||||||
|
"props": [
|
||||||
|
{
|
||||||
|
"name": "instance",
|
||||||
|
"optional": false,
|
||||||
|
"readonly": false,
|
||||||
|
"type": "any",
|
||||||
|
"default": "",
|
||||||
|
"description": "Defines instance."
|
||||||
|
},
|
||||||
|
{
|
||||||
|
"name": "props",
|
||||||
|
"optional": false,
|
||||||
|
"readonly": false,
|
||||||
|
"type": "InputSwitchProps",
|
||||||
|
"default": "",
|
||||||
|
"description": "Defines valid properties."
|
||||||
|
},
|
||||||
|
{
|
||||||
|
"name": "state",
|
||||||
|
"optional": false,
|
||||||
|
"readonly": false,
|
||||||
|
"type": "InputSwitchState",
|
||||||
|
"default": "",
|
||||||
|
"description": "Defines current inline state."
|
||||||
|
},
|
||||||
|
{
|
||||||
|
"name": "context",
|
||||||
|
"optional": false,
|
||||||
|
"readonly": false,
|
||||||
|
"type": "InputSwitchContext",
|
||||||
|
"default": "",
|
||||||
|
"description": "Defines current options."
|
||||||
|
},
|
||||||
|
{
|
||||||
|
"name": "attrs",
|
||||||
|
"optional": false,
|
||||||
|
"readonly": false,
|
||||||
|
"type": "any",
|
||||||
|
"default": "",
|
||||||
|
"description": "Defines valid attributes."
|
||||||
|
},
|
||||||
|
{
|
||||||
|
"name": "parent",
|
||||||
|
"optional": false,
|
||||||
|
"readonly": false,
|
||||||
|
"type": "any",
|
||||||
|
"default": "",
|
||||||
|
"description": "Defines parent options."
|
||||||
|
},
|
||||||
|
{
|
||||||
|
"name": "global",
|
||||||
|
"optional": false,
|
||||||
|
"readonly": false,
|
||||||
|
"type": "undefined | object",
|
||||||
|
"default": "",
|
||||||
|
"description": "Defines passthrough(pt) options in global config."
|
||||||
|
}
|
||||||
|
],
|
||||||
|
"methods": []
|
||||||
|
},
|
||||||
|
"InputSwitchPassThroughOptions": {
|
||||||
|
"description": "Custom passthrough(pt) options.",
|
||||||
|
"relatedProp": "InputSwitchProps.pt",
|
||||||
|
"props": [
|
||||||
|
{
|
||||||
|
"name": "root",
|
||||||
|
"optional": true,
|
||||||
|
"readonly": false,
|
||||||
|
"type": "InputSwitchPassThroughOptionType",
|
||||||
|
"default": "",
|
||||||
|
"description": "Used to pass attributes to the root's DOM element."
|
||||||
|
},
|
||||||
|
{
|
||||||
|
"name": "input",
|
||||||
|
"optional": true,
|
||||||
|
"readonly": false,
|
||||||
|
"type": "InputSwitchPassThroughOptionType",
|
||||||
|
"default": "",
|
||||||
|
"description": "Used to pass attributes to the input's DOM element."
|
||||||
|
},
|
||||||
|
{
|
||||||
|
"name": "slider",
|
||||||
|
"optional": true,
|
||||||
|
"readonly": false,
|
||||||
|
"type": "InputSwitchPassThroughOptionType",
|
||||||
|
"default": "",
|
||||||
|
"description": "Used to pass attributes to the slider's DOM element."
|
||||||
|
},
|
||||||
|
{
|
||||||
|
"name": "hooks",
|
||||||
|
"optional": true,
|
||||||
|
"readonly": false,
|
||||||
|
"type": "ComponentHooks",
|
||||||
|
"default": "",
|
||||||
|
"description": "Used to manage all lifecycle hooks."
|
||||||
|
}
|
||||||
|
],
|
||||||
|
"methods": []
|
||||||
|
},
|
||||||
|
"InputSwitchPassThroughAttributes": {
|
||||||
|
"description": "Custom passthrough attributes for each DOM elements",
|
||||||
|
"relatedProp": "",
|
||||||
|
"props": [
|
||||||
|
{
|
||||||
|
"name": "[key: string]",
|
||||||
|
"optional": false,
|
||||||
|
"readonly": false,
|
||||||
|
"type": "any"
|
||||||
|
}
|
||||||
|
],
|
||||||
|
"methods": []
|
||||||
|
},
|
||||||
|
"InputSwitchState": {
|
||||||
|
"description": "Defines current inline state in InputSwitch component.",
|
||||||
|
"relatedProp": "",
|
||||||
|
"props": [
|
||||||
|
{
|
||||||
|
"name": "[key: string]",
|
||||||
|
"optional": false,
|
||||||
|
"readonly": false,
|
||||||
|
"type": "any"
|
||||||
|
}
|
||||||
|
],
|
||||||
|
"methods": []
|
||||||
|
},
|
||||||
|
"InputSwitchProps": {
|
||||||
|
"description": "Defines valid properties in InputSwitch component.",
|
||||||
|
"relatedProp": "",
|
||||||
|
"props": [
|
||||||
|
{
|
||||||
|
"name": "modelValue",
|
||||||
|
"optional": true,
|
||||||
|
"readonly": false,
|
||||||
|
"type": "string | boolean",
|
||||||
|
"default": "false",
|
||||||
|
"description": "Specifies whether a inputswitch should be checked or not."
|
||||||
|
},
|
||||||
|
{
|
||||||
|
"name": "trueValue",
|
||||||
|
"optional": true,
|
||||||
|
"readonly": false,
|
||||||
|
"type": "any",
|
||||||
|
"default": "true",
|
||||||
|
"description": "Value in checked state."
|
||||||
|
},
|
||||||
|
{
|
||||||
|
"name": "falseValue",
|
||||||
|
"optional": true,
|
||||||
|
"readonly": false,
|
||||||
|
"type": "any",
|
||||||
|
"default": "false",
|
||||||
|
"description": "Value in unchecked state."
|
||||||
|
},
|
||||||
|
{
|
||||||
|
"name": "invalid",
|
||||||
|
"optional": true,
|
||||||
|
"readonly": false,
|
||||||
|
"type": "boolean",
|
||||||
|
"default": "false",
|
||||||
|
"description": "When present, it specifies that the component should have invalid state style."
|
||||||
|
},
|
||||||
|
{
|
||||||
|
"name": "disabled",
|
||||||
|
"optional": true,
|
||||||
|
"readonly": false,
|
||||||
|
"type": "boolean",
|
||||||
|
"default": "false",
|
||||||
|
"description": "When present, it specifies that the component should be disabled."
|
||||||
|
},
|
||||||
|
{
|
||||||
|
"name": "readonly",
|
||||||
|
"optional": true,
|
||||||
|
"readonly": false,
|
||||||
|
"type": "boolean",
|
||||||
|
"default": "",
|
||||||
|
"description": "When present, it specifies that an input field is read-only."
|
||||||
|
},
|
||||||
|
{
|
||||||
|
"name": "tabindex",
|
||||||
|
"optional": true,
|
||||||
|
"readonly": false,
|
||||||
|
"type": "number",
|
||||||
|
"default": "",
|
||||||
|
"description": "Index of the element in tabbing order."
|
||||||
|
},
|
||||||
|
{
|
||||||
|
"name": "inputId",
|
||||||
|
"optional": true,
|
||||||
|
"readonly": false,
|
||||||
|
"type": "string",
|
||||||
|
"default": "",
|
||||||
|
"description": "Identifier of the underlying input element."
|
||||||
|
},
|
||||||
|
{
|
||||||
|
"name": "inputClass",
|
||||||
|
"optional": true,
|
||||||
|
"readonly": false,
|
||||||
|
"type": "string | object",
|
||||||
|
"default": "",
|
||||||
|
"description": "Style class of the input field."
|
||||||
|
},
|
||||||
|
{
|
||||||
|
"name": "inputStyle",
|
||||||
|
"optional": true,
|
||||||
|
"readonly": false,
|
||||||
|
"type": "object",
|
||||||
|
"default": "",
|
||||||
|
"description": "Inline style of the input field."
|
||||||
|
},
|
||||||
|
{
|
||||||
|
"name": "ariaLabelledby",
|
||||||
|
"optional": true,
|
||||||
|
"readonly": false,
|
||||||
|
"type": "string",
|
||||||
|
"default": "",
|
||||||
|
"description": "Establishes relationships between the component and label(s) where its value should be one or more element IDs."
|
||||||
|
},
|
||||||
|
{
|
||||||
|
"name": "ariaLabel",
|
||||||
|
"optional": true,
|
||||||
|
"readonly": false,
|
||||||
|
"type": "string",
|
||||||
|
"default": "",
|
||||||
|
"description": "Establishes a string value that labels the component."
|
||||||
|
},
|
||||||
|
{
|
||||||
|
"name": "pt",
|
||||||
|
"optional": true,
|
||||||
|
"readonly": false,
|
||||||
|
"type": "PassThrough<InputSwitchPassThroughOptions>",
|
||||||
|
"default": "",
|
||||||
|
"description": "Used to pass attributes to DOM elements inside the component."
|
||||||
|
},
|
||||||
|
{
|
||||||
|
"name": "ptOptions",
|
||||||
|
"optional": true,
|
||||||
|
"readonly": false,
|
||||||
|
"type": "PassThroughOptions",
|
||||||
|
"default": "",
|
||||||
|
"description": "Used to configure passthrough(pt) options of the component."
|
||||||
|
},
|
||||||
|
{
|
||||||
|
"name": "unstyled",
|
||||||
|
"optional": true,
|
||||||
|
"readonly": false,
|
||||||
|
"type": "boolean",
|
||||||
|
"default": "false",
|
||||||
|
"description": "When enabled, it removes component related styles in the core."
|
||||||
|
}
|
||||||
|
],
|
||||||
|
"methods": []
|
||||||
|
},
|
||||||
|
"InputSwitchContext": {
|
||||||
|
"description": "Defines current options in InputSwitch component.",
|
||||||
|
"relatedProp": "",
|
||||||
|
"props": [
|
||||||
|
{
|
||||||
|
"name": "checked",
|
||||||
|
"optional": false,
|
||||||
|
"readonly": false,
|
||||||
|
"type": "boolean",
|
||||||
|
"default": "false",
|
||||||
|
"description": "Current checked state of the item as a boolean."
|
||||||
|
},
|
||||||
|
{
|
||||||
|
"name": "disabled",
|
||||||
|
"optional": false,
|
||||||
|
"readonly": false,
|
||||||
|
"type": "boolean",
|
||||||
|
"default": "false",
|
||||||
|
"description": "Current disabled state of the item as a boolean."
|
||||||
|
}
|
||||||
|
],
|
||||||
|
"methods": []
|
||||||
|
},
|
||||||
|
"InputSwitchSlots": {
|
||||||
|
"relatedProp": "",
|
||||||
|
"props": [],
|
||||||
|
"methods": []
|
||||||
|
},
|
||||||
|
"InputSwitchEmits": {
|
||||||
|
"description": "Defines valid emits in InputSwitch component.",
|
||||||
|
"relatedProp": "",
|
||||||
|
"props": [],
|
||||||
|
"methods": [
|
||||||
|
{
|
||||||
|
"name": "update:modelValue",
|
||||||
|
"parameters": [
|
||||||
|
{
|
||||||
|
"name": "value",
|
||||||
|
"optional": false,
|
||||||
|
"type": "boolean",
|
||||||
|
"description": "New value."
|
||||||
|
}
|
||||||
|
],
|
||||||
|
"returnType": "void",
|
||||||
|
"description": "Emitted when the value changes."
|
||||||
|
},
|
||||||
|
{
|
||||||
|
"name": "change",
|
||||||
|
"parameters": [
|
||||||
|
{
|
||||||
|
"name": "event",
|
||||||
|
"optional": false,
|
||||||
|
"type": "Event",
|
||||||
|
"description": "Browser event."
|
||||||
|
}
|
||||||
|
],
|
||||||
|
"returnType": "void",
|
||||||
|
"description": "Callback to invoke on value change."
|
||||||
|
},
|
||||||
|
{
|
||||||
|
"name": "focus",
|
||||||
|
"parameters": [
|
||||||
|
{
|
||||||
|
"name": "event",
|
||||||
|
"optional": false,
|
||||||
|
"type": "Event",
|
||||||
|
"description": "Browser event."
|
||||||
|
}
|
||||||
|
],
|
||||||
|
"returnType": "void",
|
||||||
|
"description": "Callback to invoke when the component receives focus."
|
||||||
|
},
|
||||||
|
{
|
||||||
|
"name": "blur",
|
||||||
|
"parameters": [
|
||||||
|
{
|
||||||
|
"name": "event",
|
||||||
|
"optional": false,
|
||||||
|
"type": "Event",
|
||||||
|
"description": "Browser event."
|
||||||
|
}
|
||||||
|
],
|
||||||
|
"returnType": "void",
|
||||||
|
"description": "Callback to invoke when the component loses focus."
|
||||||
|
}
|
||||||
|
]
|
||||||
|
}
|
||||||
|
}
|
||||||
|
},
|
||||||
|
"types": {
|
||||||
|
"description": "Defines the custom types used by the module.",
|
||||||
|
"values": {
|
||||||
|
"InputSwitchPassThroughOptionType": {
|
||||||
|
"values": "InputSwitchPassThroughAttributes | (options: InputSwitchPassThroughMethodOptions) => undefined | string | null | undefined"
|
||||||
|
}
|
||||||
|
}
|
||||||
|
}
|
||||||
|
},
|
||||||
|
"inputotp/style/InputOtpStyle": {
|
||||||
|
"interfaces": {
|
||||||
|
"description": "Defines the custom interfaces used by the module.",
|
||||||
|
"eventDescription": "Defines the custom events used by the component's emit.",
|
||||||
|
"methodDescription": "Defines methods that can be accessed by the component's reference.",
|
||||||
|
"typeDescription": "Defines the custom types used by the module.",
|
||||||
|
"values": {
|
||||||
|
"InputOtpStyle": {
|
||||||
|
"relatedProp": "",
|
||||||
|
"props": [
|
||||||
|
{
|
||||||
|
"name": "name",
|
||||||
|
"optional": true,
|
||||||
|
"readonly": false,
|
||||||
|
"type": "string",
|
||||||
|
"default": ""
|
||||||
|
},
|
||||||
|
{
|
||||||
|
"name": "css",
|
||||||
|
"optional": true,
|
||||||
|
"readonly": false,
|
||||||
|
"type": "string",
|
||||||
|
"default": ""
|
||||||
|
},
|
||||||
|
{
|
||||||
|
"name": "classes",
|
||||||
|
"optional": true,
|
||||||
|
"readonly": false,
|
||||||
|
"type": "object",
|
||||||
|
"default": ""
|
||||||
|
},
|
||||||
|
{
|
||||||
|
"name": "inlineStyles",
|
||||||
|
"optional": true,
|
||||||
|
"readonly": false,
|
||||||
|
"type": "object",
|
||||||
|
"default": ""
|
||||||
|
},
|
||||||
|
{
|
||||||
|
"name": "loadStyle",
|
||||||
|
"optional": true,
|
||||||
|
"readonly": false,
|
||||||
|
"type": "Function",
|
||||||
|
"default": ""
|
||||||
|
},
|
||||||
|
{
|
||||||
|
"name": "getStyleSheet",
|
||||||
|
"optional": true,
|
||||||
|
"readonly": false,
|
||||||
|
"type": "Function",
|
||||||
|
"default": ""
|
||||||
|
}
|
||||||
|
],
|
||||||
|
"methods": [],
|
||||||
|
"extendedTypes": "BaseStyle"
|
||||||
|
}
|
||||||
|
}
|
||||||
|
}
|
||||||
|
},
|
||||||
"inputswitch": {
|
"inputswitch": {
|
||||||
"description": "InputSwitch is used to select a boolean value.\n\n[Live Demo](https://www.primevue.org/inputswitch/)",
|
"description": "InputSwitch is used to select a boolean value.\n\n[Live Demo](https://www.primevue.org/inputswitch/)",
|
||||||
"components": {
|
"components": {
|
||||||
|
|
|
@ -0,0 +1,32 @@
|
||||||
|
<template>
|
||||||
|
<DocSectionText id="accessibility" label="Accessibility" v-bind="$attrs">
|
||||||
|
<h3>Screen Reader</h3>
|
||||||
|
<p>Input OTP uses a set of InputText components, refer to the <NuxtLink to="/inputtext">InputText</NuxtLink> component for more information about the screen reader support.</p>
|
||||||
|
|
||||||
|
<h3>Keyboard Support</h3>
|
||||||
|
<div class="doc-tablewrapper">
|
||||||
|
<table class="doc-table">
|
||||||
|
<thead>
|
||||||
|
<tr>
|
||||||
|
<th>Key</th>
|
||||||
|
<th>Function</th>
|
||||||
|
</tr>
|
||||||
|
</thead>
|
||||||
|
<tbody>
|
||||||
|
<tr>
|
||||||
|
<td><i>tab</i></td>
|
||||||
|
<td>Moves focus to the input otp.</td>
|
||||||
|
</tr>
|
||||||
|
<tr>
|
||||||
|
<td><i>right arrow</i></td>
|
||||||
|
<td>Moves focus to the next input element.</td>
|
||||||
|
</tr>
|
||||||
|
<tr>
|
||||||
|
<td><i>left arrow</i></td>
|
||||||
|
<td>Moves focus to the next previous element.</td>
|
||||||
|
</tr>
|
||||||
|
</tbody>
|
||||||
|
</table>
|
||||||
|
</div>
|
||||||
|
</DocSectionText>
|
||||||
|
</template>
|
|
@ -0,0 +1,54 @@
|
||||||
|
<template>
|
||||||
|
<DocSectionText v-bind="$attrs">
|
||||||
|
<p>Two-way value binding is defined using <i>v-model</i>. The number of characters is defined with the <i>length</i> property, which is set to 4 by default.</p>
|
||||||
|
</DocSectionText>
|
||||||
|
<div class="card flex justify-content-center">
|
||||||
|
<InputOtp v-model="value" />
|
||||||
|
</div>
|
||||||
|
<DocSectionCode :code="code" />
|
||||||
|
</template>
|
||||||
|
|
||||||
|
<script>
|
||||||
|
export default {
|
||||||
|
data() {
|
||||||
|
return {
|
||||||
|
value: null,
|
||||||
|
code: {
|
||||||
|
basic: `
|
||||||
|
<InputOtp v-model="value" />
|
||||||
|
`,
|
||||||
|
options: `
|
||||||
|
<template>
|
||||||
|
<div class="card flex justify-content-center">
|
||||||
|
<InputOtp v-model="value" />
|
||||||
|
</div>
|
||||||
|
</template>
|
||||||
|
|
||||||
|
<script>
|
||||||
|
export default {
|
||||||
|
data() {
|
||||||
|
return {
|
||||||
|
value: null
|
||||||
|
}
|
||||||
|
}
|
||||||
|
};
|
||||||
|
<\/script>
|
||||||
|
`,
|
||||||
|
composition: `
|
||||||
|
<template>
|
||||||
|
<div class="card flex justify-content-center">
|
||||||
|
<InputOtp v-model="value" />
|
||||||
|
</div>
|
||||||
|
</template>
|
||||||
|
|
||||||
|
<script setup>
|
||||||
|
import { ref } from 'vue';
|
||||||
|
|
||||||
|
const value = ref(null);
|
||||||
|
<\/script>
|
||||||
|
`
|
||||||
|
}
|
||||||
|
};
|
||||||
|
}
|
||||||
|
};
|
||||||
|
</script>
|
|
@ -0,0 +1,18 @@
|
||||||
|
<template>
|
||||||
|
<DocSectionText v-bind="$attrs" />
|
||||||
|
<DocSectionCode :code="code" hideToggleCode importCode hideStackBlitz />
|
||||||
|
</template>
|
||||||
|
|
||||||
|
<script>
|
||||||
|
export default {
|
||||||
|
data() {
|
||||||
|
return {
|
||||||
|
code: {
|
||||||
|
basic: `
|
||||||
|
import InputOtp from 'primevue/inputotp';
|
||||||
|
`
|
||||||
|
}
|
||||||
|
};
|
||||||
|
}
|
||||||
|
};
|
||||||
|
</script>
|
|
@ -0,0 +1,54 @@
|
||||||
|
<template>
|
||||||
|
<DocSectionText v-bind="$attrs">
|
||||||
|
<p>When <i>integerOnly</i> is present, only integers can be accepted as input.</p>
|
||||||
|
</DocSectionText>
|
||||||
|
<div class="card flex justify-content-center">
|
||||||
|
<InputOtp v-model="value" integerOnly />
|
||||||
|
</div>
|
||||||
|
<DocSectionCode :code="code" />
|
||||||
|
</template>
|
||||||
|
|
||||||
|
<script>
|
||||||
|
export default {
|
||||||
|
data() {
|
||||||
|
return {
|
||||||
|
value: null,
|
||||||
|
code: {
|
||||||
|
basic: `
|
||||||
|
<InputOtp v-model="value" integerOnly />
|
||||||
|
`,
|
||||||
|
options: `
|
||||||
|
<template>
|
||||||
|
<div class="card flex justify-content-center">
|
||||||
|
<InputOtp v-model="value" integerOnly />
|
||||||
|
</div>
|
||||||
|
</template>
|
||||||
|
|
||||||
|
<script>
|
||||||
|
export default {
|
||||||
|
data() {
|
||||||
|
return {
|
||||||
|
value: null
|
||||||
|
}
|
||||||
|
}
|
||||||
|
};
|
||||||
|
<\/script>
|
||||||
|
`,
|
||||||
|
composition: `
|
||||||
|
<template>
|
||||||
|
<div class="card flex justify-content-center">
|
||||||
|
<InputOtp v-model="value" integerOnly />
|
||||||
|
</div>
|
||||||
|
</template>
|
||||||
|
|
||||||
|
<script setup>
|
||||||
|
import { ref } from 'vue';
|
||||||
|
|
||||||
|
const value = ref(null);
|
||||||
|
<\/script>
|
||||||
|
`
|
||||||
|
}
|
||||||
|
};
|
||||||
|
}
|
||||||
|
};
|
||||||
|
</script>
|
|
@ -0,0 +1,54 @@
|
||||||
|
<template>
|
||||||
|
<DocSectionText v-bind="$attrs">
|
||||||
|
<p>Enable the <i>mask</i> option to hide the values in the input fields.</p>
|
||||||
|
</DocSectionText>
|
||||||
|
<div class="card flex justify-content-center">
|
||||||
|
<InputOtp v-model="value" mask />
|
||||||
|
</div>
|
||||||
|
<DocSectionCode :code="code" />
|
||||||
|
</template>
|
||||||
|
|
||||||
|
<script>
|
||||||
|
export default {
|
||||||
|
data() {
|
||||||
|
return {
|
||||||
|
value: null,
|
||||||
|
code: {
|
||||||
|
basic: `
|
||||||
|
<InputOtp v-model="value" mask />
|
||||||
|
`,
|
||||||
|
options: `
|
||||||
|
<template>
|
||||||
|
<div class="card flex justify-content-center">
|
||||||
|
<InputOtp v-model="value" mask />
|
||||||
|
</div>
|
||||||
|
</template>
|
||||||
|
|
||||||
|
<script>
|
||||||
|
export default {
|
||||||
|
data() {
|
||||||
|
return {
|
||||||
|
value: null
|
||||||
|
}
|
||||||
|
}
|
||||||
|
};
|
||||||
|
<\/script>
|
||||||
|
`,
|
||||||
|
composition: `
|
||||||
|
<template>
|
||||||
|
<div class="card flex justify-content-center">
|
||||||
|
<InputOtp v-model="value" mask />
|
||||||
|
</div>
|
||||||
|
</template>
|
||||||
|
|
||||||
|
<script setup>
|
||||||
|
import { ref } from 'vue';
|
||||||
|
|
||||||
|
const value = ref(null);
|
||||||
|
<\/script>
|
||||||
|
`
|
||||||
|
}
|
||||||
|
};
|
||||||
|
}
|
||||||
|
};
|
||||||
|
</script>
|
|
@ -0,0 +1,8 @@
|
||||||
|
<template>
|
||||||
|
<DocSectionText v-bind="$attrs">
|
||||||
|
<p>{{ $attrs.description }}</p>
|
||||||
|
</DocSectionText>
|
||||||
|
<div class="card">
|
||||||
|
<img class="w-full" src="https://primefaces.org/cdn/primevue/images/pt/inputswitch.jpg" />
|
||||||
|
</div>
|
||||||
|
</template>
|
|
@ -0,0 +1,35 @@
|
||||||
|
<template>
|
||||||
|
<div class="doc-main">
|
||||||
|
<div class="doc-intro">
|
||||||
|
<h1>InputSwitch Pass Through</h1>
|
||||||
|
</div>
|
||||||
|
<DocSections :docs="docs" />
|
||||||
|
</div>
|
||||||
|
<DocSectionNav :docs="docs" />
|
||||||
|
</template>
|
||||||
|
|
||||||
|
<script>
|
||||||
|
import DocApiTable from '@/components/doc/DocApiTable.vue';
|
||||||
|
import { getPTOption } from '@/components/doc/helpers/PTHelper.js';
|
||||||
|
import PTImage from './PTImage.vue';
|
||||||
|
|
||||||
|
export default {
|
||||||
|
data() {
|
||||||
|
return {
|
||||||
|
docs: [
|
||||||
|
{
|
||||||
|
id: 'pt.image',
|
||||||
|
label: 'Wireframe',
|
||||||
|
component: PTImage
|
||||||
|
},
|
||||||
|
{
|
||||||
|
id: 'pt.doc.inputswitch',
|
||||||
|
label: 'InputSwitch PT Options',
|
||||||
|
component: DocApiTable,
|
||||||
|
data: getPTOption('InputSwitch')
|
||||||
|
}
|
||||||
|
]
|
||||||
|
};
|
||||||
|
}
|
||||||
|
};
|
||||||
|
</script>
|
|
@ -0,0 +1,29 @@
|
||||||
|
<template>
|
||||||
|
<DocSectionText v-bind="$attrs">
|
||||||
|
<p>List of class names used in the styled mode.</p>
|
||||||
|
</DocSectionText>
|
||||||
|
<div class="doc-tablewrapper">
|
||||||
|
<table class="doc-table">
|
||||||
|
<thead>
|
||||||
|
<tr>
|
||||||
|
<th>Name</th>
|
||||||
|
<th>Element</th>
|
||||||
|
</tr>
|
||||||
|
</thead>
|
||||||
|
<tbody>
|
||||||
|
<tr>
|
||||||
|
<td>p-inputswitch</td>
|
||||||
|
<td>Container element.</td>
|
||||||
|
</tr>
|
||||||
|
<tr>
|
||||||
|
<td>p-inputswitch-checked</td>
|
||||||
|
<td>Container element in active state.</td>
|
||||||
|
</tr>
|
||||||
|
<tr>
|
||||||
|
<td>p-inputswitch-slider</td>
|
||||||
|
<td>Slider element behind the handle.</td>
|
||||||
|
</tr>
|
||||||
|
</tbody>
|
||||||
|
</table>
|
||||||
|
</div>
|
||||||
|
</template>
|
|
@ -0,0 +1,6 @@
|
||||||
|
<template>
|
||||||
|
<DocSectionText v-bind="$attrs">
|
||||||
|
Visit <a href="https://github.com/primefaces/primevue-tailwind" target="_blank" rel="noopener noreferrer">Tailwind Presets</a> project for detailed documentation, examples and ready-to-use presets about how to style PrimeVue components with
|
||||||
|
Tailwind CSS.
|
||||||
|
</DocSectionText>
|
||||||
|
</template>
|
|
@ -0,0 +1,40 @@
|
||||||
|
<template>
|
||||||
|
<div class="doc-main">
|
||||||
|
<div class="doc-intro">
|
||||||
|
<h1>InputSwitch Theming</h1>
|
||||||
|
</div>
|
||||||
|
<DocSections :docs="docs" />
|
||||||
|
</div>
|
||||||
|
<DocSectionNav :docs="docs" />
|
||||||
|
</template>
|
||||||
|
|
||||||
|
<script>
|
||||||
|
import StyledDoc from './StyledDoc.vue';
|
||||||
|
import TailwindDoc from './TailwindDoc.vue';
|
||||||
|
|
||||||
|
export default {
|
||||||
|
data() {
|
||||||
|
return {
|
||||||
|
docs: [
|
||||||
|
{
|
||||||
|
id: 'theming.styled',
|
||||||
|
label: 'Styled',
|
||||||
|
component: StyledDoc
|
||||||
|
},
|
||||||
|
{
|
||||||
|
id: 'theming.unstyled',
|
||||||
|
label: 'Unstyled',
|
||||||
|
description: 'Theming is implemented with the pass through properties in unstyled mode.',
|
||||||
|
children: [
|
||||||
|
{
|
||||||
|
id: 'theming.tailwind',
|
||||||
|
label: 'Tailwind',
|
||||||
|
component: TailwindDoc
|
||||||
|
}
|
||||||
|
]
|
||||||
|
}
|
||||||
|
]
|
||||||
|
};
|
||||||
|
}
|
||||||
|
};
|
||||||
|
</script>
|
|
@ -14,6 +14,7 @@ const form = [
|
||||||
'InputIcon',
|
'InputIcon',
|
||||||
'InputMask',
|
'InputMask',
|
||||||
'InputNumber',
|
'InputNumber',
|
||||||
|
'InputOtp',
|
||||||
'InputSwitch',
|
'InputSwitch',
|
||||||
'InputText',
|
'InputText',
|
||||||
'Knob',
|
'Knob',
|
||||||
|
|
|
@ -56,6 +56,7 @@ const STYLE_ALIAS = {
|
||||||
'primevue/inputicon/style': path.resolve(__dirname, './components/lib/inputicon/style/InputIconStyle.js'),
|
'primevue/inputicon/style': path.resolve(__dirname, './components/lib/inputicon/style/InputIconStyle.js'),
|
||||||
'primevue/inputmask/style': path.resolve(__dirname, './components/lib/inputmask/style/InputMaskStyle.js'),
|
'primevue/inputmask/style': path.resolve(__dirname, './components/lib/inputmask/style/InputMaskStyle.js'),
|
||||||
'primevue/inputnumber/style': path.resolve(__dirname, './components/lib/inputnumber/style/InputNumberStyle.js'),
|
'primevue/inputnumber/style': path.resolve(__dirname, './components/lib/inputnumber/style/InputNumberStyle.js'),
|
||||||
|
'primevue/inputotp/style': path.resolve(__dirname, './components/lib/inputotp/style/InputOtpStyle.js'),
|
||||||
'primevue/inputswitch/style': path.resolve(__dirname, './components/lib/inputswitch/style/InputSwitchStyle.js'),
|
'primevue/inputswitch/style': path.resolve(__dirname, './components/lib/inputswitch/style/InputSwitchStyle.js'),
|
||||||
'primevue/inputtext/style': path.resolve(__dirname, './components/lib/inputtext/style/InputTextStyle.js'),
|
'primevue/inputtext/style': path.resolve(__dirname, './components/lib/inputtext/style/InputTextStyle.js'),
|
||||||
'primevue/knob/style': path.resolve(__dirname, './components/lib/knob/style/KnobStyle.js'),
|
'primevue/knob/style': path.resolve(__dirname, './components/lib/knob/style/KnobStyle.js'),
|
||||||
|
|
|
@ -0,0 +1,49 @@
|
||||||
|
<template>
|
||||||
|
<DocComponent title="Vue Otp Input Component" header="InputOtp" description="Input Otp is used to enter one time passwords." :componentDocs="docs" :apiDocs="['InputOtp']" :ptTabComponent="ptComponent" :themingDocs="themingDoc" />
|
||||||
|
</template>
|
||||||
|
|
||||||
|
<script>
|
||||||
|
import AccessibilityDoc from '@/doc/inputotp/AccessibilityDoc.vue';
|
||||||
|
import BasicDoc from '@/doc/inputotp/BasicDoc.vue';
|
||||||
|
import ImportDoc from '@/doc/inputotp/ImportDoc.vue';
|
||||||
|
import MaskDoc from '@/doc/inputotp/MaskDoc.vue';
|
||||||
|
import IntegerOnlyDoc from '@/doc/inputotp/integerOnlyDoc.vue';
|
||||||
|
import PTComponent from '@/doc/inputotp/pt/index.vue';
|
||||||
|
import ThemingDoc from '@/doc/inputotp/theming/index.vue';
|
||||||
|
|
||||||
|
export default {
|
||||||
|
data() {
|
||||||
|
return {
|
||||||
|
docs: [
|
||||||
|
{
|
||||||
|
id: 'import',
|
||||||
|
label: 'Import',
|
||||||
|
component: ImportDoc
|
||||||
|
},
|
||||||
|
{
|
||||||
|
id: 'basic',
|
||||||
|
label: 'Basic',
|
||||||
|
component: BasicDoc
|
||||||
|
},
|
||||||
|
{
|
||||||
|
id: 'mask',
|
||||||
|
label: 'Mask',
|
||||||
|
component: MaskDoc
|
||||||
|
},
|
||||||
|
{
|
||||||
|
id: 'integeronly',
|
||||||
|
label: 'Integer Only',
|
||||||
|
component: IntegerOnlyDoc
|
||||||
|
},
|
||||||
|
{
|
||||||
|
id: 'accessibility',
|
||||||
|
label: 'Accessibility',
|
||||||
|
component: AccessibilityDoc
|
||||||
|
}
|
||||||
|
],
|
||||||
|
ptComponent: PTComponent,
|
||||||
|
themingDoc: ThemingDoc
|
||||||
|
};
|
||||||
|
}
|
||||||
|
};
|
||||||
|
</script>
|
|
@ -1713,6 +1713,17 @@
|
||||||
margin-top: -0.5rem;
|
margin-top: -0.5rem;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
.p-inputotp {
|
||||||
|
display: flex;
|
||||||
|
align-items: center;
|
||||||
|
gap: 0.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
|
.p-inputotp-input {
|
||||||
|
text-align: center;
|
||||||
|
width: 2rem;
|
||||||
|
}
|
||||||
|
|
||||||
.p-inputgroup {
|
.p-inputgroup {
|
||||||
display: flex;
|
display: flex;
|
||||||
align-items: stretch;
|
align-items: stretch;
|
||||||
|
|
|
@ -1713,6 +1713,17 @@
|
||||||
margin-top: -0.5rem;
|
margin-top: -0.5rem;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
.p-inputotp {
|
||||||
|
display: flex;
|
||||||
|
align-items: center;
|
||||||
|
gap: 0.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
|
.p-inputotp-input {
|
||||||
|
text-align: center;
|
||||||
|
width: 2rem;
|
||||||
|
}
|
||||||
|
|
||||||
.p-inputgroup {
|
.p-inputgroup {
|
||||||
display: flex;
|
display: flex;
|
||||||
align-items: stretch;
|
align-items: stretch;
|
||||||
|
|
|
@ -1713,6 +1713,17 @@
|
||||||
margin-top: -0.5rem;
|
margin-top: -0.5rem;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
.p-inputotp {
|
||||||
|
display: flex;
|
||||||
|
align-items: center;
|
||||||
|
gap: 0.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
|
.p-inputotp-input {
|
||||||
|
text-align: center;
|
||||||
|
width: 2rem;
|
||||||
|
}
|
||||||
|
|
||||||
.p-inputgroup {
|
.p-inputgroup {
|
||||||
display: flex;
|
display: flex;
|
||||||
align-items: stretch;
|
align-items: stretch;
|
||||||
|
|
|
@ -1713,6 +1713,17 @@
|
||||||
margin-top: -0.5rem;
|
margin-top: -0.5rem;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
.p-inputotp {
|
||||||
|
display: flex;
|
||||||
|
align-items: center;
|
||||||
|
gap: 0.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
|
.p-inputotp-input {
|
||||||
|
text-align: center;
|
||||||
|
width: 2rem;
|
||||||
|
}
|
||||||
|
|
||||||
.p-inputgroup {
|
.p-inputgroup {
|
||||||
display: flex;
|
display: flex;
|
||||||
align-items: stretch;
|
align-items: stretch;
|
||||||
|
|
|
@ -1732,6 +1732,17 @@
|
||||||
margin-top: -0.5rem;
|
margin-top: -0.5rem;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
.p-inputotp {
|
||||||
|
display: flex;
|
||||||
|
align-items: center;
|
||||||
|
gap: 0.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
|
.p-inputotp-input {
|
||||||
|
text-align: center;
|
||||||
|
width: 2.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
.p-inputgroup {
|
.p-inputgroup {
|
||||||
display: flex;
|
display: flex;
|
||||||
align-items: stretch;
|
align-items: stretch;
|
||||||
|
|
|
@ -1732,6 +1732,17 @@
|
||||||
margin-top: -0.5rem;
|
margin-top: -0.5rem;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
.p-inputotp {
|
||||||
|
display: flex;
|
||||||
|
align-items: center;
|
||||||
|
gap: 0.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
|
.p-inputotp-input {
|
||||||
|
text-align: center;
|
||||||
|
width: 2.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
.p-inputgroup {
|
.p-inputgroup {
|
||||||
display: flex;
|
display: flex;
|
||||||
align-items: stretch;
|
align-items: stretch;
|
||||||
|
|
|
@ -1732,6 +1732,17 @@
|
||||||
margin-top: -0.5rem;
|
margin-top: -0.5rem;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
.p-inputotp {
|
||||||
|
display: flex;
|
||||||
|
align-items: center;
|
||||||
|
gap: 0.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
|
.p-inputotp-input {
|
||||||
|
text-align: center;
|
||||||
|
width: 2.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
.p-inputgroup {
|
.p-inputgroup {
|
||||||
display: flex;
|
display: flex;
|
||||||
align-items: stretch;
|
align-items: stretch;
|
||||||
|
|
|
@ -1732,6 +1732,17 @@
|
||||||
margin-top: -0.5rem;
|
margin-top: -0.5rem;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
.p-inputotp {
|
||||||
|
display: flex;
|
||||||
|
align-items: center;
|
||||||
|
gap: 0.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
|
.p-inputotp-input {
|
||||||
|
text-align: center;
|
||||||
|
width: 2.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
.p-inputgroup {
|
.p-inputgroup {
|
||||||
display: flex;
|
display: flex;
|
||||||
align-items: stretch;
|
align-items: stretch;
|
||||||
|
|
|
@ -1732,6 +1732,17 @@
|
||||||
margin-top: -0.5rem;
|
margin-top: -0.5rem;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
.p-inputotp {
|
||||||
|
display: flex;
|
||||||
|
align-items: center;
|
||||||
|
gap: 0.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
|
.p-inputotp-input {
|
||||||
|
text-align: center;
|
||||||
|
width: 2.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
.p-inputgroup {
|
.p-inputgroup {
|
||||||
display: flex;
|
display: flex;
|
||||||
align-items: stretch;
|
align-items: stretch;
|
||||||
|
|
|
@ -1732,6 +1732,17 @@
|
||||||
margin-top: -0.5rem;
|
margin-top: -0.5rem;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
.p-inputotp {
|
||||||
|
display: flex;
|
||||||
|
align-items: center;
|
||||||
|
gap: 0.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
|
.p-inputotp-input {
|
||||||
|
text-align: center;
|
||||||
|
width: 2.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
.p-inputgroup {
|
.p-inputgroup {
|
||||||
display: flex;
|
display: flex;
|
||||||
align-items: stretch;
|
align-items: stretch;
|
||||||
|
|
|
@ -1732,6 +1732,17 @@
|
||||||
margin-top: -0.5rem;
|
margin-top: -0.5rem;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
.p-inputotp {
|
||||||
|
display: flex;
|
||||||
|
align-items: center;
|
||||||
|
gap: 0.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
|
.p-inputotp-input {
|
||||||
|
text-align: center;
|
||||||
|
width: 2.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
.p-inputgroup {
|
.p-inputgroup {
|
||||||
display: flex;
|
display: flex;
|
||||||
align-items: stretch;
|
align-items: stretch;
|
||||||
|
|
|
@ -1732,6 +1732,17 @@
|
||||||
margin-top: -0.5rem;
|
margin-top: -0.5rem;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
.p-inputotp {
|
||||||
|
display: flex;
|
||||||
|
align-items: center;
|
||||||
|
gap: 0.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
|
.p-inputotp-input {
|
||||||
|
text-align: center;
|
||||||
|
width: 2.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
.p-inputgroup {
|
.p-inputgroup {
|
||||||
display: flex;
|
display: flex;
|
||||||
align-items: stretch;
|
align-items: stretch;
|
||||||
|
|
|
@ -1732,6 +1732,17 @@
|
||||||
margin-top: -0.5rem;
|
margin-top: -0.5rem;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
.p-inputotp {
|
||||||
|
display: flex;
|
||||||
|
align-items: center;
|
||||||
|
gap: 0.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
|
.p-inputotp-input {
|
||||||
|
text-align: center;
|
||||||
|
width: 2.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
.p-inputgroup {
|
.p-inputgroup {
|
||||||
display: flex;
|
display: flex;
|
||||||
align-items: stretch;
|
align-items: stretch;
|
||||||
|
|
|
@ -1732,6 +1732,17 @@
|
||||||
margin-top: -0.5rem;
|
margin-top: -0.5rem;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
.p-inputotp {
|
||||||
|
display: flex;
|
||||||
|
align-items: center;
|
||||||
|
gap: 0.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
|
.p-inputotp-input {
|
||||||
|
text-align: center;
|
||||||
|
width: 2.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
.p-inputgroup {
|
.p-inputgroup {
|
||||||
display: flex;
|
display: flex;
|
||||||
align-items: stretch;
|
align-items: stretch;
|
||||||
|
|
|
@ -1734,6 +1734,17 @@
|
||||||
margin-top: -0.5rem;
|
margin-top: -0.5rem;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
.p-inputotp {
|
||||||
|
display: flex;
|
||||||
|
align-items: center;
|
||||||
|
gap: 0.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
|
.p-inputotp-input {
|
||||||
|
text-align: center;
|
||||||
|
width: 2.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
.p-inputgroup {
|
.p-inputgroup {
|
||||||
display: flex;
|
display: flex;
|
||||||
align-items: stretch;
|
align-items: stretch;
|
||||||
|
|
|
@ -1734,6 +1734,17 @@
|
||||||
margin-top: -0.5rem;
|
margin-top: -0.5rem;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
.p-inputotp {
|
||||||
|
display: flex;
|
||||||
|
align-items: center;
|
||||||
|
gap: 0.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
|
.p-inputotp-input {
|
||||||
|
text-align: center;
|
||||||
|
width: 2.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
.p-inputgroup {
|
.p-inputgroup {
|
||||||
display: flex;
|
display: flex;
|
||||||
align-items: stretch;
|
align-items: stretch;
|
||||||
|
|
|
@ -1734,6 +1734,17 @@
|
||||||
margin-top: -0.5rem;
|
margin-top: -0.5rem;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
.p-inputotp {
|
||||||
|
display: flex;
|
||||||
|
align-items: center;
|
||||||
|
gap: 0.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
|
.p-inputotp-input {
|
||||||
|
text-align: center;
|
||||||
|
width: 2.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
.p-inputgroup {
|
.p-inputgroup {
|
||||||
display: flex;
|
display: flex;
|
||||||
align-items: stretch;
|
align-items: stretch;
|
||||||
|
|
|
@ -1734,6 +1734,17 @@
|
||||||
margin-top: -0.5rem;
|
margin-top: -0.5rem;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
.p-inputotp {
|
||||||
|
display: flex;
|
||||||
|
align-items: center;
|
||||||
|
gap: 0.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
|
.p-inputotp-input {
|
||||||
|
text-align: center;
|
||||||
|
width: 2.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
.p-inputgroup {
|
.p-inputgroup {
|
||||||
display: flex;
|
display: flex;
|
||||||
align-items: stretch;
|
align-items: stretch;
|
||||||
|
|
|
@ -1734,6 +1734,17 @@
|
||||||
margin-top: -0.5rem;
|
margin-top: -0.5rem;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
.p-inputotp {
|
||||||
|
display: flex;
|
||||||
|
align-items: center;
|
||||||
|
gap: 0.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
|
.p-inputotp-input {
|
||||||
|
text-align: center;
|
||||||
|
width: 2.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
.p-inputgroup {
|
.p-inputgroup {
|
||||||
display: flex;
|
display: flex;
|
||||||
align-items: stretch;
|
align-items: stretch;
|
||||||
|
|
|
@ -1734,6 +1734,17 @@
|
||||||
margin-top: -0.5rem;
|
margin-top: -0.5rem;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
.p-inputotp {
|
||||||
|
display: flex;
|
||||||
|
align-items: center;
|
||||||
|
gap: 0.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
|
.p-inputotp-input {
|
||||||
|
text-align: center;
|
||||||
|
width: 2.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
.p-inputgroup {
|
.p-inputgroup {
|
||||||
display: flex;
|
display: flex;
|
||||||
align-items: stretch;
|
align-items: stretch;
|
||||||
|
|
|
@ -1738,6 +1738,17 @@
|
||||||
margin-top: -0.5rem;
|
margin-top: -0.5rem;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
.p-inputotp {
|
||||||
|
display: flex;
|
||||||
|
align-items: center;
|
||||||
|
gap: 0.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
|
.p-inputotp-input {
|
||||||
|
text-align: center;
|
||||||
|
width: 2.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
.p-inputgroup {
|
.p-inputgroup {
|
||||||
display: flex;
|
display: flex;
|
||||||
align-items: stretch;
|
align-items: stretch;
|
||||||
|
|
|
@ -1734,6 +1734,17 @@
|
||||||
margin-top: -0.5rem;
|
margin-top: -0.5rem;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
.p-inputotp {
|
||||||
|
display: flex;
|
||||||
|
align-items: center;
|
||||||
|
gap: 0.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
|
.p-inputotp-input {
|
||||||
|
text-align: center;
|
||||||
|
width: 2.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
.p-inputgroup {
|
.p-inputgroup {
|
||||||
display: flex;
|
display: flex;
|
||||||
align-items: stretch;
|
align-items: stretch;
|
||||||
|
|
|
@ -1734,6 +1734,17 @@
|
||||||
margin-top: -0.5rem;
|
margin-top: -0.5rem;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
.p-inputotp {
|
||||||
|
display: flex;
|
||||||
|
align-items: center;
|
||||||
|
gap: 0.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
|
.p-inputotp-input {
|
||||||
|
text-align: center;
|
||||||
|
width: 2.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
.p-inputgroup {
|
.p-inputgroup {
|
||||||
display: flex;
|
display: flex;
|
||||||
align-items: stretch;
|
align-items: stretch;
|
||||||
|
|
|
@ -1734,6 +1734,17 @@
|
||||||
margin-top: -0.5rem;
|
margin-top: -0.5rem;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
.p-inputotp {
|
||||||
|
display: flex;
|
||||||
|
align-items: center;
|
||||||
|
gap: 0.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
|
.p-inputotp-input {
|
||||||
|
text-align: center;
|
||||||
|
width: 2.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
.p-inputgroup {
|
.p-inputgroup {
|
||||||
display: flex;
|
display: flex;
|
||||||
align-items: stretch;
|
align-items: stretch;
|
||||||
|
|
|
@ -1717,6 +1717,17 @@
|
||||||
margin-top: -0.5rem;
|
margin-top: -0.5rem;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
.p-inputotp {
|
||||||
|
display: flex;
|
||||||
|
align-items: center;
|
||||||
|
gap: 0.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
|
.p-inputotp-input {
|
||||||
|
text-align: center;
|
||||||
|
width: 2.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
.p-inputgroup {
|
.p-inputgroup {
|
||||||
display: flex;
|
display: flex;
|
||||||
align-items: stretch;
|
align-items: stretch;
|
||||||
|
|
|
@ -1717,6 +1717,17 @@
|
||||||
margin-top: -0.5rem;
|
margin-top: -0.5rem;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
.p-inputotp {
|
||||||
|
display: flex;
|
||||||
|
align-items: center;
|
||||||
|
gap: 0.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
|
.p-inputotp-input {
|
||||||
|
text-align: center;
|
||||||
|
width: 2.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
.p-inputgroup {
|
.p-inputgroup {
|
||||||
display: flex;
|
display: flex;
|
||||||
align-items: stretch;
|
align-items: stretch;
|
||||||
|
|
|
@ -1717,6 +1717,17 @@
|
||||||
margin-top: -0.5rem;
|
margin-top: -0.5rem;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
.p-inputotp {
|
||||||
|
display: flex;
|
||||||
|
align-items: center;
|
||||||
|
gap: 0.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
|
.p-inputotp-input {
|
||||||
|
text-align: center;
|
||||||
|
width: 2.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
.p-inputgroup {
|
.p-inputgroup {
|
||||||
display: flex;
|
display: flex;
|
||||||
align-items: stretch;
|
align-items: stretch;
|
||||||
|
|
|
@ -1717,6 +1717,17 @@
|
||||||
margin-top: -0.5rem;
|
margin-top: -0.5rem;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
.p-inputotp {
|
||||||
|
display: flex;
|
||||||
|
align-items: center;
|
||||||
|
gap: 0.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
|
.p-inputotp-input {
|
||||||
|
text-align: center;
|
||||||
|
width: 2.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
.p-inputgroup {
|
.p-inputgroup {
|
||||||
display: flex;
|
display: flex;
|
||||||
align-items: stretch;
|
align-items: stretch;
|
||||||
|
|
|
@ -1713,6 +1713,17 @@
|
||||||
margin-top: -0.5rem;
|
margin-top: -0.5rem;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
.p-inputotp {
|
||||||
|
display: flex;
|
||||||
|
align-items: center;
|
||||||
|
gap: 0.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
|
.p-inputotp-input {
|
||||||
|
text-align: center;
|
||||||
|
width: 2rem;
|
||||||
|
}
|
||||||
|
|
||||||
.p-inputgroup {
|
.p-inputgroup {
|
||||||
display: flex;
|
display: flex;
|
||||||
align-items: stretch;
|
align-items: stretch;
|
||||||
|
|
|
@ -1732,6 +1732,17 @@
|
||||||
margin-top: -0.5rem;
|
margin-top: -0.5rem;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
.p-inputotp {
|
||||||
|
display: flex;
|
||||||
|
align-items: center;
|
||||||
|
gap: 0.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
|
.p-inputotp-input {
|
||||||
|
text-align: center;
|
||||||
|
width: 2.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
.p-inputgroup {
|
.p-inputgroup {
|
||||||
display: flex;
|
display: flex;
|
||||||
align-items: stretch;
|
align-items: stretch;
|
||||||
|
|
|
@ -1732,6 +1732,17 @@
|
||||||
margin-top: -0.5rem;
|
margin-top: -0.5rem;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
.p-inputotp {
|
||||||
|
display: flex;
|
||||||
|
align-items: center;
|
||||||
|
gap: 0.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
|
.p-inputotp-input {
|
||||||
|
text-align: center;
|
||||||
|
width: 2.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
.p-inputgroup {
|
.p-inputgroup {
|
||||||
display: flex;
|
display: flex;
|
||||||
align-items: stretch;
|
align-items: stretch;
|
||||||
|
|
|
@ -1732,6 +1732,17 @@
|
||||||
margin-top: -0.5rem;
|
margin-top: -0.5rem;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
.p-inputotp {
|
||||||
|
display: flex;
|
||||||
|
align-items: center;
|
||||||
|
gap: 0.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
|
.p-inputotp-input {
|
||||||
|
text-align: center;
|
||||||
|
width: 2.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
.p-inputgroup {
|
.p-inputgroup {
|
||||||
display: flex;
|
display: flex;
|
||||||
align-items: stretch;
|
align-items: stretch;
|
||||||
|
|
|
@ -1732,6 +1732,17 @@
|
||||||
margin-top: -0.5rem;
|
margin-top: -0.5rem;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
.p-inputotp {
|
||||||
|
display: flex;
|
||||||
|
align-items: center;
|
||||||
|
gap: 0.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
|
.p-inputotp-input {
|
||||||
|
text-align: center;
|
||||||
|
width: 2.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
.p-inputgroup {
|
.p-inputgroup {
|
||||||
display: flex;
|
display: flex;
|
||||||
align-items: stretch;
|
align-items: stretch;
|
||||||
|
|
|
@ -1732,6 +1732,17 @@
|
||||||
margin-top: -0.5rem;
|
margin-top: -0.5rem;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
.p-inputotp {
|
||||||
|
display: flex;
|
||||||
|
align-items: center;
|
||||||
|
gap: 0.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
|
.p-inputotp-input {
|
||||||
|
text-align: center;
|
||||||
|
width: 2.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
.p-inputgroup {
|
.p-inputgroup {
|
||||||
display: flex;
|
display: flex;
|
||||||
align-items: stretch;
|
align-items: stretch;
|
||||||
|
|
|
@ -1732,6 +1732,17 @@
|
||||||
margin-top: -0.5rem;
|
margin-top: -0.5rem;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
.p-inputotp {
|
||||||
|
display: flex;
|
||||||
|
align-items: center;
|
||||||
|
gap: 0.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
|
.p-inputotp-input {
|
||||||
|
text-align: center;
|
||||||
|
width: 2.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
.p-inputgroup {
|
.p-inputgroup {
|
||||||
display: flex;
|
display: flex;
|
||||||
align-items: stretch;
|
align-items: stretch;
|
||||||
|
|
|
@ -1732,6 +1732,17 @@
|
||||||
margin-top: -0.5rem;
|
margin-top: -0.5rem;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
.p-inputotp {
|
||||||
|
display: flex;
|
||||||
|
align-items: center;
|
||||||
|
gap: 0.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
|
.p-inputotp-input {
|
||||||
|
text-align: center;
|
||||||
|
width: 2.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
.p-inputgroup {
|
.p-inputgroup {
|
||||||
display: flex;
|
display: flex;
|
||||||
align-items: stretch;
|
align-items: stretch;
|
||||||
|
|
|
@ -1732,6 +1732,17 @@
|
||||||
margin-top: -0.5rem;
|
margin-top: -0.5rem;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
.p-inputotp {
|
||||||
|
display: flex;
|
||||||
|
align-items: center;
|
||||||
|
gap: 0.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
|
.p-inputotp-input {
|
||||||
|
text-align: center;
|
||||||
|
width: 2.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
.p-inputgroup {
|
.p-inputgroup {
|
||||||
display: flex;
|
display: flex;
|
||||||
align-items: stretch;
|
align-items: stretch;
|
||||||
|
|
|
@ -1732,6 +1732,17 @@
|
||||||
margin-top: -0.5rem;
|
margin-top: -0.5rem;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
.p-inputotp {
|
||||||
|
display: flex;
|
||||||
|
align-items: center;
|
||||||
|
gap: 0.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
|
.p-inputotp-input {
|
||||||
|
text-align: center;
|
||||||
|
width: 2.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
.p-inputgroup {
|
.p-inputgroup {
|
||||||
display: flex;
|
display: flex;
|
||||||
align-items: stretch;
|
align-items: stretch;
|
||||||
|
|
|
@ -1732,6 +1732,17 @@
|
||||||
margin-top: -0.5rem;
|
margin-top: -0.5rem;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
.p-inputotp {
|
||||||
|
display: flex;
|
||||||
|
align-items: center;
|
||||||
|
gap: 0.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
|
.p-inputotp-input {
|
||||||
|
text-align: center;
|
||||||
|
width: 2.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
.p-inputgroup {
|
.p-inputgroup {
|
||||||
display: flex;
|
display: flex;
|
||||||
align-items: stretch;
|
align-items: stretch;
|
||||||
|
|
|
@ -1732,6 +1732,17 @@
|
||||||
margin-top: -0.5rem;
|
margin-top: -0.5rem;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
.p-inputotp {
|
||||||
|
display: flex;
|
||||||
|
align-items: center;
|
||||||
|
gap: 0.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
|
.p-inputotp-input {
|
||||||
|
text-align: center;
|
||||||
|
width: 2.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
.p-inputgroup {
|
.p-inputgroup {
|
||||||
display: flex;
|
display: flex;
|
||||||
align-items: stretch;
|
align-items: stretch;
|
||||||
|
|
|
@ -1732,6 +1732,17 @@
|
||||||
margin-top: -0.5rem;
|
margin-top: -0.5rem;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
.p-inputotp {
|
||||||
|
display: flex;
|
||||||
|
align-items: center;
|
||||||
|
gap: 0.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
|
.p-inputotp-input {
|
||||||
|
text-align: center;
|
||||||
|
width: 2.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
.p-inputgroup {
|
.p-inputgroup {
|
||||||
display: flex;
|
display: flex;
|
||||||
align-items: stretch;
|
align-items: stretch;
|
||||||
|
|
|
@ -1732,6 +1732,17 @@
|
||||||
margin-top: -0.5rem;
|
margin-top: -0.5rem;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
.p-inputotp {
|
||||||
|
display: flex;
|
||||||
|
align-items: center;
|
||||||
|
gap: 0.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
|
.p-inputotp-input {
|
||||||
|
text-align: center;
|
||||||
|
width: 2.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
.p-inputgroup {
|
.p-inputgroup {
|
||||||
display: flex;
|
display: flex;
|
||||||
align-items: stretch;
|
align-items: stretch;
|
||||||
|
|
|
@ -1732,6 +1732,17 @@
|
||||||
margin-top: -0.5rem;
|
margin-top: -0.5rem;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
.p-inputotp {
|
||||||
|
display: flex;
|
||||||
|
align-items: center;
|
||||||
|
gap: 0.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
|
.p-inputotp-input {
|
||||||
|
text-align: center;
|
||||||
|
width: 2.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
.p-inputgroup {
|
.p-inputgroup {
|
||||||
display: flex;
|
display: flex;
|
||||||
align-items: stretch;
|
align-items: stretch;
|
||||||
|
|
|
@ -1732,6 +1732,17 @@
|
||||||
margin-top: -0.5rem;
|
margin-top: -0.5rem;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
.p-inputotp {
|
||||||
|
display: flex;
|
||||||
|
align-items: center;
|
||||||
|
gap: 0.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
|
.p-inputotp-input {
|
||||||
|
text-align: center;
|
||||||
|
width: 2.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
.p-inputgroup {
|
.p-inputgroup {
|
||||||
display: flex;
|
display: flex;
|
||||||
align-items: stretch;
|
align-items: stretch;
|
||||||
|
|
|
@ -1732,6 +1732,17 @@
|
||||||
margin-top: -0.5rem;
|
margin-top: -0.5rem;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
.p-inputotp {
|
||||||
|
display: flex;
|
||||||
|
align-items: center;
|
||||||
|
gap: 0.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
|
.p-inputotp-input {
|
||||||
|
text-align: center;
|
||||||
|
width: 2.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
.p-inputgroup {
|
.p-inputgroup {
|
||||||
display: flex;
|
display: flex;
|
||||||
align-items: stretch;
|
align-items: stretch;
|
||||||
|
|
|
@ -1717,6 +1717,17 @@
|
||||||
margin-top: -0.5rem;
|
margin-top: -0.5rem;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
.p-inputotp {
|
||||||
|
display: flex;
|
||||||
|
align-items: center;
|
||||||
|
gap: 0.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
|
.p-inputotp-input {
|
||||||
|
text-align: center;
|
||||||
|
width: 1.858rem;
|
||||||
|
}
|
||||||
|
|
||||||
.p-inputgroup {
|
.p-inputgroup {
|
||||||
display: flex;
|
display: flex;
|
||||||
align-items: stretch;
|
align-items: stretch;
|
||||||
|
|
|
@ -1717,6 +1717,17 @@
|
||||||
margin-top: -0.5rem;
|
margin-top: -0.5rem;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
.p-inputotp {
|
||||||
|
display: flex;
|
||||||
|
align-items: center;
|
||||||
|
gap: 0.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
|
.p-inputotp-input {
|
||||||
|
text-align: center;
|
||||||
|
width: 1.858rem;
|
||||||
|
}
|
||||||
|
|
||||||
.p-inputgroup {
|
.p-inputgroup {
|
||||||
display: flex;
|
display: flex;
|
||||||
align-items: stretch;
|
align-items: stretch;
|
||||||
|
|
|
@ -1717,6 +1717,17 @@
|
||||||
margin-top: -0.5rem;
|
margin-top: -0.5rem;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
.p-inputotp {
|
||||||
|
display: flex;
|
||||||
|
align-items: center;
|
||||||
|
gap: 0.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
|
.p-inputotp-input {
|
||||||
|
text-align: center;
|
||||||
|
width: 1.858rem;
|
||||||
|
}
|
||||||
|
|
||||||
.p-inputgroup {
|
.p-inputgroup {
|
||||||
display: flex;
|
display: flex;
|
||||||
align-items: stretch;
|
align-items: stretch;
|
||||||
|
|
|
@ -1717,6 +1717,17 @@
|
||||||
margin-top: -0.5rem;
|
margin-top: -0.5rem;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
.p-inputotp {
|
||||||
|
display: flex;
|
||||||
|
align-items: center;
|
||||||
|
gap: 0.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
|
.p-inputotp-input {
|
||||||
|
text-align: center;
|
||||||
|
width: 1.858rem;
|
||||||
|
}
|
||||||
|
|
||||||
.p-inputgroup {
|
.p-inputgroup {
|
||||||
display: flex;
|
display: flex;
|
||||||
align-items: stretch;
|
align-items: stretch;
|
||||||
|
|
|
@ -1738,6 +1738,17 @@
|
||||||
margin-top: -0.5rem;
|
margin-top: -0.5rem;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
.p-inputotp {
|
||||||
|
display: flex;
|
||||||
|
align-items: center;
|
||||||
|
gap: 0.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
|
.p-inputotp-input {
|
||||||
|
text-align: center;
|
||||||
|
width: 3rem;
|
||||||
|
}
|
||||||
|
|
||||||
.p-inputgroup {
|
.p-inputgroup {
|
||||||
display: flex;
|
display: flex;
|
||||||
align-items: stretch;
|
align-items: stretch;
|
||||||
|
|
|
@ -1738,6 +1738,17 @@
|
||||||
margin-top: -0.5rem;
|
margin-top: -0.5rem;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
.p-inputotp {
|
||||||
|
display: flex;
|
||||||
|
align-items: center;
|
||||||
|
gap: 0.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
|
.p-inputotp-input {
|
||||||
|
text-align: center;
|
||||||
|
width: 3rem;
|
||||||
|
}
|
||||||
|
|
||||||
.p-inputgroup {
|
.p-inputgroup {
|
||||||
display: flex;
|
display: flex;
|
||||||
align-items: stretch;
|
align-items: stretch;
|
||||||
|
|
|
@ -1737,6 +1737,17 @@
|
||||||
margin-top: -0.5rem;
|
margin-top: -0.5rem;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
.p-inputotp {
|
||||||
|
display: flex;
|
||||||
|
align-items: center;
|
||||||
|
gap: 0.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
|
.p-inputotp-input {
|
||||||
|
text-align: center;
|
||||||
|
width: 3rem;
|
||||||
|
}
|
||||||
|
|
||||||
.p-inputgroup {
|
.p-inputgroup {
|
||||||
display: flex;
|
display: flex;
|
||||||
align-items: stretch;
|
align-items: stretch;
|
||||||
|
|
|
@ -1737,6 +1737,17 @@
|
||||||
margin-top: -0.5rem;
|
margin-top: -0.5rem;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
.p-inputotp {
|
||||||
|
display: flex;
|
||||||
|
align-items: center;
|
||||||
|
gap: 0.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
|
.p-inputotp-input {
|
||||||
|
text-align: center;
|
||||||
|
width: 3rem;
|
||||||
|
}
|
||||||
|
|
||||||
.p-inputgroup {
|
.p-inputgroup {
|
||||||
display: flex;
|
display: flex;
|
||||||
align-items: stretch;
|
align-items: stretch;
|
||||||
|
|
|
@ -1738,6 +1738,17 @@
|
||||||
margin-top: -0.5rem;
|
margin-top: -0.5rem;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
.p-inputotp {
|
||||||
|
display: flex;
|
||||||
|
align-items: center;
|
||||||
|
gap: 0.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
|
.p-inputotp-input {
|
||||||
|
text-align: center;
|
||||||
|
width: 2.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
.p-inputgroup {
|
.p-inputgroup {
|
||||||
display: flex;
|
display: flex;
|
||||||
align-items: stretch;
|
align-items: stretch;
|
||||||
|
|
|
@ -1738,6 +1738,17 @@
|
||||||
margin-top: -0.5rem;
|
margin-top: -0.5rem;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
.p-inputotp {
|
||||||
|
display: flex;
|
||||||
|
align-items: center;
|
||||||
|
gap: 0.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
|
.p-inputotp-input {
|
||||||
|
text-align: center;
|
||||||
|
width: 2.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
.p-inputgroup {
|
.p-inputgroup {
|
||||||
display: flex;
|
display: flex;
|
||||||
align-items: stretch;
|
align-items: stretch;
|
||||||
|
|
|
@ -1737,6 +1737,17 @@
|
||||||
margin-top: -0.5rem;
|
margin-top: -0.5rem;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
.p-inputotp {
|
||||||
|
display: flex;
|
||||||
|
align-items: center;
|
||||||
|
gap: 0.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
|
.p-inputotp-input {
|
||||||
|
text-align: center;
|
||||||
|
width: 2.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
.p-inputgroup {
|
.p-inputgroup {
|
||||||
display: flex;
|
display: flex;
|
||||||
align-items: stretch;
|
align-items: stretch;
|
||||||
|
|
|
@ -1737,6 +1737,17 @@
|
||||||
margin-top: -0.5rem;
|
margin-top: -0.5rem;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
.p-inputotp {
|
||||||
|
display: flex;
|
||||||
|
align-items: center;
|
||||||
|
gap: 0.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
|
.p-inputotp-input {
|
||||||
|
text-align: center;
|
||||||
|
width: 2.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
.p-inputgroup {
|
.p-inputgroup {
|
||||||
display: flex;
|
display: flex;
|
||||||
align-items: stretch;
|
align-items: stretch;
|
||||||
|
|
|
@ -1741,6 +1741,17 @@
|
||||||
margin-top: -0.5rem;
|
margin-top: -0.5rem;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
.p-inputotp {
|
||||||
|
display: flex;
|
||||||
|
align-items: center;
|
||||||
|
gap: 0.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
|
.p-inputotp-input {
|
||||||
|
text-align: center;
|
||||||
|
width: 2rem;
|
||||||
|
}
|
||||||
|
|
||||||
.p-inputgroup {
|
.p-inputgroup {
|
||||||
display: flex;
|
display: flex;
|
||||||
align-items: stretch;
|
align-items: stretch;
|
||||||
|
|
|
@ -1713,6 +1713,17 @@
|
||||||
margin-top: -0.5rem;
|
margin-top: -0.5rem;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
.p-inputotp {
|
||||||
|
display: flex;
|
||||||
|
align-items: center;
|
||||||
|
gap: 0.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
|
.p-inputotp-input {
|
||||||
|
text-align: center;
|
||||||
|
width: 1.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
.p-inputgroup {
|
.p-inputgroup {
|
||||||
display: flex;
|
display: flex;
|
||||||
align-items: stretch;
|
align-items: stretch;
|
||||||
|
|
|
@ -1713,6 +1713,17 @@
|
||||||
margin-top: -0.5rem;
|
margin-top: -0.5rem;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
.p-inputotp {
|
||||||
|
display: flex;
|
||||||
|
align-items: center;
|
||||||
|
gap: 0.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
|
.p-inputotp-input {
|
||||||
|
text-align: center;
|
||||||
|
width: 1.858rem;
|
||||||
|
}
|
||||||
|
|
||||||
.p-inputgroup {
|
.p-inputgroup {
|
||||||
display: flex;
|
display: flex;
|
||||||
align-items: stretch;
|
align-items: stretch;
|
||||||
|
|
|
@ -1717,6 +1717,17 @@
|
||||||
margin-top: -0.5rem;
|
margin-top: -0.5rem;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
.p-inputotp {
|
||||||
|
display: flex;
|
||||||
|
align-items: center;
|
||||||
|
gap: 0.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
|
.p-inputotp-input {
|
||||||
|
text-align: center;
|
||||||
|
width: 1.858rem;
|
||||||
|
}
|
||||||
|
|
||||||
.p-inputgroup {
|
.p-inputgroup {
|
||||||
display: flex;
|
display: flex;
|
||||||
align-items: stretch;
|
align-items: stretch;
|
||||||
|
|
|
@ -1717,6 +1717,17 @@
|
||||||
margin-top: -0.5rem;
|
margin-top: -0.5rem;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
.p-inputotp {
|
||||||
|
display: flex;
|
||||||
|
align-items: center;
|
||||||
|
gap: 0.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
|
.p-inputotp-input {
|
||||||
|
text-align: center;
|
||||||
|
width: 1.858rem;
|
||||||
|
}
|
||||||
|
|
||||||
.p-inputgroup {
|
.p-inputgroup {
|
||||||
display: flex;
|
display: flex;
|
||||||
align-items: stretch;
|
align-items: stretch;
|
||||||
|
|
|
@ -1717,6 +1717,17 @@
|
||||||
margin-top: -0.5rem;
|
margin-top: -0.5rem;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
.p-inputotp {
|
||||||
|
display: flex;
|
||||||
|
align-items: center;
|
||||||
|
gap: 0.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
|
.p-inputotp-input {
|
||||||
|
text-align: center;
|
||||||
|
width: 1.858rem;
|
||||||
|
}
|
||||||
|
|
||||||
.p-inputgroup {
|
.p-inputgroup {
|
||||||
display: flex;
|
display: flex;
|
||||||
align-items: stretch;
|
align-items: stretch;
|
||||||
|
|
|
@ -1713,6 +1713,17 @@
|
||||||
margin-top: -0.5rem;
|
margin-top: -0.5rem;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
.p-inputotp {
|
||||||
|
display: flex;
|
||||||
|
align-items: center;
|
||||||
|
gap: 0.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
|
.p-inputotp-input {
|
||||||
|
text-align: center;
|
||||||
|
width: 1.858rem;
|
||||||
|
}
|
||||||
|
|
||||||
.p-inputgroup {
|
.p-inputgroup {
|
||||||
display: flex;
|
display: flex;
|
||||||
align-items: stretch;
|
align-items: stretch;
|
||||||
|
|
|
@ -1713,6 +1713,17 @@
|
||||||
margin-top: -0.5rem;
|
margin-top: -0.5rem;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
.p-inputotp {
|
||||||
|
display: flex;
|
||||||
|
align-items: center;
|
||||||
|
gap: 0.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
|
.p-inputotp-input {
|
||||||
|
text-align: center;
|
||||||
|
width: 2rem;
|
||||||
|
}
|
||||||
|
|
||||||
.p-inputgroup {
|
.p-inputgroup {
|
||||||
display: flex;
|
display: flex;
|
||||||
align-items: stretch;
|
align-items: stretch;
|
||||||
|
|
|
@ -1713,6 +1713,17 @@
|
||||||
margin-top: -0.5rem;
|
margin-top: -0.5rem;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
.p-inputotp {
|
||||||
|
display: flex;
|
||||||
|
align-items: center;
|
||||||
|
gap: 0.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
|
.p-inputotp-input {
|
||||||
|
text-align: center;
|
||||||
|
width: 2rem;
|
||||||
|
}
|
||||||
|
|
||||||
.p-inputgroup {
|
.p-inputgroup {
|
||||||
display: flex;
|
display: flex;
|
||||||
align-items: stretch;
|
align-items: stretch;
|
||||||
|
|
|
@ -1713,6 +1713,17 @@
|
||||||
margin-top: -0.5rem;
|
margin-top: -0.5rem;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
.p-inputotp {
|
||||||
|
display: flex;
|
||||||
|
align-items: center;
|
||||||
|
gap: 0.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
|
.p-inputotp-input {
|
||||||
|
text-align: center;
|
||||||
|
width: 2rem;
|
||||||
|
}
|
||||||
|
|
||||||
.p-inputgroup {
|
.p-inputgroup {
|
||||||
display: flex;
|
display: flex;
|
||||||
align-items: stretch;
|
align-items: stretch;
|
||||||
|
|
|
@ -1713,6 +1713,17 @@
|
||||||
margin-top: -0.5rem;
|
margin-top: -0.5rem;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
.p-inputotp {
|
||||||
|
display: flex;
|
||||||
|
align-items: center;
|
||||||
|
gap: 0.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
|
.p-inputotp-input {
|
||||||
|
text-align: center;
|
||||||
|
width: 2rem;
|
||||||
|
}
|
||||||
|
|
||||||
.p-inputgroup {
|
.p-inputgroup {
|
||||||
display: flex;
|
display: flex;
|
||||||
align-items: stretch;
|
align-items: stretch;
|
||||||
|
|
|
@ -1737,6 +1737,17 @@
|
||||||
margin-top: -0.5rem;
|
margin-top: -0.5rem;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
.p-inputotp {
|
||||||
|
display: flex;
|
||||||
|
align-items: center;
|
||||||
|
gap: 0.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
|
.p-inputotp-input {
|
||||||
|
text-align: center;
|
||||||
|
width: 2.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
.p-inputgroup {
|
.p-inputgroup {
|
||||||
display: flex;
|
display: flex;
|
||||||
align-items: stretch;
|
align-items: stretch;
|
||||||
|
|
|
@ -1737,6 +1737,17 @@
|
||||||
margin-top: -0.5rem;
|
margin-top: -0.5rem;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
.p-inputotp {
|
||||||
|
display: flex;
|
||||||
|
align-items: center;
|
||||||
|
gap: 0.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
|
.p-inputotp-input {
|
||||||
|
text-align: center;
|
||||||
|
width: 2.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
.p-inputgroup {
|
.p-inputgroup {
|
||||||
display: flex;
|
display: flex;
|
||||||
align-items: stretch;
|
align-items: stretch;
|
||||||
|
|
|
@ -1748,6 +1748,17 @@
|
||||||
margin-top: -0.5rem;
|
margin-top: -0.5rem;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
.p-inputotp {
|
||||||
|
display: flex;
|
||||||
|
align-items: center;
|
||||||
|
gap: 0.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
|
.p-inputotp-input {
|
||||||
|
text-align: center;
|
||||||
|
width: 2.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
.p-inputgroup {
|
.p-inputgroup {
|
||||||
display: flex;
|
display: flex;
|
||||||
align-items: stretch;
|
align-items: stretch;
|
||||||
|
|
|
@ -1713,6 +1713,17 @@
|
||||||
margin-top: -0.5rem;
|
margin-top: -0.5rem;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
.p-inputotp {
|
||||||
|
display: flex;
|
||||||
|
align-items: center;
|
||||||
|
gap: 0.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
|
.p-inputotp-input {
|
||||||
|
text-align: center;
|
||||||
|
width: 2rem;
|
||||||
|
}
|
||||||
|
|
||||||
.p-inputgroup {
|
.p-inputgroup {
|
||||||
display: flex;
|
display: flex;
|
||||||
align-items: stretch;
|
align-items: stretch;
|
||||||
|
|
|
@ -1713,6 +1713,17 @@
|
||||||
margin-top: -0.5rem;
|
margin-top: -0.5rem;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
.p-inputotp {
|
||||||
|
display: flex;
|
||||||
|
align-items: center;
|
||||||
|
gap: 0.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
|
.p-inputotp-input {
|
||||||
|
text-align: center;
|
||||||
|
width: 2rem;
|
||||||
|
}
|
||||||
|
|
||||||
.p-inputgroup {
|
.p-inputgroup {
|
||||||
display: flex;
|
display: flex;
|
||||||
align-items: stretch;
|
align-items: stretch;
|
||||||
|
|
|
@ -1713,6 +1713,17 @@
|
||||||
margin-top: -0.5rem;
|
margin-top: -0.5rem;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
.p-inputotp {
|
||||||
|
display: flex;
|
||||||
|
align-items: center;
|
||||||
|
gap: 0.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
|
.p-inputotp-input {
|
||||||
|
text-align: center;
|
||||||
|
width: 2rem;
|
||||||
|
}
|
||||||
|
|
||||||
.p-inputgroup {
|
.p-inputgroup {
|
||||||
display: flex;
|
display: flex;
|
||||||
align-items: stretch;
|
align-items: stretch;
|
||||||
|
|
|
@ -1713,6 +1713,17 @@
|
||||||
margin-top: -0.5rem;
|
margin-top: -0.5rem;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
.p-inputotp {
|
||||||
|
display: flex;
|
||||||
|
align-items: center;
|
||||||
|
gap: 0.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
|
.p-inputotp-input {
|
||||||
|
text-align: center;
|
||||||
|
width: 2rem;
|
||||||
|
}
|
||||||
|
|
||||||
.p-inputgroup {
|
.p-inputgroup {
|
||||||
display: flex;
|
display: flex;
|
||||||
align-items: stretch;
|
align-items: stretch;
|
||||||
|
|
|
@ -1745,6 +1745,17 @@
|
||||||
margin-top: -0.5rem;
|
margin-top: -0.5rem;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
.p-inputotp {
|
||||||
|
display: flex;
|
||||||
|
align-items: center;
|
||||||
|
gap: 0.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
|
.p-inputotp-input {
|
||||||
|
text-align: center;
|
||||||
|
width: 2.5rem;
|
||||||
|
}
|
||||||
|
|
||||||
.p-inputgroup {
|
.p-inputgroup {
|
||||||
display: flex;
|
display: flex;
|
||||||
align-items: stretch;
|
align-items: stretch;
|
||||||
|
|
Some files were not shown because too many files have changed in this diff Show More
Loading…
Reference in New Issue