diff --git a/components/lib/iconfield/BaseIconField.vue b/components/lib/iconfield/BaseIconField.vue
new file mode 100644
index 000000000..57503366f
--- /dev/null
+++ b/components/lib/iconfield/BaseIconField.vue
@@ -0,0 +1,21 @@
+
diff --git a/components/lib/iconfield/IconField.d.ts b/components/lib/iconfield/IconField.d.ts
new file mode 100644
index 000000000..2de091822
--- /dev/null
+++ b/components/lib/iconfield/IconField.d.ts
@@ -0,0 +1,127 @@
+/**
+ *
+ * IconField is used to select a boolean value.
+ *
+ * [Live Demo](https://www.primevue.org/inputtext/)
+ *
+ * @module iconfield
+ *
+ */
+import { InputHTMLAttributes, VNode } from 'vue';
+import { ComponentHooks } from '../basecomponent';
+import { PassThroughOptions } from '../passthrough';
+import { ClassComponent, GlobalComponentConstructor, PassThrough } from '../ts-helpers';
+
+export declare type IconFieldPassThroughOptionType = IconFieldPassThroughAttributes | ((options: IconFieldPassThroughMethodOptions) => IconFieldPassThroughAttributes | string) | string | null | undefined;
+
+/**
+ * Custom passthrough(pt) option method.
+ */
+export interface IconFieldPassThroughMethodOptions {
+ /**
+ * Defines instance.
+ */
+ instance: any;
+ /**
+ * Defines valid properties.
+ */
+ props: IconFieldProps;
+ /**
+ * Defines valid attributes.
+ */
+ attrs: any;
+ /**
+ * Defines parent options.
+ */
+ parent: any;
+ /**
+ * Defines passthrough(pt) options in global config.
+ */
+ global: object | undefined;
+}
+
+/**
+ * Custom passthrough(pt) options.
+ * @see {@link IconFieldProps.pt}
+ */
+export interface IconFieldPassThroughOptions {
+ /**
+ * Used to pass attributes to the root's DOM element.
+ */
+ root?: IconFieldPassThroughOptionType;
+ /**
+ * Used to manage all lifecycle hooks.
+ * @see {@link BaseComponent.ComponentHooks}
+ */
+ hooks?: ComponentHooks;
+}
+
+/**
+ * Custom passthrough attributes for each DOM elements
+ */
+export interface IconFieldPassThroughAttributes {
+ [key: string]: any;
+}
+
+/**
+ * Defines valid properties in IconField component.
+ */
+export interface IconFieldProps {
+ /**
+ * Position of the icon
+ * @defaultValue right
+ */
+ iconPosition?: 'left' | 'right' | undefined;
+ /**
+ * Used to pass attributes to DOM elements inside the component.
+ * @type {IconFieldPassThroughOptions}
+ */
+ pt?: PassThrough;
+ /**
+ * Used to configure passthrough(pt) options of the component.
+ * @type {PassThroughOptions}
+ */
+ ptOptions?: PassThroughOptions;
+ /**
+ * When enabled, it removes component related styles in the core.
+ * @defaultValue false
+ */
+ unstyled?: boolean;
+}
+
+/**
+ * Defines valid slots in IconField component.
+ */
+export interface IconFieldSlots {
+ /**
+ * Default slot for content.
+ */
+ default(): VNode[];
+}
+
+/**
+ * Defines valid emits in IconField component.
+ */
+export interface IconFieldEmits {}
+
+/**
+ * **PrimeVue - IconField**
+ *
+ * _IconField is used to select a boolean value._
+ *
+ * [Live Demo](https://www.primevue.org/inputtext/)
+ * --- ---
+ * 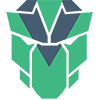
+ *
+ * @group Component
+ *
+ */
+declare class IconField extends ClassComponent {}
+
+declare module '@vue/runtime-core' {
+ interface GlobalComponents {
+ iconfield: GlobalComponentConstructor;
+ }
+}
+
+export default IconField;
diff --git a/components/lib/iconfield/IconField.vue b/components/lib/iconfield/IconField.vue
new file mode 100644
index 000000000..d4a648ecc
--- /dev/null
+++ b/components/lib/iconfield/IconField.vue
@@ -0,0 +1,14 @@
+
+
+
+
+
+
+
diff --git a/components/lib/iconfield/package.json b/components/lib/iconfield/package.json
new file mode 100644
index 000000000..440633c43
--- /dev/null
+++ b/components/lib/iconfield/package.json
@@ -0,0 +1,9 @@
+{
+ "main": "./iconfield.cjs.js",
+ "module": "./iconfield.esm.js",
+ "unpkg": "./iconfield.min.js",
+ "types": "./IconField.d.ts",
+ "browser": {
+ "./sfc": "./IconField.vue"
+ }
+}
diff --git a/components/lib/iconfield/style/IconField.d.ts b/components/lib/iconfield/style/IconField.d.ts
new file mode 100644
index 000000000..0642ad13d
--- /dev/null
+++ b/components/lib/iconfield/style/IconField.d.ts
@@ -0,0 +1,3 @@
+import { BaseStyle } from '../../base/style';
+
+export interface IconFieldStyle extends BaseStyle {}
diff --git a/components/lib/iconfield/style/IconFieldStyle.js b/components/lib/iconfield/style/IconFieldStyle.js
new file mode 100644
index 000000000..9b3ed73d1
--- /dev/null
+++ b/components/lib/iconfield/style/IconFieldStyle.js
@@ -0,0 +1,41 @@
+import BaseStyle from 'primevue/base/style';
+
+const css = `
+@layer primevue {
+ .p-input-icon-left,
+ .p-input-icon-right {
+ position: relative;
+ display: inline-block;
+ }
+
+ .p-input-icon-left > i,
+ .p-input-icon-left > svg,
+ .p-input-icon-right > i,
+ .p-input-icon-right > svg {
+ position: absolute;
+ top: 50%;
+ margin-top: -.5rem;
+ }
+
+ .p-fluid .p-input-icon-left,
+ .p-fluid .p-input-icon-right {
+ display: block;
+ width: 100%;
+ }
+}
+`;
+
+const classes = {
+ root: ({ props }) => [
+ {
+ 'p-input-icon-right': props.iconPosition === 'right',
+ 'p-input-icon-left': props.iconPosition === 'left'
+ }
+ ]
+};
+
+export default BaseStyle.extend({
+ name: 'iconfield',
+ css,
+ classes
+});
diff --git a/components/lib/iconfield/style/package.json b/components/lib/iconfield/style/package.json
new file mode 100644
index 000000000..a9f8fe0bb
--- /dev/null
+++ b/components/lib/iconfield/style/package.json
@@ -0,0 +1,6 @@
+{
+ "main": "./iconfieldstyle.cjs.js",
+ "module": "./iconfieldstyle.esm.js",
+ "unpkg": "./iconfieldstyle.min.js",
+ "types": "./IconFieldStyle.d.ts"
+}