diff --git a/packages/primevue/src/radiobutton/RadioButton.vue b/packages/primevue/src/radiobutton/RadioButton.vue
index 60ffe736b..fd3401007 100755
--- a/packages/primevue/src/radiobutton/RadioButton.vue
+++ b/packages/primevue/src/radiobutton/RadioButton.vue
@@ -6,7 +6,7 @@
:class="[cx('input'), inputClass]"
:style="inputStyle"
:value="value"
- :name="name"
+ :name="groupName"
:checked="checked"
:tabindex="tabindex"
:disabled="disabled"
@@ -34,6 +34,11 @@ export default {
extends: BaseRadioButton,
inheritAttrs: false,
emits: ['change', 'focus', 'blur'],
+ inject: {
+ $pcRadioButtonGroup: {
+ default: undefined
+ }
+ },
methods: {
getPTOptions(key) {
const _ptm = key === 'root' ? this.ptmi : this.ptm;
@@ -49,7 +54,7 @@ export default {
if (!this.disabled && !this.readonly) {
const newModelValue = this.binary ? !this.checked : this.value;
- this.updateValue(newModelValue, event);
+ this.$pcRadioButtonGroup ? this.$pcRadioButtonGroup.updateValue(newModelValue, event) : this.updateValue(newModelValue, event);
this.$emit('change', event);
}
},
@@ -62,8 +67,13 @@ export default {
}
},
computed: {
+ groupName() {
+ return this.$pcRadioButtonGroup ? this.$pcRadioButtonGroup.groupName : this.name;
+ },
checked() {
- return this.d_value != null && (this.binary ? !!this.d_value : equals(this.d_value, this.value));
+ const value = this.$pcRadioButtonGroup ? this.$pcRadioButtonGroup.d_value : this.d_value;
+
+ return value != null && (this.binary ? !!value : equals(value, this.value));
}
}
};
diff --git a/packages/primevue/src/radiobutton/style/RadioButtonStyle.js b/packages/primevue/src/radiobutton/style/RadioButtonStyle.js
index d0416577e..69af9c53e 100644
--- a/packages/primevue/src/radiobutton/style/RadioButtonStyle.js
+++ b/packages/primevue/src/radiobutton/style/RadioButtonStyle.js
@@ -125,7 +125,7 @@ const classes = {
{
'p-radiobutton-checked': instance.checked,
'p-disabled': props.disabled,
- 'p-invalid': instance.$invalid,
+ 'p-invalid': instance.$pcRadioButtonGroup ? instance.$pcRadioButtonGroup.$invalid : instance.$invalid,
'p-variant-filled': instance.$variant === 'filled'
}
],
diff --git a/packages/primevue/src/radiobuttongroup/BaseRadioButtonGroup.vue b/packages/primevue/src/radiobuttongroup/BaseRadioButtonGroup.vue
new file mode 100644
index 000000000..5dc30b203
--- /dev/null
+++ b/packages/primevue/src/radiobuttongroup/BaseRadioButtonGroup.vue
@@ -0,0 +1,16 @@
+
diff --git a/packages/primevue/src/radiobuttongroup/RadioButtonGroup.d.ts b/packages/primevue/src/radiobuttongroup/RadioButtonGroup.d.ts
new file mode 100644
index 000000000..f4f1b6c52
--- /dev/null
+++ b/packages/primevue/src/radiobuttongroup/RadioButtonGroup.d.ts
@@ -0,0 +1,135 @@
+/**
+ *
+ * FloatLabel visually integrates a label with its form element.
+ *
+ * [Live Demo](https://www.primevue.org/floatlabel/)
+ *
+ * @module floatlabel
+ *
+ */
+import type { DefineComponent, DesignToken, EmitFn, PassThrough } from '@primevue/core';
+import type { ComponentHooks } from '@primevue/core/basecomponent';
+import type { PassThroughOptions } from 'primevue/passthrough';
+import { TransitionProps, VNode } from 'vue';
+
+export declare type FloatLabelPassThroughOptionType = FloatLabelPassThroughAttributes | ((options: FloatLabelPassThroughMethodOptions) => FloatLabelPassThroughAttributes | string) | string | null | undefined;
+
+export declare type FloatLabelPassThroughTransitionType = TransitionProps | ((options: FloatLabelPassThroughMethodOptions) => TransitionProps) | undefined;
+
+/**
+ * Custom passthrough(pt) option method.
+ */
+export interface FloatLabelPassThroughMethodOptions {
+ /**
+ * Defines instance.
+ */
+ instance: any;
+ /**
+ * Defines valid properties.
+ */
+ props: FloatLabelProps;
+ /**
+ * Defines valid attributes.
+ */
+ attrs: any;
+ /**
+ * Defines parent options.
+ */
+ parent: any;
+ /**
+ * Defines passthrough(pt) options in global config.
+ */
+ global: object | undefined;
+}
+
+/**
+ * Custom passthrough(pt) options.
+ * @see {@link FloatLabelProps.pt}
+ */
+export interface FloatLabelPassThroughOptions {
+ /**
+ * Used to pass attributes to the root's DOM element.
+ */
+ root?: FloatLabelPassThroughOptionType;
+ /**
+ * Used to manage all lifecycle hooks.
+ * @see {@link BaseComponent.ComponentHooks}
+ */
+ hooks?: ComponentHooks;
+}
+
+/**
+ * Custom passthrough attributes for each DOM elements
+ */
+export interface FloatLabelPassThroughAttributes {
+ [key: string]: any;
+}
+
+/**
+ * Defines valid properties in FloatLabel component.
+ */
+export interface FloatLabelProps {
+ /**
+ * It generates scoped CSS variables using design tokens for the component.
+ */
+ dt?: DesignToken;
+ /**
+ * Used to pass attributes to DOM elements inside the component.
+ * @type {FloatLabelPassThroughOptions}
+ */
+ pt?: PassThrough;
+ /**
+ * Used to configure passthrough(pt) options of the component.
+ * @type {PassThroughOptions}
+ */
+ ptOptions?: PassThroughOptions;
+ /**
+ * When enabled, it removes component related styles in the core.
+ * @defaultValue false
+ */
+ unstyled?: boolean;
+ /**
+ * Defines the positioning of the label relative to the input.
+ * @defaultValue false
+ */
+ variant?: 'over' | 'in' | 'on' | undefined;
+}
+
+/**
+ * Defines valid slots in FloatLabel component.
+ */
+export interface FloatLabelSlots {
+ /**
+ * Default content slot.
+ */
+ default: () => VNode[];
+}
+
+/**
+ * Defines valid emits in FloatLabel component.
+ */
+export interface FloatLabelEmitsOptions {}
+
+export declare type FloatLabelEmits = EmitFn;
+
+/**
+ * **PrimeVue - FloatLabel**
+ *
+ * _FloatLabel visually integrates a label with its form element._
+ *
+ * [Live Demo](https://www.primevue.org/inputtext/)
+ * --- ---
+ * 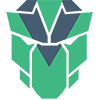
+ *
+ * @group Component
+ *
+ */
+declare const FloatLabel: DefineComponent;
+
+declare module 'vue' {
+ export interface GlobalComponents {
+ FloatLabel: DefineComponent;
+ }
+}
+
+export default FloatLabel;
diff --git a/packages/primevue/src/radiobuttongroup/RadioButtonGroup.vue b/packages/primevue/src/radiobuttongroup/RadioButtonGroup.vue
new file mode 100644
index 000000000..66120108b
--- /dev/null
+++ b/packages/primevue/src/radiobuttongroup/RadioButtonGroup.vue
@@ -0,0 +1,29 @@
+
+
+
+
+
+
+
diff --git a/packages/primevue/src/radiobuttongroup/package.json b/packages/primevue/src/radiobuttongroup/package.json
new file mode 100644
index 000000000..436c3ef18
--- /dev/null
+++ b/packages/primevue/src/radiobuttongroup/package.json
@@ -0,0 +1,11 @@
+{
+ "main": "./RadioButtonGroup.vue",
+ "module": "./RadioButtonGroup.vue",
+ "types": "./RadioButtonGroup.d.ts",
+ "browser": {
+ "./sfc": "./RadioButtonGroup.vue"
+ },
+ "sideEffects": [
+ "*.vue"
+ ]
+}
diff --git a/packages/primevue/src/radiobuttongroup/style/RadioButtonGroupStyle.d.ts b/packages/primevue/src/radiobuttongroup/style/RadioButtonGroupStyle.d.ts
new file mode 100644
index 000000000..1e5f8fa5b
--- /dev/null
+++ b/packages/primevue/src/radiobuttongroup/style/RadioButtonGroupStyle.d.ts
@@ -0,0 +1,19 @@
+/**
+ *
+ * FloatLabel visually integrates a label with its form element.
+ *
+ * [Live Demo](https://www.primevue.org/floatlabel/)
+ *
+ * @module radiobuttongroupstyle
+ *
+ */
+import type { BaseStyle } from '@primevue/core/base/style';
+
+export enum RadioButtonGroupClasses {
+ /**
+ * Class name of the root element
+ */
+ root = 'p-radiobutton-group'
+}
+
+export interface RadioButtonGroupStyle extends BaseStyle {}
diff --git a/packages/primevue/src/radiobuttongroup/style/RadioButtonGroupStyle.js b/packages/primevue/src/radiobuttongroup/style/RadioButtonGroupStyle.js
new file mode 100644
index 000000000..ef01f4ca6
--- /dev/null
+++ b/packages/primevue/src/radiobuttongroup/style/RadioButtonGroupStyle.js
@@ -0,0 +1,17 @@
+import BaseStyle from '@primevue/core/base/style';
+
+const theme = ({ dt }) => `
+.p-radiobutton-group {
+ display: inline-flex;
+}
+`;
+
+const classes = {
+ root: 'p-radiobutton-group p-component'
+};
+
+export default BaseStyle.extend({
+ name: 'radiobuttongroup',
+ theme,
+ classes
+});
diff --git a/packages/primevue/src/radiobuttongroup/style/package.json b/packages/primevue/src/radiobuttongroup/style/package.json
new file mode 100644
index 000000000..9553818fe
--- /dev/null
+++ b/packages/primevue/src/radiobuttongroup/style/package.json
@@ -0,0 +1,6 @@
+{
+ "main": "./RadioButtonGroupStyle.js",
+ "module": "./RadioButtonGroupStyle.js",
+ "types": "./RadioButtonGroupStyle.d.ts",
+ "sideEffects": false
+}