InputOtp documentation
parent
3abe35a3df
commit
2d232f93bb
|
@ -1,9 +1,9 @@
|
|||
const InputSwitchProps = [
|
||||
const InputOtpProps = [
|
||||
{
|
||||
name: 'modelValue',
|
||||
type: 'boolean',
|
||||
default: 'null',
|
||||
description: 'Specifies whether a inputswitch should be checked or not.'
|
||||
description: 'Specifies whether a inputOtp should be checked or not.'
|
||||
},
|
||||
{
|
||||
name: 'trueValue',
|
||||
|
@ -55,7 +55,7 @@ const InputSwitchProps = [
|
|||
}
|
||||
];
|
||||
|
||||
const InputSwitchEvents = [
|
||||
const InputOtpEvents = [
|
||||
{
|
||||
name: 'click',
|
||||
description: 'Callback to invoke on click.'
|
||||
|
@ -71,10 +71,10 @@ const InputSwitchEvents = [
|
|||
];
|
||||
|
||||
module.exports = {
|
||||
inputswitch: {
|
||||
name: 'InputSwitch',
|
||||
description: 'InputSwitch is used to select a boolean value.',
|
||||
props: InputSwitchProps,
|
||||
events: InputSwitchEvents
|
||||
inputotp: {
|
||||
name: 'InputOtp',
|
||||
description: 'InputOtp is used to enter one time passwords.',
|
||||
props: InputOtpProps,
|
||||
events: InputOtpEvents
|
||||
}
|
||||
};
|
||||
|
|
|
@ -193,10 +193,11 @@ import InlineMessage from 'primevue/inlinemessage';
|
|||
import Inplace from 'primevue/inplace';
|
||||
import InputGroup from 'primevue/inputgroup';
|
||||
import InputGroupAddon from 'primevue/inputgroupaddon';
|
||||
import InputSwitch from 'primevue/inputswitch';
|
||||
import InputText from 'primevue/inputtext';
|
||||
import InputMask from 'primevue/inputmask';
|
||||
import InputNumber from 'primevue/inputnumber';
|
||||
import InputOtp from 'primevue/inputotp';
|
||||
import InputSwitch from 'primevue/inputswitch';
|
||||
import InputText from 'primevue/inputtext';
|
||||
import Knob from 'primevue/knob';
|
||||
import Listbox from 'primevue/listbox';
|
||||
import MegaMenu from 'primevue/megamenu';
|
||||
|
@ -313,6 +314,7 @@ app.component('InputGroupAddon', InputGroupAddon);
|
|||
app.component('InputIcon', InputIcon);
|
||||
app.component('InputMask', InputMask);
|
||||
app.component('InputNumber', InputNumber);
|
||||
app.component('InputOtp', InputOtp);
|
||||
app.component('InputSwitch', InputSwitch);
|
||||
app.component('InputText', InputText);
|
||||
app.component('Knob', Knob);
|
||||
|
|
|
@ -1,22 +1,24 @@
|
|||
/**
|
||||
*
|
||||
* InputSwitch is used to select a boolean value.
|
||||
* InputOtp is used to enter one time passwords.
|
||||
*
|
||||
* [Live Demo](https://www.primevue.org/inputswitch/)
|
||||
* [Live Demo](https://www.primevue.org/inputotp/)
|
||||
*
|
||||
* @module inputotp
|
||||
*
|
||||
*/
|
||||
import { VNode } from 'vue';
|
||||
import { ComponentHooks } from '../basecomponent/BaseComponent';
|
||||
import { InputTextPassThroughOptions } from '../inputtext';
|
||||
import { PassThroughOptions } from '../passthrough';
|
||||
import { ClassComponent, GlobalComponentConstructor, PassThrough } from '../ts-helpers';
|
||||
|
||||
export declare type InputSwitchPassThroughOptionType = InputSwitchPassThroughAttributes | ((options: InputSwitchPassThroughMethodOptions) => InputSwitchPassThroughAttributes | string) | string | null | undefined;
|
||||
export declare type InputOtpPassThroughOptionType = InputOtpPassThroughAttributes | ((options: InputOtpPassThroughMethodOptions) => InputOtpPassThroughAttributes | string) | string | null | undefined;
|
||||
|
||||
/**
|
||||
* Custom passthrough(pt) option method.
|
||||
*/
|
||||
export interface InputSwitchPassThroughMethodOptions {
|
||||
export interface InputOtpPassThroughMethodOptions {
|
||||
/**
|
||||
* Defines instance.
|
||||
*/
|
||||
|
@ -24,23 +26,15 @@ export interface InputSwitchPassThroughMethodOptions {
|
|||
/**
|
||||
* Defines valid properties.
|
||||
*/
|
||||
props: InputSwitchProps;
|
||||
props: InputOtpProps;
|
||||
/**
|
||||
* Defines current inline state.
|
||||
*/
|
||||
state: InputSwitchState;
|
||||
/**
|
||||
* Defines current options.
|
||||
*/
|
||||
context: InputSwitchContext;
|
||||
state: InputOtpState;
|
||||
/**
|
||||
* Defines valid attributes.
|
||||
*/
|
||||
attrs: any;
|
||||
/**
|
||||
* Defines parent options.
|
||||
*/
|
||||
parent: any;
|
||||
/**
|
||||
* Defines passthrough(pt) options in global config.
|
||||
*/
|
||||
|
@ -48,22 +42,33 @@ export interface InputSwitchPassThroughMethodOptions {
|
|||
}
|
||||
|
||||
/**
|
||||
* Custom passthrough(pt) options.
|
||||
* @see {@link InputSwitchProps.pt}
|
||||
* Custom shared passthrough(pt) option method.
|
||||
*/
|
||||
export interface InputSwitchPassThroughOptions {
|
||||
export interface InputOtpSharedPassThroughMethodOptions {
|
||||
/**
|
||||
* Defines valid properties.
|
||||
*/
|
||||
props: InputOtpProps;
|
||||
/**
|
||||
* Defines current inline state.
|
||||
*/
|
||||
state: InputOtpState;
|
||||
}
|
||||
|
||||
/**
|
||||
* Custom passthrough(pt) options.
|
||||
* @see {@link InputOtpProps.pt}
|
||||
*/
|
||||
export interface InputOtpPassThroughOptions {
|
||||
/**
|
||||
* Used to pass attributes to the root's DOM element.
|
||||
*/
|
||||
root?: InputSwitchPassThroughOptionType;
|
||||
root?: InputOtpPassThroughOptionType;
|
||||
/**
|
||||
* Used to pass attributes to the input's DOM element.
|
||||
* Used to pass attributes to the InputText component.
|
||||
* @see {@link InputTextPassThroughOptions}
|
||||
*/
|
||||
input?: InputSwitchPassThroughOptionType;
|
||||
/**
|
||||
* Used to pass attributes to the slider's DOM element.
|
||||
*/
|
||||
slider?: InputSwitchPassThroughOptionType;
|
||||
input?: InputTextPassThroughOptions<InputOtpSharedPassThroughMethodOptions>;
|
||||
/**
|
||||
* Used to manage all lifecycle hooks.
|
||||
* @see {@link BaseComponent.ComponentHooks}
|
||||
|
@ -74,36 +79,70 @@ export interface InputSwitchPassThroughOptions {
|
|||
/**
|
||||
* Custom passthrough attributes for each DOM elements
|
||||
*/
|
||||
export interface InputSwitchPassThroughAttributes {
|
||||
export interface InputOtpPassThroughAttributes {
|
||||
[key: string]: any;
|
||||
}
|
||||
|
||||
/**
|
||||
* Defines current inline state in InputSwitch component.
|
||||
* Defines current inline state in InputOtp component.
|
||||
*/
|
||||
export interface InputSwitchState {
|
||||
[key: string]: any;
|
||||
}
|
||||
|
||||
/**
|
||||
* Defines valid properties in InputSwitch component.
|
||||
*/
|
||||
export interface InputSwitchProps {
|
||||
export interface InputOtpState {
|
||||
/**
|
||||
* Specifies whether a inputswitch should be checked or not.
|
||||
* Array of input tokens
|
||||
*/
|
||||
tokens: string[] | number[];
|
||||
}
|
||||
|
||||
/**
|
||||
* InputOtp attr options
|
||||
*/
|
||||
export interface InputOtpTemplateAttrsOptions {
|
||||
/**
|
||||
* Input token value
|
||||
*/
|
||||
value: string;
|
||||
}
|
||||
|
||||
/**
|
||||
* InputOtp templating events
|
||||
*/
|
||||
export interface InputOtpTemplateEvents {
|
||||
/**
|
||||
* Input event
|
||||
* @param {Event} event - Browser event
|
||||
*/
|
||||
input: (event: Event) => void;
|
||||
/**
|
||||
* Keydown event
|
||||
* @param {Event} event - Browser event
|
||||
*/
|
||||
keydown: (event: Event) => void;
|
||||
/**
|
||||
* Focus event
|
||||
* @param {Event} event - Browser event
|
||||
*/
|
||||
focus: (event: Event) => void;
|
||||
/**
|
||||
* Blur event
|
||||
* @param {Event} event - Browser event
|
||||
*/
|
||||
blur: (event: Event) => void;
|
||||
/**
|
||||
* Paste event
|
||||
* @param {Event} event - Browser event
|
||||
*/
|
||||
paste: (event: Event) => void;
|
||||
}
|
||||
|
||||
/**
|
||||
* Defines valid properties in InputOtp component.
|
||||
*/
|
||||
export interface InputOtpProps {
|
||||
/**
|
||||
* Specifies whether a inputotp should be checked or not.
|
||||
* @defaultValue false
|
||||
*/
|
||||
modelValue?: boolean | string | undefined;
|
||||
/**
|
||||
* Value in checked state.
|
||||
* @defaultValue true
|
||||
*/
|
||||
trueValue?: any;
|
||||
/**
|
||||
* Value in unchecked state.
|
||||
* @defaultValue false
|
||||
*/
|
||||
falseValue?: any;
|
||||
/**
|
||||
* When present, it specifies that the component should have invalid state style.
|
||||
* @defaultValue false
|
||||
|
@ -116,38 +155,38 @@ export interface InputSwitchProps {
|
|||
disabled?: boolean | undefined;
|
||||
/**
|
||||
* When present, it specifies that an input field is read-only.
|
||||
* @default false
|
||||
* @defaultValue false
|
||||
*/
|
||||
readonly?: boolean | undefined;
|
||||
/**
|
||||
* Specifies the input variant of the component.
|
||||
* @defaultValue outlined
|
||||
*/
|
||||
variant?: 'outlined' | 'filled' | undefined;
|
||||
/**
|
||||
* Index of the element in tabbing order.
|
||||
*/
|
||||
tabindex?: number | undefined;
|
||||
/**
|
||||
* Identifier of the underlying input element.
|
||||
* Number of characters to initiate.
|
||||
* @defaultValue 4
|
||||
*/
|
||||
inputId?: string | undefined;
|
||||
length?: number | undefined;
|
||||
/**
|
||||
* Style class of the input field.
|
||||
* Mask pattern.
|
||||
* @defaultValue false
|
||||
*/
|
||||
inputClass?: string | object | undefined;
|
||||
mask?: boolean | undefined;
|
||||
/**
|
||||
* Inline style of the input field.
|
||||
* When present, it specifies that an input field is integer-only.
|
||||
* @defaultValue false
|
||||
*/
|
||||
inputStyle?: object | undefined;
|
||||
/**
|
||||
* Establishes relationships between the component and label(s) where its value should be one or more element IDs.
|
||||
*/
|
||||
ariaLabelledby?: string | undefined;
|
||||
/**
|
||||
* Establishes a string value that labels the component.
|
||||
*/
|
||||
ariaLabel?: string | undefined;
|
||||
integerOnly?: boolean | undefined;
|
||||
/**
|
||||
* Used to pass attributes to DOM elements inside the component.
|
||||
* @type {InputSwitchPassThroughOptions}
|
||||
* @type {InputOtpPassThroughOptions}
|
||||
*/
|
||||
pt?: PassThrough<InputSwitchPassThroughOptions>;
|
||||
pt?: PassThrough<InputOtpPassThroughOptions>;
|
||||
/**
|
||||
* Used to configure passthrough(pt) options of the component.
|
||||
* @type {PassThroughOptions}
|
||||
|
@ -161,27 +200,36 @@ export interface InputSwitchProps {
|
|||
}
|
||||
|
||||
/**
|
||||
* Defines current options in InputSwitch component.
|
||||
*
|
||||
*/
|
||||
export interface InputSwitchContext {
|
||||
export interface InputOtpSlots {
|
||||
/**
|
||||
* Current checked state of the item as a boolean.
|
||||
* @defaultValue false
|
||||
* Default content slot.
|
||||
*/
|
||||
checked: boolean;
|
||||
default(scope: {
|
||||
/**
|
||||
* Current disabled state of the item as a boolean.
|
||||
* @defaultValue false
|
||||
* Events of the component
|
||||
* @param {number} index - Index of the input field
|
||||
* @return {InputOtpTemplateEvents}
|
||||
*/
|
||||
disabled: boolean;
|
||||
events: (index: number) => InputOtpTemplateEvents;
|
||||
/**
|
||||
* Attributes of the component
|
||||
* @param {number} index - Index of the input field
|
||||
* @return {InputOtpTemplateAttrsOptions}
|
||||
*/
|
||||
attrs: (index: number) => InputOtpTemplateAttrsOptions;
|
||||
/**
|
||||
* Index of the input field
|
||||
*/
|
||||
index: number;
|
||||
}): VNode[];
|
||||
}
|
||||
|
||||
export interface InputSwitchSlots {}
|
||||
|
||||
/**
|
||||
* Defines valid emits in InputSwitch component.
|
||||
* Defines valid emits in InputOtp component.
|
||||
*/
|
||||
export interface InputSwitchEmits {
|
||||
export interface InputOtpEmits {
|
||||
/**
|
||||
* Emitted when the value changes.
|
||||
* @param {boolean} value - New value.
|
||||
|
@ -205,23 +253,23 @@ export interface InputSwitchEmits {
|
|||
}
|
||||
|
||||
/**
|
||||
* **PrimeVue - InputSwitch**
|
||||
* **PrimeVue - InputOtp**
|
||||
*
|
||||
* _InputSwitch is used to select a boolean value._
|
||||
* _InputOtp is used to enter one time passwords._
|
||||
*
|
||||
* [Live Demo](https://www.primevue.org/inputswitch/)
|
||||
* [Live Demo](https://www.primevue.org/inputotp/)
|
||||
* --- ---
|
||||
* 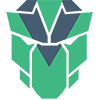
|
||||
*
|
||||
* @group Component
|
||||
*
|
||||
*/
|
||||
declare class InputSwitch extends ClassComponent<InputSwitchProps, InputSwitchSlots, InputSwitchEmits> {}
|
||||
declare class InputOtp extends ClassComponent<InputOtpProps, InputOtpSlots, InputOtpEmits> {}
|
||||
|
||||
declare module '@vue/runtime-core' {
|
||||
interface GlobalComponents {
|
||||
InputSwitch: GlobalComponentConstructor<InputSwitch>;
|
||||
InputOtp: GlobalComponentConstructor<InputOtp>;
|
||||
}
|
||||
}
|
||||
|
||||
export default InputSwitch;
|
||||
export default InputOtp;
|
||||
|
|
|
@ -12,6 +12,7 @@
|
|||
:disabled="disabled"
|
||||
:invalid="invalid"
|
||||
:tabindex="tabindex"
|
||||
:unstyled="unstyled"
|
||||
@input="onInput($event, i - 1)"
|
||||
@focus="onFocus($event)"
|
||||
@blur="onBlur($event)"
|
||||
|
|
|
@ -1,7 +1,7 @@
|
|||
import BaseStyle from 'primevue/base/style';
|
||||
|
||||
const classes = {
|
||||
root: ({ props }) => ['p-inputotp p-component'],
|
||||
root: 'p-inputotp p-component',
|
||||
input: 'p-inputotp-input'
|
||||
};
|
||||
|
||||
|
|
|
@ -3,6 +3,6 @@
|
|||
<p>{{ $attrs.description }}</p>
|
||||
</DocSectionText>
|
||||
<div class="card">
|
||||
<img class="w-full" src="https://primefaces.org/cdn/primevue/images/pt/inputswitch.jpg" />
|
||||
<img class="w-full" src="https://primefaces.org/cdn/primevue/images/pt/wireframe-placeholder.jpg" />
|
||||
</div>
|
||||
</template>
|
||||
|
|
|
@ -1,7 +1,7 @@
|
|||
<template>
|
||||
<div class="doc-main">
|
||||
<div class="doc-intro">
|
||||
<h1>InputSwitch Pass Through</h1>
|
||||
<h1>InputOtp Pass Through</h1>
|
||||
</div>
|
||||
<DocSections :docs="docs" />
|
||||
</div>
|
||||
|
@ -23,10 +23,10 @@ export default {
|
|||
component: PTImage
|
||||
},
|
||||
{
|
||||
id: 'pt.doc.inputswitch',
|
||||
label: 'InputSwitch PT Options',
|
||||
id: 'pt.doc.inputotp',
|
||||
label: 'InputOtp PT Options',
|
||||
component: DocApiTable,
|
||||
data: getPTOption('InputSwitch')
|
||||
data: getPTOption('InputOtp')
|
||||
}
|
||||
]
|
||||
};
|
||||
|
|
|
@ -12,16 +12,12 @@
|
|||
</thead>
|
||||
<tbody>
|
||||
<tr>
|
||||
<td>p-inputswitch</td>
|
||||
<td>p-inputotp</td>
|
||||
<td>Container element.</td>
|
||||
</tr>
|
||||
<tr>
|
||||
<td>p-inputswitch-checked</td>
|
||||
<td>Container element in active state.</td>
|
||||
</tr>
|
||||
<tr>
|
||||
<td>p-inputswitch-slider</td>
|
||||
<td>Slider element behind the handle.</td>
|
||||
<td>p-inputotp-input</td>
|
||||
<td>Input element of the component.</td>
|
||||
</tr>
|
||||
</tbody>
|
||||
</table>
|
||||
|
|
Loading…
Reference in New Issue