Refactor #5175 - FloatLabel
parent
0dd5d5cea3
commit
355bbebe7e
|
@ -75,102 +75,6 @@ const inputTextCSS = `
|
||||||
.p-fluid .p-inputtext {
|
.p-fluid .p-inputtext {
|
||||||
width: 100%;
|
width: 100%;
|
||||||
}
|
}
|
||||||
|
|
||||||
/* InputGroup */
|
|
||||||
.p-inputgroup {
|
|
||||||
display: flex;
|
|
||||||
align-items: stretch;
|
|
||||||
width: 100%;
|
|
||||||
}
|
|
||||||
|
|
||||||
.p-inputgroup-addon {
|
|
||||||
display: flex;
|
|
||||||
align-items: center;
|
|
||||||
justify-content: center;
|
|
||||||
}
|
|
||||||
|
|
||||||
.p-inputgroup .p-float-label {
|
|
||||||
display: flex;
|
|
||||||
align-items: stretch;
|
|
||||||
width: 100%;
|
|
||||||
}
|
|
||||||
|
|
||||||
.p-inputgroup .p-inputtext,
|
|
||||||
.p-fluid .p-inputgroup .p-inputtext,
|
|
||||||
.p-inputgroup .p-inputwrapper,
|
|
||||||
.p-fluid .p-inputgroup .p-input {
|
|
||||||
flex: 1 1 auto;
|
|
||||||
width: 1%;
|
|
||||||
}
|
|
||||||
|
|
||||||
/* Floating Label */
|
|
||||||
.p-float-label {
|
|
||||||
display: block;
|
|
||||||
position: relative;
|
|
||||||
}
|
|
||||||
|
|
||||||
.p-float-label label {
|
|
||||||
position: absolute;
|
|
||||||
pointer-events: none;
|
|
||||||
top: 50%;
|
|
||||||
margin-top: -.5rem;
|
|
||||||
transition-property: all;
|
|
||||||
transition-timing-function: ease;
|
|
||||||
line-height: 1;
|
|
||||||
}
|
|
||||||
|
|
||||||
.p-float-label textarea ~ label {
|
|
||||||
top: 1rem;
|
|
||||||
}
|
|
||||||
|
|
||||||
.p-float-label input:focus ~ label,
|
|
||||||
.p-float-label input.p-filled ~ label,
|
|
||||||
.p-float-label input:-webkit-autofill ~ label,
|
|
||||||
.p-float-label textarea:focus ~ label,
|
|
||||||
.p-float-label textarea.p-filled ~ label,
|
|
||||||
.p-float-label .p-inputwrapper-focus ~ label,
|
|
||||||
.p-float-label .p-inputwrapper-filled ~ label {
|
|
||||||
top: -.75rem;
|
|
||||||
font-size: 12px;
|
|
||||||
}
|
|
||||||
|
|
||||||
|
|
||||||
.p-float-label .p-placeholder,
|
|
||||||
.p-float-label input::placeholder,
|
|
||||||
.p-float-label .p-inputtext::placeholder {
|
|
||||||
opacity: 0;
|
|
||||||
transition-property: all;
|
|
||||||
transition-timing-function: ease;
|
|
||||||
}
|
|
||||||
|
|
||||||
.p-float-label .p-focus .p-placeholder,
|
|
||||||
.p-float-label input:focus::placeholder,
|
|
||||||
.p-float-label .p-inputtext:focus::placeholder {
|
|
||||||
opacity: 1;
|
|
||||||
transition-property: all;
|
|
||||||
transition-timing-function: ease;
|
|
||||||
}
|
|
||||||
|
|
||||||
.p-input-icon-left,
|
|
||||||
.p-input-icon-right {
|
|
||||||
position: relative;
|
|
||||||
display: inline-block;
|
|
||||||
}
|
|
||||||
|
|
||||||
.p-input-icon-left > i,
|
|
||||||
.p-input-icon-left > svg,
|
|
||||||
.p-input-icon-right > i,
|
|
||||||
.p-input-icon-right > svg {
|
|
||||||
position: absolute;
|
|
||||||
top: 50%;
|
|
||||||
margin-top: -.5rem;
|
|
||||||
}
|
|
||||||
|
|
||||||
.p-fluid .p-input-icon-left,
|
|
||||||
.p-fluid .p-input-icon-right {
|
|
||||||
display: block;
|
|
||||||
width: 100%;
|
|
||||||
}
|
|
||||||
`;
|
`;
|
||||||
|
|
||||||
const css = `
|
const css = `
|
||||||
|
|
|
@ -0,0 +1,16 @@
|
||||||
|
<script>
|
||||||
|
import BaseComponent from 'primevue/basecomponent';
|
||||||
|
import FloatLabelStyle from 'primevue/floatlabel/style';
|
||||||
|
|
||||||
|
export default {
|
||||||
|
name: 'BaseFloatLabel',
|
||||||
|
extends: BaseComponent,
|
||||||
|
props: {},
|
||||||
|
style: FloatLabelStyle,
|
||||||
|
provide() {
|
||||||
|
return {
|
||||||
|
$parentInstance: this
|
||||||
|
};
|
||||||
|
}
|
||||||
|
};
|
||||||
|
</script>
|
|
@ -0,0 +1,128 @@
|
||||||
|
/**
|
||||||
|
*
|
||||||
|
* FloatLabel is a grouping component with the optional content toggle feature.
|
||||||
|
*
|
||||||
|
* [Live Demo](https://www.primevue.org/inputtext/)
|
||||||
|
*
|
||||||
|
* @module floatlabel
|
||||||
|
*
|
||||||
|
*/
|
||||||
|
import { AnchorHTMLAttributes, TransitionProps, VNode } from 'vue';
|
||||||
|
import { ComponentHooks } from '../basecomponent';
|
||||||
|
import { PassThroughOptions } from '../passthrough';
|
||||||
|
import { ClassComponent, GlobalComponentConstructor, PassThrough } from '../ts-helpers';
|
||||||
|
|
||||||
|
export declare type FloatLabelPassThroughOptionType = FloatLabelPassThroughAttributes | ((options: FloatLabelPassThroughMethodOptions) => FloatLabelPassThroughAttributes | string) | string | null | undefined;
|
||||||
|
|
||||||
|
export declare type FloatLabelPassThroughTransitionType = TransitionProps | ((options: FloatLabelPassThroughMethodOptions) => TransitionProps) | undefined;
|
||||||
|
|
||||||
|
/**
|
||||||
|
* Custom passthrough(pt) option method.
|
||||||
|
*/
|
||||||
|
export interface FloatLabelPassThroughMethodOptions {
|
||||||
|
/**
|
||||||
|
* Defines instance.
|
||||||
|
*/
|
||||||
|
instance: any;
|
||||||
|
/**
|
||||||
|
* Defines valid properties.
|
||||||
|
*/
|
||||||
|
props: FloatLabelProps;
|
||||||
|
/**
|
||||||
|
* Defines valid attributes.
|
||||||
|
*/
|
||||||
|
attrs: any;
|
||||||
|
/**
|
||||||
|
* Defines parent options.
|
||||||
|
*/
|
||||||
|
parent: any;
|
||||||
|
/**
|
||||||
|
* Defines passthrough(pt) options in global config.
|
||||||
|
*/
|
||||||
|
global: object | undefined;
|
||||||
|
}
|
||||||
|
|
||||||
|
/**
|
||||||
|
* Custom passthrough(pt) options.
|
||||||
|
* @see {@link FloatLabelProps.pt}
|
||||||
|
*/
|
||||||
|
export interface FloatLabelPassThroughOptions {
|
||||||
|
/**
|
||||||
|
* Used to pass attributes to the root's DOM element.
|
||||||
|
*/
|
||||||
|
root?: FloatLabelPassThroughOptionType;
|
||||||
|
/**
|
||||||
|
* Used to manage all lifecycle hooks.
|
||||||
|
* @see {@link BaseComponent.ComponentHooks}
|
||||||
|
*/
|
||||||
|
hooks?: ComponentHooks;
|
||||||
|
/**
|
||||||
|
* Used to control Vue Transition API.
|
||||||
|
*/
|
||||||
|
transition?: FloatLabelPassThroughTransitionType;
|
||||||
|
}
|
||||||
|
|
||||||
|
/**
|
||||||
|
* Custom passthrough attributes for each DOM elements
|
||||||
|
*/
|
||||||
|
export interface FloatLabelPassThroughAttributes {
|
||||||
|
[key: string]: any;
|
||||||
|
}
|
||||||
|
|
||||||
|
/**
|
||||||
|
* Defines valid properties in FloatLabel component.
|
||||||
|
*/
|
||||||
|
export interface FloatLabelProps {
|
||||||
|
/**
|
||||||
|
* Used to pass attributes to DOM elements inside the component.
|
||||||
|
* @type {FloatLabelPassThroughOptions}
|
||||||
|
*/
|
||||||
|
pt?: PassThrough<FloatLabelPassThroughOptions>;
|
||||||
|
/**
|
||||||
|
* Used to configure passthrough(pt) options of the component.
|
||||||
|
* @type {PassThroughOptions}
|
||||||
|
*/
|
||||||
|
ptOptions?: PassThroughOptions;
|
||||||
|
/**
|
||||||
|
* When enabled, it removes component related styles in the core.
|
||||||
|
* @defaultValue false
|
||||||
|
*/
|
||||||
|
unstyled?: boolean;
|
||||||
|
}
|
||||||
|
|
||||||
|
/**
|
||||||
|
* Defines valid slots in FloatLabel component.
|
||||||
|
*/
|
||||||
|
export interface FloatLabelSlots {
|
||||||
|
/**
|
||||||
|
* Default content slot.
|
||||||
|
*/
|
||||||
|
default: () => VNode[];
|
||||||
|
}
|
||||||
|
|
||||||
|
/**
|
||||||
|
* Defines valid emits in FloatLabel component.
|
||||||
|
*/
|
||||||
|
export interface FloatLabelEmits {}
|
||||||
|
|
||||||
|
/**
|
||||||
|
* **PrimeVue - FloatLabel**
|
||||||
|
*
|
||||||
|
* _FloatLabel is a grouping component with the optional content toggle feature._
|
||||||
|
*
|
||||||
|
* [Live Demo](https://www.primevue.org/inputtext/)
|
||||||
|
* --- ---
|
||||||
|
* 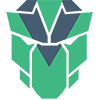
|
||||||
|
*
|
||||||
|
* @group Component
|
||||||
|
*
|
||||||
|
*/
|
||||||
|
declare class FloatLabel extends ClassComponent<FloatLabelProps, FloatLabelSlots, FloatLabelEmits> {}
|
||||||
|
|
||||||
|
declare module '@vue/runtime-core' {
|
||||||
|
interface GlobalComponents {
|
||||||
|
FloatLabel: GlobalComponentConstructor<FloatLabel>;
|
||||||
|
}
|
||||||
|
}
|
||||||
|
|
||||||
|
export default FloatLabel;
|
|
@ -0,0 +1,14 @@
|
||||||
|
<template>
|
||||||
|
<span :class="cx('root')" v-bind="ptm('root')" data-pc-name="floatlabel">
|
||||||
|
<slot />
|
||||||
|
</span>
|
||||||
|
</template>
|
||||||
|
|
||||||
|
<script>
|
||||||
|
import BaseFloatLabel from './BaseFloatLabel.vue';
|
||||||
|
|
||||||
|
export default {
|
||||||
|
name: 'FloatLabel',
|
||||||
|
extends: BaseFloatLabel
|
||||||
|
};
|
||||||
|
</script>
|
|
@ -0,0 +1,9 @@
|
||||||
|
{
|
||||||
|
"main": "./floatlabel.cjs.js",
|
||||||
|
"module": "./floatlabel.esm.js",
|
||||||
|
"unpkg": "./floatlabel.min.js",
|
||||||
|
"types": "./FloatLabel.d.ts",
|
||||||
|
"browser": {
|
||||||
|
"./sfc": "./FloatLabel.vue"
|
||||||
|
}
|
||||||
|
}
|
|
@ -0,0 +1,3 @@
|
||||||
|
import { BaseStyle } from '../../base/style';
|
||||||
|
|
||||||
|
export interface FloatLabelStyle extends BaseStyle {}
|
|
@ -0,0 +1,59 @@
|
||||||
|
import BaseStyle from 'primevue/base/style';
|
||||||
|
|
||||||
|
const css = `
|
||||||
|
.p-float-label {
|
||||||
|
display: block;
|
||||||
|
position: relative;
|
||||||
|
}
|
||||||
|
|
||||||
|
.p-float-label label {
|
||||||
|
position: absolute;
|
||||||
|
pointer-events: none;
|
||||||
|
top: 50%;
|
||||||
|
margin-top: -.5rem;
|
||||||
|
transition-property: all;
|
||||||
|
transition-timing-function: ease;
|
||||||
|
line-height: 1;
|
||||||
|
}
|
||||||
|
|
||||||
|
.p-float-label:has(textarea) label {
|
||||||
|
top: 1rem;
|
||||||
|
}
|
||||||
|
|
||||||
|
.p-float-label:has(input:focus) label,
|
||||||
|
.p-float-label:has(input.p-filled) label,
|
||||||
|
.p-float-label:has(input:-webkit-autofill) label,
|
||||||
|
.p-float-label:has(textarea:focus) label,
|
||||||
|
.p-float-label:has(textarea.p-filled) label,
|
||||||
|
.p-float-label:has(.p-inputwrapper-focus) label,
|
||||||
|
.p-float-label:has(.p-inputwrapper-filled) label {
|
||||||
|
top: -.75rem;
|
||||||
|
font-size: 12px;
|
||||||
|
}
|
||||||
|
|
||||||
|
.p-float-label .p-placeholder,
|
||||||
|
.p-float-label input::placeholder,
|
||||||
|
.p-float-label .p-inputtext::placeholder {
|
||||||
|
opacity: 0;
|
||||||
|
transition-property: all;
|
||||||
|
transition-timing-function: ease;
|
||||||
|
}
|
||||||
|
|
||||||
|
.p-float-label .p-focus .p-placeholder,
|
||||||
|
.p-float-label input:focus::placeholder,
|
||||||
|
.p-float-label .p-inputtext:focus::placeholder {
|
||||||
|
opacity: 1;
|
||||||
|
transition-property: all;
|
||||||
|
transition-timing-function: ease;
|
||||||
|
}
|
||||||
|
`;
|
||||||
|
|
||||||
|
const classes = {
|
||||||
|
root: 'p-float-label'
|
||||||
|
};
|
||||||
|
|
||||||
|
export default BaseStyle.extend({
|
||||||
|
name: 'floatlabel',
|
||||||
|
css,
|
||||||
|
classes
|
||||||
|
});
|
|
@ -0,0 +1,6 @@
|
||||||
|
{
|
||||||
|
"main": "./floatlabelstyle.cjs.js",
|
||||||
|
"module": "./floatlabelstyle.esm.js",
|
||||||
|
"unpkg": "./floatlabelstyle.min.js",
|
||||||
|
"types": "./FloatLabelStyle.d.ts"
|
||||||
|
}
|
|
@ -7,8 +7,11 @@ const form = [
|
||||||
'ColorPicker',
|
'ColorPicker',
|
||||||
'Dropdown',
|
'Dropdown',
|
||||||
'Editor',
|
'Editor',
|
||||||
|
'FloatLabel',
|
||||||
|
'IconField',
|
||||||
'InputGroup',
|
'InputGroup',
|
||||||
'InputGroupAddon',
|
'InputGroupAddon',
|
||||||
|
'InputIcon',
|
||||||
'InputMask',
|
'InputMask',
|
||||||
'InputNumber',
|
'InputNumber',
|
||||||
'InputSwitch',
|
'InputSwitch',
|
||||||
|
|
|
@ -42,14 +42,17 @@ const STYLE_ALIAS = {
|
||||||
'primevue/dynamicdialog/style': path.resolve(__dirname, './components/lib/dynamicdialog/style/DynamicDialogStyle.js'),
|
'primevue/dynamicdialog/style': path.resolve(__dirname, './components/lib/dynamicdialog/style/DynamicDialogStyle.js'),
|
||||||
'primevue/editor/style': path.resolve(__dirname, './components/lib/editor/style/EditorStyle.js'),
|
'primevue/editor/style': path.resolve(__dirname, './components/lib/editor/style/EditorStyle.js'),
|
||||||
'primevue/fieldset/style': path.resolve(__dirname, './components/lib/fieldset/style/FieldsetStyle.js'),
|
'primevue/fieldset/style': path.resolve(__dirname, './components/lib/fieldset/style/FieldsetStyle.js'),
|
||||||
|
'primevue/floatlabel/style': path.resolve(__dirname, './components/lib/floatlabel/style/FloatLabelStyle.js'),
|
||||||
'primevue/fileupload/style': path.resolve(__dirname, './components/lib/fileupload/style/FileUploadStyle.js'),
|
'primevue/fileupload/style': path.resolve(__dirname, './components/lib/fileupload/style/FileUploadStyle.js'),
|
||||||
'primevue/focustrap/style': path.resolve(__dirname, './components/lib/focustrap/style/FocusTrapStyle.js'),
|
'primevue/focustrap/style': path.resolve(__dirname, './components/lib/focustrap/style/FocusTrapStyle.js'),
|
||||||
'primevue/galleria/style': path.resolve(__dirname, './components/lib/galleria/style/GalleriaStyle.js'),
|
'primevue/galleria/style': path.resolve(__dirname, './components/lib/galleria/style/GalleriaStyle.js'),
|
||||||
'primevue/image/style': path.resolve(__dirname, './components/lib/image/style/ImageStyle.js'),
|
'primevue/image/style': path.resolve(__dirname, './components/lib/image/style/ImageStyle.js'),
|
||||||
|
'primevue/iconfield/style': path.resolve(__dirname, './components/lib/iconfield/style/IconFieldStyle.js'),
|
||||||
'primevue/inlinemessage/style': path.resolve(__dirname, './components/lib/inlinemessage/style/InlineMessageStyle.js'),
|
'primevue/inlinemessage/style': path.resolve(__dirname, './components/lib/inlinemessage/style/InlineMessageStyle.js'),
|
||||||
'primevue/inplace/style': path.resolve(__dirname, './components/lib/inplace/style/InplaceStyle.js'),
|
'primevue/inplace/style': path.resolve(__dirname, './components/lib/inplace/style/InplaceStyle.js'),
|
||||||
'primevue/inputgroup/style': path.resolve(__dirname, './components/lib/inputgroup/style/InputGroupStyle.js'),
|
'primevue/inputgroup/style': path.resolve(__dirname, './components/lib/inputgroup/style/InputGroupStyle.js'),
|
||||||
'primevue/inputgroupaddon/style': path.resolve(__dirname, './components/lib/inputgroupaddon/style/InputGroupAddonStyle.js'),
|
'primevue/inputgroupaddon/style': path.resolve(__dirname, './components/lib/inputgroupaddon/style/InputGroupAddonStyle.js'),
|
||||||
|
'primevue/inputicon/style': path.resolve(__dirname, './components/lib/inputicon/style/InputIconStyle.js'),
|
||||||
'primevue/inputmask/style': path.resolve(__dirname, './components/lib/inputmask/style/InputMaskStyle.js'),
|
'primevue/inputmask/style': path.resolve(__dirname, './components/lib/inputmask/style/InputMaskStyle.js'),
|
||||||
'primevue/inputnumber/style': path.resolve(__dirname, './components/lib/inputnumber/style/InputNumberStyle.js'),
|
'primevue/inputnumber/style': path.resolve(__dirname, './components/lib/inputnumber/style/InputNumberStyle.js'),
|
||||||
'primevue/inputswitch/style': path.resolve(__dirname, './components/lib/inputswitch/style/InputSwitchStyle.js'),
|
'primevue/inputswitch/style': path.resolve(__dirname, './components/lib/inputswitch/style/InputSwitchStyle.js'),
|
||||||
|
|
Loading…
Reference in New Issue