Calendar d.ts updated
parent
bc0f312772
commit
38f1eef021
|
@ -1,18 +1,18 @@
|
||||||
|
/**
|
||||||
|
*
|
||||||
|
* Calendar also known as DatePicker, is a form component to work with dates.
|
||||||
|
*
|
||||||
|
* [Live Demo](https://www.primevue.org/calendar/)
|
||||||
|
*
|
||||||
|
* @module calendar
|
||||||
|
*
|
||||||
|
*/
|
||||||
import { HTMLAttributes, InputHTMLAttributes, VNode } from 'vue';
|
import { HTMLAttributes, InputHTMLAttributes, VNode } from 'vue';
|
||||||
import { ClassComponent, GlobalComponentConstructor } from '../ts-helpers';
|
import { ClassComponent, GlobalComponentConstructor } from '../ts-helpers';
|
||||||
|
|
||||||
type CalendarValueType = string | Date | string[] | Date[] | undefined;
|
/**
|
||||||
|
* Custom Calendar responsive options metadata.
|
||||||
type CalendarSlotDateType = { day: number; month: number; year: number; today: boolean; selectable: boolean };
|
*/
|
||||||
|
|
||||||
type CalendarSelectionModeType = 'single' | 'multiple' | 'range' | undefined;
|
|
||||||
|
|
||||||
type CalendarViewType = 'date' | 'month' | 'year' | undefined;
|
|
||||||
|
|
||||||
type CalendarHourFormatType = '12' | '24' | undefined;
|
|
||||||
|
|
||||||
type CalendarAppendToType = 'body' | 'self' | string | undefined | HTMLElement;
|
|
||||||
|
|
||||||
export interface CalendarResponsiveOptions {
|
export interface CalendarResponsiveOptions {
|
||||||
/**
|
/**
|
||||||
* Breakpoint for responsive mode. Exp; @media screen and (max-width: ${breakpoint}) {...}
|
* Breakpoint for responsive mode. Exp; @media screen and (max-width: ${breakpoint}) {...}
|
||||||
|
@ -24,6 +24,9 @@ export interface CalendarResponsiveOptions {
|
||||||
numMonths: number;
|
numMonths: number;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
/**
|
||||||
|
* Custom Calendar month change event.
|
||||||
|
*/
|
||||||
export interface CalendarMonthChangeEvent {
|
export interface CalendarMonthChangeEvent {
|
||||||
/**
|
/**
|
||||||
* New month.
|
* New month.
|
||||||
|
@ -35,6 +38,9 @@ export interface CalendarMonthChangeEvent {
|
||||||
year: number;
|
year: number;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
/**
|
||||||
|
* Custom Calendar year change event.
|
||||||
|
*/
|
||||||
export interface CalendarYearChangeEvent {
|
export interface CalendarYearChangeEvent {
|
||||||
/**
|
/**
|
||||||
* New month.
|
* New month.
|
||||||
|
@ -46,6 +52,9 @@ export interface CalendarYearChangeEvent {
|
||||||
year: number;
|
year: number;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
/**
|
||||||
|
* Custom Calendar blur event.
|
||||||
|
*/
|
||||||
export interface CalendarBlurEvent {
|
export interface CalendarBlurEvent {
|
||||||
/**
|
/**
|
||||||
* Browser event
|
* Browser event
|
||||||
|
@ -57,99 +66,107 @@ export interface CalendarBlurEvent {
|
||||||
value: string;
|
value: string;
|
||||||
}
|
}
|
||||||
|
|
||||||
|
/**
|
||||||
|
* Defines valid properties in Calendar component.
|
||||||
|
*/
|
||||||
export interface CalendarProps {
|
export interface CalendarProps {
|
||||||
/**
|
/**
|
||||||
* Value of the component.
|
* Value of the component.
|
||||||
|
* @defaultValue null
|
||||||
*/
|
*/
|
||||||
modelValue?: CalendarValueType;
|
modelValue?: string | Date | string[] | Date[] | undefined | null;
|
||||||
/**
|
/**
|
||||||
* Defines the quantity of the selection, valid values are 'single', 'multiple' and 'range'.
|
* Defines the quantity of the selection.
|
||||||
* @see CalendarSelectionModeType
|
* @defaultValue single
|
||||||
* Default value is 'single'.
|
|
||||||
*/
|
*/
|
||||||
selectionMode?: CalendarSelectionModeType;
|
selectionMode?: 'single' | 'multiple' | 'range' | undefined;
|
||||||
/**
|
/**
|
||||||
* Format of the date. Defaults to PrimeVue Locale configuration.
|
* Format of the date. Defaults to PrimeVue Locale configuration.
|
||||||
*/
|
*/
|
||||||
dateFormat?: string | undefined;
|
dateFormat?: string | undefined;
|
||||||
/**
|
/**
|
||||||
* When enabled, displays the calendar as inline instead of an overlay.
|
* When enabled, displays the calendar as inline instead of an overlay.
|
||||||
|
* @defaultValue false
|
||||||
*/
|
*/
|
||||||
inline?: boolean | undefined;
|
inline?: boolean | undefined;
|
||||||
/**
|
/**
|
||||||
* Whether to display dates in other months (non-selectable) at the start or end of the current month. To make these days selectable use the selectOtherMonths option.
|
* Whether to display dates in other months (non-selectable) at the start or end of the current month. To make these days selectable use the selectOtherMonths option.
|
||||||
* Default value is true.
|
* @defaultValue true
|
||||||
*/
|
*/
|
||||||
showOtherMonths?: boolean | undefined;
|
showOtherMonths?: boolean | undefined;
|
||||||
/**
|
/**
|
||||||
* Whether days in other months shown before or after the current month are selectable. This only applies if the showOtherMonths option is set to true.
|
* Whether days in other months shown before or after the current month are selectable. This only applies if the showOtherMonths option is set to true.
|
||||||
|
* @defaultValue false
|
||||||
*/
|
*/
|
||||||
selectOtherMonths?: boolean | undefined;
|
selectOtherMonths?: boolean | undefined;
|
||||||
/**
|
/**
|
||||||
* When enabled, displays a button with icon next to input.
|
* When enabled, displays a button with icon next to input.
|
||||||
|
* @defaultValue false
|
||||||
*/
|
*/
|
||||||
showIcon?: boolean | undefined;
|
showIcon?: boolean | undefined;
|
||||||
/**
|
/**
|
||||||
* Icon of the calendar button.
|
* Icon of the calendar button.
|
||||||
* Default value is 'pi pi-calendar'.
|
* @defaultValue pi pi-calendar
|
||||||
*/
|
*/
|
||||||
icon?: string | undefined;
|
icon?: string | undefined;
|
||||||
/**
|
/**
|
||||||
* Icon to show in the previous button.
|
* Icon to show in the previous button.
|
||||||
* Default value is 'pi pi-chevron-left'.
|
* @defaultValue pi pi-chevron-left
|
||||||
*/
|
*/
|
||||||
previousIcon?: string | undefined;
|
previousIcon?: string | undefined;
|
||||||
/**
|
/**
|
||||||
* Icon to show in the next button.
|
* Icon to show in the next button.
|
||||||
* Default value is 'pi pi-chevron-right'.
|
* @defaultValue pi pi-chevron-right
|
||||||
*/
|
*/
|
||||||
nextIcon?: string | undefined;
|
nextIcon?: string | undefined;
|
||||||
/**
|
/**
|
||||||
* Icon to show in each of the increment buttons.
|
* Icon to show in each of the increment buttons.
|
||||||
* Default value is 'pi pi-chevron-up'.
|
* @defaultValue pi pi-chevron-up
|
||||||
*/
|
*/
|
||||||
incrementIcon?: string | undefined;
|
incrementIcon?: string | undefined;
|
||||||
/**
|
/**
|
||||||
* Icon to show in each of the decrement buttons.
|
* Icon to show in each of the decrement buttons.
|
||||||
* Default value is 'pi pi-chevron-down'.
|
* @defaultValue pi pi-chevron-down
|
||||||
*/
|
*/
|
||||||
decrementIcon?: string | undefined;
|
decrementIcon?: string | undefined;
|
||||||
/**
|
/**
|
||||||
* Number of months to display.
|
* Number of months to display.
|
||||||
* Default value is 1.
|
* @defaultValue 1
|
||||||
*/
|
*/
|
||||||
numberOfMonths?: number | undefined;
|
numberOfMonths?: number | undefined;
|
||||||
/**
|
/**
|
||||||
* An array of options for responsive design.
|
* An array of options for responsive design.
|
||||||
* @see CalendarResponsiveOptions
|
|
||||||
*/
|
*/
|
||||||
responsiveOptions?: CalendarResponsiveOptions[];
|
responsiveOptions?: CalendarResponsiveOptions[];
|
||||||
/**
|
/**
|
||||||
* Type of view to display, valid values are 'date', 'month' and 'year'.
|
* Type of view to display.
|
||||||
* @see CalendarViewType
|
* @defaultValue date
|
||||||
* Default value is 'date'.
|
|
||||||
*/
|
*/
|
||||||
view?: CalendarViewType;
|
view?: 'date' | 'month' | 'year' | undefined;
|
||||||
/**
|
/**
|
||||||
* When enabled, calendar overlay is displayed as optimized for touch devices.
|
* When enabled, calendar overlay is displayed as optimized for touch devices.
|
||||||
|
* @defaultValue false
|
||||||
*/
|
*/
|
||||||
touchUI?: boolean | undefined;
|
touchUI?: boolean | undefined;
|
||||||
/**
|
/**
|
||||||
* Whether the month should be rendered as a dropdown instead of text.
|
* Whether the month should be rendered as a dropdown instead of text.
|
||||||
*
|
*
|
||||||
* @deprecated since version 3.9.0, Navigator is always on.
|
* @deprecated since version 3.9.0, Navigator is always on.
|
||||||
|
* @defaultValue false
|
||||||
*/
|
*/
|
||||||
monthNavigator?: boolean | undefined;
|
monthNavigator?: boolean | undefined;
|
||||||
/**
|
/**
|
||||||
* Whether the year should be rendered as a dropdown instead of text.
|
* Whether the year should be rendered as a dropdown instead of text.
|
||||||
*
|
*
|
||||||
* @deprecated since version 3.9.0, Navigator is always on.
|
* @deprecated since version 3.9.0, Navigator is always on.
|
||||||
|
* @defaultValue false
|
||||||
*/
|
*/
|
||||||
yearNavigator?: boolean | undefined;
|
yearNavigator?: boolean | undefined;
|
||||||
/**
|
/**
|
||||||
* The range of years displayed in the year drop-down in (nnnn:nnnn) format such as (2000:2020).
|
* The range of years displayed in the year drop-down in (nnnn:nnnn) format such as (2000:2020).
|
||||||
*
|
*
|
||||||
* @deprecated since version 3.9.0, Years are based on decades by default.
|
* @deprecated since version 3.9.0, Years are based on decades by default.
|
||||||
|
* @defaultValue false
|
||||||
*/
|
*/
|
||||||
yearRange?: string | undefined;
|
yearRange?: string | undefined;
|
||||||
/**
|
/**
|
||||||
|
@ -174,87 +191,97 @@ export interface CalendarProps {
|
||||||
maxDateCount?: number | undefined;
|
maxDateCount?: number | undefined;
|
||||||
/**
|
/**
|
||||||
* When disabled, datepicker will not be visible with input focus.
|
* When disabled, datepicker will not be visible with input focus.
|
||||||
* Default value is true.
|
* @defaultValue true
|
||||||
*/
|
*/
|
||||||
showOnFocus?: boolean | undefined;
|
showOnFocus?: boolean | undefined;
|
||||||
/**
|
/**
|
||||||
* Whether to automatically manage layering.
|
* Whether to automatically manage layering.
|
||||||
* Default value is true.
|
* @defaultValue true
|
||||||
*/
|
*/
|
||||||
autoZIndex?: boolean | undefined;
|
autoZIndex?: boolean | undefined;
|
||||||
/**
|
/**
|
||||||
* Base zIndex value to use in layering.
|
* Base zIndex value to use in layering.
|
||||||
* Default value is 0.
|
* @defaultValue 0
|
||||||
*/
|
*/
|
||||||
baseZIndex?: number | undefined;
|
baseZIndex?: number | undefined;
|
||||||
/**
|
/**
|
||||||
* Whether to display today and clear buttons at the footer.
|
* Whether to display today and clear buttons at the footer.
|
||||||
|
* @defaultValue false
|
||||||
*/
|
*/
|
||||||
showButtonBar?: boolean | undefined;
|
showButtonBar?: boolean | undefined;
|
||||||
/**
|
/**
|
||||||
* The cutoff year for determining the century for a date.
|
* The cutoff year for determining the century for a date.
|
||||||
* Default value is +10.
|
* @defaultValue +10
|
||||||
*/
|
*/
|
||||||
shortYearCutoff?: string | undefined;
|
shortYearCutoff?: string | undefined;
|
||||||
/**
|
/**
|
||||||
* Whether to display timepicker.
|
* Whether to display timepicker.
|
||||||
|
* @defaultValue false
|
||||||
*/
|
*/
|
||||||
showTime?: boolean | undefined;
|
showTime?: boolean | undefined;
|
||||||
/**
|
/**
|
||||||
* Whether to display timepicker only.
|
* Whether to display timepicker only.
|
||||||
|
* @defaultValue false
|
||||||
*/
|
*/
|
||||||
timeOnly?: boolean | undefined;
|
timeOnly?: boolean | undefined;
|
||||||
/**
|
/**
|
||||||
* Specifies 12 or 24 hour format.
|
* Specifies hour format.
|
||||||
|
* @defaultValue 24
|
||||||
*/
|
*/
|
||||||
hourFormat?: CalendarHourFormatType;
|
hourFormat?: '12' | '24' | undefined;
|
||||||
/**
|
/**
|
||||||
* Hours to change per step.
|
* Hours to change per step.
|
||||||
* Default value is 1.
|
* @defaultValue 1
|
||||||
*/
|
*/
|
||||||
stepHour?: number | undefined;
|
stepHour?: number | undefined;
|
||||||
/**
|
/**
|
||||||
* Minutes to change per step.
|
* Minutes to change per step.
|
||||||
* Default value is 1.
|
* @defaultValue 1
|
||||||
*/
|
*/
|
||||||
stepMinute?: number | undefined;
|
stepMinute?: number | undefined;
|
||||||
/**
|
/**
|
||||||
* Seconds to change per step.
|
* Seconds to change per step.
|
||||||
* Default value is 1.
|
* @defaultValue 1
|
||||||
*/
|
*/
|
||||||
stepSecond?: number | undefined;
|
stepSecond?: number | undefined;
|
||||||
/**
|
/**
|
||||||
* Whether to show the seconds in time picker.
|
* Whether to show the seconds in time picker.
|
||||||
|
* @defaultValue false
|
||||||
*/
|
*/
|
||||||
showSeconds?: boolean | undefined;
|
showSeconds?: boolean | undefined;
|
||||||
/**
|
/**
|
||||||
* Whether to hide the overlay on date selection when showTime is enabled.
|
* Whether to hide the overlay on date selection when showTime is enabled.
|
||||||
|
* @defaultValue false
|
||||||
*/
|
*/
|
||||||
hideOnDateTimeSelect?: boolean | undefined;
|
hideOnDateTimeSelect?: boolean | undefined;
|
||||||
/**
|
/**
|
||||||
* Whether to hide the overlay on date selection is completed when selectionMode is range.
|
* Whether to hide the overlay on date selection is completed when selectionMode is range.
|
||||||
|
* @defaultValue false
|
||||||
*/
|
*/
|
||||||
hideOnRangeSelection?: boolean | undefined;
|
hideOnRangeSelection?: boolean | undefined;
|
||||||
/**
|
/**
|
||||||
* Separator of time selector.
|
* Separator of time selector.
|
||||||
* Default value is ':'.
|
* @defaultValue :
|
||||||
*/
|
*/
|
||||||
timeSeparator?: string | undefined;
|
timeSeparator?: string | undefined;
|
||||||
/**
|
/**
|
||||||
* When enabled, calendar will show week numbers.
|
* When enabled, calendar will show week numbers.
|
||||||
|
* @defaultValue false
|
||||||
*/
|
*/
|
||||||
showWeek?: boolean | undefined;
|
showWeek?: boolean | undefined;
|
||||||
/**
|
/**
|
||||||
* Wheter to allow prevents entering the date manually via typing.
|
* Wheter to allow prevents entering the date manually via typing.
|
||||||
* Default value is true.
|
* @defaultValue true
|
||||||
*/
|
*/
|
||||||
manualInput?: boolean | undefined;
|
manualInput?: boolean | undefined;
|
||||||
/**
|
/**
|
||||||
* When present, it specifies that the component should be disabled.
|
* When present, it specifies that the component should be disabled.
|
||||||
|
* @defaultValue false
|
||||||
*/
|
*/
|
||||||
disabled?: boolean | undefined;
|
disabled?: boolean | undefined;
|
||||||
/**
|
/**
|
||||||
* When present, it specifies that an input field is read-only.
|
* When present, it specifies that an input field is read-only.
|
||||||
|
* @defaultValue false
|
||||||
*/
|
*/
|
||||||
readonly?: boolean | undefined;
|
readonly?: boolean | undefined;
|
||||||
/**
|
/**
|
||||||
|
@ -262,11 +289,10 @@ export interface CalendarProps {
|
||||||
*/
|
*/
|
||||||
placeholder?: string | undefined;
|
placeholder?: string | undefined;
|
||||||
/**
|
/**
|
||||||
* A valid query selector or an HTMLElement to specify where the overlay gets attached. Special keywords are 'body' for document body and 'self' for the element itself.
|
* A valid query selector or an HTMLElement to specify where the overlay gets attached.
|
||||||
* @see CalendarAppendToType
|
* @defaultValue body
|
||||||
* Default value is 'body'.
|
|
||||||
*/
|
*/
|
||||||
appendTo?: CalendarAppendToType;
|
appendTo?: 'body' | 'self' | string | undefined | HTMLElement;
|
||||||
/**
|
/**
|
||||||
* Identifier of the element.
|
* Identifier of the element.
|
||||||
*/
|
*/
|
||||||
|
@ -309,61 +335,65 @@ export interface CalendarProps {
|
||||||
'aria-label'?: string | undefined;
|
'aria-label'?: string | undefined;
|
||||||
}
|
}
|
||||||
|
|
||||||
export interface CalendarSlots {
|
/**
|
||||||
|
* Defines valid slots in Calendar component.
|
||||||
|
*/
|
||||||
|
export declare type CalendarSlots = {
|
||||||
/**
|
/**
|
||||||
* Custom header template of panel.
|
* Custom header template of panel.
|
||||||
*/
|
*/
|
||||||
header: () => VNode[];
|
header(): VNode[];
|
||||||
/**
|
/**
|
||||||
* Custom footer template of panel.
|
* Custom footer template of panel.
|
||||||
*/
|
*/
|
||||||
footer: () => VNode[];
|
footer(): VNode[];
|
||||||
/**
|
/**
|
||||||
* Custom date template.
|
* Custom date template.
|
||||||
* @param {Object} scope - date slot's params.
|
|
||||||
*/
|
*/
|
||||||
date: (scope: {
|
date(scope: {
|
||||||
/**
|
/**
|
||||||
* Value of the component.
|
* Value of the component.
|
||||||
*/
|
*/
|
||||||
date: CalendarSlotDateType;
|
date: { day: number; month: number; year: number; today: boolean; selectable: boolean };
|
||||||
}) => VNode[];
|
}): VNode[];
|
||||||
/**
|
/**
|
||||||
* Custom decade template.
|
* Custom decade template.
|
||||||
* @param {CalendarDecadeSlot} scope - decade slot's params.
|
|
||||||
*/
|
*/
|
||||||
decade: (scope: {
|
decade(scope: {
|
||||||
/**
|
/**
|
||||||
* An array containing the start and and year of a decade to display at header of the year picker.
|
* An array containing the start and and year of a decade to display at header of the year picker.
|
||||||
*/
|
*/
|
||||||
years: string[] | undefined;
|
years: string[] | undefined;
|
||||||
}) => VNode[];
|
}): VNode[];
|
||||||
}
|
};
|
||||||
|
|
||||||
export declare type CalendarEmits = {
|
/**
|
||||||
|
* Defines valid emits in Calendar component.
|
||||||
|
*/
|
||||||
|
export interface CalendarEmits {
|
||||||
/**
|
/**
|
||||||
* Emitted when the value changes.
|
* Emitted when the value changes.
|
||||||
* @param {CalendarValueType} value - New value.
|
* @param {string | Date | string[] | Date[] | undefined} value - New value.
|
||||||
*/
|
*/
|
||||||
'update:modelValue': (value: CalendarValueType) => void;
|
'update:modelValue'(value: string | Date | string[] | Date[] | undefined): void;
|
||||||
/**
|
/**
|
||||||
* Callback to invoke when input field is being typed.
|
* Callback to invoke when input field is being typed.
|
||||||
* @param {Event} event - Browser event
|
* @param {Event} event - Browser event
|
||||||
*/
|
*/
|
||||||
input: (event: Event) => void;
|
input(event: Event): void;
|
||||||
/**
|
/**
|
||||||
* Callback to invoke when a date is selected.
|
* Callback to invoke when a date is selected.
|
||||||
* @param {Date} value - Selected value.
|
* @param {Date} value - Selected value.
|
||||||
*/
|
*/
|
||||||
'date-select': (value: Date) => void;
|
'date-select'(value: Date): void;
|
||||||
/**
|
/**
|
||||||
* Callback to invoke when datepicker panel is shown.
|
* Callback to invoke when datepicker panel is shown.
|
||||||
*/
|
*/
|
||||||
show: () => void;
|
show(): void;
|
||||||
/**
|
/**
|
||||||
* Callback to invoke when datepicker panel is hidden.
|
* Callback to invoke when datepicker panel is hidden.
|
||||||
*/
|
*/
|
||||||
hide: () => void;
|
hide(): void;
|
||||||
/**
|
/**
|
||||||
* Callback to invoke when today button is clicked.
|
* Callback to invoke when today button is clicked.
|
||||||
* @param {Date} date - Today as a date instance.
|
* @param {Date} date - Today as a date instance.
|
||||||
|
@ -398,9 +428,20 @@ export declare type CalendarEmits = {
|
||||||
* Callback to invoke when a key is pressed.
|
* Callback to invoke when a key is pressed.
|
||||||
*/
|
*/
|
||||||
keydown: (event: Event) => void;
|
keydown: (event: Event) => void;
|
||||||
};
|
}
|
||||||
|
|
||||||
declare class Calendar extends ClassComponent<CalendarProps, CalendarSlots, CalendarEmits> {}
|
/**
|
||||||
|
* **PrimeVue - Calendar**
|
||||||
|
*
|
||||||
|
* _Calendar also known as DatePicker, is a form component to work with dates._
|
||||||
|
*
|
||||||
|
* [Live Demo](https://www.primevue.org/calendar/)
|
||||||
|
* --- ---
|
||||||
|
* 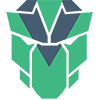
|
||||||
|
*
|
||||||
|
* @group Component
|
||||||
|
*/
|
||||||
|
export declare class Calendar extends ClassComponent<CalendarProps, CalendarSlots, CalendarEmits> {}
|
||||||
|
|
||||||
declare module '@vue/runtime-core' {
|
declare module '@vue/runtime-core' {
|
||||||
interface GlobalComponents {
|
interface GlobalComponents {
|
||||||
|
@ -408,13 +449,4 @@ declare module '@vue/runtime-core' {
|
||||||
}
|
}
|
||||||
}
|
}
|
||||||
|
|
||||||
/**
|
|
||||||
*
|
|
||||||
* Calendar is an input component to select a date.
|
|
||||||
*
|
|
||||||
* Demos:
|
|
||||||
*
|
|
||||||
* - [Calendar](https://www.primefaces.org/primevue/calendar)
|
|
||||||
*
|
|
||||||
*/
|
|
||||||
export default Calendar;
|
export default Calendar;
|
||||||
|
|
|
@ -3349,7 +3349,17 @@
|
||||||
}
|
}
|
||||||
}
|
}
|
||||||
},
|
},
|
||||||
"calendar/Calendar": {
|
"calendar": {
|
||||||
|
"description": "Calendar also known as DatePicker, is a form component to work with dates.\n\n[Live Demo](https://www.primevue.org/calendar/)",
|
||||||
|
"components": {
|
||||||
|
"Calendar": {
|
||||||
|
"description": "Calendar also known as DatePicker, is a form component to work with dates.",
|
||||||
|
"methods": {
|
||||||
|
"description": "Defines methods that can be accessed by the component's reference.",
|
||||||
|
"values": []
|
||||||
|
}
|
||||||
|
}
|
||||||
|
},
|
||||||
"interfaces": {
|
"interfaces": {
|
||||||
"description": "Defines the custom interfaces used by the module.",
|
"description": "Defines the custom interfaces used by the module.",
|
||||||
"eventDescription": "Defines the custom events used by the component's emit.",
|
"eventDescription": "Defines the custom events used by the component's emit.",
|
||||||
|
@ -3359,6 +3369,7 @@
|
||||||
"slotDescription": "Defines the slots used by the component.",
|
"slotDescription": "Defines the slots used by the component.",
|
||||||
"values": {
|
"values": {
|
||||||
"CalendarBlurEvent": {
|
"CalendarBlurEvent": {
|
||||||
|
"description": "Custom Calendar blur event.",
|
||||||
"relatedProp": "",
|
"relatedProp": "",
|
||||||
"props": [
|
"props": [
|
||||||
{
|
{
|
||||||
|
@ -3380,7 +3391,116 @@
|
||||||
],
|
],
|
||||||
"methods": []
|
"methods": []
|
||||||
},
|
},
|
||||||
|
"CalendarEmits": {
|
||||||
|
"description": "Defines valid emits in Calendar component.",
|
||||||
|
"relatedProp": "",
|
||||||
|
"props": [
|
||||||
|
{
|
||||||
|
"name": "blur",
|
||||||
|
"optional": false,
|
||||||
|
"readonly": false,
|
||||||
|
"type": "Function",
|
||||||
|
"default": ""
|
||||||
|
},
|
||||||
|
{
|
||||||
|
"name": "clear-click",
|
||||||
|
"optional": false,
|
||||||
|
"readonly": false,
|
||||||
|
"type": "Function",
|
||||||
|
"default": ""
|
||||||
|
},
|
||||||
|
{
|
||||||
|
"name": "focus",
|
||||||
|
"optional": false,
|
||||||
|
"readonly": false,
|
||||||
|
"type": "Function",
|
||||||
|
"default": ""
|
||||||
|
},
|
||||||
|
{
|
||||||
|
"name": "keydown",
|
||||||
|
"optional": false,
|
||||||
|
"readonly": false,
|
||||||
|
"type": "Function",
|
||||||
|
"default": ""
|
||||||
|
},
|
||||||
|
{
|
||||||
|
"name": "month-change",
|
||||||
|
"optional": false,
|
||||||
|
"readonly": false,
|
||||||
|
"type": "Function",
|
||||||
|
"default": ""
|
||||||
|
},
|
||||||
|
{
|
||||||
|
"name": "today-click",
|
||||||
|
"optional": false,
|
||||||
|
"readonly": false,
|
||||||
|
"type": "Function",
|
||||||
|
"default": ""
|
||||||
|
},
|
||||||
|
{
|
||||||
|
"name": "year-change",
|
||||||
|
"optional": false,
|
||||||
|
"readonly": false,
|
||||||
|
"type": "Function",
|
||||||
|
"default": ""
|
||||||
|
}
|
||||||
|
],
|
||||||
|
"methods": [
|
||||||
|
{
|
||||||
|
"name": "date-select",
|
||||||
|
"parameters": [
|
||||||
|
{
|
||||||
|
"name": "value",
|
||||||
|
"optional": false,
|
||||||
|
"type": "Date",
|
||||||
|
"description": "Selected value."
|
||||||
|
}
|
||||||
|
],
|
||||||
|
"returnType": "void",
|
||||||
|
"description": "Callback to invoke when a date is selected."
|
||||||
|
},
|
||||||
|
{
|
||||||
|
"name": "hide",
|
||||||
|
"parameters": [],
|
||||||
|
"returnType": "void",
|
||||||
|
"description": "Callback to invoke when datepicker panel is hidden."
|
||||||
|
},
|
||||||
|
{
|
||||||
|
"name": "input",
|
||||||
|
"parameters": [
|
||||||
|
{
|
||||||
|
"name": "event",
|
||||||
|
"optional": false,
|
||||||
|
"type": "Event",
|
||||||
|
"description": "Browser event"
|
||||||
|
}
|
||||||
|
],
|
||||||
|
"returnType": "void",
|
||||||
|
"description": "Callback to invoke when input field is being typed."
|
||||||
|
},
|
||||||
|
{
|
||||||
|
"name": "show",
|
||||||
|
"parameters": [],
|
||||||
|
"returnType": "void",
|
||||||
|
"description": "Callback to invoke when datepicker panel is shown."
|
||||||
|
},
|
||||||
|
{
|
||||||
|
"name": "update:modelValue",
|
||||||
|
"parameters": [
|
||||||
|
{
|
||||||
|
"name": "value",
|
||||||
|
"optional": false,
|
||||||
|
"type": "undefined | string | string[] | Date | Date[]",
|
||||||
|
"description": "New value."
|
||||||
|
}
|
||||||
|
],
|
||||||
|
"returnType": "void",
|
||||||
|
"description": "Emitted when the value changes."
|
||||||
|
}
|
||||||
|
]
|
||||||
|
},
|
||||||
"CalendarMonthChangeEvent": {
|
"CalendarMonthChangeEvent": {
|
||||||
|
"description": "Custom Calendar month change event.",
|
||||||
"relatedProp": "",
|
"relatedProp": "",
|
||||||
"props": [
|
"props": [
|
||||||
{
|
{
|
||||||
|
@ -3403,6 +3523,7 @@
|
||||||
"methods": []
|
"methods": []
|
||||||
},
|
},
|
||||||
"CalendarProps": {
|
"CalendarProps": {
|
||||||
|
"description": "Defines valid properties in Calendar component.",
|
||||||
"relatedProp": "",
|
"relatedProp": "",
|
||||||
"props": [
|
"props": [
|
||||||
{
|
{
|
||||||
|
@ -3434,16 +3555,16 @@
|
||||||
"optional": true,
|
"optional": true,
|
||||||
"readonly": false,
|
"readonly": false,
|
||||||
"type": "boolean",
|
"type": "boolean",
|
||||||
"default": "",
|
"default": "true",
|
||||||
"description": "Whether to automatically manage layering.\nDefault value is true."
|
"description": "Whether to automatically manage layering."
|
||||||
},
|
},
|
||||||
{
|
{
|
||||||
"name": "baseZIndex",
|
"name": "baseZIndex",
|
||||||
"optional": true,
|
"optional": true,
|
||||||
"readonly": false,
|
"readonly": false,
|
||||||
"type": "number",
|
"type": "number",
|
||||||
"default": "",
|
"default": "0",
|
||||||
"description": "Base zIndex value to use in layering.\nDefault value is 0."
|
"description": "Base zIndex value to use in layering."
|
||||||
},
|
},
|
||||||
{
|
{
|
||||||
"name": "dateFormat",
|
"name": "dateFormat",
|
||||||
|
@ -3458,15 +3579,15 @@
|
||||||
"optional": true,
|
"optional": true,
|
||||||
"readonly": false,
|
"readonly": false,
|
||||||
"type": "string",
|
"type": "string",
|
||||||
"default": "",
|
"default": "pi pi-chevron-down",
|
||||||
"description": "Icon to show in each of the decrement buttons.\nDefault value is 'pi pi-chevron-down'."
|
"description": "Icon to show in each of the decrement buttons."
|
||||||
},
|
},
|
||||||
{
|
{
|
||||||
"name": "disabled",
|
"name": "disabled",
|
||||||
"optional": true,
|
"optional": true,
|
||||||
"readonly": false,
|
"readonly": false,
|
||||||
"type": "boolean",
|
"type": "boolean",
|
||||||
"default": "",
|
"default": "false",
|
||||||
"description": "When present, it specifies that the component should be disabled."
|
"description": "When present, it specifies that the component should be disabled."
|
||||||
},
|
},
|
||||||
{
|
{
|
||||||
|
@ -3490,7 +3611,7 @@
|
||||||
"optional": true,
|
"optional": true,
|
||||||
"readonly": false,
|
"readonly": false,
|
||||||
"type": "boolean",
|
"type": "boolean",
|
||||||
"default": "",
|
"default": "false",
|
||||||
"description": "Whether to hide the overlay on date selection when showTime is enabled."
|
"description": "Whether to hide the overlay on date selection when showTime is enabled."
|
||||||
},
|
},
|
||||||
{
|
{
|
||||||
|
@ -3498,7 +3619,7 @@
|
||||||
"optional": true,
|
"optional": true,
|
||||||
"readonly": false,
|
"readonly": false,
|
||||||
"type": "boolean",
|
"type": "boolean",
|
||||||
"default": "",
|
"default": "false",
|
||||||
"description": "Whether to hide the overlay on date selection is completed when selectionMode is range."
|
"description": "Whether to hide the overlay on date selection is completed when selectionMode is range."
|
||||||
},
|
},
|
||||||
{
|
{
|
||||||
|
@ -3506,7 +3627,7 @@
|
||||||
"optional": true,
|
"optional": true,
|
||||||
"readonly": false,
|
"readonly": false,
|
||||||
"type": "\"12\" | \"24\"",
|
"type": "\"12\" | \"24\"",
|
||||||
"default": "",
|
"default": "24",
|
||||||
"description": "Specifies hour format."
|
"description": "Specifies hour format."
|
||||||
},
|
},
|
||||||
{
|
{
|
||||||
|
@ -3514,8 +3635,8 @@
|
||||||
"optional": true,
|
"optional": true,
|
||||||
"readonly": false,
|
"readonly": false,
|
||||||
"type": "string",
|
"type": "string",
|
||||||
"default": "",
|
"default": "pi pi-calendar",
|
||||||
"description": "Icon of the calendar button.\nDefault value is 'pi pi-calendar'."
|
"description": "Icon of the calendar button."
|
||||||
},
|
},
|
||||||
{
|
{
|
||||||
"name": "id",
|
"name": "id",
|
||||||
|
@ -3530,15 +3651,15 @@
|
||||||
"optional": true,
|
"optional": true,
|
||||||
"readonly": false,
|
"readonly": false,
|
||||||
"type": "string",
|
"type": "string",
|
||||||
"default": "",
|
"default": "pi pi-chevron-up",
|
||||||
"description": "Icon to show in each of the increment buttons.\nDefault value is 'pi pi-chevron-up'."
|
"description": "Icon to show in each of the increment buttons."
|
||||||
},
|
},
|
||||||
{
|
{
|
||||||
"name": "inline",
|
"name": "inline",
|
||||||
"optional": true,
|
"optional": true,
|
||||||
"readonly": false,
|
"readonly": false,
|
||||||
"type": "boolean",
|
"type": "boolean",
|
||||||
"default": "",
|
"default": "false",
|
||||||
"description": "When enabled, displays the calendar as inline instead of an overlay."
|
"description": "When enabled, displays the calendar as inline instead of an overlay."
|
||||||
},
|
},
|
||||||
{
|
{
|
||||||
|
@ -3578,8 +3699,8 @@
|
||||||
"optional": true,
|
"optional": true,
|
||||||
"readonly": false,
|
"readonly": false,
|
||||||
"type": "boolean",
|
"type": "boolean",
|
||||||
"default": "",
|
"default": "true",
|
||||||
"description": "Wheter to allow prevents entering the date manually via typing.\nDefault value is true."
|
"description": "Wheter to allow prevents entering the date manually via typing."
|
||||||
},
|
},
|
||||||
{
|
{
|
||||||
"name": "maxDate",
|
"name": "maxDate",
|
||||||
|
@ -3618,7 +3739,7 @@
|
||||||
"optional": true,
|
"optional": true,
|
||||||
"readonly": false,
|
"readonly": false,
|
||||||
"type": "boolean",
|
"type": "boolean",
|
||||||
"default": "",
|
"default": "false",
|
||||||
"description": "Whether the month should be rendered as a dropdown instead of text."
|
"description": "Whether the month should be rendered as a dropdown instead of text."
|
||||||
},
|
},
|
||||||
{
|
{
|
||||||
|
@ -3626,16 +3747,16 @@
|
||||||
"optional": true,
|
"optional": true,
|
||||||
"readonly": false,
|
"readonly": false,
|
||||||
"type": "string",
|
"type": "string",
|
||||||
"default": "",
|
"default": "pi pi-chevron-right",
|
||||||
"description": "Icon to show in the next button.\nDefault value is 'pi pi-chevron-right'."
|
"description": "Icon to show in the next button."
|
||||||
},
|
},
|
||||||
{
|
{
|
||||||
"name": "numberOfMonths",
|
"name": "numberOfMonths",
|
||||||
"optional": true,
|
"optional": true,
|
||||||
"readonly": false,
|
"readonly": false,
|
||||||
"type": "number",
|
"type": "number",
|
||||||
"default": "",
|
"default": "1",
|
||||||
"description": "Number of months to display.\nDefault value is 1."
|
"description": "Number of months to display."
|
||||||
},
|
},
|
||||||
{
|
{
|
||||||
"name": "panelClass",
|
"name": "panelClass",
|
||||||
|
@ -3674,15 +3795,15 @@
|
||||||
"optional": true,
|
"optional": true,
|
||||||
"readonly": false,
|
"readonly": false,
|
||||||
"type": "string",
|
"type": "string",
|
||||||
"default": "",
|
"default": "pi pi-chevron-left",
|
||||||
"description": "Icon to show in the previous button.\nDefault value is 'pi pi-chevron-left'."
|
"description": "Icon to show in the previous button."
|
||||||
},
|
},
|
||||||
{
|
{
|
||||||
"name": "readonly",
|
"name": "readonly",
|
||||||
"optional": true,
|
"optional": true,
|
||||||
"readonly": false,
|
"readonly": false,
|
||||||
"type": "boolean",
|
"type": "boolean",
|
||||||
"default": "",
|
"default": "false",
|
||||||
"description": "When present, it specifies that an input field is read-only."
|
"description": "When present, it specifies that an input field is read-only."
|
||||||
},
|
},
|
||||||
{
|
{
|
||||||
|
@ -3698,7 +3819,7 @@
|
||||||
"optional": true,
|
"optional": true,
|
||||||
"readonly": false,
|
"readonly": false,
|
||||||
"type": "boolean",
|
"type": "boolean",
|
||||||
"default": "",
|
"default": "false",
|
||||||
"description": "Whether days in other months shown before or after the current month are selectable. This only applies if the showOtherMonths option is set to true."
|
"description": "Whether days in other months shown before or after the current month are selectable. This only applies if the showOtherMonths option is set to true."
|
||||||
},
|
},
|
||||||
{
|
{
|
||||||
|
@ -3714,15 +3835,15 @@
|
||||||
"optional": true,
|
"optional": true,
|
||||||
"readonly": false,
|
"readonly": false,
|
||||||
"type": "string",
|
"type": "string",
|
||||||
"default": "",
|
"default": "+10",
|
||||||
"description": "The cutoff year for determining the century for a date.\nDefault value is +10."
|
"description": "The cutoff year for determining the century for a date."
|
||||||
},
|
},
|
||||||
{
|
{
|
||||||
"name": "showButtonBar",
|
"name": "showButtonBar",
|
||||||
"optional": true,
|
"optional": true,
|
||||||
"readonly": false,
|
"readonly": false,
|
||||||
"type": "boolean",
|
"type": "boolean",
|
||||||
"default": "",
|
"default": "false",
|
||||||
"description": "Whether to display today and clear buttons at the footer."
|
"description": "Whether to display today and clear buttons at the footer."
|
||||||
},
|
},
|
||||||
{
|
{
|
||||||
|
@ -3730,7 +3851,7 @@
|
||||||
"optional": true,
|
"optional": true,
|
||||||
"readonly": false,
|
"readonly": false,
|
||||||
"type": "boolean",
|
"type": "boolean",
|
||||||
"default": "",
|
"default": "false",
|
||||||
"description": "When enabled, displays a button with icon next to input."
|
"description": "When enabled, displays a button with icon next to input."
|
||||||
},
|
},
|
||||||
{
|
{
|
||||||
|
@ -3738,23 +3859,23 @@
|
||||||
"optional": true,
|
"optional": true,
|
||||||
"readonly": false,
|
"readonly": false,
|
||||||
"type": "boolean",
|
"type": "boolean",
|
||||||
"default": "",
|
"default": "true",
|
||||||
"description": "When disabled, datepicker will not be visible with input focus.\nDefault value is true."
|
"description": "When disabled, datepicker will not be visible with input focus."
|
||||||
},
|
},
|
||||||
{
|
{
|
||||||
"name": "showOtherMonths",
|
"name": "showOtherMonths",
|
||||||
"optional": true,
|
"optional": true,
|
||||||
"readonly": false,
|
"readonly": false,
|
||||||
"type": "boolean",
|
"type": "boolean",
|
||||||
"default": "",
|
"default": "true",
|
||||||
"description": "Whether to display dates in other months (non-selectable) at the start or end of the current month. To make these days selectable use the selectOtherMonths option.\nDefault value is true."
|
"description": "Whether to display dates in other months (non-selectable) at the start or end of the current month. To make these days selectable use the selectOtherMonths option."
|
||||||
},
|
},
|
||||||
{
|
{
|
||||||
"name": "showSeconds",
|
"name": "showSeconds",
|
||||||
"optional": true,
|
"optional": true,
|
||||||
"readonly": false,
|
"readonly": false,
|
||||||
"type": "boolean",
|
"type": "boolean",
|
||||||
"default": "",
|
"default": "false",
|
||||||
"description": "Whether to show the seconds in time picker."
|
"description": "Whether to show the seconds in time picker."
|
||||||
},
|
},
|
||||||
{
|
{
|
||||||
|
@ -3762,7 +3883,7 @@
|
||||||
"optional": true,
|
"optional": true,
|
||||||
"readonly": false,
|
"readonly": false,
|
||||||
"type": "boolean",
|
"type": "boolean",
|
||||||
"default": "",
|
"default": "false",
|
||||||
"description": "Whether to display timepicker."
|
"description": "Whether to display timepicker."
|
||||||
},
|
},
|
||||||
{
|
{
|
||||||
|
@ -3770,7 +3891,7 @@
|
||||||
"optional": true,
|
"optional": true,
|
||||||
"readonly": false,
|
"readonly": false,
|
||||||
"type": "boolean",
|
"type": "boolean",
|
||||||
"default": "",
|
"default": "false",
|
||||||
"description": "When enabled, calendar will show week numbers."
|
"description": "When enabled, calendar will show week numbers."
|
||||||
},
|
},
|
||||||
{
|
{
|
||||||
|
@ -3778,31 +3899,31 @@
|
||||||
"optional": true,
|
"optional": true,
|
||||||
"readonly": false,
|
"readonly": false,
|
||||||
"type": "number",
|
"type": "number",
|
||||||
"default": "",
|
"default": "1",
|
||||||
"description": "Hours to change per step.\nDefault value is 1."
|
"description": "Hours to change per step."
|
||||||
},
|
},
|
||||||
{
|
{
|
||||||
"name": "stepMinute",
|
"name": "stepMinute",
|
||||||
"optional": true,
|
"optional": true,
|
||||||
"readonly": false,
|
"readonly": false,
|
||||||
"type": "number",
|
"type": "number",
|
||||||
"default": "",
|
"default": "1",
|
||||||
"description": "Minutes to change per step.\nDefault value is 1."
|
"description": "Minutes to change per step."
|
||||||
},
|
},
|
||||||
{
|
{
|
||||||
"name": "stepSecond",
|
"name": "stepSecond",
|
||||||
"optional": true,
|
"optional": true,
|
||||||
"readonly": false,
|
"readonly": false,
|
||||||
"type": "number",
|
"type": "number",
|
||||||
"default": "",
|
"default": "1",
|
||||||
"description": "Seconds to change per step.\nDefault value is 1."
|
"description": "Seconds to change per step."
|
||||||
},
|
},
|
||||||
{
|
{
|
||||||
"name": "timeOnly",
|
"name": "timeOnly",
|
||||||
"optional": true,
|
"optional": true,
|
||||||
"readonly": false,
|
"readonly": false,
|
||||||
"type": "boolean",
|
"type": "boolean",
|
||||||
"default": "",
|
"default": "false",
|
||||||
"description": "Whether to display timepicker only."
|
"description": "Whether to display timepicker only."
|
||||||
},
|
},
|
||||||
{
|
{
|
||||||
|
@ -3810,15 +3931,15 @@
|
||||||
"optional": true,
|
"optional": true,
|
||||||
"readonly": false,
|
"readonly": false,
|
||||||
"type": "string",
|
"type": "string",
|
||||||
"default": "",
|
"default": ":",
|
||||||
"description": "Separator of time selector.\nDefault value is ':'."
|
"description": "Separator of time selector."
|
||||||
},
|
},
|
||||||
{
|
{
|
||||||
"name": "touchUI",
|
"name": "touchUI",
|
||||||
"optional": true,
|
"optional": true,
|
||||||
"readonly": false,
|
"readonly": false,
|
||||||
"type": "boolean",
|
"type": "boolean",
|
||||||
"default": "",
|
"default": "false",
|
||||||
"description": "When enabled, calendar overlay is displayed as optimized for touch devices."
|
"description": "When enabled, calendar overlay is displayed as optimized for touch devices."
|
||||||
},
|
},
|
||||||
{
|
{
|
||||||
|
@ -3834,7 +3955,7 @@
|
||||||
"optional": true,
|
"optional": true,
|
||||||
"readonly": false,
|
"readonly": false,
|
||||||
"type": "boolean",
|
"type": "boolean",
|
||||||
"default": "",
|
"default": "false",
|
||||||
"description": "Whether the year should be rendered as a dropdown instead of text."
|
"description": "Whether the year should be rendered as a dropdown instead of text."
|
||||||
},
|
},
|
||||||
{
|
{
|
||||||
|
@ -3842,13 +3963,14 @@
|
||||||
"optional": true,
|
"optional": true,
|
||||||
"readonly": false,
|
"readonly": false,
|
||||||
"type": "string",
|
"type": "string",
|
||||||
"default": "",
|
"default": "false",
|
||||||
"description": "The range of years displayed in the year drop-down in (nnnn:nnnn) format such as (2000:2020)."
|
"description": "The range of years displayed in the year drop-down in (nnnn:nnnn) format such as (2000:2020)."
|
||||||
}
|
}
|
||||||
],
|
],
|
||||||
"methods": []
|
"methods": []
|
||||||
},
|
},
|
||||||
"CalendarResponsiveOptions": {
|
"CalendarResponsiveOptions": {
|
||||||
|
"description": "Custom Calendar responsive options metadata.",
|
||||||
"relatedProp": "",
|
"relatedProp": "",
|
||||||
"props": [
|
"props": [
|
||||||
{
|
{
|
||||||
|
@ -3870,41 +3992,8 @@
|
||||||
],
|
],
|
||||||
"methods": []
|
"methods": []
|
||||||
},
|
},
|
||||||
"CalendarSlots": {
|
|
||||||
"relatedProp": "",
|
|
||||||
"props": [
|
|
||||||
{
|
|
||||||
"name": "date",
|
|
||||||
"optional": false,
|
|
||||||
"readonly": false,
|
|
||||||
"type": "Function",
|
|
||||||
"default": ""
|
|
||||||
},
|
|
||||||
{
|
|
||||||
"name": "decade",
|
|
||||||
"optional": false,
|
|
||||||
"readonly": false,
|
|
||||||
"type": "Function",
|
|
||||||
"default": ""
|
|
||||||
},
|
|
||||||
{
|
|
||||||
"name": "footer",
|
|
||||||
"optional": false,
|
|
||||||
"readonly": false,
|
|
||||||
"type": "Function",
|
|
||||||
"default": ""
|
|
||||||
},
|
|
||||||
{
|
|
||||||
"name": "header",
|
|
||||||
"optional": false,
|
|
||||||
"readonly": false,
|
|
||||||
"type": "Function",
|
|
||||||
"default": ""
|
|
||||||
}
|
|
||||||
],
|
|
||||||
"methods": []
|
|
||||||
},
|
|
||||||
"CalendarYearChangeEvent": {
|
"CalendarYearChangeEvent": {
|
||||||
|
"description": "Custom Calendar year change event.",
|
||||||
"relatedProp": "",
|
"relatedProp": "",
|
||||||
"props": [
|
"props": [
|
||||||
{
|
{
|
||||||
|
@ -3931,8 +4020,9 @@
|
||||||
"types": {
|
"types": {
|
||||||
"description": "Defines the custom types used by the module.",
|
"description": "Defines the custom types used by the module.",
|
||||||
"values": {
|
"values": {
|
||||||
"CalendarEmits": {
|
"CalendarSlots": {
|
||||||
"values": "{\n \"blur\": \"Function, \",\n \"clear-click\": \"Function, \",\n \"date-select\": \"Function, \",\n \"focus\": \"Function, \",\n \"hide\": \"Function, \",\n \"input\": \"Function, \",\n \"keydown\": \"Function, \",\n \"month-change\": \"Function, \",\n \"show\": \"Function, \",\n \"today-click\": \"Function, \",\n \"update:modelValue\": \"Function, \",\n \"year-change\": \"Function, \"\n}"
|
"values": "{}",
|
||||||
|
"description": "Defines valid slots in Calendar component."
|
||||||
}
|
}
|
||||||
}
|
}
|
||||||
}
|
}
|
||||||
|
|
Loading…
Reference in New Issue