Theme types .d.ts added
parent
8cb2f744e1
commit
4d90410cba
|
@ -0,0 +1,166 @@
|
||||||
|
const fs = require('fs');
|
||||||
|
const path = require('path');
|
||||||
|
|
||||||
|
// prettier-ignore
|
||||||
|
const THEME_COMPONENTS = ['Accordion','AutoComplete','Avatar','Badge','BlockUI','Breadcrumb','Button','Card','Carousel','CascadeSelect','Checkbox','Chip','ColorPicker','ConfirmDialog','ConfirmPopup','ContextMenu','DataTable','DataView','DatePicker','Dialog','Divider','Dock','Drawer','Editor','Fieldset','FileUpload','FloatLabel','Galleria','IconField','Image','InlineMessage','Inplace','InputChips','InputGroup','InputNumber','InputText','Knob','Listbox','MegaMenu','Menu','Menubar','Message','MeterGroup','MultiSelect','OrderList','OrganizationChart','Paginator','Panel','PanelMenu','Password','PickList','Popover','ProgressBar','ProgressSpinner','RadioButton','Rating','Ripple','ScrollPanel','Select','SelectButton','Skeleton','Slider','SpeedDial','SplitButton','Splitter','Stepper','Steps','Tabmenu','Tabs','TabView','Tag','Terminal','Textarea','TieredMenu','Timeline','Toast','ToggleButton','ToggleSwitch','Toolbar','Tooltip','Tree','TreeSelect','TreeTable'];
|
||||||
|
|
||||||
|
const themeName = 'aura';
|
||||||
|
const rootDir = path.resolve(__dirname, '../');
|
||||||
|
// const overwrittenDirPath = path.resolve(rootDir, 'types/overwritten');
|
||||||
|
const overwrittenDirPath = path.resolve(rootDir, 'components/lib/themes/types/overwritten');
|
||||||
|
|
||||||
|
try {
|
||||||
|
!fs.existsSync(overwrittenDirPath) && fs.mkdirSync(overwrittenDirPath, { recursive: true });
|
||||||
|
} catch (err) {
|
||||||
|
console.error(err);
|
||||||
|
}
|
||||||
|
|
||||||
|
THEME_COMPONENTS.forEach((comp) => {
|
||||||
|
const data = fs.readFileSync(path.resolve(rootDir, `components/lib/themes/${themeName}/${comp.toLowerCase()}/index.js`), { encoding: 'utf8', flag: 'r' });
|
||||||
|
let theme = data.replace('export default', 'module.exports = ');
|
||||||
|
|
||||||
|
try {
|
||||||
|
fs.writeFileSync(path.resolve(rootDir, `components/lib/themes/types/overwritten/${comp.toLowerCase()}.js`), theme);
|
||||||
|
|
||||||
|
const component = require(path.resolve(rootDir, `components/lib/themes/types/overwritten/${comp.toLowerCase()}.js`));
|
||||||
|
const filePath = path.resolve(rootDir, `components/lib/themes/types/${comp.toLowerCase()}`);
|
||||||
|
let outputFile = path.resolve(filePath, `index.d.ts`);
|
||||||
|
let defaultText = '';
|
||||||
|
|
||||||
|
!fs.existsSync(filePath) && fs.mkdirSync(filePath);
|
||||||
|
|
||||||
|
const splitTokenName = (text) => {
|
||||||
|
return text.split(/(?=[A-Z])/).map((part) => part.toLowerCase());
|
||||||
|
};
|
||||||
|
|
||||||
|
const createSubTokens = (tokenKeys, tokenValue) => {
|
||||||
|
let subTokens = '';
|
||||||
|
|
||||||
|
Object.entries(tokenValue).forEach(([tokenKey, tokenSubValue]) => {
|
||||||
|
if (typeof tokenSubValue === 'string') {
|
||||||
|
subTokens += createToken([...tokenKeys, tokenKey]);
|
||||||
|
} else {
|
||||||
|
subTokens += createNestedToken([...tokenKeys, tokenKey], tokenSubValue);
|
||||||
|
}
|
||||||
|
});
|
||||||
|
|
||||||
|
return subTokens;
|
||||||
|
};
|
||||||
|
|
||||||
|
const createDescription = (tokenKeys) => {
|
||||||
|
let description = '';
|
||||||
|
|
||||||
|
tokenKeys.forEach((tokenPart, i) => {
|
||||||
|
if (i !== 0) description += `${splitTokenName(tokenPart).join(' ')} `;
|
||||||
|
});
|
||||||
|
description = description.charAt(0).toUpperCase() + description.slice(1);
|
||||||
|
|
||||||
|
return `${description}of ${splitTokenName(tokenKeys[0]).join(' ')}`;
|
||||||
|
};
|
||||||
|
|
||||||
|
const createToken = (tokenKey) => {
|
||||||
|
let tokenName = tokenKey[tokenKey.length - 1];
|
||||||
|
let designTokenName = `${comp.toLowerCase()}`;
|
||||||
|
let tokenDescription = createDescription(tokenKey);
|
||||||
|
|
||||||
|
tokenKey.forEach((t) => {
|
||||||
|
if (t !== 'root') {
|
||||||
|
designTokenName += `.${splitTokenName(t).join('.')}`;
|
||||||
|
}
|
||||||
|
|
||||||
|
if (tokenName.indexOf('.') > -1 && tokenName.indexOf("'")) {
|
||||||
|
tokenName = `'${tokenName}'`;
|
||||||
|
}
|
||||||
|
});
|
||||||
|
|
||||||
|
return `
|
||||||
|
/**
|
||||||
|
* ${tokenDescription}
|
||||||
|
*
|
||||||
|
* @designToken ${designTokenName}
|
||||||
|
*/
|
||||||
|
${tokenName}?: string;`;
|
||||||
|
};
|
||||||
|
|
||||||
|
const createNestedToken = (tokenKeys, tokenValue) => {
|
||||||
|
let tokenName = tokenKeys[tokenKeys.length - 1];
|
||||||
|
let tokenDescription = createDescription(tokenKeys);
|
||||||
|
|
||||||
|
return `
|
||||||
|
/**
|
||||||
|
* ${tokenDescription}
|
||||||
|
*/
|
||||||
|
${tokenName}?: {
|
||||||
|
${createSubTokens(tokenKeys, tokenValue)}
|
||||||
|
};`;
|
||||||
|
};
|
||||||
|
|
||||||
|
const generateTokens = (tokenKey, tokenValue) => {
|
||||||
|
let subTokens = '';
|
||||||
|
|
||||||
|
Object.entries(tokenValue).forEach(([subTokenKey, subTokenValue]) => {
|
||||||
|
if (typeof subTokenValue === 'string') {
|
||||||
|
subTokens += createToken([tokenKey, subTokenKey]);
|
||||||
|
} else {
|
||||||
|
subTokens += createNestedToken([tokenKey, subTokenKey], subTokenValue);
|
||||||
|
}
|
||||||
|
});
|
||||||
|
|
||||||
|
defaultText += `
|
||||||
|
/**
|
||||||
|
* Used to pass tokens of the ${splitTokenName(tokenKey).join(' ')} section
|
||||||
|
*/
|
||||||
|
${tokenKey}?: {
|
||||||
|
${subTokens}
|
||||||
|
}`;
|
||||||
|
};
|
||||||
|
|
||||||
|
Object.entries(component).forEach(([tname, tvalue]) => {
|
||||||
|
if (tname !== 'colorScheme') {
|
||||||
|
if (Object.keys(component).includes('colorScheme')) {
|
||||||
|
if (Object.keys(component['colorScheme']['light']).includes(tname)) {
|
||||||
|
tvalue = { ...tvalue, ...component['colorScheme']['light'][tname] };
|
||||||
|
}
|
||||||
|
}
|
||||||
|
|
||||||
|
generateTokens(tname, tvalue);
|
||||||
|
} else {
|
||||||
|
Object.entries(tvalue['light']).forEach(([subtname, subtvalue]) => {
|
||||||
|
if (!Object.keys(component).includes(subtname)) {
|
||||||
|
generateTokens(subtname, subtvalue);
|
||||||
|
}
|
||||||
|
});
|
||||||
|
}
|
||||||
|
});
|
||||||
|
|
||||||
|
let text = `
|
||||||
|
/**
|
||||||
|
*
|
||||||
|
* ${comp} Design Tokens
|
||||||
|
*
|
||||||
|
* [Live Demo](https://www.primevue.org/${comp.toLowerCase()}/)
|
||||||
|
*
|
||||||
|
* @module themes/${comp.toLowerCase()}
|
||||||
|
*
|
||||||
|
*/
|
||||||
|
|
||||||
|
import { ColorSchemeDesignToken } from '..';
|
||||||
|
|
||||||
|
export interface ${comp}DesignTokens extends ColorSchemeDesignToken<${comp}DesignTokens> {
|
||||||
|
${defaultText}
|
||||||
|
}
|
||||||
|
`;
|
||||||
|
|
||||||
|
fs.writeFileSync(outputFile, text, 'utf8');
|
||||||
|
} catch (err) {
|
||||||
|
console.error(err);
|
||||||
|
}
|
||||||
|
});
|
||||||
|
|
||||||
|
try {
|
||||||
|
if (fs.existsSync(overwrittenDirPath)) {
|
||||||
|
fs.rmSync(overwrittenDirPath, { recursive: true, force: true });
|
||||||
|
}
|
||||||
|
} catch (err) {
|
||||||
|
console.error(err);
|
||||||
|
}
|
|
@ -2,5 +2,5 @@
|
||||||
"main": "./index.cjs.js",
|
"main": "./index.cjs.js",
|
||||||
"module": "./index.esm.js",
|
"module": "./index.esm.js",
|
||||||
"unpkg": "./index.min.js",
|
"unpkg": "./index.min.js",
|
||||||
"types": "./index.d.ts"
|
"types": "../types/accordion/index.d.ts"
|
||||||
}
|
}
|
||||||
|
|
|
@ -1,114 +0,0 @@
|
||||||
/**
|
|
||||||
*
|
|
||||||
* AutoComplete is an input component that provides real-time suggestions while being typed.
|
|
||||||
*
|
|
||||||
* [Live Demo](https://www.primevue.org/autocomplete/)
|
|
||||||
*
|
|
||||||
* @module aura/autocomplete
|
|
||||||
*
|
|
||||||
*/
|
|
||||||
import { ColorSchemeDesignToken } from '..';
|
|
||||||
|
|
||||||
/**
|
|
||||||
* **PrimeVue - AutoComplete**
|
|
||||||
*
|
|
||||||
* _AutoComplete is an input component that provides real-time suggestions while being typed._
|
|
||||||
*
|
|
||||||
* [Live Demo](https://www.primevue.org/autocomplete/)
|
|
||||||
* --- ---
|
|
||||||
* 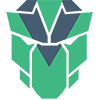
|
|
||||||
*
|
|
||||||
* @group DesignTokens
|
|
||||||
*
|
|
||||||
*/
|
|
||||||
export interface AutoCompleteDesignTokens extends ColorSchemeDesignToken<AutoCompleteDesignTokens> {
|
|
||||||
/**
|
|
||||||
* Used to pass tokens of the root section
|
|
||||||
*/
|
|
||||||
root: {
|
|
||||||
background?: string;
|
|
||||||
disabledBackground?: string;
|
|
||||||
filledBackground?: string;
|
|
||||||
filledFocusBackground?: string;
|
|
||||||
borderColor?: string;
|
|
||||||
hoverBorderColor?: string;
|
|
||||||
focusBorderColor?: string;
|
|
||||||
invalidBorderColor?: string;
|
|
||||||
color?: string;
|
|
||||||
disabledColor?: string;
|
|
||||||
placeholderColor?: string;
|
|
||||||
shadow?: string;
|
|
||||||
paddingX?: string;
|
|
||||||
paddingY?: string;
|
|
||||||
borderRadius?: string;
|
|
||||||
focusRing: {
|
|
||||||
width?: string;
|
|
||||||
style?: string;
|
|
||||||
color?: string;
|
|
||||||
offset?: string;
|
|
||||||
shadow?: string;
|
|
||||||
};
|
|
||||||
};
|
|
||||||
overlay: {
|
|
||||||
background?: string;
|
|
||||||
borderColor?: string;
|
|
||||||
borderRadius?: string;
|
|
||||||
color?: string;
|
|
||||||
shadow?: string;
|
|
||||||
};
|
|
||||||
list: {
|
|
||||||
padding?: string;
|
|
||||||
gap?: string;
|
|
||||||
};
|
|
||||||
option: {
|
|
||||||
focusBackground?: string;
|
|
||||||
selectedBackground?: string;
|
|
||||||
selectedFocusBackground?: string;
|
|
||||||
color?: string;
|
|
||||||
focusColor?: string;
|
|
||||||
selectedColor?: string;
|
|
||||||
selectedFocusColor?: string;
|
|
||||||
padding?: string;
|
|
||||||
borderRadius?: string;
|
|
||||||
};
|
|
||||||
optionGroup: {
|
|
||||||
background?: string;
|
|
||||||
color?: string;
|
|
||||||
fontWeight?: string;
|
|
||||||
padding?: string;
|
|
||||||
};
|
|
||||||
dropdown: {
|
|
||||||
width?: string;
|
|
||||||
borderColor?: string;
|
|
||||||
hoverBorderColor?: string;
|
|
||||||
activeBorderColor?: string;
|
|
||||||
borderRadius?: string;
|
|
||||||
focusRing: {
|
|
||||||
width?: string;
|
|
||||||
style?: string;
|
|
||||||
color?: string;
|
|
||||||
offset?: string;
|
|
||||||
shadow?: string;
|
|
||||||
};
|
|
||||||
background?: string;
|
|
||||||
hoverBackground?: string;
|
|
||||||
/**
|
|
||||||
*
|
|
||||||
* @designToken autocomplete.dropdown.active.background
|
|
||||||
*/
|
|
||||||
activeBackground?: string;
|
|
||||||
color?: string;
|
|
||||||
hoverColor?: string;
|
|
||||||
activeColor?: string;
|
|
||||||
};
|
|
||||||
chip: {
|
|
||||||
borderRadius?: string;
|
|
||||||
};
|
|
||||||
emptyMessage: {
|
|
||||||
/**
|
|
||||||
*
|
|
||||||
* @designToken autocomplete.empty.message.padding
|
|
||||||
*/
|
|
||||||
padding?: string;
|
|
||||||
};
|
|
||||||
}
|
|
|
@ -2,5 +2,5 @@
|
||||||
"main": "./index.cjs.js",
|
"main": "./index.cjs.js",
|
||||||
"module": "./index.esm.js",
|
"module": "./index.esm.js",
|
||||||
"unpkg": "./index.min.js",
|
"unpkg": "./index.min.js",
|
||||||
"types": "./index.d.ts"
|
"types": "../types/autocomplete/index.d.ts"
|
||||||
}
|
}
|
||||||
|
|
|
@ -2,5 +2,5 @@
|
||||||
"main": "./index.cjs.js",
|
"main": "./index.cjs.js",
|
||||||
"module": "./index.esm.js",
|
"module": "./index.esm.js",
|
||||||
"unpkg": "./index.min.js",
|
"unpkg": "./index.min.js",
|
||||||
"types": "./index.d.ts"
|
"types": "../types/avatar/index.d.ts"
|
||||||
}
|
}
|
||||||
|
|
|
@ -2,5 +2,5 @@
|
||||||
"main": "./index.cjs.js",
|
"main": "./index.cjs.js",
|
||||||
"module": "./index.esm.js",
|
"module": "./index.esm.js",
|
||||||
"unpkg": "./index.min.js",
|
"unpkg": "./index.min.js",
|
||||||
"types": "./index.d.ts"
|
"types": "../types/badge/index.d.ts"
|
||||||
}
|
}
|
||||||
|
|
|
@ -2,5 +2,5 @@
|
||||||
"main": "./index.cjs.js",
|
"main": "./index.cjs.js",
|
||||||
"module": "./index.esm.js",
|
"module": "./index.esm.js",
|
||||||
"unpkg": "./index.min.js",
|
"unpkg": "./index.min.js",
|
||||||
"types": "./index.d.ts"
|
"types": "../types/blockui/index.d.ts"
|
||||||
}
|
}
|
||||||
|
|
|
@ -2,5 +2,5 @@
|
||||||
"main": "./index.cjs.js",
|
"main": "./index.cjs.js",
|
||||||
"module": "./index.esm.js",
|
"module": "./index.esm.js",
|
||||||
"unpkg": "./index.min.js",
|
"unpkg": "./index.min.js",
|
||||||
"types": "./index.d.ts"
|
"types": "../types/breadcrumb/index.d.ts"
|
||||||
}
|
}
|
||||||
|
|
|
@ -2,5 +2,5 @@
|
||||||
"main": "./index.cjs.js",
|
"main": "./index.cjs.js",
|
||||||
"module": "./index.esm.js",
|
"module": "./index.esm.js",
|
||||||
"unpkg": "./index.min.js",
|
"unpkg": "./index.min.js",
|
||||||
"types": "./index.d.ts"
|
"types": "../types/button/index.d.ts"
|
||||||
}
|
}
|
||||||
|
|
|
@ -1 +0,0 @@
|
||||||
export default {};
|
|
|
@ -1,6 +0,0 @@
|
||||||
{
|
|
||||||
"main": "./index.cjs.js",
|
|
||||||
"module": "./index.esm.js",
|
|
||||||
"unpkg": "./index.min.js",
|
|
||||||
"types": "./index.d.ts"
|
|
||||||
}
|
|
|
@ -2,5 +2,5 @@
|
||||||
"main": "./index.cjs.js",
|
"main": "./index.cjs.js",
|
||||||
"module": "./index.esm.js",
|
"module": "./index.esm.js",
|
||||||
"unpkg": "./index.min.js",
|
"unpkg": "./index.min.js",
|
||||||
"types": "./index.d.ts"
|
"types": "../types/card/index.d.ts"
|
||||||
}
|
}
|
||||||
|
|
|
@ -2,5 +2,5 @@
|
||||||
"main": "./index.cjs.js",
|
"main": "./index.cjs.js",
|
||||||
"module": "./index.esm.js",
|
"module": "./index.esm.js",
|
||||||
"unpkg": "./index.min.js",
|
"unpkg": "./index.min.js",
|
||||||
"types": "./index.d.ts"
|
"types": "../types/carousel/index.d.ts"
|
||||||
}
|
}
|
||||||
|
|
|
@ -2,5 +2,5 @@
|
||||||
"main": "./index.cjs.js",
|
"main": "./index.cjs.js",
|
||||||
"module": "./index.esm.js",
|
"module": "./index.esm.js",
|
||||||
"unpkg": "./index.min.js",
|
"unpkg": "./index.min.js",
|
||||||
"types": "./index.d.ts"
|
"types": "../types/cascadeselect/index.d.ts"
|
||||||
}
|
}
|
||||||
|
|
|
@ -2,5 +2,5 @@
|
||||||
"main": "./index.cjs.js",
|
"main": "./index.cjs.js",
|
||||||
"module": "./index.esm.js",
|
"module": "./index.esm.js",
|
||||||
"unpkg": "./index.min.js",
|
"unpkg": "./index.min.js",
|
||||||
"types": "./index.d.ts"
|
"types": "../types/checkbox/index.d.ts"
|
||||||
}
|
}
|
||||||
|
|
|
@ -2,5 +2,5 @@
|
||||||
"main": "./index.cjs.js",
|
"main": "./index.cjs.js",
|
||||||
"module": "./index.esm.js",
|
"module": "./index.esm.js",
|
||||||
"unpkg": "./index.min.js",
|
"unpkg": "./index.min.js",
|
||||||
"types": "./index.d.ts"
|
"types": "../types/chip/index.d.ts"
|
||||||
}
|
}
|
||||||
|
|
|
@ -2,5 +2,5 @@
|
||||||
"main": "./index.cjs.js",
|
"main": "./index.cjs.js",
|
||||||
"module": "./index.esm.js",
|
"module": "./index.esm.js",
|
||||||
"unpkg": "./index.min.js",
|
"unpkg": "./index.min.js",
|
||||||
"types": "./index.d.ts"
|
"types": "../types/colorpicker/index.d.ts"
|
||||||
}
|
}
|
||||||
|
|
|
@ -2,5 +2,5 @@
|
||||||
"main": "./index.cjs.js",
|
"main": "./index.cjs.js",
|
||||||
"module": "./index.esm.js",
|
"module": "./index.esm.js",
|
||||||
"unpkg": "./index.min.js",
|
"unpkg": "./index.min.js",
|
||||||
"types": "./index.d.ts"
|
"types": "../types/confirmdialog/index.d.ts"
|
||||||
}
|
}
|
||||||
|
|
|
@ -2,5 +2,5 @@
|
||||||
"main": "./index.cjs.js",
|
"main": "./index.cjs.js",
|
||||||
"module": "./index.esm.js",
|
"module": "./index.esm.js",
|
||||||
"unpkg": "./index.min.js",
|
"unpkg": "./index.min.js",
|
||||||
"types": "./index.d.ts"
|
"types": "../types/confirmpopup/index.d.ts"
|
||||||
}
|
}
|
||||||
|
|
|
@ -2,5 +2,5 @@
|
||||||
"main": "./index.cjs.js",
|
"main": "./index.cjs.js",
|
||||||
"module": "./index.esm.js",
|
"module": "./index.esm.js",
|
||||||
"unpkg": "./index.min.js",
|
"unpkg": "./index.min.js",
|
||||||
"types": "./index.d.ts"
|
"types": "../types/contextmenu/index.d.ts"
|
||||||
}
|
}
|
||||||
|
|
|
@ -2,5 +2,5 @@
|
||||||
"main": "./index.cjs.js",
|
"main": "./index.cjs.js",
|
||||||
"module": "./index.esm.js",
|
"module": "./index.esm.js",
|
||||||
"unpkg": "./index.min.js",
|
"unpkg": "./index.min.js",
|
||||||
"types": "./index.d.ts"
|
"types": "../types/datatable/index.d.ts"
|
||||||
}
|
}
|
||||||
|
|
|
@ -2,5 +2,5 @@
|
||||||
"main": "./index.cjs.js",
|
"main": "./index.cjs.js",
|
||||||
"module": "./index.esm.js",
|
"module": "./index.esm.js",
|
||||||
"unpkg": "./index.min.js",
|
"unpkg": "./index.min.js",
|
||||||
"types": "./index.d.ts"
|
"types": "../types/dataview/index.d.ts"
|
||||||
}
|
}
|
||||||
|
|
|
@ -2,5 +2,5 @@
|
||||||
"main": "./index.cjs.js",
|
"main": "./index.cjs.js",
|
||||||
"module": "./index.esm.js",
|
"module": "./index.esm.js",
|
||||||
"unpkg": "./index.min.js",
|
"unpkg": "./index.min.js",
|
||||||
"types": "./index.d.ts"
|
"types": "../types/datepicker/index.d.ts"
|
||||||
}
|
}
|
||||||
|
|
|
@ -2,5 +2,5 @@
|
||||||
"main": "./index.cjs.js",
|
"main": "./index.cjs.js",
|
||||||
"module": "./index.esm.js",
|
"module": "./index.esm.js",
|
||||||
"unpkg": "./index.min.js",
|
"unpkg": "./index.min.js",
|
||||||
"types": "./index.d.ts"
|
"types": "../types/dialog/index.d.ts"
|
||||||
}
|
}
|
||||||
|
|
|
@ -2,5 +2,5 @@
|
||||||
"main": "./index.cjs.js",
|
"main": "./index.cjs.js",
|
||||||
"module": "./index.esm.js",
|
"module": "./index.esm.js",
|
||||||
"unpkg": "./index.min.js",
|
"unpkg": "./index.min.js",
|
||||||
"types": "./index.d.ts"
|
"types": "../types/divider/index.d.ts"
|
||||||
}
|
}
|
||||||
|
|
|
@ -2,5 +2,5 @@
|
||||||
"main": "./index.cjs.js",
|
"main": "./index.cjs.js",
|
||||||
"module": "./index.esm.js",
|
"module": "./index.esm.js",
|
||||||
"unpkg": "./index.min.js",
|
"unpkg": "./index.min.js",
|
||||||
"types": "./index.d.ts"
|
"types": "../types/dock/index.d.ts"
|
||||||
}
|
}
|
||||||
|
|
|
@ -2,5 +2,5 @@
|
||||||
"main": "./index.cjs.js",
|
"main": "./index.cjs.js",
|
||||||
"module": "./index.esm.js",
|
"module": "./index.esm.js",
|
||||||
"unpkg": "./index.min.js",
|
"unpkg": "./index.min.js",
|
||||||
"types": "./index.d.ts"
|
"types": "../types/drawer/index.d.ts"
|
||||||
}
|
}
|
||||||
|
|
|
@ -2,5 +2,5 @@
|
||||||
"main": "./index.cjs.js",
|
"main": "./index.cjs.js",
|
||||||
"module": "./index.esm.js",
|
"module": "./index.esm.js",
|
||||||
"unpkg": "./index.min.js",
|
"unpkg": "./index.min.js",
|
||||||
"types": "./index.d.ts"
|
"types": "../types/editor/index.d.ts"
|
||||||
}
|
}
|
||||||
|
|
|
@ -2,5 +2,5 @@
|
||||||
"main": "./index.cjs.js",
|
"main": "./index.cjs.js",
|
||||||
"module": "./index.esm.js",
|
"module": "./index.esm.js",
|
||||||
"unpkg": "./index.min.js",
|
"unpkg": "./index.min.js",
|
||||||
"types": "./index.d.ts"
|
"types": "../types/fieldset/index.d.ts"
|
||||||
}
|
}
|
||||||
|
|
|
@ -2,5 +2,5 @@
|
||||||
"main": "./index.cjs.js",
|
"main": "./index.cjs.js",
|
||||||
"module": "./index.esm.js",
|
"module": "./index.esm.js",
|
||||||
"unpkg": "./index.min.js",
|
"unpkg": "./index.min.js",
|
||||||
"types": "./index.d.ts"
|
"types": "../types/fileupload/index.d.ts"
|
||||||
}
|
}
|
||||||
|
|
|
@ -2,5 +2,5 @@
|
||||||
"main": "./index.cjs.js",
|
"main": "./index.cjs.js",
|
||||||
"module": "./index.esm.js",
|
"module": "./index.esm.js",
|
||||||
"unpkg": "./index.min.js",
|
"unpkg": "./index.min.js",
|
||||||
"types": "./index.d.ts"
|
"types": "../types/floatlabel/index.d.ts"
|
||||||
}
|
}
|
||||||
|
|
|
@ -2,5 +2,5 @@
|
||||||
"main": "./index.cjs.js",
|
"main": "./index.cjs.js",
|
||||||
"module": "./index.esm.js",
|
"module": "./index.esm.js",
|
||||||
"unpkg": "./index.min.js",
|
"unpkg": "./index.min.js",
|
||||||
"types": "./index.d.ts"
|
"types": "../types/galleria/index.d.ts"
|
||||||
}
|
}
|
||||||
|
|
|
@ -2,5 +2,5 @@
|
||||||
"main": "./index.cjs.js",
|
"main": "./index.cjs.js",
|
||||||
"module": "./index.esm.js",
|
"module": "./index.esm.js",
|
||||||
"unpkg": "./index.min.js",
|
"unpkg": "./index.min.js",
|
||||||
"types": "./index.d.ts"
|
"types": "../types/iconfield/index.d.ts"
|
||||||
}
|
}
|
||||||
|
|
|
@ -2,5 +2,5 @@
|
||||||
"main": "./index.cjs.js",
|
"main": "./index.cjs.js",
|
||||||
"module": "./index.esm.js",
|
"module": "./index.esm.js",
|
||||||
"unpkg": "./index.min.js",
|
"unpkg": "./index.min.js",
|
||||||
"types": "./index.d.ts"
|
"types": "../types/image/index.d.ts"
|
||||||
}
|
}
|
||||||
|
|
|
@ -5,7 +5,6 @@ import badge from 'primevue/themes/aura/badge';
|
||||||
import blockui from 'primevue/themes/aura/blockui';
|
import blockui from 'primevue/themes/aura/blockui';
|
||||||
import breadcrumb from 'primevue/themes/aura/breadcrumb';
|
import breadcrumb from 'primevue/themes/aura/breadcrumb';
|
||||||
import button from 'primevue/themes/aura/button';
|
import button from 'primevue/themes/aura/button';
|
||||||
import buttongroup from 'primevue/themes/aura/buttongroup';
|
|
||||||
import card from 'primevue/themes/aura/card';
|
import card from 'primevue/themes/aura/card';
|
||||||
import carousel from 'primevue/themes/aura/carousel';
|
import carousel from 'primevue/themes/aura/carousel';
|
||||||
import cascadeselect from 'primevue/themes/aura/cascadeselect';
|
import cascadeselect from 'primevue/themes/aura/cascadeselect';
|
||||||
|
@ -34,7 +33,6 @@ import inplace from 'primevue/themes/aura/inplace';
|
||||||
import inputchips from 'primevue/themes/aura/inputchips';
|
import inputchips from 'primevue/themes/aura/inputchips';
|
||||||
import inputgroup from 'primevue/themes/aura/inputgroup';
|
import inputgroup from 'primevue/themes/aura/inputgroup';
|
||||||
import inputnumber from 'primevue/themes/aura/inputnumber';
|
import inputnumber from 'primevue/themes/aura/inputnumber';
|
||||||
import inputotp from 'primevue/themes/aura/inputotp';
|
|
||||||
import inputtext from 'primevue/themes/aura/inputtext';
|
import inputtext from 'primevue/themes/aura/inputtext';
|
||||||
import knob from 'primevue/themes/aura/knob';
|
import knob from 'primevue/themes/aura/knob';
|
||||||
import listbox from 'primevue/themes/aura/listbox';
|
import listbox from 'primevue/themes/aura/listbox';
|
||||||
|
@ -58,7 +56,6 @@ import radiobutton from 'primevue/themes/aura/radiobutton';
|
||||||
import rating from 'primevue/themes/aura/rating';
|
import rating from 'primevue/themes/aura/rating';
|
||||||
import ripple from 'primevue/themes/aura/ripple';
|
import ripple from 'primevue/themes/aura/ripple';
|
||||||
import scrollpanel from 'primevue/themes/aura/scrollpanel';
|
import scrollpanel from 'primevue/themes/aura/scrollpanel';
|
||||||
import scrolltop from 'primevue/themes/aura/scrolltop';
|
|
||||||
import select from 'primevue/themes/aura/select';
|
import select from 'primevue/themes/aura/select';
|
||||||
import selectbutton from 'primevue/themes/aura/selectbutton';
|
import selectbutton from 'primevue/themes/aura/selectbutton';
|
||||||
import skeleton from 'primevue/themes/aura/skeleton';
|
import skeleton from 'primevue/themes/aura/skeleton';
|
||||||
|
@ -465,7 +462,6 @@ export default {
|
||||||
blockui,
|
blockui,
|
||||||
breadcrumb,
|
breadcrumb,
|
||||||
button,
|
button,
|
||||||
buttongroup,
|
|
||||||
datepicker,
|
datepicker,
|
||||||
card,
|
card,
|
||||||
carousel,
|
carousel,
|
||||||
|
@ -494,8 +490,6 @@ export default {
|
||||||
inputchips,
|
inputchips,
|
||||||
inputgroup,
|
inputgroup,
|
||||||
inputnumber,
|
inputnumber,
|
||||||
inputotp,
|
|
||||||
toggleswitch,
|
|
||||||
inputtext,
|
inputtext,
|
||||||
knob,
|
knob,
|
||||||
listbox,
|
listbox,
|
||||||
|
@ -518,7 +512,6 @@ export default {
|
||||||
radiobutton,
|
radiobutton,
|
||||||
rating,
|
rating,
|
||||||
scrollpanel,
|
scrollpanel,
|
||||||
scrolltop,
|
|
||||||
select,
|
select,
|
||||||
selectbutton,
|
selectbutton,
|
||||||
skeleton,
|
skeleton,
|
||||||
|
@ -537,6 +530,7 @@ export default {
|
||||||
terminal,
|
terminal,
|
||||||
timeline,
|
timeline,
|
||||||
togglebutton,
|
togglebutton,
|
||||||
|
toggleswitch,
|
||||||
tree,
|
tree,
|
||||||
treeselect,
|
treeselect,
|
||||||
treetable,
|
treetable,
|
||||||
|
|
|
@ -2,5 +2,5 @@
|
||||||
"main": "./index.cjs.js",
|
"main": "./index.cjs.js",
|
||||||
"module": "./index.esm.js",
|
"module": "./index.esm.js",
|
||||||
"unpkg": "./index.min.js",
|
"unpkg": "./index.min.js",
|
||||||
"types": "./index.d.ts"
|
"types": "../types/inlinemessage/index.d.ts"
|
||||||
}
|
}
|
||||||
|
|
|
@ -2,5 +2,5 @@
|
||||||
"main": "./index.cjs.js",
|
"main": "./index.cjs.js",
|
||||||
"module": "./index.esm.js",
|
"module": "./index.esm.js",
|
||||||
"unpkg": "./index.min.js",
|
"unpkg": "./index.min.js",
|
||||||
"types": "./index.d.ts"
|
"types": "../types/inplace/index.d.ts"
|
||||||
}
|
}
|
||||||
|
|
|
@ -2,5 +2,5 @@
|
||||||
"main": "./index.cjs.js",
|
"main": "./index.cjs.js",
|
||||||
"module": "./index.esm.js",
|
"module": "./index.esm.js",
|
||||||
"unpkg": "./index.min.js",
|
"unpkg": "./index.min.js",
|
||||||
"types": "./index.d.ts"
|
"types": "../types/inputchips/index.d.ts"
|
||||||
}
|
}
|
||||||
|
|
|
@ -2,5 +2,5 @@
|
||||||
"main": "./index.cjs.js",
|
"main": "./index.cjs.js",
|
||||||
"module": "./index.esm.js",
|
"module": "./index.esm.js",
|
||||||
"unpkg": "./index.min.js",
|
"unpkg": "./index.min.js",
|
||||||
"types": "./index.d.ts"
|
"types": "../types/inputgroup/index.d.ts"
|
||||||
}
|
}
|
||||||
|
|
|
@ -2,5 +2,5 @@
|
||||||
"main": "./index.cjs.js",
|
"main": "./index.cjs.js",
|
||||||
"module": "./index.esm.js",
|
"module": "./index.esm.js",
|
||||||
"unpkg": "./index.min.js",
|
"unpkg": "./index.min.js",
|
||||||
"types": "./index.d.ts"
|
"types": "../types/inputnumber/index.d.ts"
|
||||||
}
|
}
|
||||||
|
|
|
@ -1 +0,0 @@
|
||||||
export default {};
|
|
|
@ -1,6 +0,0 @@
|
||||||
{
|
|
||||||
"main": "./index.cjs.js",
|
|
||||||
"module": "./index.esm.js",
|
|
||||||
"unpkg": "./index.min.js",
|
|
||||||
"types": "./index.d.ts"
|
|
||||||
}
|
|
|
@ -2,5 +2,5 @@
|
||||||
"main": "./index.cjs.js",
|
"main": "./index.cjs.js",
|
||||||
"module": "./index.esm.js",
|
"module": "./index.esm.js",
|
||||||
"unpkg": "./index.min.js",
|
"unpkg": "./index.min.js",
|
||||||
"types": "./index.d.ts"
|
"types": "../types/inputtext/index.d.ts"
|
||||||
}
|
}
|
||||||
|
|
|
@ -2,5 +2,5 @@
|
||||||
"main": "./index.cjs.js",
|
"main": "./index.cjs.js",
|
||||||
"module": "./index.esm.js",
|
"module": "./index.esm.js",
|
||||||
"unpkg": "./index.min.js",
|
"unpkg": "./index.min.js",
|
||||||
"types": "./index.d.ts"
|
"types": "../types/knob/index.d.ts"
|
||||||
}
|
}
|
||||||
|
|
|
@ -2,5 +2,5 @@
|
||||||
"main": "./index.cjs.js",
|
"main": "./index.cjs.js",
|
||||||
"module": "./index.esm.js",
|
"module": "./index.esm.js",
|
||||||
"unpkg": "./index.min.js",
|
"unpkg": "./index.min.js",
|
||||||
"types": "./index.d.ts"
|
"types": "../types/listbox/index.d.ts"
|
||||||
}
|
}
|
||||||
|
|
|
@ -2,5 +2,5 @@
|
||||||
"main": "./index.cjs.js",
|
"main": "./index.cjs.js",
|
||||||
"module": "./index.esm.js",
|
"module": "./index.esm.js",
|
||||||
"unpkg": "./index.min.js",
|
"unpkg": "./index.min.js",
|
||||||
"types": "./index.d.ts"
|
"types": "../types/megamenu/index.d.ts"
|
||||||
}
|
}
|
||||||
|
|
|
@ -2,5 +2,5 @@
|
||||||
"main": "./index.cjs.js",
|
"main": "./index.cjs.js",
|
||||||
"module": "./index.esm.js",
|
"module": "./index.esm.js",
|
||||||
"unpkg": "./index.min.js",
|
"unpkg": "./index.min.js",
|
||||||
"types": "./index.d.ts"
|
"types": "../types/menu/index.d.ts"
|
||||||
}
|
}
|
||||||
|
|
|
@ -2,5 +2,5 @@
|
||||||
"main": "./index.cjs.js",
|
"main": "./index.cjs.js",
|
||||||
"module": "./index.esm.js",
|
"module": "./index.esm.js",
|
||||||
"unpkg": "./index.min.js",
|
"unpkg": "./index.min.js",
|
||||||
"types": "./index.d.ts"
|
"types": "../types/menubar/index.d.ts"
|
||||||
}
|
}
|
||||||
|
|
|
@ -2,5 +2,5 @@
|
||||||
"main": "./index.cjs.js",
|
"main": "./index.cjs.js",
|
||||||
"module": "./index.esm.js",
|
"module": "./index.esm.js",
|
||||||
"unpkg": "./index.min.js",
|
"unpkg": "./index.min.js",
|
||||||
"types": "./index.d.ts"
|
"types": "../types/message/index.d.ts"
|
||||||
}
|
}
|
||||||
|
|
|
@ -2,5 +2,5 @@
|
||||||
"main": "./index.cjs.js",
|
"main": "./index.cjs.js",
|
||||||
"module": "./index.esm.js",
|
"module": "./index.esm.js",
|
||||||
"unpkg": "./index.min.js",
|
"unpkg": "./index.min.js",
|
||||||
"types": "./index.d.ts"
|
"types": "../types/metergroup/index.d.ts"
|
||||||
}
|
}
|
||||||
|
|
|
@ -2,5 +2,5 @@
|
||||||
"main": "./index.cjs.js",
|
"main": "./index.cjs.js",
|
||||||
"module": "./index.esm.js",
|
"module": "./index.esm.js",
|
||||||
"unpkg": "./index.min.js",
|
"unpkg": "./index.min.js",
|
||||||
"types": "./index.d.ts"
|
"types": "../types/multiselect/index.d.ts"
|
||||||
}
|
}
|
||||||
|
|
|
@ -2,5 +2,5 @@
|
||||||
"main": "./index.cjs.js",
|
"main": "./index.cjs.js",
|
||||||
"module": "./index.esm.js",
|
"module": "./index.esm.js",
|
||||||
"unpkg": "./index.min.js",
|
"unpkg": "./index.min.js",
|
||||||
"types": "./index.d.ts"
|
"types": "../types/orderlist/index.d.ts"
|
||||||
}
|
}
|
||||||
|
|
|
@ -2,5 +2,5 @@
|
||||||
"main": "./index.cjs.js",
|
"main": "./index.cjs.js",
|
||||||
"module": "./index.esm.js",
|
"module": "./index.esm.js",
|
||||||
"unpkg": "./index.min.js",
|
"unpkg": "./index.min.js",
|
||||||
"types": "./index.d.ts"
|
"types": "../types/organizationchart/index.d.ts"
|
||||||
}
|
}
|
||||||
|
|
Some files were not shown because too many files have changed in this diff Show More
Loading…
Reference in New Issue