Fixed #4742 - New InputGroup Component
parent
bba5d21060
commit
5d8babd349
|
@ -0,0 +1,15 @@
|
|||
<script>
|
||||
import BaseComponent from 'primevue/basecomponent';
|
||||
import InputGroupStyle from 'primevue/inputgroup/style';
|
||||
|
||||
export default {
|
||||
name: 'BaseInputGroup',
|
||||
extends: BaseComponent,
|
||||
style: InputGroupStyle,
|
||||
provide() {
|
||||
return {
|
||||
$parentInstance: this
|
||||
};
|
||||
}
|
||||
};
|
||||
</script>
|
|
@ -0,0 +1,114 @@
|
|||
/**
|
||||
*
|
||||
* InputGroup displays text, icon, buttons and other content can be grouped next to an input.
|
||||
*
|
||||
* [Live Demo](https://www.primevue.org/inputgroup/)
|
||||
*
|
||||
* @module inputgroup
|
||||
*
|
||||
*/
|
||||
import { VNode } from 'vue';
|
||||
import { ComponentHooks } from '../basecomponent';
|
||||
import { PassThroughOptions } from '../passthrough';
|
||||
import { ClassComponent, GlobalComponentConstructor, PassThrough } from '../ts-helpers';
|
||||
|
||||
export declare type InputGroupPassThroughOptionType = InputGroupPassThroughAttributes | ((options: InputGroupPassThroughMethodOptions) => InputGroupPassThroughAttributes | string) | string | null | undefined;
|
||||
|
||||
/**
|
||||
* Custom passthrough(pt) option method.
|
||||
*/
|
||||
export interface InputGroupPassThroughMethodOptions {
|
||||
/**
|
||||
* Defines instance.
|
||||
*/
|
||||
instance: any;
|
||||
/**
|
||||
* Defines passthrough(pt) options in global config.
|
||||
*/
|
||||
global: object | undefined;
|
||||
}
|
||||
|
||||
/**
|
||||
* Custom passthrough(pt) options.
|
||||
* @see {@link InputGroupProps.pt}
|
||||
*/
|
||||
export interface InputGroupPassThroughOptions {
|
||||
/**
|
||||
* Used to pass attributes to the root's DOM element.
|
||||
*/
|
||||
root?: InputGroupPassThroughOptionType;
|
||||
/**
|
||||
* Used to manage all lifecycle hooks
|
||||
* @see {@link BaseComponent.ComponentHooks}
|
||||
*/
|
||||
hooks?: ComponentHooks;
|
||||
}
|
||||
|
||||
/**
|
||||
* Custom passthrough attributes for each DOM elements
|
||||
*/
|
||||
export interface InputGroupPassThroughAttributes {
|
||||
[key: string]: any;
|
||||
}
|
||||
|
||||
/**
|
||||
* Defines valid properties in InputGroup component.
|
||||
*/
|
||||
export interface InputGroupProps {
|
||||
/**
|
||||
* Used to pass attributes to DOM elements inside the component.
|
||||
* @type {InputGroupPassThroughOptions}
|
||||
*/
|
||||
pt?: PassThrough<InputGroupPassThroughOptions>;
|
||||
/**
|
||||
* Used to configure passthrough(pt) options of the component.
|
||||
* @type {PassThroughOptions}
|
||||
*/
|
||||
ptOptions?: PassThroughOptions;
|
||||
/**
|
||||
* When enabled, it removes component related styles in the core.
|
||||
* @defaultValue false
|
||||
*/
|
||||
unstyled?: boolean;
|
||||
}
|
||||
|
||||
/**
|
||||
* Defines valid slots in InputGroup component.
|
||||
*/
|
||||
export interface InputGroupSlots {
|
||||
/**
|
||||
* Custom default template.
|
||||
*/
|
||||
default(): VNode[];
|
||||
/**
|
||||
* Dynamic content template.
|
||||
* @todo
|
||||
*/
|
||||
[key: string]: (node: any) => VNode[];
|
||||
}
|
||||
|
||||
/**
|
||||
* Defines valid emits in InputGroup component.
|
||||
*/
|
||||
export interface InputGroupEmits {}
|
||||
|
||||
/**
|
||||
* **PrimeVue - InputGroup**
|
||||
*
|
||||
* _InputGroup displays text, icon, buttons and other content can be grouped next to an input._
|
||||
*
|
||||
* [Live Demo](https://www.primevue.org/inputgroup/)
|
||||
* --- ---
|
||||
* 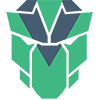
|
||||
*
|
||||
* @group Component
|
||||
*/
|
||||
declare class InputGroup extends ClassComponent<InputGroupProps, InputGroupSlots, InputGroupEmits> {}
|
||||
|
||||
declare module '@vue/runtime-core' {
|
||||
interface GlobalComponents {
|
||||
InputGroup: GlobalComponentConstructor<InputGroup>;
|
||||
}
|
||||
}
|
||||
|
||||
export default InputGroup;
|
|
@ -0,0 +1,14 @@
|
|||
<template>
|
||||
<div :class="cx('root')" v-bind="ptm('root')" data-pc-name="inputgroup">
|
||||
<slot />
|
||||
</div>
|
||||
</template>
|
||||
|
||||
<script>
|
||||
import BaseInputGroup from './BaseInputGroup.vue';
|
||||
|
||||
export default {
|
||||
name: 'InputGroup',
|
||||
extends: BaseInputGroup
|
||||
};
|
||||
</script>
|
|
@ -0,0 +1,9 @@
|
|||
{
|
||||
"main": "./inputgroup.cjs.js",
|
||||
"module": "./inputgroup.esm.js",
|
||||
"unpkg": "./inputgroup.min.js",
|
||||
"types": "./InputGroup.d.ts",
|
||||
"browser": {
|
||||
"./sfc": "./InputGroup.vue"
|
||||
}
|
||||
}
|
|
@ -0,0 +1,3 @@
|
|||
import { BaseStyle } from '../../base/style';
|
||||
|
||||
export interface InputGroupStyle extends BaseStyle {}
|
|
@ -0,0 +1,10 @@
|
|||
import BaseStyle from 'primevue/base/style';
|
||||
|
||||
const classes = {
|
||||
root: 'p-inputgroup'
|
||||
};
|
||||
|
||||
export default BaseStyle.extend({
|
||||
name: 'inputgroup',
|
||||
classes
|
||||
});
|
|
@ -0,0 +1,6 @@
|
|||
{
|
||||
"main": "./inputgroupstyle.cjs.js",
|
||||
"module": "./inputgroupstyle.esm.js",
|
||||
"unpkg": "./inputgroupstyle.min.js",
|
||||
"types": "./InputGroupStyle.d.ts"
|
||||
}
|
|
@ -0,0 +1,15 @@
|
|||
<script>
|
||||
import BaseComponent from 'primevue/basecomponent';
|
||||
import InputGroupAddonStyle from 'primevue/inputgroupaddon/style';
|
||||
|
||||
export default {
|
||||
name: 'BaseInputGroupAddon',
|
||||
extends: BaseComponent,
|
||||
style: InputGroupAddonStyle,
|
||||
provide() {
|
||||
return {
|
||||
$parentInstance: this
|
||||
};
|
||||
}
|
||||
};
|
||||
</script>
|
|
@ -0,0 +1,114 @@
|
|||
/**
|
||||
*
|
||||
* InputGroupAddon displays text, icon, buttons and other content can be grouped next to an input.
|
||||
*
|
||||
* [Live Demo](https://www.primevue.org/inputgroup/)
|
||||
*
|
||||
* @module inputgroupaddon
|
||||
*
|
||||
*/
|
||||
import { VNode } from 'vue';
|
||||
import { ComponentHooks } from '../basecomponent';
|
||||
import { PassThroughOptions } from '../passthrough';
|
||||
import { ClassComponent, GlobalComponentConstructor, PassThrough } from '../ts-helpers';
|
||||
|
||||
export declare type InputGroupPassThroughOptionType = InputGroupPassThroughAttributes | ((options: InputGroupPassThroughMethodOptions) => InputGroupPassThroughAttributes | string) | string | null | undefined;
|
||||
|
||||
/**
|
||||
* Custom passthrough(pt) option method.
|
||||
*/
|
||||
export interface InputGroupPassThroughMethodOptions {
|
||||
/**
|
||||
* Defines instance.
|
||||
*/
|
||||
instance: any;
|
||||
/**
|
||||
* Defines passthrough(pt) options in global config.
|
||||
*/
|
||||
global: object | undefined;
|
||||
}
|
||||
|
||||
/**
|
||||
* Custom passthrough(pt) options.
|
||||
* @see {@link InputGroupProps.pt}
|
||||
*/
|
||||
export interface InputGroupPassThroughOptions {
|
||||
/**
|
||||
* Used to pass attributes to the root's DOM element.
|
||||
*/
|
||||
root?: InputGroupPassThroughOptionType;
|
||||
/**
|
||||
* Used to manage all lifecycle hooks
|
||||
* @see {@link BaseComponent.ComponentHooks}
|
||||
*/
|
||||
hooks?: ComponentHooks;
|
||||
}
|
||||
|
||||
/**
|
||||
* Custom passthrough attributes for each DOM elements
|
||||
*/
|
||||
export interface InputGroupPassThroughAttributes {
|
||||
[key: string]: any;
|
||||
}
|
||||
|
||||
/**
|
||||
* Defines valid properties in InputGroupAddon component.
|
||||
*/
|
||||
export interface InputGroupProps {
|
||||
/**
|
||||
* Used to pass attributes to DOM elements inside the component.
|
||||
* @type {InputGroupPassThroughOptions}
|
||||
*/
|
||||
pt?: PassThrough<InputGroupPassThroughOptions>;
|
||||
/**
|
||||
* Used to configure passthrough(pt) options of the component.
|
||||
* @type {PassThroughOptions}
|
||||
*/
|
||||
ptOptions?: PassThroughOptions;
|
||||
/**
|
||||
* When enabled, it removes component related styles in the core.
|
||||
* @defaultValue false
|
||||
*/
|
||||
unstyled?: boolean;
|
||||
}
|
||||
|
||||
/**
|
||||
* Defines valid slots in InputGroupAddon component.
|
||||
*/
|
||||
export interface InputGroupSlots {
|
||||
/**
|
||||
* Custom default template.
|
||||
*/
|
||||
default(): VNode[];
|
||||
/**
|
||||
* Dynamic content template.
|
||||
* @todo
|
||||
*/
|
||||
[key: string]: (node: any) => VNode[];
|
||||
}
|
||||
|
||||
/**
|
||||
* Defines valid emits in InputGroupAddon component.
|
||||
*/
|
||||
export interface InputGroupEmits {}
|
||||
|
||||
/**
|
||||
* **PrimeVue - InputGroupAddon**
|
||||
*
|
||||
* _InputGroup displays text, icon, buttons and other content can be grouped next to an input._
|
||||
*
|
||||
* [Live Demo](https://www.primevue.org/inputgroup/)
|
||||
* --- ---
|
||||
* 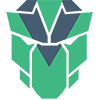
|
||||
*
|
||||
* @group Component
|
||||
*/
|
||||
declare class InputGroupAddon extends ClassComponent<InputGroupProps, InputGroupSlots, InputGroupEmits> {}
|
||||
|
||||
declare module '@vue/runtime-core' {
|
||||
interface GlobalComponents {
|
||||
InputGroupAddon: GlobalComponentConstructor<InputGroupAddon>;
|
||||
}
|
||||
}
|
||||
|
||||
export default InputGroupAddon;
|
|
@ -0,0 +1,14 @@
|
|||
<template>
|
||||
<div :class="cx('root')" v-bind="ptm('root')" data-pc-name="inputgroupaddon">
|
||||
<slot />
|
||||
</div>
|
||||
</template>
|
||||
|
||||
<script>
|
||||
import BaseInputGroupAddon from './BaseInputGroupAddon.vue';
|
||||
|
||||
export default {
|
||||
name: 'InputGroupAddon',
|
||||
extends: BaseInputGroupAddon
|
||||
};
|
||||
</script>
|
|
@ -0,0 +1,9 @@
|
|||
{
|
||||
"main": "./inputgroupaddon.cjs.js",
|
||||
"module": "./inputgrouaddonp.esm.js",
|
||||
"unpkg": "./inputgroupaddon.min.js",
|
||||
"types": "./InputGroupAddon.d.ts",
|
||||
"browser": {
|
||||
"./sfc": "./InputGroupAddon.vue"
|
||||
}
|
||||
}
|
|
@ -0,0 +1,3 @@
|
|||
import { BaseStyle } from '../../base/style';
|
||||
|
||||
export interface InputGroupAddonStyle extends BaseStyle {}
|
|
@ -0,0 +1,10 @@
|
|||
import BaseStyle from 'primevue/base/style';
|
||||
|
||||
const classes = {
|
||||
root: 'p-inputgroup-addon'
|
||||
};
|
||||
|
||||
export default BaseStyle.extend({
|
||||
name: 'inputgroupaddon',
|
||||
classes
|
||||
});
|
|
@ -0,0 +1,6 @@
|
|||
{
|
||||
"main": "./inputgroupaddonstyle.cjs.js",
|
||||
"module": "./inputgroupaddonstyle.esm.js",
|
||||
"unpkg": "./inputgroupaddonstyle.min.js",
|
||||
"types": "./InputGroupAddonStyle.d.ts"
|
||||
}
|
|
@ -7,6 +7,8 @@ const form = [
|
|||
'ColorPicker',
|
||||
'Dropdown',
|
||||
'Editor',
|
||||
'InputGroup',
|
||||
'InputGroupAddon',
|
||||
'InputMask',
|
||||
'InputNumber',
|
||||
'InputSwitch',
|
||||
|
|
|
@ -48,6 +48,8 @@ const STYLE_ALIAS = {
|
|||
'primevue/image/style': path.resolve(__dirname, './components/lib/image/style/ImageStyle.js'),
|
||||
'primevue/inlinemessage/style': path.resolve(__dirname, './components/lib/inlinemessage/style/InlineMessageStyle.js'),
|
||||
'primevue/inplace/style': path.resolve(__dirname, './components/lib/inplace/style/InplaceStyle.js'),
|
||||
'primevue/inputgroup/style': path.resolve(__dirname, './components/lib/inputgroup/style/InputGroupStyle.js'),
|
||||
'primevue/inputgroupaddon/style': path.resolve(__dirname, './components/lib/inputgroupaddon/style/InputGroupAddonStyle.js'),
|
||||
'primevue/inputmask/style': path.resolve(__dirname, './components/lib/inputmask/style/InputMaskStyle.js'),
|
||||
'primevue/inputnumber/style': path.resolve(__dirname, './components/lib/inputnumber/style/InputNumberStyle.js'),
|
||||
'primevue/inputswitch/style': path.resolve(__dirname, './components/lib/inputswitch/style/InputSwitchStyle.js'),
|
||||
|
|
|
@ -112,6 +112,8 @@ const CORE_STYLE_DEPENDENCIES = {
|
|||
'primevue/image/style': 'primevue.image.style',
|
||||
'primevue/inlinemessage/style': 'primevue.inlinemessage.style',
|
||||
'primevue/inplace/style': 'primevue.inplace.style',
|
||||
'primevue/inputgroup/style': 'primevue.inputgroup.style',
|
||||
'primevue/inputgroupaddon/style': 'primevue.inputgroupaddon.style',
|
||||
'primevue/inputmask/style': 'primevue.inputmask.style',
|
||||
'primevue/inputnumber/style': 'primevue.inputnumber.style',
|
||||
'primevue/inputswitch/style': 'primevue.inputswitch.style',
|
||||
|
|
Loading…
Reference in New Issue