diff --git a/components/lib/stepper/BaseStepper.vue b/components/lib/stepper/BaseStepper.vue
new file mode 100644
index 000000000..85de0ac0d
--- /dev/null
+++ b/components/lib/stepper/BaseStepper.vue
@@ -0,0 +1,29 @@
+
diff --git a/components/lib/stepper/Stepper.d.ts b/components/lib/stepper/Stepper.d.ts
new file mode 100644
index 000000000..a94ec129b
--- /dev/null
+++ b/components/lib/stepper/Stepper.d.ts
@@ -0,0 +1,191 @@
+/**
+ *
+ * Stepper is a component that streamlines a wizard-like workflow, organizing content into coherent steps and visually guiding users through a numbered progression in a multi-step process.
+ *
+ * [Live Demo](https://www.primevue.org/stepper/)
+ *
+ * @module stepper
+ *
+ */
+import { ComponentHooks } from '../basecomponent';
+import { PassThroughOptions } from '../passthrough';
+import { StepperPanelPassThroughOptionType } from '../stepperpanel';
+import { ClassComponent, GlobalComponentConstructor, PassThrough } from '../ts-helpers';
+
+export declare type StepperPassThroughOptionType = StepperPassThroughAttributes | ((options: StepperPassThroughMethodOptions) => StepperPassThroughAttributes | string) | string | null | undefined;
+
+/**
+ * Custom passthrough(pt) option method.
+ */
+export interface StepperPassThroughMethodOptions {
+ /**
+ * Defines instance.
+ */
+ instance: any;
+ /**
+ * Defines valid properties.
+ */
+ props: StepperProps;
+ /**
+ * Defines current inline state.
+ */
+ state: StepperState;
+ /**
+ * Defines valid attributes.
+ */
+ attrs: any;
+ /**
+ * Defines parent options.
+ */
+ parent: any;
+ /**
+ * Defines passthrough(pt) options in global config.
+ */
+ global: object | undefined;
+}
+
+/**
+ * Custom passthrough(pt) options.
+ * @see {@link StepperProps.pt}
+ */
+export interface StepperPassThroughOptions {
+ /**
+ * Used to pass attributes to the root's DOM element.
+ */
+ root?: StepperPassThroughOptionType;
+ /**
+ * Used to pass attributes to the nav container's DOM element.
+ */
+ navContainer?: StepperPassThroughOptionType;
+ /**
+ * Used to pass attributes to the nav's DOM element.
+ */
+ nav?: StepperPassThroughOptionType;
+ /**
+ * Used to pass attributes to the panel container's DOM element.
+ */
+ panelContainer?: StepperPassThroughOptionType;
+ /**
+ * Used to pass attributes to the end handler's DOM element.
+ */
+ stepperpanel?: StepperPanelPassThroughOptionType;
+ /**
+ * Used to manage all lifecycle hooks.
+ * @see {@link BaseComponent.ComponentHooks}
+ */
+ hooks?: ComponentHooks;
+}
+
+/**
+ * Custom passthrough attributes for each DOM elements
+ */
+export interface StepperPassThroughAttributes {
+ [key: string]: any;
+}
+
+/**
+ * Defines current inline state in Stepper component.
+ */
+export interface StepperState {
+ /**
+ * Current active index state.
+ */
+ d_activeStep: number;
+ /**
+ * Unique id for the Stepper component.
+ */
+ id: string;
+}
+
+/**
+ * Custom tab change event.
+ * @see {@link StepperEmits['step-change']}
+ */
+export interface StepperChangeEvent {
+ /**
+ * Browser event
+ */
+ originalEvent: Event;
+ /**
+ * Index of the selected stepper panel
+ */
+ index: number;
+}
+
+/**
+ * Defines valid properties in Stepper component.
+ */
+export interface StepperProps {
+ /**
+ * Active step index of stepper.
+ * @defaultValue 0
+ */
+ activeStep?: number | undefined;
+ /**
+ * Orientation of the stepper.
+ * @defaultValue horizontal
+ */
+ orientation?: 'horizontal' | 'vertical' | undefined;
+ /**
+ * Whether the steps are clickable or not.
+ * @defaultValue false
+ */
+ linear?: boolean | undefined;
+ /**
+ * Used to pass attributes to DOM elements inside the component.
+ * @type {StepperPassThroughOptions}
+ */
+ pt?: PassThrough;
+ /**
+ * Used to configure passthrough(pt) options of the component.
+ * @type {PassThroughOptions}
+ */
+ ptOptions?: PassThroughOptions;
+ /**
+ * When enabled, it removes component related styles in the core.
+ * @defaultValue false
+ */
+ unstyled?: boolean;
+}
+
+/**
+ * Defines valid slots in Stepper component.
+ */
+export interface StepperSlots {}
+
+/**
+ * Defines valid emits in Stepper component.
+ */
+export interface StepperEmits {
+ /**
+ * Emitted when the value changes.
+ * @param {number | number[]} value - New value.
+ */
+ 'update:activeStep'(value: number): void;
+ /**
+ * Callback to invoke when an active panel is changed.
+ */
+ 'step-change'(event: StepperChangeEvent): void;
+}
+
+/**
+ * **PrimeVue - Stepper**
+ *
+ * _Stepper is a component that streamlines a wizard-like workflow, organizing content into coherent steps and visually guiding users through a numbered progression in a multi-step process._
+ *
+ * [Live Demo](https://www.primevue.org/stepper/)
+ * --- ---
+ * 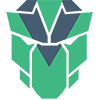
+ *
+ * @group Component
+ *
+ */
+declare class Stepper extends ClassComponent {}
+
+declare module '@vue/runtime-core' {
+ interface GlobalComponents {
+ Stepper: GlobalComponentConstructor;
+ }
+}
+
+export default Stepper;
diff --git a/components/lib/stepper/Stepper.vue b/components/lib/stepper/Stepper.vue
new file mode 100644
index 000000000..56e81e49c
--- /dev/null
+++ b/components/lib/stepper/Stepper.vue
@@ -0,0 +1,269 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/components/lib/stepper/StepperContent.vue b/components/lib/stepper/StepperContent.vue
new file mode 100644
index 000000000..095aca2e5
--- /dev/null
+++ b/components/lib/stepper/StepperContent.vue
@@ -0,0 +1,47 @@
+
+
+
+
+
+
+
+
diff --git a/components/lib/stepper/StepperHeader.vue b/components/lib/stepper/StepperHeader.vue
new file mode 100644
index 000000000..0986ed081
--- /dev/null
+++ b/components/lib/stepper/StepperHeader.vue
@@ -0,0 +1,30 @@
+
+
+
+
+
+
diff --git a/components/lib/stepper/StepperSeparator.vue b/components/lib/stepper/StepperSeparator.vue
new file mode 100644
index 000000000..e071b1bec
--- /dev/null
+++ b/components/lib/stepper/StepperSeparator.vue
@@ -0,0 +1,23 @@
+
+
+
+
+
+
diff --git a/components/lib/stepper/package.json b/components/lib/stepper/package.json
new file mode 100644
index 000000000..9bd5df35d
--- /dev/null
+++ b/components/lib/stepper/package.json
@@ -0,0 +1,9 @@
+{
+ "main": "./stepper.cjs.js",
+ "module": "./stepper.esm.js",
+ "unpkg": "./stepper.min.js",
+ "types": "./Stepper.d.ts",
+ "browser": {
+ "./sfc": "./Stepper.vue"
+ }
+}
diff --git a/components/lib/stepper/style/StepperStyle.d.ts b/components/lib/stepper/style/StepperStyle.d.ts
new file mode 100644
index 000000000..91b9eb2ff
--- /dev/null
+++ b/components/lib/stepper/style/StepperStyle.d.ts
@@ -0,0 +1,3 @@
+import { BaseStyle } from '../../base/style';
+
+export interface StepperStyle extends BaseStyle {}
diff --git a/components/lib/stepper/style/StepperStyle.js b/components/lib/stepper/style/StepperStyle.js
new file mode 100644
index 000000000..11e7ce872
--- /dev/null
+++ b/components/lib/stepper/style/StepperStyle.js
@@ -0,0 +1,46 @@
+import BaseStyle from 'primevue/base/style';
+
+const classes = {
+ root: ({ props }) => [
+ 'p-stepper p-component',
+ {
+ 'p-stepper-horizontal': props.orientation === 'horizontal',
+ 'p-stepper-vertical': props.orientation === 'vertical',
+ 'p-readonly': props.linear
+ }
+ ],
+ navContainer: 'p-stepper-nav-container',
+ nav: 'p-stepper-nav',
+ stepper: {
+ header: ({ instance, step, index }) => [
+ 'p-stepper-header',
+ {
+ 'p-highlight': instance.isStepActive(index),
+ 'p-disabled': instance.isItemDisabled(step, index)
+ }
+ ],
+ action: 'p-stepper-action',
+ number: 'p-stepper-number',
+ title: 'p-stepper-title',
+ separator: 'p-stepper-separator',
+ toggleableContent: 'p-stepper-toggleable-content',
+ content: ({ props }) => [
+ 'p-stepper-content',
+ {
+ 'p-toggleable-content': props.orientation === 'vertical'
+ }
+ ]
+ },
+ panelContainer: 'p-stepper-panels',
+ panel: ({ instance, props, index }) => [
+ 'p-stepper-panel',
+ {
+ 'p-stepper-panel-active': props.orientation === 'vertical' && instance.isStepActive(index)
+ }
+ ]
+};
+
+export default BaseStyle.extend({
+ name: 'stepper',
+ classes
+});
diff --git a/components/lib/stepper/style/package.json b/components/lib/stepper/style/package.json
new file mode 100644
index 000000000..0919bf966
--- /dev/null
+++ b/components/lib/stepper/style/package.json
@@ -0,0 +1,6 @@
+{
+ "main": "./stepperpanel.cjs.js",
+ "module": "./stepperpanel.esm.js",
+ "unpkg": "./stepperpanel.min.js",
+ "types": "./StepperPanel.d.ts"
+}
diff --git a/components/lib/stepperpanel/BaseStepperPanel.vue b/components/lib/stepperpanel/BaseStepperPanel.vue
new file mode 100644
index 000000000..4262bb43e
--- /dev/null
+++ b/components/lib/stepperpanel/BaseStepperPanel.vue
@@ -0,0 +1,18 @@
+
diff --git a/components/lib/stepperpanel/StepperPanel.d.ts b/components/lib/stepperpanel/StepperPanel.d.ts
new file mode 100644
index 000000000..8d477c10a
--- /dev/null
+++ b/components/lib/stepperpanel/StepperPanel.d.ts
@@ -0,0 +1,274 @@
+/**
+ *
+ * StepperPanel is a helper component for StepperPanel component.
+ *
+ * [Live Demo](https://www.primevue.org/stepper/)
+ *
+ * @module stepperpanel
+ *
+ */
+import { TransitionProps, VNode } from 'vue';
+import { ComponentHooks } from '../basecomponent';
+import { PassThroughOptions } from '../passthrough';
+import { ClassComponent, GlobalComponentConstructor, PassThrough } from '../ts-helpers';
+
+export declare type StepperPanelPassThroughOptionType = StepperPanelPassThroughAttributes | ((options: StepperPanelPassThroughMethodOptions) => StepperPanelPassThroughAttributes | string) | string | null | undefined;
+
+export declare type StepperPanelPassThroughTransitionType = TransitionProps | ((options: StepperPanelPassThroughMethodOptions) => TransitionProps) | undefined;
+
+/**
+ * Custom passthrough(pt) option method.
+ */
+export interface StepperPanelPassThroughMethodOptions {
+ /**
+ * Defines instance.
+ */
+ instance: any;
+ /**
+ * Defines valid properties.
+ */
+ props: StepperPanelProps;
+ /**
+ * Defines current options.
+ */
+ context: StepperPanelContext;
+ /**
+ * Defines valid attributes.
+ */
+ attrs: any;
+ /**
+ * Defines parent options.
+ */
+ parent: any;
+ /**
+ * Defines passthrough(pt) options in global config.
+ */
+ global: object | undefined;
+}
+
+/**
+ * Custom passthrough(pt) options.
+ * @see {@link StepperPanelProps.pt}
+ */
+export interface StepperPanelPassThroughOptions {
+ /**
+ * Used to pass attributes to the root's DOM element.
+ */
+ root?: StepperPanelPassThroughOptionType;
+ /**
+ * Used to pass attributes to the header's DOM element.
+ */
+ header?: StepperPanelPassThroughOptionType;
+ /**
+ * Used to pass attributes to the action's DOM element.
+ */
+ action?: StepperPanelPassThroughOptionType;
+ /**
+ * Used to pass attributes to the number's DOM element.
+ */
+ number?: StepperPanelPassThroughOptionType;
+ /**
+ * Used to pass attributes to the title's DOM element.
+ */
+ title?: StepperPanelPassThroughOptionType;
+ /**
+ * Used to pass attributes to the separator's DOM element.
+ */
+ separator?: StepperPanelPassThroughOptionType;
+ /**
+ * Used to pass attributes to the content's DOM element.
+ */
+ content?: StepperPanelPassThroughOptionType;
+ /**
+ * Used to pass attributes to the panel's DOM element.
+ */
+ panel?: StepperPanelPassThroughOptionType;
+ /**
+ * Used to pass attributes to the toggleable content's DOM element.
+ */
+ toggleableContent?: StepperPanelPassThroughOptionType;
+ /**
+ * Used to control Vue Transition API.
+ */
+ transition?: StepperPanelPassThroughTransitionType;
+ /**
+ * Used to manage all lifecycle hooks.
+ * @see {@link BaseComponent.ComponentHooks}
+ */
+ hooks?: ComponentHooks;
+}
+
+export interface StepperPanelPassThroughAttributes {
+ [key: string]: any;
+}
+
+/**
+ * Defines valid properties in StepperPanel component.
+ */
+export interface StepperPanelProps {
+ /**
+ * Orientation of tab headers.
+ */
+ header?: string | undefined;
+ /**
+ * Used to pass attributes to DOM elements inside the component.
+ * @type {StepperPanelPassThroughOptions}
+ */
+ pt?: PassThrough;
+ /**
+ * Used to configure passthrough(pt) options of the component.
+ * @type {PassThroughOptions}
+ */
+ ptOptions?: PassThroughOptions;
+}
+
+/**
+ * Defines current options in StepperPanel component.
+ */
+export interface StepperPanelContext {
+ /**
+ * Current index of the stepperpanel.
+ */
+ index: number;
+ /**
+ * Count of stepperpanels
+ */
+ count: number;
+ /**
+ * Whether the stepperpanel is first.
+ */
+ first: boolean;
+ /**
+ * Whether the stepperpanel is last.
+ */
+ last: boolean;
+ /**
+ * Whether the stepperpanel is active.
+ */
+ active: boolean;
+ /**
+ * Whether the stepperpanel is highlighted.
+ */
+ highlighted: boolean;
+ /**
+ * Whether the stepperpanel is disabled.
+ */
+ disabled: boolean;
+}
+
+/**
+ * Defines valid slots in StepperPanel slots.
+ */
+export interface StepperPanelSlots {
+ /**
+ * Custom content template.
+ */
+ default(): VNode[];
+ /**
+ * Custom header template.
+ */
+ header(scope: {
+ /**
+ * Index of the stepperpanel
+ */
+ index: number;
+ /**
+ * Current active state of the stepperpanel
+ */
+ active: boolean;
+ /**
+ * Current highlighted state of the stepperpanel
+ */
+ highlighted: boolean;
+ /**
+ * Style class of the stepperpanel
+ */
+ class: string;
+ /**
+ * Header click function.
+ * @param {Event} event - Browser event
+ */
+ clickCallback: (event: Event) => void;
+ }): VNode[];
+ /**
+ * Custom content template.
+ */
+ content(scope: {
+ /**
+ * Index of the stepperpanel
+ */
+ index: number;
+ /**
+ * Current active state of the stepperpanel
+ */
+ active: boolean;
+ /**
+ * Current highlighted state of the stepperpanel
+ */
+ highlighted: boolean;
+ /**
+ * Style class of the stepperpanel
+ */
+ class: string;
+ /**
+ * Content click function.
+ * @param {Event} event - Browser event
+ */
+ clickCallback: (event: Event) => void;
+ /**
+ * Content previous panel click function.
+ * @param {Event} event - Browser event
+ */
+ prevCallback: (event: Event) => void;
+ /**
+ * Content next panel click function.
+ * @param {Event} event - Browser event
+ */
+ nextCallback: (event: Event) => void;
+ }): VNode[];
+ /**
+ * Custom separator template.
+ */
+ separator(scope: {
+ /**
+ * Index of the stepperpanel
+ */
+ index: number;
+ /**
+ * Current active state of the stepperpanel
+ */
+ active: boolean;
+ /**
+ * Current highlighted state of the stepperpanel
+ */
+ highlighted: boolean;
+ /**
+ * Style class of the stepperpanel
+ */
+ class: string;
+ }): VNode[];
+}
+
+export interface StepperPanelEmits {}
+
+/**
+ * **PrimeVue - StepperPanel**
+ *
+ * _StepperPanel is a helper component for Stepper component._
+ *
+ * [Live Demo](https://www.primevue.org/stepper/)
+ * --- ---
+ * 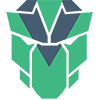
+ *
+ * @group Component
+ *
+ */
+declare class StepperPanel extends ClassComponent {}
+
+declare module '@vue/runtime-core' {
+ interface GlobalComponents {
+ StepperPanel: GlobalComponentConstructor;
+ }
+}
+
+export default StepperPanel;
diff --git a/components/lib/stepperpanel/StepperPanel.vue b/components/lib/stepperpanel/StepperPanel.vue
new file mode 100644
index 000000000..47865642f
--- /dev/null
+++ b/components/lib/stepperpanel/StepperPanel.vue
@@ -0,0 +1,12 @@
+
+
+
+
+
diff --git a/components/lib/stepperpanel/package.json b/components/lib/stepperpanel/package.json
new file mode 100644
index 000000000..a0dc90ecf
--- /dev/null
+++ b/components/lib/stepperpanel/package.json
@@ -0,0 +1,9 @@
+{
+ "main": "./stepperpanel.cjs.js",
+ "module": "./stepperpanel.esm.js",
+ "unpkg": "./stepperpanel.min.js",
+ "types": "./StepperPanel.d.ts",
+ "browser": {
+ "./sfc": "./StepperPanel.vue"
+ }
+}
diff --git a/components/lib/stepperpanel/style/StepperPanelStyle.d.ts b/components/lib/stepperpanel/style/StepperPanelStyle.d.ts
new file mode 100644
index 000000000..7738f1455
--- /dev/null
+++ b/components/lib/stepperpanel/style/StepperPanelStyle.d.ts
@@ -0,0 +1,3 @@
+import { BaseStyle } from '../../base/style';
+
+export interface StepperPanelStyle extends BaseStyle {}
diff --git a/components/lib/stepperpanel/style/StepperPanelStyle.js b/components/lib/stepperpanel/style/StepperPanelStyle.js
new file mode 100644
index 000000000..ff8b4c563
--- /dev/null
+++ b/components/lib/stepperpanel/style/StepperPanelStyle.js
@@ -0,0 +1 @@
+export default {};
diff --git a/components/lib/stepperpanel/style/package.json b/components/lib/stepperpanel/style/package.json
new file mode 100644
index 000000000..dc26b2f47
--- /dev/null
+++ b/components/lib/stepperpanel/style/package.json
@@ -0,0 +1,6 @@
+{
+ "main": "./stepperpanelstyle.cjs.js",
+ "module": "./stepperpanelstyle.esm.js",
+ "unpkg": "./stepperpanelstyle.min.js",
+ "types": "./StepperPanelStyle.d.ts"
+}