Merge branch 'master' of https://github.com/primefaces/primevue
commit
6582826b81
|
@ -1,7 +1,19 @@
|
|||
/**
|
||||
*
|
||||
* ConfirmPopup displays a confirmation overlay displayed relatively to its target.
|
||||
*
|
||||
* [Live Demo](https://www.primevue.org/confirmpopup)
|
||||
*
|
||||
* @module confirmpopup
|
||||
*
|
||||
*/
|
||||
import { VNode } from 'vue';
|
||||
import { ClassComponent, GlobalComponentConstructor } from '../ts-helpers';
|
||||
import { ConfirmationOptions } from '../confirmationoptions';
|
||||
import { ClassComponent, GlobalComponentConstructor } from '../ts-helpers';
|
||||
|
||||
/**
|
||||
* Defines valid properties in ConfirmPopup component.
|
||||
*/
|
||||
export interface ConfirmPopupProps {
|
||||
/**
|
||||
* Optional key to match the key of the confirmation, useful to target a specific confirm dialog instance.
|
||||
|
@ -9,17 +21,34 @@ export interface ConfirmPopupProps {
|
|||
group?: string;
|
||||
}
|
||||
|
||||
/**
|
||||
* Defines valid slots in ConfirmPopup component.
|
||||
*/
|
||||
export interface ConfirmPopupSlots {
|
||||
/**
|
||||
* Custom message template.
|
||||
* @param {Object} scope - message slot's params.
|
||||
*/
|
||||
message: (scope: { message: ConfirmationOptions }) => VNode[];
|
||||
message(scope: { message: ConfirmationOptions }): VNode[];
|
||||
}
|
||||
|
||||
export declare type ConfirmPopupEmits = {};
|
||||
/**
|
||||
* Defines valid emits in ConfirmPopup component.
|
||||
*/
|
||||
export interface ConfirmPopupEmits {}
|
||||
|
||||
declare class ConfirmPopup extends ClassComponent<ConfirmPopupProps, ConfirmPopupSlots, ConfirmPopupEmits> {}
|
||||
/**
|
||||
* **PrimeVue - ConfirmPopup**
|
||||
*
|
||||
* _ConfirmPopup displays a confirmation overlay displayed relatively to its target._
|
||||
*
|
||||
* [Live Demo](https://www.primevue.org/confirmpopup/)
|
||||
* --- ---
|
||||
* 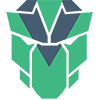
|
||||
*
|
||||
* @group Component
|
||||
*/
|
||||
export declare class ConfirmPopup extends ClassComponent<ConfirmPopupProps, ConfirmPopupSlots, ConfirmPopupEmits> {}
|
||||
|
||||
declare module '@vue/runtime-core' {
|
||||
interface GlobalComponents {
|
||||
|
@ -27,18 +56,4 @@ declare module '@vue/runtime-core' {
|
|||
}
|
||||
}
|
||||
|
||||
/**
|
||||
*
|
||||
* ConfirmPopup displays a confirmation overlay displayed relatively to its target.
|
||||
*
|
||||
* Helper API:
|
||||
*
|
||||
* - Confirmation API
|
||||
* - ConfirmationService
|
||||
*
|
||||
* Demos:
|
||||
*
|
||||
* - [ConfirmPopup](https://www.primefaces.org/primevue/confirmpopup)
|
||||
*
|
||||
*/
|
||||
export default ConfirmPopup;
|
||||
|
|
|
@ -1,10 +1,18 @@
|
|||
/**
|
||||
*
|
||||
* Dialog is a container to display content in an overlay window.
|
||||
*
|
||||
* [Live Demo](https://www.primevue.org/dialog)
|
||||
*
|
||||
* @module dialog
|
||||
*
|
||||
*/
|
||||
import { HTMLAttributes, VNode } from 'vue';
|
||||
import { ClassComponent, GlobalComponentConstructor } from '../ts-helpers';
|
||||
|
||||
type DialogPositionType = 'center' | 'top' | 'bottom' | 'left' | 'right' | 'topleft' | 'topright' | 'bottomleft' | 'bottomright' | undefined;
|
||||
|
||||
type DialogAppendToType = 'body' | 'self' | string | undefined | HTMLElement;
|
||||
|
||||
/**
|
||||
* Custom breakpoint metadata.
|
||||
*/
|
||||
export interface DialogBreakpoints {
|
||||
/**
|
||||
* Breakpoint for responsive mode.
|
||||
|
@ -24,6 +32,9 @@ export interface DialogBreakpoints {
|
|||
[key: string]: string;
|
||||
}
|
||||
|
||||
/**
|
||||
* Defines valid properties in Dialog component.
|
||||
*/
|
||||
export interface DialogProps {
|
||||
/**
|
||||
* Title content of the dialog.
|
||||
|
@ -35,10 +46,12 @@ export interface DialogProps {
|
|||
footer?: string | undefined;
|
||||
/**
|
||||
* Specifies the visibility of the dialog.
|
||||
* @defaultValue false
|
||||
*/
|
||||
visible?: boolean | undefined;
|
||||
/**
|
||||
* Defines if background should be blocked when dialog is displayed.
|
||||
* @defaultValue false
|
||||
*/
|
||||
modal?: boolean | undefined;
|
||||
/**
|
||||
|
@ -55,150 +68,167 @@ export interface DialogProps {
|
|||
contentProps?: HTMLAttributes | undefined;
|
||||
/**
|
||||
* When enabled dialog is displayed in RTL direction.
|
||||
* @defaultValue false
|
||||
*/
|
||||
rtl?: boolean | undefined;
|
||||
/**
|
||||
* Adds a close icon to the header to hide the dialog.
|
||||
* Default value is true.
|
||||
* @defaultValue true
|
||||
*/
|
||||
closable?: boolean | undefined;
|
||||
/**
|
||||
* Specifies if clicking the modal background should hide the dialog.
|
||||
* @defaultValue false
|
||||
*/
|
||||
dismissableMask?: boolean | undefined;
|
||||
/**
|
||||
* Specifies if pressing escape key should hide the dialog.
|
||||
* Default value is true.
|
||||
* @defaultValue true
|
||||
*/
|
||||
closeOnEscape?: boolean | undefined;
|
||||
/**
|
||||
* Whether to show the header or not.
|
||||
* Default value is true.
|
||||
* @defaultValue true
|
||||
*/
|
||||
showHeader?: boolean | undefined;
|
||||
/**
|
||||
* Base zIndex value to use in layering.
|
||||
* Default value is 0.
|
||||
* @defaultValue 0
|
||||
*/
|
||||
baseZIndex?: number | undefined;
|
||||
/**
|
||||
* Whether to automatically manage layering.
|
||||
* Default value is true.
|
||||
* @defaultValue true
|
||||
*/
|
||||
autoZIndex?: boolean | undefined;
|
||||
/**
|
||||
* Position of the dialog, options are 'center', 'top', 'bottom', 'left', 'right', 'topleft', 'topright', 'bottomleft' or 'bottomright'.
|
||||
* @see DialogPositionType
|
||||
* Default value is 'center'.
|
||||
* Position of the dialog.
|
||||
* @defaultValue center
|
||||
*/
|
||||
position?: DialogPositionType;
|
||||
position?: 'center' | 'top' | 'bottom' | 'left' | 'right' | 'topleft' | 'topright' | 'bottomleft' | 'bottomright' | undefined;
|
||||
/**
|
||||
* Whether the dialog can be displayed full screen.
|
||||
* @defaultValue false
|
||||
*/
|
||||
maximizable?: boolean | undefined;
|
||||
/**
|
||||
* Object literal to define widths per screen size.
|
||||
* @see DialogBreakpoints
|
||||
*/
|
||||
breakpoints?: DialogBreakpoints;
|
||||
/**
|
||||
* Enables dragging to change the position using header.
|
||||
* Default value is true.
|
||||
* @defaultValue true
|
||||
*/
|
||||
draggable?: boolean | undefined;
|
||||
/**
|
||||
* Keeps dialog in the viewport when dragging.
|
||||
* Default value is true.
|
||||
* @defaultValue true
|
||||
*/
|
||||
keepInViewPort?: boolean | undefined;
|
||||
/**
|
||||
* Minimum value for the left coordinate of dialog in dragging.
|
||||
* Default value is 0.
|
||||
* @defaultValue 0.
|
||||
*/
|
||||
minX?: number | undefined;
|
||||
/**
|
||||
* Minimum value for the top coordinate of dialog in dragging.
|
||||
* Default value is 0.
|
||||
* @defaultValue 0
|
||||
*/
|
||||
minY?: number | undefined;
|
||||
/**
|
||||
* A valid query selector or an HTMLElement to specify where the dialog gets attached. Special keywords are 'body' for document body and 'self' for the element itself.
|
||||
* @see DialogAppendToType
|
||||
* Default value is 'body'.
|
||||
* A valid query selector or an HTMLElement to specify where the dialog gets attached.
|
||||
* @defaultValue body
|
||||
*/
|
||||
appendTo?: DialogAppendToType;
|
||||
appendTo?: 'body' | 'self' | string | undefined | HTMLElement;
|
||||
/**
|
||||
* Style of the dynamic dialog.
|
||||
*/
|
||||
style?: any;
|
||||
/**
|
||||
* Icon to display in the dialog close button.
|
||||
* Default value is 'pi pi-times'.
|
||||
* @defaultValue pi pi-times
|
||||
*/
|
||||
closeIcon?: string | undefined;
|
||||
/**
|
||||
* Icon to display in the dialog maximize button when dialog is not maximized.
|
||||
* Default value is 'pi pi-window-maximize'.
|
||||
* @defaultValue pi pi-window-maximize
|
||||
*/
|
||||
maximizeIcon?: string | undefined;
|
||||
/**
|
||||
* Icon to display in the dialog maximize button when dialog is maximized.
|
||||
* Default value is 'pi pi-window-minimize'.
|
||||
* @defaultValue pi pi-window-minimize
|
||||
*/
|
||||
minimizeIcon?: string | undefined;
|
||||
}
|
||||
|
||||
/**
|
||||
* Defines valid slots in Dialog component.
|
||||
*/
|
||||
export interface DialogSlots {
|
||||
/**
|
||||
* Default content slot.
|
||||
*/
|
||||
default: () => VNode[];
|
||||
default(): VNode[];
|
||||
/**
|
||||
* Custom header template.
|
||||
*/
|
||||
header: () => VNode[];
|
||||
header(): VNode[];
|
||||
/**
|
||||
* Custom footer template.
|
||||
*/
|
||||
footer: () => VNode[];
|
||||
footer(): VNode[];
|
||||
}
|
||||
|
||||
export declare type DialogEmits = {
|
||||
/**
|
||||
* Defines valid emits in Dialog component.
|
||||
*/
|
||||
export interface DialogEmits {
|
||||
/**
|
||||
* Emitted when the visible changes.
|
||||
* @param {boolean} value - New value.
|
||||
*/
|
||||
'update:visible': (value: boolean) => void;
|
||||
'update:visible'(value: boolean): void;
|
||||
/**
|
||||
* Callback to invoke when dialog is hidden.
|
||||
*/
|
||||
hide: () => void;
|
||||
hide(): void;
|
||||
/**
|
||||
* Callback to invoke after dialog is hidden.
|
||||
*/
|
||||
'after-hide': () => void;
|
||||
'after-hide'(): void;
|
||||
/**
|
||||
* Callback to invoke when dialog is shown.
|
||||
*/
|
||||
show: () => void;
|
||||
show(): void;
|
||||
/**
|
||||
* Fired when a dialog gets maximized.
|
||||
* @param {event} event - Browser event.
|
||||
*/
|
||||
maximize: (event: Event) => void;
|
||||
maximize(event: Event): void;
|
||||
/**
|
||||
* Fired when a dialog gets unmaximized.
|
||||
* @param {event} event - Browser event.
|
||||
*/
|
||||
unmaximize: (event: Event) => void;
|
||||
unmaximize(event: Event): void;
|
||||
/**
|
||||
* Fired when a dialog drag completes.
|
||||
* @param {event} event - Browser event.
|
||||
*/
|
||||
dragend: (event: Event) => void;
|
||||
};
|
||||
dragend(event: Event): void;
|
||||
}
|
||||
|
||||
declare class Dialog extends ClassComponent<DialogProps, DialogSlots, DialogEmits> {}
|
||||
/**
|
||||
* **PrimeVue - Dialog**
|
||||
*
|
||||
* _Dialog is a container to display content in an overlay window._
|
||||
*
|
||||
* [Live Demo](https://www.primevue.org/dialog/)
|
||||
* --- ---
|
||||
* 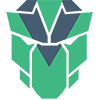
|
||||
*
|
||||
* @group Component
|
||||
*/
|
||||
export declare class Dialog extends ClassComponent<DialogProps, DialogSlots, DialogEmits> {}
|
||||
|
||||
declare module '@vue/runtime-core' {
|
||||
interface GlobalComponents {
|
||||
|
@ -206,13 +236,4 @@ declare module '@vue/runtime-core' {
|
|||
}
|
||||
}
|
||||
|
||||
/**
|
||||
*
|
||||
* Dialog is a container to display content in an overlay window.
|
||||
*
|
||||
* Demos:
|
||||
*
|
||||
* - [Dialog](https://www.primefaces.org/primevue/dialog)
|
||||
*
|
||||
*/
|
||||
export default Dialog;
|
||||
|
|
|
@ -1,12 +1,41 @@
|
|||
/**
|
||||
*
|
||||
* DynamicDialogs can be created dynamically with any component as the content using a DialogService.
|
||||
*
|
||||
* - [Live Demo](https://www.primefaces.org/primevue/dynamicdialog)
|
||||
*
|
||||
* @module dynamicdialog
|
||||
*
|
||||
*/
|
||||
import { ClassComponent, GlobalComponentConstructor } from '../ts-helpers';
|
||||
|
||||
/**
|
||||
* Defines valid properties in DynamicDialog component.
|
||||
*/
|
||||
export interface DynamicDialogProps {}
|
||||
|
||||
export declare type DynamicDialogEmits = {};
|
||||
/**
|
||||
* Defines valid emits in DynamicDialog component.
|
||||
*/
|
||||
export interface DynamicDialogEmits {}
|
||||
|
||||
/**
|
||||
* Defines valid slots in DynamicDialog component.
|
||||
*/
|
||||
export interface DynamicDialogSlots {}
|
||||
|
||||
declare class DynamicDialog extends ClassComponent<DynamicDialogProps, DynamicDialogSlots, DynamicDialogEmits> {}
|
||||
/**
|
||||
* **PrimeVue - DynamicDialog**
|
||||
*
|
||||
* _DynamicDialogs can be created dynamically with any component as the content using a DialogService._
|
||||
*
|
||||
* [Live Demo](https://www.primevue.org/dynamicdialog/)
|
||||
* --- ---
|
||||
* 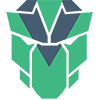
|
||||
*
|
||||
* @group Component
|
||||
*/
|
||||
export declare class DynamicDialog extends ClassComponent<DynamicDialogProps, DynamicDialogSlots, DynamicDialogEmits> {}
|
||||
|
||||
declare module '@vue/runtime-core' {
|
||||
interface GlobalComponents {
|
||||
|
@ -14,13 +43,4 @@ declare module '@vue/runtime-core' {
|
|||
}
|
||||
}
|
||||
|
||||
/**
|
||||
*
|
||||
* DynamicDialogs can be created dynamically with any component as the content using a DialogService.
|
||||
*
|
||||
* Demos:
|
||||
*
|
||||
* - [DynamicDialog](https://www.primefaces.org/primevue/dynamicdialog)
|
||||
*
|
||||
*/
|
||||
export default DynamicDialog;
|
||||
|
|
|
@ -74,7 +74,7 @@ export interface KnobProps {
|
|||
showValue?: boolean | undefined;
|
||||
/**
|
||||
* Template string of the value.
|
||||
* @defaultValue {value}
|
||||
* @defaultValue \{value}
|
||||
*/
|
||||
valueTemplate?: string | undefined;
|
||||
/**
|
||||
|
|
|
@ -1,8 +1,18 @@
|
|||
/**
|
||||
*
|
||||
* OverlayPanel is a container component positioned as connected to its target.
|
||||
*
|
||||
* - [Live Demo](https://www.primefaces.org/primevue/overlaypanel)
|
||||
*
|
||||
* @module overlaypanel
|
||||
*
|
||||
*/
|
||||
import { VNode } from 'vue';
|
||||
import { ClassComponent, GlobalComponentConstructor } from '../ts-helpers';
|
||||
|
||||
type OverlayPanelAppendToType = 'body' | 'self' | string | undefined | HTMLElement;
|
||||
|
||||
/**
|
||||
* OverlayPanel breakpoint metadata.
|
||||
*/
|
||||
export interface OverlayPanelBreakpoints {
|
||||
/**
|
||||
* Breakpoint for responsive mode.
|
||||
|
@ -22,58 +32,77 @@ export interface OverlayPanelBreakpoints {
|
|||
[key: string]: string;
|
||||
}
|
||||
|
||||
/**
|
||||
* Defines valid properties in OverlayPanel component.
|
||||
*/
|
||||
export interface OverlayPanelProps {
|
||||
/**
|
||||
* Enables to hide the overlay when outside is clicked.
|
||||
* Default value is true.
|
||||
* @defaultValue true
|
||||
*/
|
||||
dismissable?: boolean;
|
||||
/**
|
||||
* When enabled, displays a close icon at top right corner.
|
||||
* @defaultValue false
|
||||
*/
|
||||
showCloseIcon?: boolean;
|
||||
/**
|
||||
* A valid query selector or an HTMLElement to specify where the overlay gets attached.
|
||||
* @see OverlayPanelAppendToType
|
||||
* Default value is 'body'.
|
||||
* @defaultValue body
|
||||
*/
|
||||
appendTo?: OverlayPanelAppendToType;
|
||||
appendTo?: 'body' | 'self' | string | undefined | HTMLElement;
|
||||
/**
|
||||
* Base zIndex value to use in layering.
|
||||
* Default value is 0.
|
||||
* @defaultValue 0
|
||||
*/
|
||||
baseZIndex?: number;
|
||||
/**
|
||||
* Whether to automatically manage layering.
|
||||
* Default value is true.
|
||||
* @defaultValue true
|
||||
*/
|
||||
autoZIndex?: boolean;
|
||||
/**
|
||||
* Object literal to define widths per screen size.
|
||||
* @see OverlayPanelBreakpoints
|
||||
*/
|
||||
breakpoints?: OverlayPanelBreakpoints;
|
||||
}
|
||||
|
||||
/**
|
||||
* Defines valid slots in OverlayPanel component.
|
||||
*/
|
||||
export interface OverlayPanelSlots {
|
||||
/**
|
||||
* Custom content template.
|
||||
*/
|
||||
default: () => VNode[];
|
||||
default(): VNode[];
|
||||
}
|
||||
|
||||
export declare type OverlayPanelEmits = {
|
||||
/**
|
||||
* Defines valid emits in OverlayPanel component.
|
||||
*/
|
||||
export interface OverlayPanelEmits {
|
||||
/**
|
||||
* Callback to invoke when the overlay is shown.
|
||||
*/
|
||||
show: () => void;
|
||||
show(): void;
|
||||
/**
|
||||
* Callback to invoke when the overlay is hidden.
|
||||
*/
|
||||
hide: () => void;
|
||||
};
|
||||
hide(): void;
|
||||
}
|
||||
|
||||
declare class OverlayPanel extends ClassComponent<OverlayPanelProps, OverlayPanelSlots, OverlayPanelEmits> {
|
||||
/**
|
||||
* **PrimeVue - OverlayPanel**
|
||||
*
|
||||
* _OverlayPanel, also known as Popover, is a container component that can overlay other components on page._
|
||||
*
|
||||
* [Live Demo](https://www.primevue.org/overlaypanel/)
|
||||
* --- ---
|
||||
* 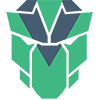
|
||||
*
|
||||
* @group Component
|
||||
*/
|
||||
export declare class OverlayPanel extends ClassComponent<OverlayPanelProps, OverlayPanelSlots, OverlayPanelEmits> {
|
||||
/**
|
||||
* Toggles the visibility of the overlay.
|
||||
* @param {Event} event - Browser event.
|
||||
|
@ -81,7 +110,7 @@ declare class OverlayPanel extends ClassComponent<OverlayPanelProps, OverlayPane
|
|||
*
|
||||
* @memberof OverlayPanel
|
||||
*/
|
||||
toggle: (event: Event, target?: any) => void;
|
||||
toggle(event: Event, target?: any): void;
|
||||
/**
|
||||
* Shows the overlay.
|
||||
* @param {Event} event - Browser event.
|
||||
|
@ -89,13 +118,13 @@ declare class OverlayPanel extends ClassComponent<OverlayPanelProps, OverlayPane
|
|||
*
|
||||
* @memberof OverlayPanel
|
||||
*/
|
||||
show: (event: Event, target?: any) => void;
|
||||
show(event: Event, target?: any): void;
|
||||
/**
|
||||
* Hides the overlay.
|
||||
*
|
||||
* @memberof OverlayPanel
|
||||
*/
|
||||
hide: () => void;
|
||||
hide(): void;
|
||||
}
|
||||
|
||||
declare module '@vue/runtime-core' {
|
||||
|
@ -104,13 +133,4 @@ declare module '@vue/runtime-core' {
|
|||
}
|
||||
}
|
||||
|
||||
/**
|
||||
*
|
||||
* OverlayPanel is a container component positioned as connected to its target.
|
||||
*
|
||||
* Demos:
|
||||
*
|
||||
* - [OverlayPanel](https://www.primefaces.org/primevue/overlaypanel)
|
||||
*
|
||||
*/
|
||||
export default OverlayPanel;
|
||||
|
|
|
@ -1,83 +1,111 @@
|
|||
/**
|
||||
*
|
||||
* Sidebar is a panel component displayed as an overlay at the edges of the screen.
|
||||
*
|
||||
* - [Live Demo](https://www.primefaces.org/primevue/sidebar)
|
||||
*
|
||||
* @module sidebar
|
||||
*
|
||||
*/
|
||||
import { VNode } from 'vue';
|
||||
import { ClassComponent, GlobalComponentConstructor } from '../ts-helpers';
|
||||
|
||||
type SidebarPositionType = 'left' | 'right' | 'top' | 'bottom' | 'full' | undefined;
|
||||
|
||||
/**
|
||||
* Defines valid properties in Sidebar component.
|
||||
*/
|
||||
export interface SidebarProps {
|
||||
/**
|
||||
* Specifies the visibility of the dialog.
|
||||
* @defaultValue false
|
||||
*/
|
||||
visible?: boolean | undefined;
|
||||
/**
|
||||
* Specifies the position of the sidebar.
|
||||
* @see SidebarPositionType
|
||||
* Default value is 'left'.
|
||||
* @defaultValue left
|
||||
*/
|
||||
position?: SidebarPositionType;
|
||||
position?: 'left' | 'right' | 'top' | 'bottom' | 'full' | undefined;
|
||||
/**
|
||||
* Base zIndex value to use in layering.
|
||||
* Default value is 0.
|
||||
* @defaultValue 0
|
||||
*/
|
||||
baseZIndex?: number | undefined;
|
||||
/**
|
||||
* Whether to automatically manage layering.
|
||||
* Default value is true.
|
||||
* @defaultValue true
|
||||
*/
|
||||
autoZIndex?: boolean | undefined;
|
||||
/**
|
||||
* Whether clicking outside closes the panel.
|
||||
* Default value is true.
|
||||
* @defaultValue true
|
||||
*/
|
||||
dismissable?: boolean | undefined;
|
||||
/**
|
||||
* Whether to display a close icon inside the panel.
|
||||
* Default value is true.
|
||||
* @defaultValue true
|
||||
*/
|
||||
showCloseIcon?: boolean | undefined;
|
||||
/**
|
||||
* Icon to display in the sidebar close button.
|
||||
* Default value is 'pi pi-times'.
|
||||
* @defaultValue pi pi-times
|
||||
*/
|
||||
closeIcon?: string | undefined;
|
||||
/**
|
||||
* Whether to a modal layer behind the sidebar.
|
||||
* Default value is true.
|
||||
* @defaultValue true
|
||||
*/
|
||||
modal?: boolean | undefined;
|
||||
/**
|
||||
* Whether background scroll should be blocked when sidebar is visible.
|
||||
* @defaultValue false
|
||||
*/
|
||||
blockScroll?: boolean | undefined;
|
||||
}
|
||||
|
||||
/**
|
||||
* Defines valid slots in Sidebar component.
|
||||
*/
|
||||
export interface SidebarSlots {
|
||||
/**
|
||||
* Custom content template.
|
||||
*/
|
||||
default: () => VNode[];
|
||||
default(): VNode[];
|
||||
/**
|
||||
* Custom header template.
|
||||
*/
|
||||
header: () => VNode[];
|
||||
header(): VNode[];
|
||||
}
|
||||
|
||||
export declare type SidebarEmits = {
|
||||
/**
|
||||
* Defines valid emits in Sidebar component.
|
||||
*/
|
||||
export interface SidebarEmits {
|
||||
/**
|
||||
* Emitted when the value changes.
|
||||
* @param {boolean} value - New value.
|
||||
*/
|
||||
'update:modelValue': (value: boolean) => void;
|
||||
'update:modelValue'(value: boolean): void;
|
||||
/**
|
||||
* Callback to invoke when sidebar gets shown.
|
||||
*/
|
||||
show: () => void;
|
||||
show(): void;
|
||||
/**
|
||||
* Callback to invoke when sidebar gets hidden.
|
||||
*/
|
||||
hide: () => void;
|
||||
};
|
||||
hide(): void;
|
||||
}
|
||||
|
||||
declare class Sidebar extends ClassComponent<SidebarProps, SidebarSlots, SidebarEmits> {}
|
||||
/**
|
||||
* **PrimeVue - Sidebar**
|
||||
*
|
||||
* _Sidebar is a panel component displayed as an overlay._
|
||||
*
|
||||
* [Live Demo](https://www.primevue.org/sidebar/)
|
||||
* --- ---
|
||||
* 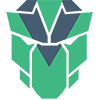
|
||||
*
|
||||
* @group Component
|
||||
*/
|
||||
export declare class Sidebar extends ClassComponent<SidebarProps, SidebarSlots, SidebarEmits> {}
|
||||
|
||||
declare module '@vue/runtime-core' {
|
||||
interface GlobalComponents {
|
||||
|
|
|
@ -1,5 +1,5 @@
|
|||
<template>
|
||||
<DocComponent title="Vue Dynamic Dialog Component" header="Dialog" description="Dynamic Dialog is a Dialog container to display content in an overlay window." :componentDocs="docs" :apiDocs="['DynamicDialog', 'Dialog']" />
|
||||
<DocComponent title="Vue Dynamic Dialog Component" header="Dynamic Dialog" description="Dynamic Dialog is a Dialog container to display content in an overlay window." :componentDocs="docs" :apiDocs="['DynamicDialog', 'Dialog']" />
|
||||
</template>
|
||||
|
||||
<script>
|
||||
|
|
Loading…
Reference in New Issue