Password d.ts updated
parent
d14f89209b
commit
d9f6b7dc6d
|
@ -1,8 +1,18 @@
|
|||
import { VNode, InputHTMLAttributes, HTMLAttributes } from 'vue';
|
||||
/**
|
||||
*
|
||||
* Password displays strength indicator for password fields.
|
||||
*
|
||||
* [Live Demo](https://www.primevue.org/password/)
|
||||
*
|
||||
* @module password
|
||||
*
|
||||
*/
|
||||
import { HTMLAttributes, InputHTMLAttributes, VNode } from 'vue';
|
||||
import { ClassComponent, GlobalComponentConstructor, Nullable } from '../ts-helpers';
|
||||
|
||||
type PasswordAppendToType = 'body' | 'self' | string | undefined | HTMLElement;
|
||||
|
||||
/**
|
||||
* Defines valid properties in Password component.
|
||||
*/
|
||||
export interface PasswordProps extends InputHTMLAttributes {
|
||||
/**
|
||||
* Value of the component.
|
||||
|
@ -14,12 +24,12 @@ export interface PasswordProps extends InputHTMLAttributes {
|
|||
promptLabel?: string | undefined;
|
||||
/**
|
||||
* Regex for a medium level password.
|
||||
* Default value is '^(((?=.*[a-z])(?=.*[A-Z]))|((?=.*[a-z])(?=.*[0-9]))|((?=.*[A-Z])(?=.*[0-9])))(?=.{6,})'.
|
||||
* @defaultValue ^(((?=.*[a-z])(?=.*[A-Z]))|((?=.*[a-z])(?=.*[0-9]))|((?=.*[A-Z])(?=.*[0-9])))(?=.{6,})
|
||||
*/
|
||||
mediumRegex?: string | undefined;
|
||||
/**
|
||||
* Regex for a strong level password.
|
||||
* Default value is '^(?=.*[a-z])(?=.*[A-Z])(?=.*[0-9])(?=.{8,})'.
|
||||
* @defaultValue ^(?=.*[a-z])(?=.*[A-Z])(?=.*[0-9])(?=.{8,})
|
||||
*/
|
||||
strongRegex?: string | undefined;
|
||||
/**
|
||||
|
@ -36,31 +46,32 @@ export interface PasswordProps extends InputHTMLAttributes {
|
|||
strongLabel?: string | undefined;
|
||||
/**
|
||||
* Whether to show the strength indicator or not.
|
||||
* Default value is true.
|
||||
* @defaultValue true
|
||||
*/
|
||||
feedback?: boolean | undefined;
|
||||
/**
|
||||
* A valid query selector or an HTMLElement to specify where the overlay gets attached. Special keywords are 'body' for document body and 'self' for the element itself.
|
||||
* @see PasswordAppendToType
|
||||
* Default value is 'body'.
|
||||
* A valid query selector or an HTMLElement to specify where the overlay gets attached.
|
||||
* @defaultValue body
|
||||
*/
|
||||
appendTo?: PasswordAppendToType;
|
||||
appendTo?: 'body' | 'self' | string | undefined | HTMLElement;
|
||||
/**
|
||||
* Whether to show an icon to display the password as plain text.
|
||||
* @defaultValue false
|
||||
*/
|
||||
toggleMask?: boolean | undefined;
|
||||
/**
|
||||
* Icon to hide displaying the password as plain text.
|
||||
* Default value is 'pi pi-eye-slash'.
|
||||
* @defaultValue pi pi-eye-slash
|
||||
*/
|
||||
hideIcon?: string | undefined;
|
||||
/**
|
||||
* Icon to show displaying the password as plain text.
|
||||
* Default value is 'pi pi-eye'.
|
||||
* @defaultValue pi pi-eye
|
||||
*/
|
||||
showIcon?: string | undefined;
|
||||
/**
|
||||
* When present, it specifies that the component should be disabled.
|
||||
* @defaultValue false
|
||||
*/
|
||||
disabled?: boolean | undefined;
|
||||
/**
|
||||
|
@ -69,7 +80,7 @@ export interface PasswordProps extends InputHTMLAttributes {
|
|||
placeholder?: string | undefined;
|
||||
/**
|
||||
* When present, it specifies that an input field must be filled out before submitting the form.
|
||||
* Default value is false.
|
||||
* @defaultValue false
|
||||
*/
|
||||
required?: boolean | undefined;
|
||||
/**
|
||||
|
@ -114,30 +125,47 @@ export interface PasswordProps extends InputHTMLAttributes {
|
|||
'aria-label'?: string | undefined;
|
||||
}
|
||||
|
||||
/**
|
||||
* Defines valid slots in Password component.
|
||||
*/
|
||||
export interface PasswordSlots {
|
||||
/**
|
||||
* Custom header template.
|
||||
*/
|
||||
header: () => VNode[];
|
||||
header(): VNode[];
|
||||
/**
|
||||
* Custom footer template.
|
||||
*/
|
||||
footer: () => VNode[];
|
||||
footer(): VNode[];
|
||||
/**
|
||||
* Custom content template.
|
||||
*/
|
||||
content: () => VNode[];
|
||||
content(): VNode[];
|
||||
}
|
||||
|
||||
export declare type PasswordEmits = {
|
||||
/**
|
||||
* Defines valid emits in Password component.
|
||||
*/
|
||||
export interface PasswordEmits {
|
||||
/**
|
||||
* Emitted when the value changes.
|
||||
* @param {string} value - New value.
|
||||
*/
|
||||
'update:modelValue': (value: string) => void;
|
||||
};
|
||||
'update:modelValue'(value: string): void;
|
||||
}
|
||||
|
||||
declare class Password extends ClassComponent<PasswordProps, PasswordSlots, PasswordEmits> {}
|
||||
/**
|
||||
* **PrimeVue - Password**
|
||||
*
|
||||
* _Password displays strength indicator for password fields._
|
||||
*
|
||||
* [Live Demo](https://www.primevue.org/password/)
|
||||
* --- ---
|
||||
* 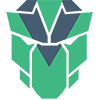
|
||||
*
|
||||
* @group Component
|
||||
*/
|
||||
export declare class Password extends ClassComponent<PasswordProps, PasswordSlots, PasswordEmits> {}
|
||||
|
||||
declare module '@vue/runtime-core' {
|
||||
interface GlobalComponents {
|
||||
|
@ -145,13 +173,4 @@ declare module '@vue/runtime-core' {
|
|||
}
|
||||
}
|
||||
|
||||
/**
|
||||
*
|
||||
* Password displays strength indicator for password fields.
|
||||
*
|
||||
* Demos:
|
||||
*
|
||||
* - [Password](https://www.primefaces.org/primevue/password)
|
||||
*
|
||||
*/
|
||||
export default Password;
|
||||
|
|
Loading…
Reference in New Issue