mirror of
https://github.com/primefaces/primevue.git
synced 2025-05-09 17:02:38 +00:00
Fixed #3802 - Improve folder structure for nuxt configurations
This commit is contained in:
parent
851950270b
commit
f5fe822afb
563 changed files with 1703 additions and 1095 deletions
230
components/dataview/DataView.d.ts
vendored
230
components/dataview/DataView.d.ts
vendored
|
@ -1,230 +0,0 @@
|
|||
/**
|
||||
*
|
||||
* DataView displays data in grid or list layout with pagination and sorting features.
|
||||
*
|
||||
* [Live Demo](https://www.primevue.org/dataview/)
|
||||
*
|
||||
* @module dataview
|
||||
*
|
||||
*/
|
||||
import { VNode } from 'vue';
|
||||
import { ClassComponent, GlobalComponentConstructor } from '../ts-helpers';
|
||||
|
||||
/**
|
||||
* Custom page event.
|
||||
* @see {@link DataViewEmits.page}
|
||||
*/
|
||||
export interface DataViewPageEvent {
|
||||
/**
|
||||
* New page number
|
||||
*/
|
||||
page: number;
|
||||
/**
|
||||
* Index of first record
|
||||
*/
|
||||
first: number;
|
||||
/**
|
||||
* Number of rows to display in new page
|
||||
*/
|
||||
rows: number;
|
||||
/**
|
||||
* Total number of pages
|
||||
*/
|
||||
pageCount: number;
|
||||
}
|
||||
|
||||
/**
|
||||
* Defines valid properties in DataView component. In addition to these, all properties of HTMLDivElement can be used in this component.
|
||||
*/
|
||||
export interface DataViewProps {
|
||||
/**
|
||||
* An array of objects to display.
|
||||
*/
|
||||
value?: any[] | undefined;
|
||||
/**
|
||||
* Layout of the items, valid values are 'list' and 'grid'.
|
||||
* @defaultValue list
|
||||
*/
|
||||
layout?: 'list' | 'grid' | undefined;
|
||||
/**
|
||||
* Number of rows to display per page.
|
||||
* @defaultValue 0
|
||||
*/
|
||||
rows?: number | undefined;
|
||||
/**
|
||||
* Index of the first record to render.
|
||||
* @defaultValue 0
|
||||
*/
|
||||
first?: number | undefined;
|
||||
/**
|
||||
* Number of total records, defaults to length of value when not defined.
|
||||
*/
|
||||
totalRecords?: number | undefined;
|
||||
/**
|
||||
* When specified as true, enables the pagination.
|
||||
* @defaultValue false
|
||||
*/
|
||||
paginator?: boolean | undefined;
|
||||
/**
|
||||
* Position of the paginator, options are 'top','bottom' or 'both'.
|
||||
* @defaultValue bottom
|
||||
*/
|
||||
paginatorPosition?: 'top' | 'bottom' | 'both' | undefined;
|
||||
/**
|
||||
* Whether to show it even there is only one page.
|
||||
* @defaultValue true
|
||||
*/
|
||||
alwaysShowPaginator?: boolean | undefined;
|
||||
/**
|
||||
* Template of the paginator. It can be customized using the template property using the predefined keys,
|
||||
*
|
||||
* - FirstPageLink
|
||||
* - PrevPageLink
|
||||
* - PageLinks
|
||||
* - NextPageLink
|
||||
* - LastPageLink
|
||||
* - RowsPerPageDropdown
|
||||
* - JumpToPageDropdown
|
||||
* - JumpToPageInput
|
||||
* - CurrentPageReport
|
||||
*
|
||||
* @defaultValue FirstPageLink PrevPageLink PageLinks NextPageLink LastPageLink RowsPerPageDropdown
|
||||
*/
|
||||
paginatorTemplate?: string | undefined;
|
||||
/**
|
||||
* Number of page links to display.
|
||||
* @defaultValue 5
|
||||
*/
|
||||
pageLinkSize?: number | undefined;
|
||||
/**
|
||||
* Array of integer values to display inside rows per page dropdown.
|
||||
*/
|
||||
rowsPerPageOptions?: number[] | undefined;
|
||||
/**
|
||||
* Template of the current page report element. It displays information about the pagination state.
|
||||
*
|
||||
* - {currentPage}
|
||||
* - {totalPages}
|
||||
* - {rows}
|
||||
* - {first}
|
||||
* - {last}
|
||||
* - {totalRecords}
|
||||
*
|
||||
* @defaultValue '({currentPage} of {totalPages})'
|
||||
*/
|
||||
currentPageReportTemplate?: string | undefined;
|
||||
/**
|
||||
* Property name or a getter function of data to use in sorting by default.
|
||||
*/
|
||||
sortField?: string | ((item: any) => string) | undefined;
|
||||
/**
|
||||
* Order to sort the data by default.
|
||||
*/
|
||||
sortOrder?: number | undefined;
|
||||
/**
|
||||
* Defines if data is loaded and interacted with in lazy manner.
|
||||
* @defaultValue false
|
||||
*/
|
||||
lazy?: boolean | undefined;
|
||||
/**
|
||||
* Name of the data that uniquely identifies the a record in the data.
|
||||
*/
|
||||
dataKey: string | undefined;
|
||||
}
|
||||
|
||||
/**
|
||||
* Defines valid slots in DataView component.
|
||||
*/
|
||||
export interface DataViewSlots {
|
||||
/**
|
||||
* Custom header template.
|
||||
*/
|
||||
header(): VNode[];
|
||||
/**
|
||||
* Custom footer template.
|
||||
*/
|
||||
footer(): VNode[];
|
||||
/**
|
||||
* Custom empty template.
|
||||
*/
|
||||
empty(): VNode[];
|
||||
/**
|
||||
* Custom paginator start template.
|
||||
*/
|
||||
paginatorstart(): VNode[];
|
||||
/**
|
||||
* Custom paginator end template.
|
||||
*/
|
||||
paginatorend(): VNode[];
|
||||
/**
|
||||
* Custom list template.
|
||||
* @param {Object} scope - list slot's params.
|
||||
*/
|
||||
list(scope: {
|
||||
/**
|
||||
* Value of the component
|
||||
*/
|
||||
data: any;
|
||||
/**
|
||||
* Index of the grid
|
||||
*/
|
||||
index: number;
|
||||
}): VNode[];
|
||||
/**
|
||||
* Custom list template.
|
||||
* @param {Object} scope - list slot's params.
|
||||
*/
|
||||
grid(scope: {
|
||||
/**
|
||||
* Value of the component
|
||||
*/
|
||||
data: any;
|
||||
/**
|
||||
* Index of the grid
|
||||
*/
|
||||
index: number;
|
||||
}): VNode[];
|
||||
}
|
||||
|
||||
/**
|
||||
* Defines valid slots in DataView component.
|
||||
*/
|
||||
export interface DataViewEmits {
|
||||
/**
|
||||
* Emitted when the first changes.
|
||||
* @param {number} value - New value.
|
||||
*/
|
||||
'update:first'(value: number): void;
|
||||
/**
|
||||
* Emitted when the rows changes.
|
||||
* @param {number} value - New value.
|
||||
*/
|
||||
'update:rows'(value: number): void;
|
||||
/**
|
||||
* Callback to invoke when page changes, the event object contains information about the new state.
|
||||
* @param {DataViewPageEvent} event - Custom page event.
|
||||
*/
|
||||
page(event: DataViewPageEvent): void;
|
||||
}
|
||||
|
||||
/**
|
||||
* **PrimeVue - DataViewLayoutOptions**
|
||||
*
|
||||
* _DataView displays data in grid or list layout with pagination and sorting features._
|
||||
*
|
||||
* [Live Demo](https://www.primevue.org/dataview/)
|
||||
* --- ---
|
||||
* 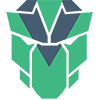
|
||||
*
|
||||
* @group Component
|
||||
*
|
||||
*/
|
||||
declare class DataView extends ClassComponent<DataViewProps, DataViewSlots, DataViewEmits> {}
|
||||
|
||||
declare module '@vue/runtime-core' {
|
||||
interface GlobalComponents {
|
||||
DataView: GlobalComponentConstructor<DataView>;
|
||||
}
|
||||
}
|
||||
|
||||
export default DataView;
|
Loading…
Add table
Add a link
Reference in a new issue