mirror of
https://github.com/primefaces/primevue.git
synced 2025-05-10 17:32:36 +00:00
Fixed #3802 - Improve folder structure for nuxt configurations
This commit is contained in:
parent
851950270b
commit
f5fe822afb
563 changed files with 1703 additions and 1095 deletions
82
components/lib/breadcrumb/Breadcrumb.d.ts
vendored
Executable file
82
components/lib/breadcrumb/Breadcrumb.d.ts
vendored
Executable file
|
@ -0,0 +1,82 @@
|
|||
/**
|
||||
*
|
||||
* Breadcrumb provides contextual information about page hierarchy.
|
||||
*
|
||||
* [Live Demo](https://www.primevue.org/breadcrumb/)
|
||||
*
|
||||
* @module breadcrumb
|
||||
*
|
||||
*/
|
||||
import { VNode } from 'vue';
|
||||
import { MenuItem } from '../menuitem';
|
||||
import { ClassComponent, GlobalComponentConstructor } from '../ts-helpers';
|
||||
|
||||
/**
|
||||
* Defines valid properties in Breadcrumb component.
|
||||
*/
|
||||
export interface BreadcrumbProps {
|
||||
/**
|
||||
* An array of menuitems.
|
||||
*/
|
||||
model?: MenuItem[] | undefined;
|
||||
/**
|
||||
* Configuration for the home icon.
|
||||
*/
|
||||
home?: MenuItem | undefined;
|
||||
/**
|
||||
* Whether to apply 'router-link-active-exact' class if route exactly matches the item path.
|
||||
* @defaultValue true
|
||||
*/
|
||||
exact?: boolean | undefined;
|
||||
/**
|
||||
* Defines a string value that labels an interactive element.
|
||||
*/
|
||||
'aria-label'?: string | undefined;
|
||||
/**
|
||||
* Identifier of the underlying menu element.
|
||||
*/
|
||||
'aria-labelledby'?: string | undefined;
|
||||
}
|
||||
|
||||
/**
|
||||
* Defines valid slots in Breadcrumb component.
|
||||
*/
|
||||
export interface BreadcrumbSlots {
|
||||
/**
|
||||
* Custom item template.
|
||||
* @param {Object} scope - item slot's params.
|
||||
*/
|
||||
item(scope: {
|
||||
/**
|
||||
* Menuitem instance
|
||||
*/
|
||||
item: MenuItem;
|
||||
}): VNode[];
|
||||
}
|
||||
|
||||
/**
|
||||
* Defines valid emits in Breadcrumb component.
|
||||
*/
|
||||
export interface BreadcrumbEmits {}
|
||||
|
||||
/**
|
||||
* **PrimeVue - Breadcrumb**
|
||||
*
|
||||
* _Breadcrumb provides contextual information about page hierarchy._
|
||||
*
|
||||
* [Live Demo](https://www.primevue.org/breadcrumb/)
|
||||
* --- ---
|
||||
* 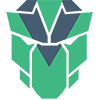
|
||||
*
|
||||
* @group Component
|
||||
*
|
||||
*/
|
||||
declare class Breadcrumb extends ClassComponent<BreadcrumbProps, BreadcrumbSlots, BreadcrumbEmits> {}
|
||||
|
||||
declare module '@vue/runtime-core' {
|
||||
interface GlobalComponents {
|
||||
Breadcrumb: GlobalComponentConstructor<Breadcrumb>;
|
||||
}
|
||||
}
|
||||
|
||||
export default Breadcrumb;
|
29
components/lib/breadcrumb/Breadcrumb.spec.js
Normal file
29
components/lib/breadcrumb/Breadcrumb.spec.js
Normal file
|
@ -0,0 +1,29 @@
|
|||
import { mount } from '@vue/test-utils';
|
||||
import Breadcrumb from './Breadcrumb.vue';
|
||||
|
||||
describe('Breadcrumb', () => {
|
||||
it('should exist', () => {
|
||||
const wrapper = mount(Breadcrumb, {
|
||||
global: {
|
||||
stubs: {
|
||||
'router-link': true
|
||||
},
|
||||
mocks: {
|
||||
$router: {
|
||||
currentRoute: {
|
||||
path: vi.fn()
|
||||
}
|
||||
}
|
||||
}
|
||||
},
|
||||
props: {
|
||||
home: { icon: 'pi pi-home', to: '/' },
|
||||
model: [{ label: 'Computer' }, { label: 'Notebook' }, { label: 'Accessories' }, { label: 'Backpacks' }, { label: 'Item' }]
|
||||
}
|
||||
});
|
||||
|
||||
expect(wrapper.find('.p-breadcrumb.p-component').exists()).toBe(true);
|
||||
expect(wrapper.findAll('.p-menuitem-separator').length).toBe(5);
|
||||
expect(wrapper.findAll('.p-menuitem-text').length).toBe(5);
|
||||
});
|
||||
});
|
72
components/lib/breadcrumb/Breadcrumb.vue
Executable file
72
components/lib/breadcrumb/Breadcrumb.vue
Executable file
|
@ -0,0 +1,72 @@
|
|||
<template>
|
||||
<nav class="p-breadcrumb p-component">
|
||||
<ol class="p-breadcrumb-list">
|
||||
<BreadcrumbItem v-if="home" :item="home" class="p-breadcrumb-home" :template="$slots.item" :exact="exact" />
|
||||
<template v-for="(item, i) of model" :key="item.label">
|
||||
<li v-if="home || i !== 0" class="p-menuitem-separator">
|
||||
<span class="pi pi-chevron-right" aria-hidden="true"></span>
|
||||
</li>
|
||||
<BreadcrumbItem :item="item" :template="$slots.item" :exact="exact" />
|
||||
</template>
|
||||
</ol>
|
||||
</nav>
|
||||
</template>
|
||||
|
||||
<script>
|
||||
import BreadcrumbItem from './BreadcrumbItem.vue';
|
||||
|
||||
export default {
|
||||
name: 'Breadcrumb',
|
||||
props: {
|
||||
model: {
|
||||
type: Array,
|
||||
default: null
|
||||
},
|
||||
home: {
|
||||
type: null,
|
||||
default: null
|
||||
},
|
||||
exact: {
|
||||
type: Boolean,
|
||||
default: true
|
||||
}
|
||||
},
|
||||
components: {
|
||||
BreadcrumbItem: BreadcrumbItem
|
||||
}
|
||||
};
|
||||
</script>
|
||||
|
||||
<style>
|
||||
.p-breadcrumb {
|
||||
overflow-x: auto;
|
||||
}
|
||||
|
||||
.p-breadcrumb .p-breadcrumb-list {
|
||||
margin: 0;
|
||||
padding: 0;
|
||||
list-style-type: none;
|
||||
display: flex;
|
||||
align-items: center;
|
||||
flex-wrap: nowrap;
|
||||
}
|
||||
|
||||
.p-breadcrumb .p-menuitem-text {
|
||||
line-height: 1;
|
||||
}
|
||||
|
||||
.p-breadcrumb .p-menuitem-link {
|
||||
text-decoration: none;
|
||||
display: flex;
|
||||
align-items: center;
|
||||
}
|
||||
|
||||
.p-breadcrumb .p-menuitem-separator {
|
||||
display: flex;
|
||||
align-items: center;
|
||||
}
|
||||
|
||||
.p-breadcrumb::-webkit-scrollbar {
|
||||
display: none;
|
||||
}
|
||||
</style>
|
91
components/lib/breadcrumb/BreadcrumbItem.spec.js
Normal file
91
components/lib/breadcrumb/BreadcrumbItem.spec.js
Normal file
|
@ -0,0 +1,91 @@
|
|||
import { RouterLinkStub, shallowMount } from '@vue/test-utils';
|
||||
import { beforeEach, expect } from 'vitest';
|
||||
import BreadcrumbItem from './BreadcrumbItem.vue';
|
||||
|
||||
let wrapper = null;
|
||||
|
||||
beforeEach(() => {
|
||||
wrapper = shallowMount(BreadcrumbItem, {
|
||||
global: {
|
||||
mocks: {
|
||||
$router: {
|
||||
currentRoute: {
|
||||
path: vi.fn()
|
||||
},
|
||||
navigate: () => true
|
||||
}
|
||||
},
|
||||
stubs: {
|
||||
'router-link': RouterLinkStub
|
||||
}
|
||||
},
|
||||
props: {
|
||||
item: { label: 'Computer', visible: () => true },
|
||||
template: null
|
||||
}
|
||||
});
|
||||
});
|
||||
|
||||
afterEach(() => {
|
||||
vi.clearAllMocks();
|
||||
});
|
||||
|
||||
describe('BreadcrumbItem', () => {
|
||||
it('When component is mount, text should be exists', () => {
|
||||
expect(wrapper.find('.p-menuitem-text').exists()).toBe(true);
|
||||
});
|
||||
|
||||
it('When tag is triggered, onClick method should be called', async () => {
|
||||
const onClickSpy = vi.spyOn(wrapper.vm, 'onClick');
|
||||
const tag = wrapper.find('a');
|
||||
|
||||
tag.trigger('click');
|
||||
expect(tag.exists()).toBe(true);
|
||||
expect(onClickSpy).toHaveBeenCalled();
|
||||
});
|
||||
|
||||
it('When onClick method called and item has a command callback, callback should be triggered', async () => {
|
||||
await wrapper.setProps({ item: { label: 'Computer', visible: () => false, command: vi.fn() } });
|
||||
|
||||
const spy = vi.spyOn(wrapper.vm.item, 'command');
|
||||
|
||||
wrapper.vm.onClick();
|
||||
expect(spy).toHaveBeenCalled();
|
||||
});
|
||||
|
||||
it('When linkClass method called and isActive-isExactActive, tag classes should be effected', async () => {
|
||||
await wrapper.setProps({ exact: true });
|
||||
|
||||
expect(wrapper.vm.linkClass({ isActive: true, isExactActive: true })).toEqual([
|
||||
'p-menuitem-link',
|
||||
{
|
||||
'router-link-active': true,
|
||||
'router-link-active-exact': true
|
||||
}
|
||||
]);
|
||||
});
|
||||
|
||||
it('When item prop has a visible, visible method should be return falsy', async () => {
|
||||
await wrapper.setProps({ item: { label: 'Computer', visible: false, command: vi.fn() } });
|
||||
|
||||
expect(wrapper.vm.visible()).toBeFalsy();
|
||||
});
|
||||
|
||||
it('When item prop has a disabled function, disabled method should be return truthy', async () => {
|
||||
await wrapper.setProps({ item: { disabled: () => true } });
|
||||
|
||||
expect(wrapper.vm.disabled()).toBeTruthy();
|
||||
});
|
||||
|
||||
it('When item prop has a label function, disabled method should be return truthy', async () => {
|
||||
await wrapper.setProps({ item: { label: () => true } });
|
||||
|
||||
expect(wrapper.vm.label()).toBeTruthy();
|
||||
});
|
||||
|
||||
it('When item prop has a icon, icon element class should contain item prop icon', async () => {
|
||||
await wrapper.setProps({ item: { icon: 'pi-discord' } });
|
||||
|
||||
expect(wrapper.find('a > span').classes()).toContain('pi-discord');
|
||||
});
|
||||
});
|
74
components/lib/breadcrumb/BreadcrumbItem.vue
Executable file
74
components/lib/breadcrumb/BreadcrumbItem.vue
Executable file
|
@ -0,0 +1,74 @@
|
|||
<template>
|
||||
<li v-if="visible()" :class="containerClass()">
|
||||
<template v-if="!template">
|
||||
<router-link v-if="item.to" v-slot="{ navigate, href, isActive, isExactActive }" :to="item.to" custom>
|
||||
<a :href="href" :class="linkClass({ isActive, isExactActive })" :aria-current="isCurrentUrl()" @click="onClick($event, navigate)">
|
||||
<span v-if="item.icon" :class="iconClass"></span>
|
||||
<span v-if="item.label" class="p-menuitem-text">{{ label() }}</span>
|
||||
</a>
|
||||
</router-link>
|
||||
<a v-else :href="item.url || '#'" :class="linkClass()" :target="item.target" :aria-current="isCurrentUrl()" @click="onClick">
|
||||
<span v-if="item.icon" :class="iconClass"></span>
|
||||
<span v-if="item.label" class="p-menuitem-text">{{ label() }}</span>
|
||||
</a>
|
||||
</template>
|
||||
<component v-else :is="template" :item="item"></component>
|
||||
</li>
|
||||
</template>
|
||||
|
||||
<script>
|
||||
export default {
|
||||
name: 'BreadcrumbItem',
|
||||
props: {
|
||||
item: null,
|
||||
template: null,
|
||||
exact: null
|
||||
},
|
||||
methods: {
|
||||
onClick(event, navigate) {
|
||||
if (this.item.command) {
|
||||
this.item.command({
|
||||
originalEvent: event,
|
||||
item: this.item
|
||||
});
|
||||
}
|
||||
|
||||
if (this.item.to && navigate) {
|
||||
navigate(event);
|
||||
}
|
||||
},
|
||||
containerClass() {
|
||||
return ['p-menuitem', { 'p-disabled': this.disabled() }, this.item.class];
|
||||
},
|
||||
linkClass(routerProps) {
|
||||
return [
|
||||
'p-menuitem-link',
|
||||
{
|
||||
'router-link-active': routerProps && routerProps.isActive,
|
||||
'router-link-active-exact': this.exact && routerProps && routerProps.isExactActive
|
||||
}
|
||||
];
|
||||
},
|
||||
visible() {
|
||||
return typeof this.item.visible === 'function' ? this.item.visible() : this.item.visible !== false;
|
||||
},
|
||||
disabled() {
|
||||
return typeof this.item.disabled === 'function' ? this.item.disabled() : this.item.disabled;
|
||||
},
|
||||
label() {
|
||||
return typeof this.item.label === 'function' ? this.item.label() : this.item.label;
|
||||
},
|
||||
isCurrentUrl() {
|
||||
const { to, url } = this.item;
|
||||
let lastPath = this.$router ? this.$router.currentRoute.path : '';
|
||||
|
||||
return to === lastPath || url === lastPath ? 'page' : undefined;
|
||||
}
|
||||
},
|
||||
computed: {
|
||||
iconClass() {
|
||||
return ['p-menuitem-icon', this.item.icon];
|
||||
}
|
||||
}
|
||||
};
|
||||
</script>
|
10
components/lib/breadcrumb/package.json
Normal file
10
components/lib/breadcrumb/package.json
Normal file
|
@ -0,0 +1,10 @@
|
|||
{
|
||||
"main": "./breadcrumb.cjs.js",
|
||||
"module": "./breadcrumb.esm.js",
|
||||
"unpkg": "./breadcrumb.min.js",
|
||||
"types": "./Breadcrumb.d.ts"
|
||||
,
|
||||
"browser": {
|
||||
"./sfc": "./Breadcrumb.vue"
|
||||
}
|
||||
}
|
Loading…
Add table
Add a link
Reference in a new issue