mirror of
https://github.com/primefaces/primevue.git
synced 2025-05-09 08:52:34 +00:00
Refactor #5612 - Sidebar / Drawer
This commit is contained in:
parent
365879a41c
commit
fcf8599cfa
16 changed files with 614 additions and 503 deletions
|
@ -1,9 +1,9 @@
|
|||
<script>
|
||||
import BaseComponent from 'primevue/basecomponent';
|
||||
import SidebarStyle from 'primevue/sidebar/style';
|
||||
import DrawerStyle from 'primevue/drawer/style';
|
||||
|
||||
export default {
|
||||
name: 'BaseSidebar',
|
||||
name: 'BaseDrawer',
|
||||
extends: BaseComponent,
|
||||
props: {
|
||||
visible: {
|
||||
|
@ -53,7 +53,7 @@ export default {
|
|||
default: false
|
||||
}
|
||||
},
|
||||
style: SidebarStyle,
|
||||
style: DrawerStyle,
|
||||
provide() {
|
||||
return {
|
||||
$parentInstance: this
|
285
components/lib/drawer/Drawer.d.ts
vendored
Executable file
285
components/lib/drawer/Drawer.d.ts
vendored
Executable file
|
@ -0,0 +1,285 @@
|
|||
/**
|
||||
*
|
||||
* Drawer is a panel component displayed as an overlay at the edges of the screen.
|
||||
*
|
||||
* [Live Demo](https://primevue.org/drawer)
|
||||
*
|
||||
* @module drawer
|
||||
*
|
||||
*/
|
||||
import { TransitionProps, VNode } from 'vue';
|
||||
import { ComponentHooks } from '../basecomponent';
|
||||
import { ButtonPassThroughOptions } from '../button';
|
||||
import { PassThroughOptions } from '../passthrough';
|
||||
import { ClassComponent, DesignToken, GlobalComponentConstructor, PassThrough } from '../ts-helpers';
|
||||
|
||||
export declare type DrawerPassThroughOptionType = DrawerPassThroughAttributes | ((options: DrawerPassThroughMethodOptions) => DrawerPassThroughAttributes | string) | string | null | undefined;
|
||||
|
||||
export declare type DrawerPassThroughTransitionType = TransitionProps | ((options: DrawerPassThroughMethodOptions) => TransitionProps) | undefined;
|
||||
|
||||
/**
|
||||
* Custom passthrough(pt) option method.
|
||||
*/
|
||||
export interface DrawerPassThroughMethodOptions {
|
||||
/**
|
||||
* Defines instance.
|
||||
*/
|
||||
instance: any;
|
||||
/**
|
||||
* Defines valid properties.
|
||||
*/
|
||||
props: DrawerProps;
|
||||
/**
|
||||
* Defines current inline state.
|
||||
*/
|
||||
state: DrawerState;
|
||||
/**
|
||||
* Defines valid attributes.
|
||||
*/
|
||||
attrs: any;
|
||||
/**
|
||||
* Defines parent options.
|
||||
*/
|
||||
parent: any;
|
||||
/**
|
||||
* Defines passthrough(pt) options in global config.
|
||||
*/
|
||||
global: object | undefined;
|
||||
}
|
||||
|
||||
/**
|
||||
* Custom shared passthrough(pt) option method.
|
||||
*/
|
||||
export interface DrawerSharedPassThroughMethodOptions {
|
||||
/**
|
||||
* Defines valid properties.
|
||||
*/
|
||||
props: DrawerProps;
|
||||
/**
|
||||
* Defines current inline state.
|
||||
*/
|
||||
state: DrawerState;
|
||||
}
|
||||
|
||||
/**
|
||||
* Custom passthrough(pt) options.
|
||||
* @see {@link DrawerProps.pt}
|
||||
*/
|
||||
export interface DrawerPassThroughOptions {
|
||||
/**
|
||||
* Used to pass attributes to the root's DOM element.
|
||||
*/
|
||||
root?: DrawerPassThroughOptionType;
|
||||
/**
|
||||
* Used to pass attributes to the header's DOM element.
|
||||
*/
|
||||
header?: DrawerPassThroughOptionType;
|
||||
/**
|
||||
* Used to pass attributes to the header content's DOM element.
|
||||
*/
|
||||
title?: DrawerPassThroughOptionType;
|
||||
/**
|
||||
* Used to pass attributes to the previous button's DOM element.
|
||||
* @see {@link ButtonPassThroughOptions}
|
||||
*/
|
||||
toggler?: ButtonPassThroughOptions<DrawerSharedPassThroughMethodOptions>;
|
||||
/**
|
||||
* Used to pass attributes to the content's DOM element.
|
||||
*/
|
||||
content?: DrawerPassThroughOptionType;
|
||||
/**
|
||||
* Used to pass attributes to the mask's DOM element.
|
||||
*/
|
||||
mask?: DrawerPassThroughOptionType;
|
||||
/**
|
||||
* Used to manage all lifecycle hooks.
|
||||
* @see {@link BaseComponent.ComponentHooks}
|
||||
*/
|
||||
hooks?: ComponentHooks;
|
||||
/**
|
||||
* Used to control Vue Transition API.
|
||||
*/
|
||||
transition?: DrawerPassThroughTransitionType;
|
||||
}
|
||||
|
||||
/**
|
||||
* Custom passthrough attributes for each DOM elements
|
||||
*/
|
||||
export interface DrawerPassThroughAttributes {
|
||||
[key: string]: any;
|
||||
}
|
||||
|
||||
/**
|
||||
* Defines current inline state in Drawer component.
|
||||
*/
|
||||
export interface DrawerState {
|
||||
/**
|
||||
* Current container visible state as a boolean.
|
||||
* @defaultValue false
|
||||
*/
|
||||
containerVisible: boolean;
|
||||
}
|
||||
|
||||
/**
|
||||
* Defines valid properties in Drawer component.
|
||||
*/
|
||||
export interface DrawerProps {
|
||||
/**
|
||||
* Specifies the visibility of the dialog.
|
||||
* @defaultValue false
|
||||
*/
|
||||
visible?: boolean | undefined;
|
||||
/**
|
||||
* Specifies the position of the drawer.
|
||||
* @defaultValue left
|
||||
*/
|
||||
position?: 'left' | 'right' | 'top' | 'bottom' | 'full' | undefined;
|
||||
/**
|
||||
* Title content of the dialog.
|
||||
*/
|
||||
header?: string | undefined;
|
||||
/**
|
||||
* Base zIndex value to use in layering.
|
||||
* @defaultValue 0
|
||||
*/
|
||||
baseZIndex?: number | undefined;
|
||||
/**
|
||||
* Whether to automatically manage layering.
|
||||
* @defaultValue true
|
||||
*/
|
||||
autoZIndex?: boolean | undefined;
|
||||
/**
|
||||
* Whether clicking outside closes the panel.
|
||||
* @defaultValue true
|
||||
*/
|
||||
dismissable?: boolean | undefined;
|
||||
/**
|
||||
* Whether to display a close icon inside the panel.
|
||||
* @defaultValue true
|
||||
*/
|
||||
showCloseIcon?: boolean | undefined;
|
||||
/**
|
||||
* Used to pass the custom value to read for the button inside the component.
|
||||
* @defaultValue { severity: 'secondary', text: true, rounded: true }
|
||||
*/
|
||||
closeButtonProps?: object | undefined;
|
||||
/**
|
||||
* Icon to display in the drawer close button.
|
||||
* @deprecated since v3.27.0. Use 'closeicon' slot.
|
||||
*/
|
||||
closeIcon?: string | undefined;
|
||||
/**
|
||||
* Whether to a modal layer behind the drawer.
|
||||
* @defaultValue true
|
||||
*/
|
||||
modal?: boolean | undefined;
|
||||
/**
|
||||
* Whether background scroll should be blocked when drawer is visible.
|
||||
* @defaultValue false
|
||||
*/
|
||||
blockScroll?: boolean | undefined;
|
||||
/**
|
||||
* It generates scoped CSS variables using design tokens for the component.
|
||||
*/
|
||||
dt?: DesignToken<any>;
|
||||
/**
|
||||
* Used to pass attributes to DOM elements inside the component.
|
||||
* @type {DrawerPassThroughOptions}
|
||||
*/
|
||||
pt?: PassThrough<DrawerPassThroughOptions>;
|
||||
/**
|
||||
* Used to configure passthrough(pt) options of the component.
|
||||
* @type {PassThroughOptions}
|
||||
*/
|
||||
ptOptions?: PassThroughOptions;
|
||||
/**
|
||||
* When enabled, it removes component related styles in the core.
|
||||
* @defaultValue false
|
||||
*/
|
||||
unstyled?: boolean;
|
||||
}
|
||||
|
||||
/**
|
||||
* Defines valid slots in Drawer component.
|
||||
*/
|
||||
export interface DrawerSlots {
|
||||
/**
|
||||
* Custom content template.
|
||||
*/
|
||||
default(): VNode[];
|
||||
/**
|
||||
* Custom header template.
|
||||
* @param {Object} scope - header slot's params.
|
||||
*/
|
||||
header(scope: {
|
||||
/**
|
||||
* Style class of the header title
|
||||
*/
|
||||
class: any;
|
||||
}): VNode[];
|
||||
/**
|
||||
* Custom close icon template.
|
||||
* @param {Object} scope - close icon slot's params.
|
||||
*/
|
||||
closeicon(scope: {
|
||||
/**
|
||||
* Style class of the close icon
|
||||
*/
|
||||
class: any;
|
||||
}): VNode[];
|
||||
/**
|
||||
* Custom container slot.
|
||||
* @param {Object} scope - container slot's params.
|
||||
*/
|
||||
container(scope: {
|
||||
/**
|
||||
* Close drawer function.
|
||||
* @deprecated since v3.39.0. Use 'closeCallback' property instead.
|
||||
*/
|
||||
onClose: () => void;
|
||||
/**
|
||||
* Close drawer function.
|
||||
*/
|
||||
closeCallback: () => void;
|
||||
}): VNode[];
|
||||
}
|
||||
|
||||
/**
|
||||
* Defines valid emits in Drawer component.
|
||||
*/
|
||||
export interface DrawerEmits {
|
||||
/**
|
||||
* Emitted when the value changes.
|
||||
* @param {boolean} value - New value.
|
||||
*/
|
||||
'update:modelValue'(value: boolean): void;
|
||||
/**
|
||||
* Callback to invoke when drawer gets shown.
|
||||
*/
|
||||
show(): void;
|
||||
/**
|
||||
* Callback to invoke when drawer gets hidden.
|
||||
*/
|
||||
hide(): void;
|
||||
}
|
||||
|
||||
/**
|
||||
* **PrimeVue - Drawer**
|
||||
*
|
||||
* _Drawer is a panel component displayed as an overlay._
|
||||
*
|
||||
* [Live Demo](https://www.primevue.org/drawer/)
|
||||
* --- ---
|
||||
* 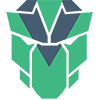
|
||||
*
|
||||
* @group Component
|
||||
*/
|
||||
declare class Drawer extends ClassComponent<DrawerProps, DrawerSlots, DrawerEmits> {}
|
||||
|
||||
declare module 'vue' {
|
||||
export interface GlobalComponents {
|
||||
Drawer: GlobalComponentConstructor<Drawer>;
|
||||
}
|
||||
}
|
||||
|
||||
export default Drawer;
|
|
@ -2,12 +2,13 @@ import { mount } from '@vue/test-utils';
|
|||
import PrimeVue from 'primevue/config';
|
||||
import { describe, expect, it } from 'vitest';
|
||||
import DomHandler from '../utils/DomHandler';
|
||||
import Sidebar from './Sidebar.vue';
|
||||
describe('Sidebar.vue', () => {
|
||||
import Drawer from './Drawer.vue';
|
||||
|
||||
describe('Drawer.vue', () => {
|
||||
let wrapper;
|
||||
|
||||
beforeEach(async () => {
|
||||
wrapper = mount(Sidebar, {
|
||||
wrapper = mount(Drawer, {
|
||||
global: {
|
||||
plugins: [PrimeVue],
|
||||
stubs: {
|
||||
|
@ -19,7 +20,7 @@ describe('Sidebar.vue', () => {
|
|||
bazeZIndex: 1000
|
||||
},
|
||||
slots: {
|
||||
default: `<h3>Left Sidebar</h3>`,
|
||||
default: `<h3>Left Drawer</h3>`,
|
||||
header: `<span class="header">Header Template</span>`
|
||||
}
|
||||
});
|
||||
|
@ -29,16 +30,16 @@ describe('Sidebar.vue', () => {
|
|||
vi.clearAllMocks();
|
||||
});
|
||||
|
||||
it('When mask element triggered, sidebar should be hide', async () => {
|
||||
it('When mask element triggered, drawer should be hide', async () => {
|
||||
const hideSpy = vi.spyOn(wrapper.vm, 'hide');
|
||||
|
||||
await wrapper.find('.p-sidebar-mask').trigger('mousedown');
|
||||
await wrapper.find('.p-drawer-mask').trigger('mousedown');
|
||||
|
||||
expect(wrapper.emitted()['update:visible'].length).toBe(1);
|
||||
expect(hideSpy).toHaveBeenCalled();
|
||||
});
|
||||
|
||||
it('When transition trigger to onEnter, sidebar should be visible', async () => {
|
||||
it('When transition trigger to onEnter, drawer should be visible', async () => {
|
||||
const focusSpy = vi.spyOn(wrapper.vm, 'focus');
|
||||
|
||||
await wrapper.vm.onEnter();
|
||||
|
@ -73,7 +74,7 @@ describe('Sidebar.vue', () => {
|
|||
it('When keydown is triggered , hide method should be triggered', async () => {
|
||||
const hideSpy = vi.spyOn(wrapper.vm, 'hide');
|
||||
|
||||
await wrapper.find('.p-sidebar-close').trigger('click');
|
||||
await wrapper.find('.p-drawer-close').trigger('click');
|
||||
|
||||
expect(hideSpy).toHaveBeenCalled();
|
||||
});
|
||||
|
@ -84,7 +85,7 @@ describe('Sidebar.vue', () => {
|
|||
await wrapper.unmount();
|
||||
|
||||
expect(unbindOutsideClickListenerSpy).toHaveBeenCalled();
|
||||
expect(Sidebar.container).toBe(null);
|
||||
expect(Drawer.container).toBe(null);
|
||||
});
|
||||
|
||||
it('When hide is triggered , removeClass util should be called', async () => {
|
225
components/lib/drawer/Drawer.vue
Executable file
225
components/lib/drawer/Drawer.vue
Executable file
|
@ -0,0 +1,225 @@
|
|||
<template>
|
||||
<Portal>
|
||||
<div v-if="containerVisible" :ref="maskRef" @mousedown="onMaskClick" :class="cx('mask')" :style="sx('mask', true, { position })" v-bind="ptm('mask')">
|
||||
<transition name="p-drawer" @enter="onEnter" @after-enter="onAfterEnter" @before-leave="onBeforeLeave" @leave="onLeave" @after-leave="onAfterLeave" appear v-bind="ptm('transition')">
|
||||
<div v-if="visible" :ref="containerRef" v-focustrap :class="cx('root')" role="complementary" :aria-modal="modal" v-bind="ptmi('root')">
|
||||
<slot v-if="$slots.container" name="container" :onClose="hide" :closeCallback="hide"></slot>
|
||||
<template v-else>
|
||||
<div :ref="headerContainerRef" :class="cx('header')" v-bind="ptm('header')">
|
||||
<slot name="header" :class="cx('title')">
|
||||
<div v-if="header" :class="cx('title')" v-bind="ptm('title')">{{ header }}</div>
|
||||
</slot>
|
||||
<Button
|
||||
v-if="showCloseIcon"
|
||||
:ref="closeButtonRef"
|
||||
type="button"
|
||||
:class="cx('closeButton')"
|
||||
:aria-label="closeAriaLabel"
|
||||
:unstyled="unstyled"
|
||||
@click="hide"
|
||||
v-bind="closeButtonProps"
|
||||
:pt="ptm('closeButton')"
|
||||
data-pc-group-section="iconcontainer"
|
||||
>
|
||||
<template #icon="slotProps">
|
||||
<slot name="closeicon" :class="cx('closeIcon')">
|
||||
<component :is="closeIcon ? 'span' : 'TimesIcon'" :class="[cx('closeIcon'), closeIcon, slotProps.class]" v-bind="ptm('closeButton')['icon']"></component>
|
||||
</slot>
|
||||
</template>
|
||||
</Button>
|
||||
</div>
|
||||
<div :ref="contentRef" :class="cx('content')" v-bind="ptm('content')">
|
||||
<slot></slot>
|
||||
</div>
|
||||
</template>
|
||||
</div>
|
||||
</transition>
|
||||
</div>
|
||||
</Portal>
|
||||
</template>
|
||||
|
||||
<script>
|
||||
import Button from 'primevue/button';
|
||||
import FocusTrap from 'primevue/focustrap';
|
||||
import TimesIcon from 'primevue/icons/times';
|
||||
import Portal from 'primevue/portal';
|
||||
import Ripple from 'primevue/ripple';
|
||||
import { DomHandler, ZIndexUtils } from 'primevue/utils';
|
||||
import BaseDrawer from './BaseDrawer.vue';
|
||||
|
||||
export default {
|
||||
name: 'Drawer',
|
||||
extends: BaseDrawer,
|
||||
inheritAttrs: false,
|
||||
emits: ['update:visible', 'show', 'hide', 'after-hide'],
|
||||
data() {
|
||||
return {
|
||||
containerVisible: this.visible
|
||||
};
|
||||
},
|
||||
container: null,
|
||||
mask: null,
|
||||
content: null,
|
||||
headerContainer: null,
|
||||
closeButton: null,
|
||||
outsideClickListener: null,
|
||||
documentKeydownListener: null,
|
||||
updated() {
|
||||
if (this.visible) {
|
||||
this.containerVisible = this.visible;
|
||||
}
|
||||
},
|
||||
beforeUnmount() {
|
||||
this.disableDocumentSettings();
|
||||
|
||||
if (this.mask && this.autoZIndex) {
|
||||
ZIndexUtils.clear(this.mask);
|
||||
}
|
||||
|
||||
this.container = null;
|
||||
this.mask = null;
|
||||
},
|
||||
methods: {
|
||||
hide() {
|
||||
this.$emit('update:visible', false);
|
||||
},
|
||||
onEnter() {
|
||||
this.$emit('show');
|
||||
this.focus();
|
||||
this.bindDocumentKeyDownListener();
|
||||
|
||||
if (this.autoZIndex) {
|
||||
ZIndexUtils.set('modal', this.mask, this.baseZIndex || this.$primevue.config.zIndex.modal);
|
||||
}
|
||||
},
|
||||
onAfterEnter() {
|
||||
this.enableDocumentSettings();
|
||||
},
|
||||
onBeforeLeave() {
|
||||
if (this.modal) {
|
||||
!this.isUnstyled && DomHandler.addClass(this.mask, 'p-component-overlay-leave');
|
||||
}
|
||||
},
|
||||
onLeave() {
|
||||
this.$emit('hide');
|
||||
},
|
||||
onAfterLeave() {
|
||||
if (this.autoZIndex) {
|
||||
ZIndexUtils.clear(this.mask);
|
||||
}
|
||||
|
||||
this.unbindDocumentKeyDownListener();
|
||||
this.containerVisible = false;
|
||||
this.disableDocumentSettings();
|
||||
this.$emit('after-hide');
|
||||
},
|
||||
onMaskClick(event) {
|
||||
if (this.dismissable && this.modal && this.mask === event.target) {
|
||||
this.hide();
|
||||
}
|
||||
},
|
||||
focus() {
|
||||
const findFocusableElement = (container) => {
|
||||
return container && container.querySelector('[autofocus]');
|
||||
};
|
||||
|
||||
let focusTarget = this.$slots.header && findFocusableElement(this.headerContainer);
|
||||
|
||||
if (!focusTarget) {
|
||||
focusTarget = this.$slots.default && findFocusableElement(this.container);
|
||||
|
||||
if (!focusTarget) {
|
||||
focusTarget = this.closeButton;
|
||||
}
|
||||
}
|
||||
|
||||
focusTarget && DomHandler.focus(focusTarget);
|
||||
},
|
||||
enableDocumentSettings() {
|
||||
if (this.dismissable && !this.modal) {
|
||||
this.bindOutsideClickListener();
|
||||
}
|
||||
|
||||
if (this.blockScroll) {
|
||||
DomHandler.blockBodyScroll();
|
||||
}
|
||||
},
|
||||
disableDocumentSettings() {
|
||||
this.unbindOutsideClickListener();
|
||||
|
||||
if (this.blockScroll) {
|
||||
DomHandler.unblockBodyScroll();
|
||||
}
|
||||
},
|
||||
onKeydown(event) {
|
||||
if (event.code === 'Escape') {
|
||||
this.hide();
|
||||
}
|
||||
},
|
||||
containerRef(el) {
|
||||
this.container = el;
|
||||
},
|
||||
maskRef(el) {
|
||||
this.mask = el;
|
||||
},
|
||||
contentRef(el) {
|
||||
this.content = el;
|
||||
},
|
||||
headerContainerRef(el) {
|
||||
this.headerContainer = el;
|
||||
},
|
||||
closeButtonRef(el) {
|
||||
this.closeButton = el ? el.$el : undefined;
|
||||
},
|
||||
bindDocumentKeyDownListener() {
|
||||
if (!this.documentKeydownListener) {
|
||||
this.documentKeydownListener = this.onKeydown;
|
||||
document.addEventListener('keydown', this.documentKeydownListener);
|
||||
}
|
||||
},
|
||||
unbindDocumentKeyDownListener() {
|
||||
if (this.documentKeydownListener) {
|
||||
document.removeEventListener('keydown', this.documentKeydownListener);
|
||||
this.documentKeydownListener = null;
|
||||
}
|
||||
},
|
||||
bindOutsideClickListener() {
|
||||
if (!this.outsideClickListener) {
|
||||
this.outsideClickListener = (event) => {
|
||||
if (this.isOutsideClicked(event)) {
|
||||
this.hide();
|
||||
}
|
||||
};
|
||||
|
||||
document.addEventListener('click', this.outsideClickListener);
|
||||
}
|
||||
},
|
||||
unbindOutsideClickListener() {
|
||||
if (this.outsideClickListener) {
|
||||
document.removeEventListener('click', this.outsideClickListener);
|
||||
this.outsideClickListener = null;
|
||||
}
|
||||
},
|
||||
isOutsideClicked(event) {
|
||||
return this.container && !this.container.contains(event.target);
|
||||
}
|
||||
},
|
||||
computed: {
|
||||
fullScreen() {
|
||||
return this.position === 'full';
|
||||
},
|
||||
closeAriaLabel() {
|
||||
return this.$primevue.config.locale.aria ? this.$primevue.config.locale.aria.close : undefined;
|
||||
}
|
||||
},
|
||||
directives: {
|
||||
focustrap: FocusTrap,
|
||||
ripple: Ripple
|
||||
},
|
||||
components: {
|
||||
Button,
|
||||
Portal,
|
||||
TimesIcon
|
||||
}
|
||||
};
|
||||
</script>
|
9
components/lib/drawer/package.json
Normal file
9
components/lib/drawer/package.json
Normal file
|
@ -0,0 +1,9 @@
|
|||
{
|
||||
"main": "./drawer.cjs.js",
|
||||
"module": "./drawer.esm.js",
|
||||
"unpkg": "./drawer.min.js",
|
||||
"types": "./Drawer.d.ts",
|
||||
"browser": {
|
||||
"./sfc": "./Drawer.vue"
|
||||
}
|
||||
}
|
3
components/lib/drawer/style/DrawerStyle.d.ts
vendored
Normal file
3
components/lib/drawer/style/DrawerStyle.d.ts
vendored
Normal file
|
@ -0,0 +1,3 @@
|
|||
import { BaseStyle } from '../../base/style';
|
||||
|
||||
export interface DrawerStyle extends BaseStyle {}
|
48
components/lib/drawer/style/DrawerStyle.js
Normal file
48
components/lib/drawer/style/DrawerStyle.js
Normal file
|
@ -0,0 +1,48 @@
|
|||
import BaseStyle from 'primevue/base/style';
|
||||
|
||||
const inlineStyles = {
|
||||
mask: ({ position }) => ({
|
||||
position: 'fixed',
|
||||
height: '100%',
|
||||
width: '100%',
|
||||
left: 0,
|
||||
top: 0,
|
||||
display: 'flex',
|
||||
justifyContent: position === 'left' ? 'flex-start' : position === 'right' ? 'flex-end' : 'center',
|
||||
alignItems: position === 'top' ? 'flex-start' : position === 'bottom' ? 'flex-end' : 'center'
|
||||
})
|
||||
};
|
||||
|
||||
const classes = {
|
||||
mask: ({ instance, props }) => {
|
||||
const positions = ['left', 'right', 'top', 'bottom'];
|
||||
const pos = positions.find((item) => item === props.position);
|
||||
|
||||
return [
|
||||
'p-drawer-mask',
|
||||
{
|
||||
'p-component-overlay p-component-overlay-enter': props.modal,
|
||||
'p-drawer-open': instance.containerVisible,
|
||||
'p-drawer-full': instance.fullScreen
|
||||
},
|
||||
pos ? `p-drawer-${pos}` : ''
|
||||
];
|
||||
},
|
||||
root: ({ instance }) => [
|
||||
'p-drawer p-component',
|
||||
{
|
||||
'p-ripple-disabled': instance.$primevue.config.ripple === false,
|
||||
'p-drawer-full': instance.fullScreen
|
||||
}
|
||||
],
|
||||
header: 'p-drawer-header',
|
||||
title: 'p-drawer-title',
|
||||
closeButton: 'p-drawer-close-button',
|
||||
content: 'p-drawer-content'
|
||||
};
|
||||
|
||||
export default BaseStyle.extend({
|
||||
name: 'drawer',
|
||||
classes,
|
||||
inlineStyles
|
||||
});
|
6
components/lib/drawer/style/package.json
Normal file
6
components/lib/drawer/style/package.json
Normal file
|
@ -0,0 +1,6 @@
|
|||
{
|
||||
"main": "./drawerstyle.cjs.js",
|
||||
"module": "./drawerstyle.esm.js",
|
||||
"unpkg": "./drawerstyle.min.js",
|
||||
"types": "./DrawerStyle.d.ts"
|
||||
}
|
239
components/lib/sidebar/Sidebar.d.ts
vendored
239
components/lib/sidebar/Sidebar.d.ts
vendored
|
@ -2,273 +2,64 @@
|
|||
*
|
||||
* Sidebar is a panel component displayed as an overlay at the edges of the screen.
|
||||
*
|
||||
* [Live Demo](https://primevue.org/sidebar)
|
||||
* [Live Demo](https://primevue.org/drawer)
|
||||
*
|
||||
* @module sidebar
|
||||
*
|
||||
*/
|
||||
import { TransitionProps, VNode } from 'vue';
|
||||
import { ComponentHooks } from '../basecomponent';
|
||||
import { ButtonPassThroughOptions } from '../button';
|
||||
import { PassThroughOptions } from '../passthrough';
|
||||
import { ClassComponent, DesignToken, GlobalComponentConstructor, PassThrough } from '../ts-helpers';
|
||||
|
||||
export declare type SidebarPassThroughOptionType = SidebarPassThroughAttributes | ((options: SidebarPassThroughMethodOptions) => SidebarPassThroughAttributes | string) | string | null | undefined;
|
||||
|
||||
export declare type SidebarPassThroughTransitionType = TransitionProps | ((options: SidebarPassThroughMethodOptions) => TransitionProps) | undefined;
|
||||
import 'vue';
|
||||
import * as Drawer from '../drawer';
|
||||
import { ClassComponent, GlobalComponentConstructor } from '../ts-helpers';
|
||||
|
||||
/**
|
||||
* Custom passthrough(pt) option method.
|
||||
*/
|
||||
export interface SidebarPassThroughMethodOptions {
|
||||
/**
|
||||
* Defines instance.
|
||||
*/
|
||||
instance: any;
|
||||
/**
|
||||
* Defines valid properties.
|
||||
*/
|
||||
props: SidebarProps;
|
||||
/**
|
||||
* Defines current inline state.
|
||||
*/
|
||||
state: SidebarState;
|
||||
/**
|
||||
* Defines valid attributes.
|
||||
*/
|
||||
attrs: any;
|
||||
/**
|
||||
* Defines parent options.
|
||||
*/
|
||||
parent: any;
|
||||
/**
|
||||
* Defines passthrough(pt) options in global config.
|
||||
*/
|
||||
global: object | undefined;
|
||||
}
|
||||
export interface SidebarPassThroughMethodOptions extends Drawer.DrawerPassThroughMethodOptions {}
|
||||
|
||||
/**
|
||||
* Custom shared passthrough(pt) option method.
|
||||
*/
|
||||
export interface SidebarSharedPassThroughMethodOptions {
|
||||
/**
|
||||
* Defines valid properties.
|
||||
*/
|
||||
props: SidebarProps;
|
||||
/**
|
||||
* Defines current inline state.
|
||||
*/
|
||||
state: SidebarState;
|
||||
}
|
||||
export interface SidebarSharedPassThroughMethodOptions extends Drawer.DrawerSharedPassThroughMethodOptions {}
|
||||
|
||||
/**
|
||||
* Custom passthrough(pt) options.
|
||||
* @see {@link SidebarProps.pt}
|
||||
*/
|
||||
export interface SidebarPassThroughOptions {
|
||||
/**
|
||||
* Used to pass attributes to the root's DOM element.
|
||||
*/
|
||||
root?: SidebarPassThroughOptionType;
|
||||
/**
|
||||
* Used to pass attributes to the header's DOM element.
|
||||
*/
|
||||
header?: SidebarPassThroughOptionType;
|
||||
/**
|
||||
* Used to pass attributes to the header content's DOM element.
|
||||
*/
|
||||
title?: SidebarPassThroughOptionType;
|
||||
/**
|
||||
* Used to pass attributes to the previous button's DOM element.
|
||||
* @see {@link ButtonPassThroughOptions}
|
||||
*/
|
||||
toggler?: ButtonPassThroughOptions<SidebarSharedPassThroughMethodOptions>;
|
||||
/**
|
||||
* Used to pass attributes to the content's DOM element.
|
||||
*/
|
||||
content?: SidebarPassThroughOptionType;
|
||||
/**
|
||||
* Used to pass attributes to the mask's DOM element.
|
||||
*/
|
||||
mask?: SidebarPassThroughOptionType;
|
||||
/**
|
||||
* Used to manage all lifecycle hooks.
|
||||
* @see {@link BaseComponent.ComponentHooks}
|
||||
*/
|
||||
hooks?: ComponentHooks;
|
||||
/**
|
||||
* Used to control Vue Transition API.
|
||||
*/
|
||||
transition?: SidebarPassThroughTransitionType;
|
||||
}
|
||||
export interface SidebarPassThroughOptions extends Drawer.DrawerPassThroughOptions {}
|
||||
|
||||
/**
|
||||
* Custom passthrough attributes for each DOM elements
|
||||
*/
|
||||
export interface SidebarPassThroughAttributes {
|
||||
[key: string]: any;
|
||||
}
|
||||
export interface SidebarPassThroughAttributes extends Drawer.DrawerPassThroughAttributes {}
|
||||
|
||||
/**
|
||||
* Defines current inline state in Sidebar component.
|
||||
*/
|
||||
export interface SidebarState {
|
||||
/**
|
||||
* Current container visible state as a boolean.
|
||||
* @defaultValue false
|
||||
*/
|
||||
containerVisible: boolean;
|
||||
}
|
||||
export interface SidebarState extends Drawer.DrawerState {}
|
||||
|
||||
/**
|
||||
* Defines valid properties in Sidebar component.
|
||||
*/
|
||||
export interface SidebarProps {
|
||||
/**
|
||||
* Specifies the visibility of the dialog.
|
||||
* @defaultValue false
|
||||
*/
|
||||
visible?: boolean | undefined;
|
||||
/**
|
||||
* Specifies the position of the sidebar.
|
||||
* @defaultValue left
|
||||
*/
|
||||
position?: 'left' | 'right' | 'top' | 'bottom' | 'full' | undefined;
|
||||
/**
|
||||
* Title content of the dialog.
|
||||
*/
|
||||
header?: string | undefined;
|
||||
/**
|
||||
* Base zIndex value to use in layering.
|
||||
* @defaultValue 0
|
||||
*/
|
||||
baseZIndex?: number | undefined;
|
||||
/**
|
||||
* Whether to automatically manage layering.
|
||||
* @defaultValue true
|
||||
*/
|
||||
autoZIndex?: boolean | undefined;
|
||||
/**
|
||||
* Whether clicking outside closes the panel.
|
||||
* @defaultValue true
|
||||
*/
|
||||
dismissable?: boolean | undefined;
|
||||
/**
|
||||
* Whether to display a close icon inside the panel.
|
||||
* @defaultValue true
|
||||
*/
|
||||
showCloseIcon?: boolean | undefined;
|
||||
/**
|
||||
* Used to pass the custom value to read for the button inside the component.
|
||||
* @defaultValue { severity: 'secondary', text: true, rounded: true }
|
||||
*/
|
||||
closeButtonProps?: object | undefined;
|
||||
/**
|
||||
* Icon to display in the sidebar close button.
|
||||
* @deprecated since v3.27.0. Use 'closeicon' slot.
|
||||
*/
|
||||
closeIcon?: string | undefined;
|
||||
/**
|
||||
* Whether to a modal layer behind the sidebar.
|
||||
* @defaultValue true
|
||||
*/
|
||||
modal?: boolean | undefined;
|
||||
/**
|
||||
* Whether background scroll should be blocked when sidebar is visible.
|
||||
* @defaultValue false
|
||||
*/
|
||||
blockScroll?: boolean | undefined;
|
||||
/**
|
||||
* It generates scoped CSS variables using design tokens for the component.
|
||||
*/
|
||||
dt?: DesignToken<any>;
|
||||
/**
|
||||
* Used to pass attributes to DOM elements inside the component.
|
||||
* @type {SidebarPassThroughOptions}
|
||||
*/
|
||||
pt?: PassThrough<SidebarPassThroughOptions>;
|
||||
/**
|
||||
* Used to configure passthrough(pt) options of the component.
|
||||
* @type {PassThroughOptions}
|
||||
*/
|
||||
ptOptions?: PassThroughOptions;
|
||||
/**
|
||||
* When enabled, it removes component related styles in the core.
|
||||
* @defaultValue false
|
||||
*/
|
||||
unstyled?: boolean;
|
||||
}
|
||||
export interface SidebarProps extends Drawer.DrawerProps {}
|
||||
|
||||
/**
|
||||
* Defines valid slots in Sidebar component.
|
||||
*/
|
||||
export interface SidebarSlots {
|
||||
/**
|
||||
* Custom content template.
|
||||
*/
|
||||
default(): VNode[];
|
||||
/**
|
||||
* Custom header template.
|
||||
* @param {Object} scope - header slot's params.
|
||||
*/
|
||||
header(scope: {
|
||||
/**
|
||||
* Style class of the header title
|
||||
*/
|
||||
class: any;
|
||||
}): VNode[];
|
||||
/**
|
||||
* Custom close icon template.
|
||||
* @param {Object} scope - close icon slot's params.
|
||||
*/
|
||||
closeicon(scope: {
|
||||
/**
|
||||
* Style class of the close icon
|
||||
*/
|
||||
class: any;
|
||||
}): VNode[];
|
||||
/**
|
||||
* Custom container slot.
|
||||
* @param {Object} scope - container slot's params.
|
||||
*/
|
||||
container(scope: {
|
||||
/**
|
||||
* Close sidebar function.
|
||||
* @deprecated since v3.39.0. Use 'closeCallback' property instead.
|
||||
*/
|
||||
onClose: () => void;
|
||||
/**
|
||||
* Close sidebar function.
|
||||
*/
|
||||
closeCallback: () => void;
|
||||
}): VNode[];
|
||||
}
|
||||
export interface SidebarSlots extends Drawer.DrawerSlots {}
|
||||
|
||||
/**
|
||||
* Defines valid emits in Sidebar component.
|
||||
*/
|
||||
export interface SidebarEmits {
|
||||
/**
|
||||
* Emitted when the value changes.
|
||||
* @param {boolean} value - New value.
|
||||
*/
|
||||
'update:modelValue'(value: boolean): void;
|
||||
/**
|
||||
* Callback to invoke when sidebar gets shown.
|
||||
*/
|
||||
show(): void;
|
||||
/**
|
||||
* Callback to invoke when sidebar gets hidden.
|
||||
*/
|
||||
hide(): void;
|
||||
}
|
||||
export interface SidebarEmits extends Drawer.DrawerEmits {}
|
||||
|
||||
/**
|
||||
* @deprecated Deprecated since v4. Use Drawer component instead.
|
||||
*
|
||||
* **PrimeVue - Sidebar**
|
||||
*
|
||||
* _Sidebar is a panel component displayed as an overlay._
|
||||
*
|
||||
* [Live Demo](https://www.primevue.org/sidebar/)
|
||||
* [Live Demo](https://www.primevue.org/drawer/)
|
||||
* --- ---
|
||||
* 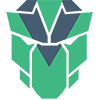
|
||||
*
|
||||
|
|
222
components/lib/sidebar/Sidebar.vue
Executable file → Normal file
222
components/lib/sidebar/Sidebar.vue
Executable file → Normal file
|
@ -1,225 +1,11 @@
|
|||
<template>
|
||||
<Portal>
|
||||
<div v-if="containerVisible" :ref="maskRef" @mousedown="onMaskClick" :class="cx('mask')" :style="sx('mask', true, { position })" v-bind="ptm('mask')">
|
||||
<transition name="p-drawer" @enter="onEnter" @after-enter="onAfterEnter" @before-leave="onBeforeLeave" @leave="onLeave" @after-leave="onAfterLeave" appear v-bind="ptm('transition')">
|
||||
<div v-if="visible" :ref="containerRef" v-focustrap :class="cx('root')" role="complementary" :aria-modal="modal" v-bind="ptmi('root')">
|
||||
<slot v-if="$slots.container" name="container" :onClose="hide" :closeCallback="hide"></slot>
|
||||
<template v-else>
|
||||
<div :ref="headerContainerRef" :class="cx('header')" v-bind="ptm('header')">
|
||||
<slot name="header" :class="cx('title')">
|
||||
<div v-if="header" :class="cx('title')" v-bind="ptm('title')">{{ header }}</div>
|
||||
</slot>
|
||||
<Button
|
||||
v-if="showCloseIcon"
|
||||
:ref="closeButtonRef"
|
||||
type="button"
|
||||
:class="cx('closeButton')"
|
||||
:aria-label="closeAriaLabel"
|
||||
:unstyled="unstyled"
|
||||
@click="hide"
|
||||
v-bind="closeButtonProps"
|
||||
:pt="ptm('closeButton')"
|
||||
data-pc-group-section="iconcontainer"
|
||||
>
|
||||
<template #icon="slotProps">
|
||||
<slot name="closeicon" :class="cx('closeIcon')">
|
||||
<component :is="closeIcon ? 'span' : 'TimesIcon'" :class="[cx('closeIcon'), closeIcon, slotProps.class]" v-bind="ptm('closeButton')['icon']"></component>
|
||||
</slot>
|
||||
</template>
|
||||
</Button>
|
||||
</div>
|
||||
<div :ref="contentRef" :class="cx('content')" v-bind="ptm('content')">
|
||||
<slot></slot>
|
||||
</div>
|
||||
</template>
|
||||
</div>
|
||||
</transition>
|
||||
</div>
|
||||
</Portal>
|
||||
</template>
|
||||
|
||||
<script>
|
||||
import Button from 'primevue/button';
|
||||
import FocusTrap from 'primevue/focustrap';
|
||||
import TimesIcon from 'primevue/icons/times';
|
||||
import Portal from 'primevue/portal';
|
||||
import Ripple from 'primevue/ripple';
|
||||
import { DomHandler, ZIndexUtils } from 'primevue/utils';
|
||||
import BaseSidebar from './BaseSidebar.vue';
|
||||
import Drawer from 'primevue/drawer';
|
||||
|
||||
export default {
|
||||
name: 'Sidebar',
|
||||
extends: BaseSidebar,
|
||||
inheritAttrs: false,
|
||||
emits: ['update:visible', 'show', 'hide', 'after-hide'],
|
||||
data() {
|
||||
return {
|
||||
containerVisible: this.visible
|
||||
};
|
||||
},
|
||||
container: null,
|
||||
mask: null,
|
||||
content: null,
|
||||
headerContainer: null,
|
||||
closeButton: null,
|
||||
outsideClickListener: null,
|
||||
documentKeydownListener: null,
|
||||
updated() {
|
||||
if (this.visible) {
|
||||
this.containerVisible = this.visible;
|
||||
}
|
||||
},
|
||||
beforeUnmount() {
|
||||
this.disableDocumentSettings();
|
||||
|
||||
if (this.mask && this.autoZIndex) {
|
||||
ZIndexUtils.clear(this.mask);
|
||||
}
|
||||
|
||||
this.container = null;
|
||||
this.mask = null;
|
||||
},
|
||||
methods: {
|
||||
hide() {
|
||||
this.$emit('update:visible', false);
|
||||
},
|
||||
onEnter() {
|
||||
this.$emit('show');
|
||||
this.focus();
|
||||
this.bindDocumentKeyDownListener();
|
||||
|
||||
if (this.autoZIndex) {
|
||||
ZIndexUtils.set('modal', this.mask, this.baseZIndex || this.$primevue.config.zIndex.modal);
|
||||
}
|
||||
},
|
||||
onAfterEnter() {
|
||||
this.enableDocumentSettings();
|
||||
},
|
||||
onBeforeLeave() {
|
||||
if (this.modal) {
|
||||
!this.isUnstyled && DomHandler.addClass(this.mask, 'p-component-overlay-leave');
|
||||
}
|
||||
},
|
||||
onLeave() {
|
||||
this.$emit('hide');
|
||||
},
|
||||
onAfterLeave() {
|
||||
if (this.autoZIndex) {
|
||||
ZIndexUtils.clear(this.mask);
|
||||
}
|
||||
|
||||
this.unbindDocumentKeyDownListener();
|
||||
this.containerVisible = false;
|
||||
this.disableDocumentSettings();
|
||||
this.$emit('after-hide');
|
||||
},
|
||||
onMaskClick(event) {
|
||||
if (this.dismissable && this.modal && this.mask === event.target) {
|
||||
this.hide();
|
||||
}
|
||||
},
|
||||
focus() {
|
||||
const findFocusableElement = (container) => {
|
||||
return container && container.querySelector('[autofocus]');
|
||||
};
|
||||
|
||||
let focusTarget = this.$slots.header && findFocusableElement(this.headerContainer);
|
||||
|
||||
if (!focusTarget) {
|
||||
focusTarget = this.$slots.default && findFocusableElement(this.container);
|
||||
|
||||
if (!focusTarget) {
|
||||
focusTarget = this.closeButton;
|
||||
}
|
||||
}
|
||||
|
||||
focusTarget && DomHandler.focus(focusTarget);
|
||||
},
|
||||
enableDocumentSettings() {
|
||||
if (this.dismissable && !this.modal) {
|
||||
this.bindOutsideClickListener();
|
||||
}
|
||||
|
||||
if (this.blockScroll) {
|
||||
DomHandler.blockBodyScroll();
|
||||
}
|
||||
},
|
||||
disableDocumentSettings() {
|
||||
this.unbindOutsideClickListener();
|
||||
|
||||
if (this.blockScroll) {
|
||||
DomHandler.unblockBodyScroll();
|
||||
}
|
||||
},
|
||||
onKeydown(event) {
|
||||
if (event.code === 'Escape') {
|
||||
this.hide();
|
||||
}
|
||||
},
|
||||
containerRef(el) {
|
||||
this.container = el;
|
||||
},
|
||||
maskRef(el) {
|
||||
this.mask = el;
|
||||
},
|
||||
contentRef(el) {
|
||||
this.content = el;
|
||||
},
|
||||
headerContainerRef(el) {
|
||||
this.headerContainer = el;
|
||||
},
|
||||
closeButtonRef(el) {
|
||||
this.closeButton = el ? el.$el : undefined;
|
||||
},
|
||||
bindDocumentKeyDownListener() {
|
||||
if (!this.documentKeydownListener) {
|
||||
this.documentKeydownListener = this.onKeydown;
|
||||
document.addEventListener('keydown', this.documentKeydownListener);
|
||||
}
|
||||
},
|
||||
unbindDocumentKeyDownListener() {
|
||||
if (this.documentKeydownListener) {
|
||||
document.removeEventListener('keydown', this.documentKeydownListener);
|
||||
this.documentKeydownListener = null;
|
||||
}
|
||||
},
|
||||
bindOutsideClickListener() {
|
||||
if (!this.outsideClickListener) {
|
||||
this.outsideClickListener = (event) => {
|
||||
if (this.isOutsideClicked(event)) {
|
||||
this.hide();
|
||||
}
|
||||
};
|
||||
|
||||
document.addEventListener('click', this.outsideClickListener);
|
||||
}
|
||||
},
|
||||
unbindOutsideClickListener() {
|
||||
if (this.outsideClickListener) {
|
||||
document.removeEventListener('click', this.outsideClickListener);
|
||||
this.outsideClickListener = null;
|
||||
}
|
||||
},
|
||||
isOutsideClicked(event) {
|
||||
return this.container && !this.container.contains(event.target);
|
||||
}
|
||||
},
|
||||
computed: {
|
||||
fullScreen() {
|
||||
return this.position === 'full';
|
||||
},
|
||||
closeAriaLabel() {
|
||||
return this.$primevue.config.locale.aria ? this.$primevue.config.locale.aria.close : undefined;
|
||||
}
|
||||
},
|
||||
directives: {
|
||||
focustrap: FocusTrap,
|
||||
ripple: Ripple
|
||||
},
|
||||
components: {
|
||||
Button,
|
||||
Portal,
|
||||
TimesIcon
|
||||
extends: Drawer,
|
||||
mounted() {
|
||||
console.warn('Deprecated since v4. Use Drawer component instead.');
|
||||
}
|
||||
};
|
||||
</script>
|
||||
|
|
|
@ -1,3 +1,3 @@
|
|||
import { BaseStyle } from '../../base/style';
|
||||
import { DrawerStyle } from '../../drawer/style/DrawerStyle';
|
||||
|
||||
export interface SidebarStyle extends BaseStyle {}
|
||||
export interface SidebarStyle extends DrawerStyle {}
|
||||
|
|
|
@ -1,48 +1,5 @@
|
|||
import BaseStyle from 'primevue/base/style';
|
||||
|
||||
const inlineStyles = {
|
||||
mask: ({ position }) => ({
|
||||
position: 'fixed',
|
||||
height: '100%',
|
||||
width: '100%',
|
||||
left: 0,
|
||||
top: 0,
|
||||
display: 'flex',
|
||||
justifyContent: position === 'left' ? 'flex-start' : position === 'right' ? 'flex-end' : 'center',
|
||||
alignItems: position === 'top' ? 'flex-start' : position === 'bottom' ? 'flex-end' : 'center'
|
||||
})
|
||||
};
|
||||
|
||||
const classes = {
|
||||
mask: ({ instance, props }) => {
|
||||
const positions = ['left', 'right', 'top', 'bottom'];
|
||||
const pos = positions.find((item) => item === props.position);
|
||||
|
||||
return [
|
||||
'p-drawer-mask',
|
||||
{
|
||||
'p-component-overlay p-component-overlay-enter': props.modal,
|
||||
'p-drawer-open': instance.containerVisible,
|
||||
'p-drawer-full': instance.fullScreen
|
||||
},
|
||||
pos ? `p-drawer-${pos}` : ''
|
||||
];
|
||||
},
|
||||
root: ({ instance }) => [
|
||||
'p-drawer p-component',
|
||||
{
|
||||
'p-ripple-disabled': instance.$primevue.config.ripple === false,
|
||||
'p-drawer-full': instance.fullScreen
|
||||
}
|
||||
],
|
||||
header: 'p-drawer-header',
|
||||
title: 'p-drawer-title',
|
||||
closeButton: 'p-drawer-close-button',
|
||||
content: 'p-drawer-content'
|
||||
};
|
||||
|
||||
export default BaseStyle.extend({
|
||||
name: 'sidebar',
|
||||
classes,
|
||||
inlineStyles
|
||||
name: 'sidebar'
|
||||
});
|
||||
|
|
|
@ -7,9 +7,9 @@ export default {
|
|||
transform: translate3d(0px, 0px, 0px);
|
||||
position: relative;
|
||||
transition: transform 0.3s;
|
||||
background: ${dt('sidebar.background')};
|
||||
color: ${dt('sidebar.color')};
|
||||
border: 1px solid ${dt('sidebar.border.color')};
|
||||
background: ${dt('drawer.background')};
|
||||
color: ${dt('drawer.color')};
|
||||
border: 1px solid ${dt('drawer.border.color')};
|
||||
box-shadow: 0 20px 25px -5px rgba(0, 0, 0, 0.1), 0 8px 10px -6px rgba(0, 0, 0, 0.1);
|
||||
}
|
||||
|
Loading…
Add table
Add a link
Reference in a new issue