2023-03-01 11:45:54 +00:00
|
|
|
/**
|
|
|
|
*
|
|
|
|
* Paginator is a generic component to display content in paged format.
|
|
|
|
*
|
2023-03-02 09:22:22 +00:00
|
|
|
* - [Paginator](https://primevue.org/paginator)
|
2023-03-01 11:45:54 +00:00
|
|
|
*
|
|
|
|
* @module paginator
|
|
|
|
*
|
|
|
|
*/
|
2022-09-06 12:03:37 +00:00
|
|
|
import { VNode } from 'vue';
|
|
|
|
import { ClassComponent, GlobalComponentConstructor } from '../ts-helpers';
|
|
|
|
|
2023-03-01 11:45:54 +00:00
|
|
|
/**
|
|
|
|
* Paginator page state metadata.
|
|
|
|
*/
|
2022-09-06 12:03:37 +00:00
|
|
|
export interface PageState {
|
|
|
|
/**
|
|
|
|
* Index of first record
|
|
|
|
*/
|
|
|
|
first: number;
|
|
|
|
/**
|
|
|
|
* Number of rows to display in new page
|
|
|
|
*/
|
|
|
|
rows: number;
|
|
|
|
/**
|
|
|
|
* New page number
|
|
|
|
*/
|
|
|
|
page: number;
|
|
|
|
/**
|
|
|
|
* Total number of pages
|
|
|
|
*/
|
|
|
|
pageCount?: number;
|
|
|
|
}
|
|
|
|
|
2023-03-01 11:45:54 +00:00
|
|
|
/**
|
|
|
|
* Defines valid properties in Paginator component.
|
|
|
|
*/
|
2022-09-06 12:03:37 +00:00
|
|
|
export interface PaginatorProps {
|
|
|
|
/**
|
|
|
|
* Number of total records.
|
2023-03-01 11:45:54 +00:00
|
|
|
* @defaultValue 0
|
2022-09-06 12:03:37 +00:00
|
|
|
*/
|
|
|
|
totalRecords?: number | undefined;
|
|
|
|
/**
|
|
|
|
* Data count to display per page.
|
2023-03-01 11:45:54 +00:00
|
|
|
* @defaultValue 0
|
2022-09-06 12:03:37 +00:00
|
|
|
*/
|
|
|
|
rows?: number | undefined;
|
|
|
|
/**
|
|
|
|
* Zero-relative number of the first row to be displayed.
|
2023-03-01 11:45:54 +00:00
|
|
|
* @defaultValue 0
|
2022-09-06 12:03:37 +00:00
|
|
|
*/
|
|
|
|
first?: number | undefined;
|
|
|
|
/**
|
|
|
|
* Number of page links to display.
|
2023-03-01 11:45:54 +00:00
|
|
|
* @defaultValue 5
|
2022-09-06 12:03:37 +00:00
|
|
|
*/
|
|
|
|
pageLinkSize?: number | undefined;
|
|
|
|
/**
|
|
|
|
* Array of integer values to display inside rows per page dropdown.
|
|
|
|
*/
|
|
|
|
rowsPerPageOptions?: number[] | undefined;
|
|
|
|
/**
|
2022-12-08 11:04:25 +00:00
|
|
|
* Template of the paginator, can either be a string or an object with key-value pairs to define templates per breakpoint.
|
2022-09-06 12:03:37 +00:00
|
|
|
*
|
|
|
|
* - FirstPageLink
|
|
|
|
* - PrevPageLink
|
|
|
|
* - PageLinks
|
|
|
|
* - NextPageLink
|
|
|
|
* - LastPageLink
|
|
|
|
* - RowsPerPageDropdown
|
|
|
|
* - JumpToPageDropdown
|
|
|
|
* - JumpToPageInput
|
|
|
|
* - CurrentPageReport
|
|
|
|
*/
|
2022-12-08 11:04:25 +00:00
|
|
|
template?: any | string;
|
2022-09-06 12:03:37 +00:00
|
|
|
/**
|
2023-03-01 11:45:54 +00:00
|
|
|
* Template of the current page report element. It displays information about the pagination state. Available placeholders are the following;
|
2022-09-06 12:03:37 +00:00
|
|
|
*
|
|
|
|
* - {currentPage}
|
|
|
|
* - {totalPages}
|
|
|
|
* - {rows}
|
|
|
|
* - {first}
|
|
|
|
* - {last}
|
|
|
|
* - {totalRecords}
|
2023-03-01 11:45:54 +00:00
|
|
|
*
|
2023-03-03 08:18:55 +00:00
|
|
|
* @defaultValue '({currentPage} of {totalPages})'
|
2022-09-06 12:03:37 +00:00
|
|
|
*/
|
|
|
|
currentPageReportTemplate?: string | undefined;
|
|
|
|
/**
|
|
|
|
* Whether to show the paginator even there is only one page.
|
2023-03-01 11:45:54 +00:00
|
|
|
* @defaultValue true
|
2022-09-06 12:03:37 +00:00
|
|
|
*/
|
|
|
|
alwaysShow?: boolean | undefined;
|
|
|
|
}
|
|
|
|
|
2023-03-01 11:45:54 +00:00
|
|
|
/**
|
|
|
|
* Defines valid slots in Paginator component.
|
|
|
|
*/
|
2022-09-06 12:03:37 +00:00
|
|
|
export interface PaginatorSlots {
|
|
|
|
/**
|
|
|
|
* Custom start template.
|
|
|
|
* @param {Object} scope - start slot's params.
|
|
|
|
*/
|
2023-03-01 11:45:54 +00:00
|
|
|
start(scope: {
|
2022-09-06 12:03:37 +00:00
|
|
|
/**
|
|
|
|
* Current state
|
|
|
|
* @see PageState
|
|
|
|
*/
|
|
|
|
state: PageState;
|
2023-03-01 11:45:54 +00:00
|
|
|
}): VNode[];
|
2022-09-06 12:03:37 +00:00
|
|
|
/**
|
|
|
|
* Custom end template.
|
|
|
|
* @param {Object} scope - end slot's params.
|
|
|
|
*/
|
2023-03-01 11:45:54 +00:00
|
|
|
end(scope: {
|
2022-09-06 12:03:37 +00:00
|
|
|
/**
|
|
|
|
* Current state
|
|
|
|
* @see PageState
|
|
|
|
*/
|
|
|
|
state: PageState;
|
2023-03-01 11:45:54 +00:00
|
|
|
}): VNode[];
|
2022-09-06 12:03:37 +00:00
|
|
|
}
|
|
|
|
|
2023-03-01 11:45:54 +00:00
|
|
|
/**
|
|
|
|
* Defines valid emits in Paginator component.
|
|
|
|
*/
|
|
|
|
export interface PaginatorEmits {
|
2022-09-06 12:03:37 +00:00
|
|
|
/**
|
|
|
|
* Emitted when the first changes.
|
|
|
|
* @param {number} value - New value.
|
|
|
|
*/
|
2023-03-01 11:45:54 +00:00
|
|
|
'update:first'(value: number): void;
|
2022-09-06 12:03:37 +00:00
|
|
|
/**
|
|
|
|
* Emitted when the rows changes.
|
|
|
|
* @param {number} value - New value.
|
|
|
|
*/
|
2023-03-01 11:45:54 +00:00
|
|
|
'update:rows'(value: number): void;
|
2022-09-06 12:03:37 +00:00
|
|
|
/**
|
|
|
|
* Callback to invoke when page changes, the event object contains information about the new state.
|
|
|
|
* @param {PageState} event - New page state.
|
|
|
|
*/
|
2023-03-01 11:45:54 +00:00
|
|
|
page(event: PageState): void;
|
|
|
|
}
|
2022-09-06 12:03:37 +00:00
|
|
|
|
2023-03-01 11:45:54 +00:00
|
|
|
/**
|
|
|
|
* **PrimeVue - Paginator**
|
|
|
|
*
|
|
|
|
* _Paginator is a generic widget to display content in paged format._
|
|
|
|
*
|
|
|
|
* [Live Demo](https://www.primevue.org/paginator/)
|
|
|
|
* --- ---
|
|
|
|
* 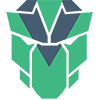
|
|
|
|
*
|
|
|
|
* @group Component
|
|
|
|
*/
|
2022-09-14 11:26:01 +00:00
|
|
|
declare class Paginator extends ClassComponent<PaginatorProps, PaginatorSlots, PaginatorEmits> {}
|
2022-09-06 12:03:37 +00:00
|
|
|
|
|
|
|
declare module '@vue/runtime-core' {
|
|
|
|
interface GlobalComponents {
|
2022-09-14 11:26:01 +00:00
|
|
|
Paginator: GlobalComponentConstructor<Paginator>;
|
2022-09-06 12:03:37 +00:00
|
|
|
}
|
|
|
|
}
|
|
|
|
|
|
|
|
export default Paginator;
|