2023-03-02 11:03:01 +00:00
|
|
|
/**
|
|
|
|
*
|
|
|
|
* Tooltip directive provides advisory information for a component.
|
|
|
|
*
|
2023-03-03 14:17:03 +00:00
|
|
|
* [Live Demo](https://primevue.org/tooltip)
|
2023-03-02 14:25:05 +00:00
|
|
|
*
|
|
|
|
* @module tooltip
|
|
|
|
*
|
2023-03-02 11:03:01 +00:00
|
|
|
*/
|
2023-03-02 09:31:36 +00:00
|
|
|
import { DirectiveBinding, ObjectDirective } from 'vue';
|
2022-09-06 12:03:37 +00:00
|
|
|
|
2023-06-21 13:58:32 +00:00
|
|
|
/**
|
2023-06-23 09:47:31 +00:00
|
|
|
* Defines options of Tooltip.
|
2023-06-21 13:58:32 +00:00
|
|
|
*/
|
2023-06-23 09:47:31 +00:00
|
|
|
export interface TooltipOptions {
|
2023-06-21 13:58:32 +00:00
|
|
|
/**
|
2023-06-23 09:47:31 +00:00
|
|
|
* Text of the tooltip.
|
2023-06-21 13:58:32 +00:00
|
|
|
*/
|
2023-06-23 09:47:31 +00:00
|
|
|
value?: string | undefined;
|
2023-06-21 13:58:32 +00:00
|
|
|
/**
|
2023-06-23 09:47:31 +00:00
|
|
|
* When present, it specifies that the component should be disabled.
|
|
|
|
* @defaultValue false
|
2023-06-21 13:58:32 +00:00
|
|
|
*/
|
2023-06-23 09:47:31 +00:00
|
|
|
disabled?: boolean | undefined;
|
2023-06-21 13:58:32 +00:00
|
|
|
/**
|
2023-06-23 09:47:31 +00:00
|
|
|
* When present, it adds a custom id to the tooltip.
|
2023-06-21 13:58:32 +00:00
|
|
|
*/
|
2023-06-23 09:47:31 +00:00
|
|
|
id?: string | undefined;
|
2023-06-21 13:58:32 +00:00
|
|
|
/**
|
2023-06-23 09:47:31 +00:00
|
|
|
* When present, it adds a custom class to the tooltip.
|
2023-06-21 13:58:32 +00:00
|
|
|
*/
|
2023-06-23 09:47:31 +00:00
|
|
|
class?: string | undefined;
|
2023-06-21 13:58:32 +00:00
|
|
|
/**
|
2023-06-23 09:47:31 +00:00
|
|
|
* By default the tooltip contents are not rendered as text. Set to true to support html tags in the content.
|
|
|
|
* @defaultValue false
|
2023-06-21 13:58:32 +00:00
|
|
|
*/
|
2023-06-23 09:47:31 +00:00
|
|
|
escape?: boolean | undefined;
|
2023-06-21 13:58:32 +00:00
|
|
|
/**
|
2023-06-23 09:47:31 +00:00
|
|
|
* Automatically adjusts the element position when there is not enough space on the selected position.
|
|
|
|
* @defaultValue true
|
2023-06-21 13:58:32 +00:00
|
|
|
*/
|
2023-06-23 09:47:31 +00:00
|
|
|
fitContent?: boolean | undefined;
|
2023-06-21 13:58:32 +00:00
|
|
|
/**
|
2023-06-23 09:47:31 +00:00
|
|
|
* When present, it adds a custom delay to the tooltip's display.
|
|
|
|
* @defaultValue 0
|
2023-06-21 13:58:32 +00:00
|
|
|
*/
|
2023-06-23 09:47:31 +00:00
|
|
|
showDelay?: number | undefined;
|
|
|
|
/**
|
|
|
|
* When present, it adds a custom delay to the tooltip's hiding.
|
|
|
|
* @defaultValue 0
|
|
|
|
*/
|
|
|
|
hideDelay?: number | undefined;
|
|
|
|
/**
|
|
|
|
* Uses to pass attributes to DOM elements inside the component.
|
|
|
|
* @type {TooltipPassThroughOptions}
|
|
|
|
*/
|
|
|
|
pt?: TooltipPassThroughOptions;
|
2023-06-21 13:58:32 +00:00
|
|
|
}
|
|
|
|
|
|
|
|
/**
|
2023-06-23 09:47:31 +00:00
|
|
|
* Defines modifiers of Tooltip.
|
2023-06-21 13:58:32 +00:00
|
|
|
*/
|
2023-06-23 09:47:31 +00:00
|
|
|
export interface TooltipDirectiveModifiers {
|
2023-06-21 13:58:32 +00:00
|
|
|
/**
|
2023-06-23 09:47:31 +00:00
|
|
|
* Right position for Tooltip.
|
|
|
|
* @defaultValue true
|
2023-06-21 13:58:32 +00:00
|
|
|
*/
|
2023-06-23 09:47:31 +00:00
|
|
|
right?: boolean | undefined;
|
2023-06-21 13:58:32 +00:00
|
|
|
/**
|
2023-06-23 09:47:31 +00:00
|
|
|
* Left position for Tooltip.
|
|
|
|
* @defaultValue false
|
2023-06-21 13:58:32 +00:00
|
|
|
*/
|
2023-06-23 09:47:31 +00:00
|
|
|
left?: boolean | undefined;
|
2023-06-21 13:58:32 +00:00
|
|
|
/**
|
2023-06-23 09:47:31 +00:00
|
|
|
* Top position for Tooltip.
|
|
|
|
* @defaultValue false
|
2023-06-21 13:58:32 +00:00
|
|
|
*/
|
2023-06-23 09:47:31 +00:00
|
|
|
top?: boolean | undefined;
|
2023-06-21 13:58:32 +00:00
|
|
|
/**
|
2023-06-23 09:47:31 +00:00
|
|
|
* Bottom position for Tooltip.
|
|
|
|
* @defaultValue false
|
2023-06-21 13:58:32 +00:00
|
|
|
*/
|
2023-06-23 09:47:31 +00:00
|
|
|
bottom?: boolean | undefined;
|
|
|
|
/**
|
|
|
|
* Focus event for Tooltip.
|
|
|
|
* @defaultValue true
|
|
|
|
*/
|
|
|
|
focus?: boolean | undefined;
|
2023-06-21 13:58:32 +00:00
|
|
|
}
|
|
|
|
|
|
|
|
/**
|
|
|
|
* Custom passthrough(pt) options.
|
|
|
|
* @see {@link TooltipOptions.pt}
|
|
|
|
*/
|
|
|
|
export interface TooltipPassThroughOptions {
|
|
|
|
/**
|
|
|
|
* Uses to pass attributes to the root's DOM element.
|
|
|
|
* @see {@link TooltipPassThroughDirectiveOptions}
|
|
|
|
*/
|
|
|
|
root?: TooltipPassThroughDirectiveOptions;
|
|
|
|
/**
|
|
|
|
* Uses to pass attributes to the text's DOM element.
|
|
|
|
* @see {@link TooltipPassThroughDirectiveOptions}
|
|
|
|
*/
|
|
|
|
text?: TooltipPassThroughDirectiveOptions;
|
|
|
|
/**
|
|
|
|
* Uses to pass attributes to the arrow's DOM element.
|
|
|
|
* @see {@link TooltipPassThroughDirectiveOptions}
|
|
|
|
*/
|
|
|
|
arrow?: TooltipPassThroughDirectiveOptions;
|
|
|
|
}
|
|
|
|
|
2023-03-02 10:54:16 +00:00
|
|
|
/**
|
2023-06-23 09:47:31 +00:00
|
|
|
* Custom passthrough(pt) directive options.
|
2023-03-02 10:54:16 +00:00
|
|
|
*/
|
2023-06-23 09:47:31 +00:00
|
|
|
export interface TooltipPassThroughDirectiveOptions {
|
2023-03-02 09:53:55 +00:00
|
|
|
/**
|
2023-06-23 09:47:31 +00:00
|
|
|
* Uses to pass attributes to the life cycle hooks.
|
|
|
|
* @see {@link TooltipPassThroughHooksOptions}
|
2023-03-02 09:53:55 +00:00
|
|
|
*/
|
2023-06-23 09:47:31 +00:00
|
|
|
hooks?: TooltipPassThroughHooksOptions;
|
2023-03-01 14:57:18 +00:00
|
|
|
/**
|
2023-06-23 09:47:31 +00:00
|
|
|
* Uses to pass attributes to the styles.
|
|
|
|
* @see {@link TooltipPassThroughCSSOptions}
|
2023-03-01 14:57:18 +00:00
|
|
|
*/
|
2023-06-23 09:47:31 +00:00
|
|
|
css?: TooltipPassThroughCSSOptions;
|
|
|
|
}
|
|
|
|
|
|
|
|
/**
|
|
|
|
* Custom passthrough(pt) hooks options.
|
|
|
|
*/
|
|
|
|
export interface TooltipPassThroughHooksOptions {
|
2023-03-01 14:57:18 +00:00
|
|
|
/**
|
2023-06-23 09:47:31 +00:00
|
|
|
* Called before bound element's attributes or event listeners are applied.
|
2023-03-01 14:57:18 +00:00
|
|
|
*/
|
2023-06-23 09:47:31 +00:00
|
|
|
created?: DirectiveBinding;
|
2023-03-01 14:57:18 +00:00
|
|
|
/**
|
2023-06-23 09:47:31 +00:00
|
|
|
* Called right before the element is inserted into the DOM.
|
2023-03-01 14:57:18 +00:00
|
|
|
*/
|
2023-06-23 09:47:31 +00:00
|
|
|
beforeMount?: DirectiveBinding;
|
2023-03-01 14:57:18 +00:00
|
|
|
/**
|
2023-06-23 09:47:31 +00:00
|
|
|
* Called when the bound element's parent component and all its children are mounted.
|
2023-03-01 14:57:18 +00:00
|
|
|
*/
|
2023-06-23 09:47:31 +00:00
|
|
|
mounted?: DirectiveBinding;
|
2023-03-01 14:57:18 +00:00
|
|
|
/**
|
2023-06-23 09:47:31 +00:00
|
|
|
* Called before the parent component is updated.
|
2023-03-01 14:57:18 +00:00
|
|
|
*/
|
2023-06-23 09:47:31 +00:00
|
|
|
beforeUpdate?: DirectiveBinding;
|
2023-04-09 15:11:41 +00:00
|
|
|
/**
|
2023-06-23 09:47:31 +00:00
|
|
|
* Called after the parent component and all of its children have updated all of its children have updated.
|
2023-04-09 15:11:41 +00:00
|
|
|
*/
|
2023-06-23 09:47:31 +00:00
|
|
|
updated?: DirectiveBinding;
|
2023-04-09 15:11:41 +00:00
|
|
|
/**
|
2023-06-23 09:47:31 +00:00
|
|
|
* Called before the parent component is unmounted.
|
2023-04-09 15:11:41 +00:00
|
|
|
*/
|
2023-06-23 09:47:31 +00:00
|
|
|
beforeUnmount?: DirectiveBinding;
|
2023-06-21 13:58:32 +00:00
|
|
|
/**
|
2023-06-23 09:47:31 +00:00
|
|
|
* Called when the parent component is unmounted.
|
2023-06-21 13:58:32 +00:00
|
|
|
*/
|
2023-06-23 09:47:31 +00:00
|
|
|
unmounted?: DirectiveBinding;
|
2023-03-02 09:53:55 +00:00
|
|
|
}
|
|
|
|
|
2023-03-02 10:54:16 +00:00
|
|
|
/**
|
2023-06-23 09:47:31 +00:00
|
|
|
* Custom passthrough(pt) css options.
|
2023-03-02 10:54:16 +00:00
|
|
|
*/
|
2023-06-23 09:47:31 +00:00
|
|
|
export interface TooltipPassThroughCSSOptions {
|
2023-03-02 10:54:16 +00:00
|
|
|
/**
|
2023-06-23 09:47:31 +00:00
|
|
|
* Style class of the element.
|
2023-03-02 10:54:16 +00:00
|
|
|
*/
|
2023-06-23 09:47:31 +00:00
|
|
|
class?: any;
|
2023-03-02 10:54:16 +00:00
|
|
|
/**
|
2023-06-23 09:47:31 +00:00
|
|
|
* Inline style of the element.
|
2023-03-02 10:54:16 +00:00
|
|
|
*/
|
2023-06-23 09:47:31 +00:00
|
|
|
style?: any;
|
2023-03-02 14:25:05 +00:00
|
|
|
}
|
2023-03-02 09:31:36 +00:00
|
|
|
|
2023-03-02 10:54:16 +00:00
|
|
|
/**
|
|
|
|
* Binding of Tooltip directive.
|
|
|
|
*/
|
2023-03-02 09:31:36 +00:00
|
|
|
export interface TooltipDirectiveBinding extends Omit<DirectiveBinding, 'modifiers' | 'value'> {
|
|
|
|
/**
|
2023-03-02 09:53:55 +00:00
|
|
|
* Value of the tooltip.
|
2023-03-02 09:31:36 +00:00
|
|
|
*/
|
2023-03-02 09:53:55 +00:00
|
|
|
value?: string | TooltipOptions | undefined;
|
2023-03-02 09:31:36 +00:00
|
|
|
/**
|
2023-03-02 09:53:55 +00:00
|
|
|
* Modifiers of the tooltip.
|
2023-03-02 09:31:36 +00:00
|
|
|
* @type {TooltipDirectiveModifiers}
|
|
|
|
*/
|
|
|
|
modifiers?: TooltipDirectiveModifiers | undefined;
|
2023-03-01 14:57:18 +00:00
|
|
|
}
|
|
|
|
|
2023-03-02 10:54:16 +00:00
|
|
|
/**
|
|
|
|
* **PrimeVue - Tooltip**
|
|
|
|
*
|
|
|
|
* _Tooltip directive provides advisory information for a component._
|
|
|
|
*
|
|
|
|
* [Live Demo](https://www.primevue.org/tooltip/)
|
|
|
|
* --- ---
|
2023-03-03 10:55:20 +00:00
|
|
|
* 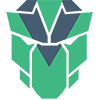
|
2023-03-02 10:54:16 +00:00
|
|
|
*
|
|
|
|
*/
|
2023-03-02 09:31:36 +00:00
|
|
|
declare const Tooltip: ObjectDirective;
|
|
|
|
|
2022-09-06 12:03:37 +00:00
|
|
|
export default Tooltip;
|