parent
ff0df14ed7
commit
033a50fcbb
|
@ -0,0 +1,34 @@
|
|||
<script>
|
||||
import BaseComponent from 'primevue/basecomponent';
|
||||
import StepStyle from 'primevue/step/style';
|
||||
|
||||
export default {
|
||||
name: 'BaseStep',
|
||||
extends: BaseComponent,
|
||||
props: {
|
||||
value: {
|
||||
type: [String, Number],
|
||||
default: undefined
|
||||
},
|
||||
disabled: {
|
||||
type: Boolean,
|
||||
default: false
|
||||
},
|
||||
asChild: {
|
||||
type: Boolean,
|
||||
default: false
|
||||
},
|
||||
as: {
|
||||
type: String,
|
||||
default: 'DIV'
|
||||
}
|
||||
},
|
||||
style: StepStyle,
|
||||
provide() {
|
||||
return {
|
||||
$pcStep: this,
|
||||
$parentInstance: this
|
||||
};
|
||||
}
|
||||
};
|
||||
</script>
|
|
@ -0,0 +1,187 @@
|
|||
/**
|
||||
*
|
||||
* Step is a helper component for Stepper component.
|
||||
*
|
||||
* [Live Demo](https://www.primevue.org/stepper/)
|
||||
*
|
||||
* @module step
|
||||
*
|
||||
*/
|
||||
import { VNode } from 'vue';
|
||||
import { ComponentHooks } from '../basecomponent';
|
||||
import { PassThroughOptions } from '../passthrough';
|
||||
import { DefineComponent, DesignToken, EmitFn, GlobalComponentConstructor, PassThrough } from '../ts-helpers';
|
||||
|
||||
export declare type StepPassThroughOptionType = StepPassThroughAttributes | ((options: StepPassThroughMethodOptions) => StepPassThroughAttributes | string) | string | null | undefined;
|
||||
|
||||
/**
|
||||
* Custom passthrough(pt) option method.
|
||||
*/
|
||||
export interface StepPassThroughMethodOptions {
|
||||
/**
|
||||
* Defines instance.
|
||||
*/
|
||||
instance: any;
|
||||
/**
|
||||
* Defines valid properties.
|
||||
*/
|
||||
props: StepProps;
|
||||
/**
|
||||
* Defines current options.
|
||||
*/
|
||||
context: StepContext;
|
||||
/**
|
||||
* Defines valid attributes.
|
||||
*/
|
||||
attrs: any;
|
||||
/**
|
||||
* Defines parent options.
|
||||
*/
|
||||
parent: any;
|
||||
/**
|
||||
* Defines passthrough(pt) options in global config.
|
||||
*/
|
||||
global: object | undefined;
|
||||
}
|
||||
|
||||
/**
|
||||
* Custom passthrough(pt) options.
|
||||
* @see {@link StepProps.pt}
|
||||
*/
|
||||
export interface StepPassThroughOptions {
|
||||
/**
|
||||
* Used to pass attributes to the root's DOM element.
|
||||
*/
|
||||
root?: StepPassThroughOptionType;
|
||||
/**
|
||||
* Used to pass attributes to the header's DOM element.
|
||||
*/
|
||||
header?: StepPassThroughOptionType;
|
||||
/**
|
||||
* Used to pass attributes to the number's DOM element.
|
||||
*/
|
||||
number?: StepPassThroughOptionType;
|
||||
/**
|
||||
* Used to pass attributes to the title's DOM element.
|
||||
*/
|
||||
title?: StepPassThroughOptionType;
|
||||
/**
|
||||
* Used to manage all lifecycle hooks.
|
||||
* @see {@link BaseComponent.ComponentHooks}
|
||||
*/
|
||||
hooks?: ComponentHooks;
|
||||
}
|
||||
|
||||
export interface StepPassThroughAttributes {
|
||||
[key: string]: any;
|
||||
}
|
||||
|
||||
/**
|
||||
* Defines valid properties in Step component.
|
||||
*/
|
||||
export interface StepProps {
|
||||
/**
|
||||
* Value of step.
|
||||
*/
|
||||
value?: string | number | undefined;
|
||||
/**
|
||||
* Whether the step is disabled.
|
||||
* @defaultValue false
|
||||
*/
|
||||
disabled?: boolean | undefined;
|
||||
/**
|
||||
* Use to change the HTML tag of root element.
|
||||
* @defaultValue BUTTON
|
||||
*/
|
||||
as?: string | undefined;
|
||||
/**
|
||||
* When enabled, it changes the default rendered element for the one passed as a child element.
|
||||
* @defaultValue false
|
||||
*/
|
||||
asChild?: boolean | undefined;
|
||||
/**
|
||||
* It generates scoped CSS variables using design tokens for the component.
|
||||
*/
|
||||
dt?: DesignToken<any>;
|
||||
/**
|
||||
* Used to pass attributes to DOM elements inside the component.
|
||||
* @type {StepPassThroughOptions}
|
||||
*/
|
||||
pt?: PassThrough<StepPassThroughOptions>;
|
||||
/**
|
||||
* Used to configure passthrough(pt) options of the component.
|
||||
* @type {PassThroughOptions}
|
||||
*/
|
||||
ptOptions?: PassThroughOptions;
|
||||
}
|
||||
|
||||
/**
|
||||
* Defines current options in Step component.
|
||||
*/
|
||||
export interface StepContext {
|
||||
/**
|
||||
* Whether the step is active.
|
||||
*/
|
||||
active: boolean;
|
||||
/**
|
||||
* Whether the step is disabled.
|
||||
*/
|
||||
disabled: boolean;
|
||||
}
|
||||
|
||||
/**
|
||||
* Defines valid slots in Step slots.
|
||||
*/
|
||||
export interface StepSlots {
|
||||
/**
|
||||
* Custom content template. Slot attributes can be used when asChild prop is true.
|
||||
*/
|
||||
default(scope: {
|
||||
/**
|
||||
* Style class of the loader.
|
||||
*/
|
||||
class: string;
|
||||
/**
|
||||
* Whether the step is active.
|
||||
*/
|
||||
active: boolean;
|
||||
/**
|
||||
* Value of step.
|
||||
*/
|
||||
value: string | number;
|
||||
/**
|
||||
* A11t attributes
|
||||
*/
|
||||
a11yAttrs: any;
|
||||
/**
|
||||
* Click function.
|
||||
*/
|
||||
activateCallback: () => void;
|
||||
}): VNode[];
|
||||
}
|
||||
|
||||
export interface StepEmitsOptions {}
|
||||
|
||||
export declare type StepEmits = EmitFn<StepEmitsOptions>;
|
||||
|
||||
/**
|
||||
* **PrimeVue - Step**
|
||||
*
|
||||
* _Step is a helper component for Stepper component._
|
||||
*
|
||||
* [Live Demo](https://www.primevue.org/stepper/)
|
||||
* --- ---
|
||||
* 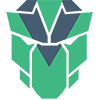
|
||||
*
|
||||
* @group Component
|
||||
*
|
||||
*/
|
||||
declare const Step: DefineComponent<StepProps, StepSlots, StepEmits>;
|
||||
|
||||
declare module 'vue' {
|
||||
export interface GlobalComponents {
|
||||
Step: GlobalComponentConstructor<StepProps, StepSlots, StepEmits>;
|
||||
}
|
||||
}
|
||||
|
||||
export default Step;
|
|
@ -0,0 +1,98 @@
|
|||
<template>
|
||||
<component v-if="!asChild" :is="as" :class="cx('root')" :aria-current="active ? 'step' : undefined" role="presentation" :data-p-active="active" :data-p-disabled="disabled" v-bind="getPTOptions('root')">
|
||||
<button :id="id" :class="cx('header')" role="tab" type="button" :tabindex="disabled ? -1 : undefined" :aria-controls="ariaControls" :disabled="disabled" @click="onStepClick" v-bind="getPTOptions('header')">
|
||||
<span :class="cx('number')" v-bind="getPTOptions('number')">{{ activeValue }}</span>
|
||||
<span :class="cx('title')" v-bind="getPTOptions('title')">
|
||||
<slot />
|
||||
</span>
|
||||
</button>
|
||||
<StepperSeparator v-if="isSeparatorVisible" />
|
||||
</component>
|
||||
<slot v-else :class="cx('root')" :active="active" :value="value" :a11yAttrs="a11yAttrs" :activateCallback="onStepClick" />
|
||||
</template>
|
||||
|
||||
<script>
|
||||
import { DomHandler, ObjectUtils } from 'primevue/utils';
|
||||
import StepperSeparator from '../stepper/StepperSeparator.vue';
|
||||
import BaseStep from './BaseStep.vue';
|
||||
|
||||
export default {
|
||||
name: 'Step',
|
||||
extends: BaseStep,
|
||||
inheritAttrs: false,
|
||||
inject: {
|
||||
$pcStepper: { default: null },
|
||||
$pcStepList: { default: null },
|
||||
$pcStepItem: { default: null }
|
||||
},
|
||||
data() {
|
||||
return {
|
||||
isSeparatorVisible: false
|
||||
};
|
||||
},
|
||||
mounted() {
|
||||
if (this.$el && this.$pcStepList) {
|
||||
let index = ObjectUtils.findIndexInList(this.$el, DomHandler.find(this.$pcStepper.$el, '[data-pc-name="step"]'));
|
||||
let stepLen = DomHandler.find(this.$pcStepper.$el, '[data-pc-name="step"]').length;
|
||||
|
||||
this.isSeparatorVisible = index !== stepLen - 1;
|
||||
}
|
||||
},
|
||||
methods: {
|
||||
getPTOptions(key) {
|
||||
const _ptm = key === 'root' ? this.ptmi : this.ptm;
|
||||
|
||||
return _ptm(key, {
|
||||
context: {
|
||||
active: this.active,
|
||||
disabled: this.disabled
|
||||
}
|
||||
});
|
||||
},
|
||||
isStepDisabled() {
|
||||
return this.$pcStepper.isStepDisabled();
|
||||
},
|
||||
onStepClick() {
|
||||
this.$pcStepper.updateValue(this.activeValue);
|
||||
}
|
||||
},
|
||||
computed: {
|
||||
active() {
|
||||
return this.$pcStepper.isStepActive(this.activeValue);
|
||||
},
|
||||
activeValue() {
|
||||
return !!this.$pcStepItem ? this.$pcStepItem?.value : this.value;
|
||||
},
|
||||
id() {
|
||||
return `${this.$pcStepper?.id}_step_${this.activeValue}`;
|
||||
},
|
||||
ariaControls() {
|
||||
return `${this.$pcStepper?.id}_steppanel_${this.activeValue}`;
|
||||
},
|
||||
a11yAttrs() {
|
||||
return {
|
||||
root: {
|
||||
role: 'presentation',
|
||||
'aria-current': this.active ? 'step' : undefined,
|
||||
'data-pc-name': 'step',
|
||||
'data-pc-section': 'root',
|
||||
'data-p-disabled': this.disabled,
|
||||
'data-p-active': this.active
|
||||
},
|
||||
header: {
|
||||
id: this.id,
|
||||
role: 'tab',
|
||||
taindex: this.disabled ? -1 : undefined,
|
||||
'aria-controls': this.ariaControls,
|
||||
'data-pc-section': 'header',
|
||||
disabled: this.disabled,
|
||||
onClick: this.onStepClick
|
||||
}
|
||||
};
|
||||
}
|
||||
},
|
||||
components: {
|
||||
StepperSeparator
|
||||
}
|
||||
};
|
||||
</script>
|
|
@ -0,0 +1,11 @@
|
|||
{
|
||||
"main": "./step.mjs",
|
||||
"module": "./step.mjs",
|
||||
"types": "./Step.d.ts",
|
||||
"browser": {
|
||||
"./sfc": "./Step.vue"
|
||||
},
|
||||
"sideEffects": [
|
||||
"*.vue"
|
||||
]
|
||||
}
|
|
@ -0,0 +1,31 @@
|
|||
/**
|
||||
*
|
||||
* Step is a helper component for Stepper component.
|
||||
*
|
||||
* [Live Demo](https://www.primevue.org/stepper/)
|
||||
*
|
||||
* @module stepstyle
|
||||
*
|
||||
*/
|
||||
import { BaseStyle } from '../../base/style';
|
||||
|
||||
export enum StepClasses {
|
||||
/**
|
||||
* Class name of the root element
|
||||
*/
|
||||
root = 'p-step',
|
||||
/**
|
||||
* Class name of the header element
|
||||
*/
|
||||
header = 'p-step-header',
|
||||
/**
|
||||
* Class name of the number element
|
||||
*/
|
||||
number = 'p-step-number',
|
||||
/**
|
||||
* Class name of the title element
|
||||
*/
|
||||
title = 'p-step-title'
|
||||
}
|
||||
|
||||
export interface StepStyle extends BaseStyle {}
|
|
@ -0,0 +1,19 @@
|
|||
import BaseStyle from 'primevue/base/style';
|
||||
|
||||
const classes = {
|
||||
root: ({ instance, props }) => [
|
||||
'p-step',
|
||||
{
|
||||
'p-step-active': instance.active,
|
||||
'p-disabled': instance.isStepDisabled() || props.disabled
|
||||
}
|
||||
],
|
||||
header: 'p-step-header',
|
||||
number: 'p-step-number',
|
||||
title: 'p-step-title'
|
||||
};
|
||||
|
||||
export default BaseStyle.extend({
|
||||
name: 'step',
|
||||
classes
|
||||
});
|
|
@ -0,0 +1,6 @@
|
|||
{
|
||||
"main": "./stepstyle.mjs",
|
||||
"module": "./stepstyle.mjs",
|
||||
"types": "./StepStyle.d.ts",
|
||||
"sideEffects": false
|
||||
}
|
|
@ -0,0 +1,22 @@
|
|||
<script>
|
||||
import BaseComponent from 'primevue/basecomponent';
|
||||
import StepItemStyle from 'primevue/stepitem/style';
|
||||
|
||||
export default {
|
||||
name: 'BaseStepItem',
|
||||
extends: BaseComponent,
|
||||
props: {
|
||||
value: {
|
||||
type: String,
|
||||
default: undefined
|
||||
}
|
||||
},
|
||||
style: StepItemStyle,
|
||||
provide() {
|
||||
return {
|
||||
$pcStepItem: this,
|
||||
$parentInstance: this
|
||||
};
|
||||
}
|
||||
};
|
||||
</script>
|
|
@ -0,0 +1,121 @@
|
|||
/**
|
||||
*
|
||||
* StepItem is a helper component for Stepper component.
|
||||
*
|
||||
* [Live Demo](https://www.primevue.org/stepper/)
|
||||
*
|
||||
* @module stepitem
|
||||
*
|
||||
*/
|
||||
import { VNode } from 'vue';
|
||||
import { ComponentHooks } from '../basecomponent';
|
||||
import { PassThroughOptions } from '../passthrough';
|
||||
import { DefineComponent, DesignToken, EmitFn, GlobalComponentConstructor, PassThrough } from '../ts-helpers';
|
||||
|
||||
export declare type StepItemPassThroughOptionType = StepItemPassThroughAttributes | ((options: StepItemPassThroughMethodOptions) => StepItemPassThroughAttributes | string) | string | null | undefined;
|
||||
|
||||
/**
|
||||
* Custom passthrough(pt) option method.
|
||||
*/
|
||||
export interface StepItemPassThroughMethodOptions {
|
||||
/**
|
||||
* Defines instance.
|
||||
*/
|
||||
instance: any;
|
||||
/**
|
||||
* Defines valid properties.
|
||||
*/
|
||||
props: StepItemProps;
|
||||
/**
|
||||
* Defines valid attributes.
|
||||
*/
|
||||
attrs: any;
|
||||
/**
|
||||
* Defines parent options.
|
||||
*/
|
||||
parent: any;
|
||||
/**
|
||||
* Defines passthrough(pt) options in global config.
|
||||
*/
|
||||
global: object | undefined;
|
||||
}
|
||||
|
||||
/**
|
||||
* Custom passthrough(pt) options.
|
||||
* @see {@link StepItemProps.pt}
|
||||
*/
|
||||
export interface StepItemPassThroughOptions {
|
||||
/**
|
||||
* Used to pass attributes to the root's DOM element.
|
||||
*/
|
||||
root?: StepItemPassThroughOptionType;
|
||||
/**
|
||||
* Used to manage all lifecycle hooks.
|
||||
* @see {@link BaseComponent.ComponentHooks}
|
||||
*/
|
||||
hooks?: ComponentHooks;
|
||||
}
|
||||
|
||||
export interface StepItemPassThroughAttributes {
|
||||
[key: string]: any;
|
||||
}
|
||||
|
||||
/**
|
||||
* Defines valid properties in StepItem component.
|
||||
*/
|
||||
export interface StepItemProps {
|
||||
/**
|
||||
* Value of step.
|
||||
*/
|
||||
value?: string | number | undefined;
|
||||
/**
|
||||
* It generates scoped CSS variables using design tokens for the component.
|
||||
*/
|
||||
dt?: DesignToken<any>;
|
||||
/**
|
||||
* Used to pass attributes to DOM elements inside the component.
|
||||
* @type {StepItemPassThroughOptions}
|
||||
*/
|
||||
pt?: PassThrough<StepItemPassThroughOptions>;
|
||||
/**
|
||||
* Used to configure passthrough(pt) options of the component.
|
||||
* @type {PassThroughOptions}
|
||||
*/
|
||||
ptOptions?: PassThroughOptions;
|
||||
}
|
||||
|
||||
/**
|
||||
* Defines valid slots in StepItem slots.
|
||||
*/
|
||||
export interface StepItemSlots {
|
||||
/**
|
||||
* Custom content template.
|
||||
*/
|
||||
default(): VNode[];
|
||||
}
|
||||
|
||||
export interface StepItemEmitsOptions {}
|
||||
|
||||
export declare type StepItemEmits = EmitFn<StepItemEmitsOptions>;
|
||||
|
||||
/**
|
||||
* **PrimeVue - StepItem**
|
||||
*
|
||||
* _StepItem is a helper component for Stepper component._
|
||||
*
|
||||
* [Live Demo](https://www.primevue.org/stepper/)
|
||||
* --- ---
|
||||
* 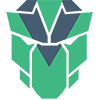
|
||||
*
|
||||
* @group Component
|
||||
*
|
||||
*/
|
||||
declare const StepItem: DefineComponent<StepItemProps, StepItemSlots, StepItemEmits>;
|
||||
|
||||
declare module 'vue' {
|
||||
export interface GlobalComponents {
|
||||
StepItem: GlobalComponentConstructor<StepItemProps, StepItemSlots, StepItemEmits>;
|
||||
}
|
||||
}
|
||||
|
||||
export default StepItem;
|
|
@ -0,0 +1,21 @@
|
|||
<template>
|
||||
<div :class="cx('root')" :data-p-active="isActive" v-bind="ptmi('root')">
|
||||
<slot />
|
||||
</div>
|
||||
</template>
|
||||
|
||||
<script>
|
||||
import BaseStepItem from './BaseStepItem.vue';
|
||||
|
||||
export default {
|
||||
name: 'StepItem',
|
||||
extends: BaseStepItem,
|
||||
inheritAttrs: false,
|
||||
inject: ['$pcStepper'],
|
||||
computed: {
|
||||
isActive() {
|
||||
return this.$pcStepper?.d_value === this.value;
|
||||
}
|
||||
}
|
||||
};
|
||||
</script>
|
|
@ -0,0 +1,11 @@
|
|||
{
|
||||
"main": "./stepitem.mjs",
|
||||
"module": "./stepitem.mjs",
|
||||
"types": "./StepItem.d.ts",
|
||||
"browser": {
|
||||
"./sfc": "./StepItem.vue"
|
||||
},
|
||||
"sideEffects": [
|
||||
"*.vue"
|
||||
]
|
||||
}
|
|
@ -0,0 +1,19 @@
|
|||
/**
|
||||
*
|
||||
* StepItem is a helper component for Stepper component.
|
||||
*
|
||||
* [Live Demo](https://www.primevue.org/stepper/)
|
||||
*
|
||||
* @module stepitemstyle
|
||||
*
|
||||
*/
|
||||
import { BaseStyle } from '../../base/style';
|
||||
|
||||
export enum StepItemClasses {
|
||||
/**
|
||||
* Class name of the root element
|
||||
*/
|
||||
root = 'p-stepitem'
|
||||
}
|
||||
|
||||
export interface StepItemStyle extends BaseStyle {}
|
|
@ -0,0 +1,15 @@
|
|||
import BaseStyle from 'primevue/base/style';
|
||||
|
||||
const classes = {
|
||||
root: ({ instance }) => [
|
||||
'p-stepitem',
|
||||
{
|
||||
'p-stepitem-active': instance.isActive
|
||||
}
|
||||
]
|
||||
};
|
||||
|
||||
export default BaseStyle.extend({
|
||||
name: 'stepitem',
|
||||
classes
|
||||
});
|
|
@ -0,0 +1,6 @@
|
|||
{
|
||||
"main": "./stepitemstyle.mjs",
|
||||
"module": "./stepitemstyle.mjs",
|
||||
"types": "./StepItemStyle.d.ts",
|
||||
"sideEffects": false
|
||||
}
|
|
@ -0,0 +1,22 @@
|
|||
<script>
|
||||
import BaseComponent from 'primevue/basecomponent';
|
||||
import StepListStyle from 'primevue/steplist/style';
|
||||
|
||||
export default {
|
||||
name: 'BaseStepList',
|
||||
extends: BaseComponent,
|
||||
props: {
|
||||
value: {
|
||||
type: String,
|
||||
default: undefined
|
||||
}
|
||||
},
|
||||
style: StepListStyle,
|
||||
provide() {
|
||||
return {
|
||||
$pcStepList: this,
|
||||
$parentInstance: this
|
||||
};
|
||||
}
|
||||
};
|
||||
</script>
|
|
@ -0,0 +1,121 @@
|
|||
/**
|
||||
*
|
||||
* StepList is a helper component for Stepper component.
|
||||
*
|
||||
* [Live Demo](https://www.primevue.org/stepper/)
|
||||
*
|
||||
* @module steplist
|
||||
*
|
||||
*/
|
||||
import { VNode } from 'vue';
|
||||
import { ComponentHooks } from '../basecomponent';
|
||||
import { PassThroughOptions } from '../passthrough';
|
||||
import { DefineComponent, DesignToken, EmitFn, GlobalComponentConstructor, PassThrough } from '../ts-helpers';
|
||||
|
||||
export declare type StepListPassThroughOptionType = StepListPassThroughAttributes | ((options: StepListPassThroughMethodOptions) => StepListPassThroughAttributes | string) | string | null | undefined;
|
||||
|
||||
/**
|
||||
* Custom passthrough(pt) option method.
|
||||
*/
|
||||
export interface StepListPassThroughMethodOptions {
|
||||
/**
|
||||
* Defines instance.
|
||||
*/
|
||||
instance: any;
|
||||
/**
|
||||
* Defines valid properties.
|
||||
*/
|
||||
props: StepListProps;
|
||||
/**
|
||||
* Defines valid attributes.
|
||||
*/
|
||||
attrs: any;
|
||||
/**
|
||||
* Defines parent options.
|
||||
*/
|
||||
parent: any;
|
||||
/**
|
||||
* Defines passthrough(pt) options in global config.
|
||||
*/
|
||||
global: object | undefined;
|
||||
}
|
||||
|
||||
/**
|
||||
* Custom passthrough(pt) options.
|
||||
* @see {@link StepListProps.pt}
|
||||
*/
|
||||
export interface StepListPassThroughOptions {
|
||||
/**
|
||||
* Used to pass attributes to the root's DOM element.
|
||||
*/
|
||||
root?: StepListPassThroughOptionType;
|
||||
/**
|
||||
* Used to manage all lifecycle hooks.
|
||||
* @see {@link BaseComponent.ComponentHooks}
|
||||
*/
|
||||
hooks?: ComponentHooks;
|
||||
}
|
||||
|
||||
export interface StepListPassThroughAttributes {
|
||||
[key: string]: any;
|
||||
}
|
||||
|
||||
/**
|
||||
* Defines valid properties in StepList component.
|
||||
*/
|
||||
export interface StepListProps {
|
||||
/**
|
||||
* Value of step.
|
||||
*/
|
||||
value: string;
|
||||
/**
|
||||
* It generates scoped CSS variables using design tokens for the component.
|
||||
*/
|
||||
dt?: DesignToken<any>;
|
||||
/**
|
||||
* Used to pass attributes to DOM elements inside the component.
|
||||
* @type {StepListPassThroughOptions}
|
||||
*/
|
||||
pt?: PassThrough<StepListPassThroughOptions>;
|
||||
/**
|
||||
* Used to configure passthrough(pt) options of the component.
|
||||
* @type {PassThroughOptions}
|
||||
*/
|
||||
ptOptions?: PassThroughOptions;
|
||||
}
|
||||
|
||||
/**
|
||||
* Defines valid slots in StepList slots.
|
||||
*/
|
||||
export interface StepListSlots {
|
||||
/**
|
||||
* Custom content template.
|
||||
*/
|
||||
default(): VNode[];
|
||||
}
|
||||
|
||||
export interface StepListEmitsOptions {}
|
||||
|
||||
export declare type StepListEmits = EmitFn<StepListEmitsOptions>;
|
||||
|
||||
/**
|
||||
* **PrimeVue - StepList**
|
||||
*
|
||||
* _StepList is a helper component for Stepper component._
|
||||
*
|
||||
* [Live Demo](https://www.primevue.org/stepper/)
|
||||
* --- ---
|
||||
* 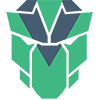
|
||||
*
|
||||
* @group Component
|
||||
*
|
||||
*/
|
||||
declare const StepList: DefineComponent<StepListProps, StepListSlots, StepListEmits>;
|
||||
|
||||
declare module 'vue' {
|
||||
export interface GlobalComponents {
|
||||
StepList: GlobalComponentConstructor<StepListProps, StepListSlots, StepListEmits>;
|
||||
}
|
||||
}
|
||||
|
||||
export default StepList;
|
|
@ -0,0 +1,15 @@
|
|||
<template>
|
||||
<div :class="cx('root')" v-bind="ptmi('root')">
|
||||
<slot />
|
||||
</div>
|
||||
</template>
|
||||
|
||||
<script>
|
||||
import BaseStepList from './BaseStepList.vue';
|
||||
|
||||
export default {
|
||||
name: 'StepList',
|
||||
extends: BaseStepList,
|
||||
inheritAttrs: false
|
||||
};
|
||||
</script>
|
|
@ -0,0 +1,11 @@
|
|||
{
|
||||
"main": "./steplist.mjs",
|
||||
"module": "./steplist.mjs",
|
||||
"types": "./StepList.d.ts",
|
||||
"browser": {
|
||||
"./sfc": "./StepList.vue"
|
||||
},
|
||||
"sideEffects": [
|
||||
"*.vue"
|
||||
]
|
||||
}
|
|
@ -0,0 +1,19 @@
|
|||
/**
|
||||
*
|
||||
* StepList is a helper component for Stepper component.
|
||||
*
|
||||
* [Live Demo](https://www.primevue.org/stepper/)
|
||||
*
|
||||
* @module stepliststyle
|
||||
*
|
||||
*/
|
||||
import { BaseStyle } from '../../base/style';
|
||||
|
||||
export enum StepListClasses {
|
||||
/**
|
||||
* Class name of the root element
|
||||
*/
|
||||
root = 'p-steplist'
|
||||
}
|
||||
|
||||
export interface StepListStyle extends BaseStyle {}
|
|
@ -0,0 +1,10 @@
|
|||
import BaseStyle from 'primevue/base/style';
|
||||
|
||||
const classes = {
|
||||
root: 'p-steplist'
|
||||
};
|
||||
|
||||
export default BaseStyle.extend({
|
||||
name: 'steplist',
|
||||
classes
|
||||
});
|
|
@ -0,0 +1,6 @@
|
|||
{
|
||||
"main": "./stepliststyle.mjs",
|
||||
"module": "./stepliststyle.mjs",
|
||||
"types": "./StepListStyle.d.ts",
|
||||
"sideEffects": false
|
||||
}
|
|
@ -0,0 +1,30 @@
|
|||
<script>
|
||||
import BaseComponent from 'primevue/basecomponent';
|
||||
import StepPanelStyle from 'primevue/steppanel/style';
|
||||
|
||||
export default {
|
||||
name: 'BaseStepPanel',
|
||||
extends: BaseComponent,
|
||||
props: {
|
||||
value: {
|
||||
type: [String, Number],
|
||||
default: undefined
|
||||
},
|
||||
asChild: {
|
||||
type: Boolean,
|
||||
default: false
|
||||
},
|
||||
as: {
|
||||
type: String,
|
||||
default: 'DIV'
|
||||
}
|
||||
},
|
||||
style: StepPanelStyle,
|
||||
provide() {
|
||||
return {
|
||||
$pcStepPanel: this,
|
||||
$parentInstance: this
|
||||
};
|
||||
}
|
||||
};
|
||||
</script>
|
|
@ -0,0 +1,148 @@
|
|||
/**
|
||||
*
|
||||
* StepPanel is a helper component for Stepper component.
|
||||
*
|
||||
* [Live Demo](https://www.primevue.org/stepper/)
|
||||
*
|
||||
* @module steppanel
|
||||
*
|
||||
*/
|
||||
import { VNode } from 'vue';
|
||||
import { ComponentHooks } from '../basecomponent';
|
||||
import { PassThroughOptions } from '../passthrough';
|
||||
import { DefineComponent, DesignToken, EmitFn, GlobalComponentConstructor, PassThrough } from '../ts-helpers';
|
||||
|
||||
export declare type StepPanelPassThroughOptionType = StepPanelPassThroughAttributes | ((options: StepPanelPassThroughMethodOptions) => StepPanelPassThroughAttributes | string) | string | null | undefined;
|
||||
|
||||
/**
|
||||
* Custom passthrough(pt) option method.
|
||||
*/
|
||||
export interface StepPanelPassThroughMethodOptions {
|
||||
/**
|
||||
* Defines instance.
|
||||
*/
|
||||
instance: any;
|
||||
/**
|
||||
* Defines valid properties.
|
||||
*/
|
||||
props: StepPanelProps;
|
||||
/**
|
||||
* Defines valid attributes.
|
||||
*/
|
||||
attrs: any;
|
||||
/**
|
||||
* Defines parent options.
|
||||
*/
|
||||
parent: any;
|
||||
/**
|
||||
* Defines passthrough(pt) options in global config.
|
||||
*/
|
||||
global: object | undefined;
|
||||
}
|
||||
|
||||
/**
|
||||
* Custom passthrough(pt) options.
|
||||
* @see {@link StepPanelProps.pt}
|
||||
*/
|
||||
export interface StepPanelPassThroughOptions {
|
||||
/**
|
||||
* Used to pass attributes to the root's DOM element.
|
||||
*/
|
||||
root?: StepPanelPassThroughOptionType;
|
||||
/**
|
||||
* Used to manage all lifecycle hooks.
|
||||
* @see {@link BaseComponent.ComponentHooks}
|
||||
*/
|
||||
hooks?: ComponentHooks;
|
||||
}
|
||||
|
||||
export interface StepPanelPassThroughAttributes {
|
||||
[key: string]: any;
|
||||
}
|
||||
|
||||
/**
|
||||
* Defines valid properties in StepPanel component.
|
||||
*/
|
||||
export interface StepPanelProps {
|
||||
/**
|
||||
* Value of step.
|
||||
*/
|
||||
value?: string | number | undefined;
|
||||
/**
|
||||
* Use to change the HTML tag of root element.
|
||||
* @defaultValue BUTTON
|
||||
*/
|
||||
as?: string | undefined;
|
||||
/**
|
||||
* When enabled, it changes the default rendered element for the one passed as a child element.
|
||||
* @defaultValue false
|
||||
*/
|
||||
asChild?: boolean | undefined;
|
||||
/**
|
||||
* It generates scoped CSS variables using design tokens for the component.
|
||||
*/
|
||||
dt?: DesignToken<any>;
|
||||
/**
|
||||
* Used to pass attributes to DOM elements inside the component.
|
||||
* @type {StepPanelPassThroughOptions}
|
||||
*/
|
||||
pt?: PassThrough<StepPanelPassThroughOptions>;
|
||||
/**
|
||||
* Used to configure passthrough(pt) options of the component.
|
||||
* @type {PassThroughOptions}
|
||||
*/
|
||||
ptOptions?: PassThroughOptions;
|
||||
}
|
||||
|
||||
/**
|
||||
* Defines valid slots in StepPanel slots.
|
||||
*/
|
||||
export interface StepPanelSlots {
|
||||
/**
|
||||
* Custom content template. Slot attributes can be used when asChild prop is true.
|
||||
*/
|
||||
default(scope: {
|
||||
/**
|
||||
* Whether the step is active.
|
||||
*/
|
||||
active: boolean;
|
||||
/**
|
||||
* Value of step.
|
||||
*/
|
||||
value: string | number;
|
||||
/**
|
||||
* A11t attributes
|
||||
*/
|
||||
a11yAttrs: any;
|
||||
/**
|
||||
* Click function.
|
||||
*/
|
||||
activateCallback: () => void;
|
||||
}): VNode[];
|
||||
}
|
||||
|
||||
export interface StepPanelEmitsOptions {}
|
||||
|
||||
export declare type StepPanelEmits = EmitFn<StepPanelEmitsOptions>;
|
||||
|
||||
/**
|
||||
* **PrimeVue - StepPanel**
|
||||
*
|
||||
* _StepPanel is a helper component for Stepper component._
|
||||
*
|
||||
* [Live Demo](https://www.primevue.org/stepper/)
|
||||
* --- ---
|
||||
* 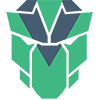
|
||||
*
|
||||
* @group Component
|
||||
*
|
||||
*/
|
||||
declare const StepPanel: DefineComponent<StepPanelProps, StepPanelSlots, StepPanelEmits>;
|
||||
|
||||
declare module 'vue' {
|
||||
export interface GlobalComponents {
|
||||
StepPanel: GlobalComponentConstructor<StepPanelProps, StepPanelSlots, StepPanelEmits>;
|
||||
}
|
||||
}
|
||||
|
||||
export default StepPanel;
|
|
@ -0,0 +1,99 @@
|
|||
<template>
|
||||
<template v-if="isVertical">
|
||||
<template v-if="!asChild">
|
||||
<transition name="p-toggleable-content" v-bind="ptm('transition')">
|
||||
<component v-show="active" :is="as" :id="id" :class="cx('root')" role="tabpanel" :aria-controls="ariaControls" v-bind="getPTOptions('root')">
|
||||
<StepperSeparator v-if="isSeparatorVisible" />
|
||||
<div :class="cx('content')" v-bind="getPTOptions('content')">
|
||||
<slot :active="active" :activateCallback="(val) => updateValue(val)" />
|
||||
</div>
|
||||
</component>
|
||||
</transition>
|
||||
</template>
|
||||
<slot v-else :active="active" :a11yAttrs="a11yAttrs" :activateCallback="(val) => updateValue(val)" />
|
||||
</template>
|
||||
<template v-else>
|
||||
<template v-if="!asChild">
|
||||
<component v-if="active" :is="as" :id="id" :class="cx('root')" role="tabpanel" :aria-controls="ariaControls" v-bind="getPTOptions('root')">
|
||||
<slot :active="active" :activateCallback="(val) => updateValue(val)" />
|
||||
</component>
|
||||
</template>
|
||||
<slot v-else-if="asChild && active" :active="active" :a11yAttrs="a11yAttrs" :activateCallback="(val) => updateValue(val)" />
|
||||
</template>
|
||||
</template>
|
||||
|
||||
<script>
|
||||
import { DomHandler, ObjectUtils } from 'primevue/utils';
|
||||
import StepperSeparator from '../stepper/StepperSeparator.vue';
|
||||
import BaseStepPanel from './BaseStepPanel.vue';
|
||||
|
||||
export default {
|
||||
name: 'StepPanel',
|
||||
extends: BaseStepPanel,
|
||||
inheritAttrs: false,
|
||||
inject: {
|
||||
$pcStepper: { default: null },
|
||||
$pcStepItem: { default: null },
|
||||
$pcStepList: { default: null }
|
||||
},
|
||||
data() {
|
||||
return {
|
||||
isSeparatorVisible: false
|
||||
};
|
||||
},
|
||||
mounted() {
|
||||
if (this.$el) {
|
||||
let stepElements = DomHandler.find(this.$pcStepper.$el, '[data-pc-name="step"]');
|
||||
let stepPanelEl = DomHandler.findSingle(this.isVertical ? this.$pcStepItem?.$el : this.$pcStepList?.$el, '[data-pc-name="step"]');
|
||||
let stepPanelIndex = ObjectUtils.findIndexInList(stepPanelEl, stepElements);
|
||||
|
||||
this.isSeparatorVisible = this.isVertical && stepPanelIndex !== stepElements.length - 1;
|
||||
}
|
||||
},
|
||||
methods: {
|
||||
getPTOptions(key) {
|
||||
const _ptm = key === 'root' ? this.ptmi : this.ptm;
|
||||
|
||||
return _ptm(key, {
|
||||
context: {
|
||||
active: this.active
|
||||
}
|
||||
});
|
||||
},
|
||||
updateValue(val) {
|
||||
this.$pcStepper.updateValue(val);
|
||||
}
|
||||
},
|
||||
computed: {
|
||||
active() {
|
||||
let activeValue = !!this.$pcStepItem ? this.$pcStepItem?.value : this.value;
|
||||
|
||||
return activeValue === this.$pcStepper?.d_value;
|
||||
},
|
||||
isVertical() {
|
||||
return !!this.$pcStepItem;
|
||||
},
|
||||
activeValue() {
|
||||
return this.isVertical ? this.$pcStepItem?.value : this.value;
|
||||
},
|
||||
id() {
|
||||
return `${this.$pcStepper?.id}_steppanel_${this.activeValue}`;
|
||||
},
|
||||
ariaControls() {
|
||||
return `${this.$pcStepper?.id}_step_${this.activeValue}`;
|
||||
},
|
||||
a11yAttrs() {
|
||||
return {
|
||||
id: this.id,
|
||||
role: 'tabpanel',
|
||||
'aria-controls': this.ariaControls,
|
||||
'data-pc-name': 'steppanel',
|
||||
'data-p-active': this.active
|
||||
};
|
||||
}
|
||||
},
|
||||
components: {
|
||||
StepperSeparator
|
||||
}
|
||||
};
|
||||
</script>
|
|
@ -0,0 +1,11 @@
|
|||
{
|
||||
"main": "./steppanel.mjs",
|
||||
"module": "./steppanel.mjs",
|
||||
"types": "./StepPanel.d.ts",
|
||||
"browser": {
|
||||
"./sfc": "./StepPanel.vue"
|
||||
},
|
||||
"sideEffects": [
|
||||
"*.vue"
|
||||
]
|
||||
}
|
|
@ -0,0 +1,19 @@
|
|||
/**
|
||||
*
|
||||
* StepPanel is a helper component for Stepper component.
|
||||
*
|
||||
* [Live Demo](https://www.primevue.org/stepper/)
|
||||
*
|
||||
* @module steppanelstyle
|
||||
*
|
||||
*/
|
||||
import { BaseStyle } from '../../base/style';
|
||||
|
||||
export enum StepPanelClasses {
|
||||
/**
|
||||
* Class name of the root element
|
||||
*/
|
||||
root = 'p-steppanel'
|
||||
}
|
||||
|
||||
export interface StepPanelStyle extends BaseStyle {}
|
|
@ -0,0 +1,16 @@
|
|||
import BaseStyle from 'primevue/base/style';
|
||||
|
||||
const classes = {
|
||||
root: ({ instance }) => [
|
||||
'p-steppanel',
|
||||
{
|
||||
'p-steppanel-active': instance.isVertical && instance.active
|
||||
}
|
||||
],
|
||||
content: 'p-steppanel-content'
|
||||
};
|
||||
|
||||
export default BaseStyle.extend({
|
||||
name: 'steppanel',
|
||||
classes
|
||||
});
|
|
@ -0,0 +1,6 @@
|
|||
{
|
||||
"main": "./steppanelstyle.mjs",
|
||||
"module": "./steppanelstyle.mjs",
|
||||
"types": "./StepPanelStyle.d.ts",
|
||||
"sideEffects": false
|
||||
}
|
|
@ -0,0 +1,22 @@
|
|||
<script>
|
||||
import BaseComponent from 'primevue/basecomponent';
|
||||
import StepPanelsStyle from 'primevue/steppanels/style';
|
||||
|
||||
export default {
|
||||
name: 'BaseStepPanels',
|
||||
extends: BaseComponent,
|
||||
props: {
|
||||
value: {
|
||||
type: String,
|
||||
default: undefined
|
||||
}
|
||||
},
|
||||
style: StepPanelsStyle,
|
||||
provide() {
|
||||
return {
|
||||
$pcStepPanels: this,
|
||||
$parentInstance: this
|
||||
};
|
||||
}
|
||||
};
|
||||
</script>
|
|
@ -0,0 +1,121 @@
|
|||
/**
|
||||
*
|
||||
* StepPanels is a helper component for Stepper component.
|
||||
*
|
||||
* [Live Demo](https://www.primevue.org/stepper/)
|
||||
*
|
||||
* @module steppanels
|
||||
*
|
||||
*/
|
||||
import { VNode } from 'vue';
|
||||
import { ComponentHooks } from '../basecomponent';
|
||||
import { PassThroughOptions } from '../passthrough';
|
||||
import { DefineComponent, DesignToken, EmitFn, GlobalComponentConstructor, PassThrough } from '../ts-helpers';
|
||||
|
||||
export declare type StepPanelsPassThroughOptionType = StepPanelsPassThroughAttributes | ((options: StepPanelsPassThroughMethodOptions) => StepPanelsPassThroughAttributes | string) | string | null | undefined;
|
||||
|
||||
/**
|
||||
* Custom passthrough(pt) option method.
|
||||
*/
|
||||
export interface StepPanelsPassThroughMethodOptions {
|
||||
/**
|
||||
* Defines instance.
|
||||
*/
|
||||
instance: any;
|
||||
/**
|
||||
* Defines valid properties.
|
||||
*/
|
||||
props: StepPanelsProps;
|
||||
/**
|
||||
* Defines valid attributes.
|
||||
*/
|
||||
attrs: any;
|
||||
/**
|
||||
* Defines parent options.
|
||||
*/
|
||||
parent: any;
|
||||
/**
|
||||
* Defines passthrough(pt) options in global config.
|
||||
*/
|
||||
global: object | undefined;
|
||||
}
|
||||
|
||||
/**
|
||||
* Custom passthrough(pt) options.
|
||||
* @see {@link StepPanelsProps.pt}
|
||||
*/
|
||||
export interface StepPanelsPassThroughOptions {
|
||||
/**
|
||||
* Used to pass attributes to the root's DOM element.
|
||||
*/
|
||||
root?: StepPanelsPassThroughOptionType;
|
||||
/**
|
||||
* Used to manage all lifecycle hooks.
|
||||
* @see {@link BaseComponent.ComponentHooks}
|
||||
*/
|
||||
hooks?: ComponentHooks;
|
||||
}
|
||||
|
||||
export interface StepPanelsPassThroughAttributes {
|
||||
[key: string]: any;
|
||||
}
|
||||
|
||||
/**
|
||||
* Defines valid properties in StepPanels component.
|
||||
*/
|
||||
export interface StepPanelsProps {
|
||||
/**
|
||||
* Value of step.
|
||||
*/
|
||||
value: string;
|
||||
/**
|
||||
* It generates scoped CSS variables using design tokens for the component.
|
||||
*/
|
||||
dt?: DesignToken<any>;
|
||||
/**
|
||||
* Used to pass attributes to DOM elements inside the component.
|
||||
* @type {StepPanelsPassThroughOptions}
|
||||
*/
|
||||
pt?: PassThrough<StepPanelsPassThroughOptions>;
|
||||
/**
|
||||
* Used to configure passthrough(pt) options of the component.
|
||||
* @type {PassThroughOptions}
|
||||
*/
|
||||
ptOptions?: PassThroughOptions;
|
||||
}
|
||||
|
||||
/**
|
||||
* Defines valid slots in StepPanels slots.
|
||||
*/
|
||||
export interface StepPanelsSlots {
|
||||
/**
|
||||
* Custom content template.
|
||||
*/
|
||||
default(): VNode[];
|
||||
}
|
||||
|
||||
export interface StepPanelsEmitsOptions {}
|
||||
|
||||
export declare type StepPanelsEmits = EmitFn<StepPanelsEmitsOptions>;
|
||||
|
||||
/**
|
||||
* **PrimeVue - StepPanels**
|
||||
*
|
||||
* _StepPanels is a helper component for Stepper component._
|
||||
*
|
||||
* [Live Demo](https://www.primevue.org/stepper/)
|
||||
* --- ---
|
||||
* 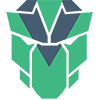
|
||||
*
|
||||
* @group Component
|
||||
*
|
||||
*/
|
||||
declare const StepPanels: DefineComponent<StepPanelsProps, StepPanelsSlots, StepPanelsEmits>;
|
||||
|
||||
declare module 'vue' {
|
||||
export interface GlobalComponents {
|
||||
StepPanels: GlobalComponentConstructor<StepPanelsProps, StepPanelsSlots, StepPanelsEmits>;
|
||||
}
|
||||
}
|
||||
|
||||
export default StepPanels;
|
|
@ -0,0 +1,15 @@
|
|||
<template>
|
||||
<div :class="cx('root')" v-bind="ptmi('root')">
|
||||
<slot />
|
||||
</div>
|
||||
</template>
|
||||
|
||||
<script>
|
||||
import BaseStepPanels from './BaseStepPanels.vue';
|
||||
|
||||
export default {
|
||||
name: 'StepPanels',
|
||||
extends: BaseStepPanels,
|
||||
inheritAttrs: false
|
||||
};
|
||||
</script>
|
|
@ -0,0 +1,11 @@
|
|||
{
|
||||
"main": "./steppanels.mjs",
|
||||
"module": "./steppanels.mjs",
|
||||
"types": "./StepPanels.d.ts",
|
||||
"browser": {
|
||||
"./sfc": "./StepPanels.vue"
|
||||
},
|
||||
"sideEffects": [
|
||||
"*.vue"
|
||||
]
|
||||
}
|
|
@ -0,0 +1,19 @@
|
|||
/**
|
||||
*
|
||||
* StepPanels
|
||||
*
|
||||
* [Live Demo](https://www.primevue.org/stepper/)
|
||||
*
|
||||
* @module steppanelsstyle
|
||||
*
|
||||
*/
|
||||
import { BaseStyle } from '../../base/style';
|
||||
|
||||
export enum StepPanelsClasses {
|
||||
/**
|
||||
* Class name of the root element
|
||||
*/
|
||||
root = 'p-steppanels'
|
||||
}
|
||||
|
||||
export interface StepPanelsStyle extends BaseStyle {}
|
|
@ -0,0 +1,10 @@
|
|||
import BaseStyle from 'primevue/base/style';
|
||||
|
||||
const classes = {
|
||||
root: 'p-steppanels'
|
||||
};
|
||||
|
||||
export default BaseStyle.extend({
|
||||
name: 'steppanels',
|
||||
classes
|
||||
});
|
|
@ -0,0 +1,6 @@
|
|||
{
|
||||
"main": "./steppanelsstyle.mjs",
|
||||
"module": "./steppanelsstyle.mjs",
|
||||
"types": "./StepPanelsStyle.d.ts",
|
||||
"sideEffects": false
|
||||
}
|
|
@ -6,13 +6,9 @@ export default {
|
|||
name: 'BaseStepper',
|
||||
extends: BaseComponent,
|
||||
props: {
|
||||
activeStep: {
|
||||
type: Number,
|
||||
default: 0
|
||||
},
|
||||
orientation: {
|
||||
type: String,
|
||||
default: 'horizontal'
|
||||
value: {
|
||||
type: [String, Number],
|
||||
default: undefined
|
||||
},
|
||||
linear: {
|
||||
type: Boolean,
|
||||
|
|
|
@ -10,7 +10,6 @@
|
|||
import { VNode } from 'vue';
|
||||
import { ComponentHooks } from '../basecomponent';
|
||||
import { PassThroughOptions } from '../passthrough';
|
||||
import { StepperPanelPassThroughOptionType } from '../stepperpanel';
|
||||
import { DefineComponent, DesignToken, EmitFn, GlobalComponentConstructor, PassThrough } from '../ts-helpers';
|
||||
|
||||
export declare type StepperPassThroughOptionType = StepperPassThroughAttributes | ((options: StepperPassThroughMethodOptions) => StepperPassThroughAttributes | string) | string | null | undefined;
|
||||
|
@ -54,18 +53,6 @@ export interface StepperPassThroughOptions {
|
|||
* Used to pass attributes to the root's DOM element.
|
||||
*/
|
||||
root?: StepperPassThroughOptionType;
|
||||
/**
|
||||
* Used to pass attributes to the list's DOM element.
|
||||
*/
|
||||
list?: StepperPassThroughOptionType;
|
||||
/**
|
||||
* Used to pass attributes to the panels' DOM element.
|
||||
*/
|
||||
panels?: StepperPassThroughOptionType;
|
||||
/**
|
||||
* Used to pass attributes to the end handler's DOM element.
|
||||
*/
|
||||
stepperpanel?: StepperPanelPassThroughOptionType;
|
||||
/**
|
||||
* Used to manage all lifecycle hooks.
|
||||
* @see {@link BaseComponent.ComponentHooks}
|
||||
|
@ -85,44 +72,24 @@ export interface StepperPassThroughAttributes {
|
|||
*/
|
||||
export interface StepperState {
|
||||
/**
|
||||
* Current active index state.
|
||||
* Current active value state.
|
||||
*/
|
||||
d_activeStep: number;
|
||||
d_value: any;
|
||||
/**
|
||||
* Unique id for the Stepper component.
|
||||
*/
|
||||
id: string;
|
||||
}
|
||||
|
||||
/**
|
||||
* Custom tab change event.
|
||||
* @see {@link StepperEmitsOptions['step-change']}
|
||||
*/
|
||||
export interface StepperChangeEvent {
|
||||
/**
|
||||
* Browser event
|
||||
*/
|
||||
originalEvent: Event;
|
||||
/**
|
||||
* Index of the selected stepper panel
|
||||
*/
|
||||
index: number;
|
||||
}
|
||||
|
||||
/**
|
||||
* Defines valid properties in Stepper component.
|
||||
*/
|
||||
export interface StepperProps {
|
||||
/**
|
||||
* Active step index of stepper.
|
||||
* @defaultValue 0
|
||||
* Active value of stepper.
|
||||
* @defaultValue null
|
||||
*/
|
||||
activeStep?: number | undefined;
|
||||
/**
|
||||
* Orientation of the stepper.
|
||||
* @defaultValue horizontal
|
||||
*/
|
||||
orientation?: 'horizontal' | 'vertical' | undefined;
|
||||
value?: string | number | undefined;
|
||||
/**
|
||||
* Whether the steps are clickable or not.
|
||||
* @defaultValue false
|
||||
|
@ -153,6 +120,10 @@ export interface StepperProps {
|
|||
* Defines valid slots in Stepper component.
|
||||
*/
|
||||
export interface StepperSlots {
|
||||
/**
|
||||
* Custom default template.
|
||||
*/
|
||||
default(): VNode[];
|
||||
/**
|
||||
* Custom start template.
|
||||
*/
|
||||
|
@ -169,13 +140,9 @@ export interface StepperSlots {
|
|||
export interface StepperEmitsOptions {
|
||||
/**
|
||||
* Emitted when the value changes.
|
||||
* @param {number | number[]} value - New value.
|
||||
* @param {any} value - New value.
|
||||
*/
|
||||
'update:activeStep'(value: number): void;
|
||||
/**
|
||||
* Callback to invoke when an active panel is changed.
|
||||
*/
|
||||
'step-change'(event: StepperChangeEvent): void;
|
||||
'update:value'(value: any): void;
|
||||
}
|
||||
|
||||
export declare type StepperEmits = EmitFn<StepperEmitsOptions>;
|
||||
|
|
|
@ -1,266 +1,50 @@
|
|||
<template>
|
||||
<div :class="cx('root')" role="tablist" v-bind="ptmi('root')">
|
||||
<slot v-if="$slots.start" name="start" />
|
||||
|
||||
<template v-if="orientation === 'horizontal'">
|
||||
<ul ref="nav" :class="cx('list')" v-bind="ptm('list')">
|
||||
<li
|
||||
v-for="(step, index) of stepperpanels"
|
||||
:key="getStepKey(step, index)"
|
||||
:class="cx('stepper.item', { step, index })"
|
||||
:aria-current="isStepActive(index) ? 'step' : undefined"
|
||||
role="presentation"
|
||||
v-bind="{ ...getStepPT(step, 'root', index), ...getStepPT(step, 'item', index) }"
|
||||
data-pc-name="stepperpanel"
|
||||
:data-p-active="isStepActive(index)"
|
||||
:data-p-disabled="isItemDisabled(index)"
|
||||
:data-pc-index="index"
|
||||
>
|
||||
<slot name="header">
|
||||
<StepperHeader
|
||||
:id="getStepHeaderActionId(index)"
|
||||
:template="step.children?.header"
|
||||
:stepperpanel="step"
|
||||
:index="index"
|
||||
:disabled="isItemDisabled(index)"
|
||||
:active="isStepActive(index)"
|
||||
:highlighted="index < d_activeStep"
|
||||
:class="cx('stepper.itemHeader')"
|
||||
:aria-controls="getStepContentId(index)"
|
||||
:clickCallback="(event) => onItemClick(event, index)"
|
||||
:getStepPT="getStepPT"
|
||||
:getStepProp="getStepProp"
|
||||
:unstyled="unstyled"
|
||||
/>
|
||||
</slot>
|
||||
<slot v-if="index !== stepperpanels.length - 1" name="separator">
|
||||
<StepperSeparator
|
||||
:template="step.children?.separator"
|
||||
:separatorClass="cx('stepper.separator')"
|
||||
:stepperpanel="step"
|
||||
:index="index"
|
||||
:active="isStepActive(index)"
|
||||
:highlighted="index < d_activeStep"
|
||||
:getStepPT="getStepPT(step, 'separator', index)"
|
||||
:unstyled="unstyled"
|
||||
/>
|
||||
</slot>
|
||||
</li>
|
||||
</ul>
|
||||
<div :class="cx('panels')" v-bind="ptm('panels')">
|
||||
<template v-for="(step, index) of stepperpanels" :key="getStepKey(step, index)">
|
||||
<StepperContent
|
||||
v-show="isStepActive(index)"
|
||||
:id="getStepContentId(index)"
|
||||
:template="step?.children?.content"
|
||||
:stepperpanel="step"
|
||||
:index="index"
|
||||
:active="isStepActive(index)"
|
||||
:highlighted="index < d_activeStep"
|
||||
:clickCallback="(event) => onItemClick(event, index)"
|
||||
:prevCallback="(event) => prevCallback(event, index)"
|
||||
:nextCallback="(event) => nextCallback(event, index)"
|
||||
:getStepPT="getStepPT"
|
||||
:aria-labelledby="getStepHeaderActionId(index)"
|
||||
:unstyled="unstyled"
|
||||
/>
|
||||
</template>
|
||||
</div>
|
||||
</template>
|
||||
<template v-else-if="orientation === 'vertical'">
|
||||
<div
|
||||
v-for="(step, index) of stepperpanels"
|
||||
ref="nav"
|
||||
:key="getStepKey(step, index)"
|
||||
:class="cx('panel', { step, index })"
|
||||
:aria-current="isStepActive(index) ? 'step' : undefined"
|
||||
v-bind="{ ...getStepPT(step, 'root', index), ...getStepPT(step, 'panel', index) }"
|
||||
data-pc-name="stepperpanel"
|
||||
:data-p-active="isStepActive(index)"
|
||||
:data-p-disabled="isItemDisabled(index)"
|
||||
:data-pc-index="index"
|
||||
>
|
||||
<div :class="cx('stepper.item', { step, index })" v-bind="getStepPT(step, 'item', index)">
|
||||
<slot name="header">
|
||||
<StepperHeader
|
||||
:id="getStepHeaderActionId(index)"
|
||||
:template="step.children?.header"
|
||||
:stepperpanel="step"
|
||||
:index="index"
|
||||
:disabled="isItemDisabled(index)"
|
||||
:active="isStepActive(index)"
|
||||
:highlighted="index < d_activeStep"
|
||||
:class="cx('stepper.itemHeader')"
|
||||
:aria-controls="getStepContentId(index)"
|
||||
:clickCallback="(event) => onItemClick(event, index)"
|
||||
:getStepPT="getStepPT"
|
||||
:getStepProp="getStepProp"
|
||||
/>
|
||||
</slot>
|
||||
</div>
|
||||
|
||||
<transition name="p-toggleable-content" v-bind="getStepPT(step, 'transition', index)">
|
||||
<div v-show="isStepActive(index)" :class="cx('stepper.panelContentContainer')" v-bind="getStepPT(step, 'panelContentContainer', index)">
|
||||
<slot v-if="index !== stepperpanels.length - 1" name="separator">
|
||||
<StepperSeparator
|
||||
:template="step.children?.separator"
|
||||
:separatorClass="cx('stepper.separator')"
|
||||
:stepperpanel="step"
|
||||
:index="index"
|
||||
:active="isStepActive(index)"
|
||||
:highlighted="index < d_activeStep"
|
||||
:getStepPT="getStepPT(step, 'separator', index)"
|
||||
/>
|
||||
</slot>
|
||||
<slot name="content">
|
||||
<StepperContent
|
||||
:id="getStepContentId(index)"
|
||||
:template="step?.children?.content"
|
||||
:stepperpanel="step"
|
||||
:index="index"
|
||||
:active="isStepActive(index)"
|
||||
:highlighted="index < d_activeStep"
|
||||
:clickCallback="(event) => onItemClick(event, index)"
|
||||
:prevCallback="(event) => prevCallback(event, index)"
|
||||
:nextCallback="(event) => nextCallback(event, index)"
|
||||
:getStepPT="getStepPT"
|
||||
:aria-labelledby="getStepHeaderActionId(index)"
|
||||
/>
|
||||
</slot>
|
||||
</div>
|
||||
</transition>
|
||||
</div>
|
||||
</template>
|
||||
<slot />
|
||||
<slot v-if="$slots.end" name="end" />
|
||||
</div>
|
||||
</template>
|
||||
|
||||
<script>
|
||||
import { UniqueComponentId } from 'primevue/utils';
|
||||
import { mergeProps } from 'vue';
|
||||
import BaseStepper from './BaseStepper.vue';
|
||||
import StepperContent from './StepperContent.vue';
|
||||
import StepperHeader from './StepperHeader.vue';
|
||||
import StepperSeparator from './StepperSeparator.vue';
|
||||
|
||||
export default {
|
||||
name: 'Stepper',
|
||||
extends: BaseStepper,
|
||||
inheritAttrs: false,
|
||||
emits: ['update:activeStep', 'step-change'],
|
||||
emits: ['update:value'],
|
||||
data() {
|
||||
return {
|
||||
id: this.$attrs.id,
|
||||
d_activeStep: this.activeStep
|
||||
d_value: this.value
|
||||
};
|
||||
},
|
||||
watch: {
|
||||
'$attrs.id': function (newValue) {
|
||||
'$attrs.id'(newValue) {
|
||||
this.id = newValue || UniqueComponentId();
|
||||
},
|
||||
activeStep(newValue) {
|
||||
this.d_activeStep = newValue;
|
||||
value(newValue) {
|
||||
this.d_value = newValue;
|
||||
}
|
||||
},
|
||||
mounted() {
|
||||
this.id = this.id || UniqueComponentId();
|
||||
},
|
||||
methods: {
|
||||
isStep(child) {
|
||||
return child.type.name === 'StepperPanel';
|
||||
},
|
||||
isStepActive(index) {
|
||||
return this.d_activeStep === index;
|
||||
},
|
||||
getStepProp(step, name) {
|
||||
return step.props ? step.props[name] : undefined;
|
||||
},
|
||||
getStepKey(step, index) {
|
||||
return this.getStepProp(step, 'header') || index;
|
||||
},
|
||||
getStepHeaderActionId(index) {
|
||||
return `${this.id}_${index}_header_action`;
|
||||
},
|
||||
getStepContentId(index) {
|
||||
return `${this.id}_${index}_content`;
|
||||
},
|
||||
getStepPT(step, key, index) {
|
||||
const count = this.stepperpanels.length;
|
||||
const stepMetaData = {
|
||||
props: step.props,
|
||||
parent: {
|
||||
instance: this,
|
||||
props: this.$props,
|
||||
state: this.$data
|
||||
},
|
||||
context: {
|
||||
index,
|
||||
count,
|
||||
first: index === 0,
|
||||
last: index === count - 1,
|
||||
active: this.isStepActive(index),
|
||||
highlighted: index < this.d_activeStep,
|
||||
disabled: this.isItemDisabled(index)
|
||||
}
|
||||
};
|
||||
|
||||
return mergeProps(this.ptm(`stepperpanel.${key}`, { stepperpanel: stepMetaData }), this.ptm(`stepperpanel.${key}`, stepMetaData), this.ptmo(this.getStepProp(step, 'pt'), key, stepMetaData));
|
||||
},
|
||||
updateActiveStep(event, index) {
|
||||
this.d_activeStep = index;
|
||||
|
||||
this.$emit('update:activeStep', index);
|
||||
this.$emit('step-change', {
|
||||
originalEvent: event,
|
||||
index
|
||||
});
|
||||
},
|
||||
onItemClick(event, index) {
|
||||
if (this.linear) {
|
||||
event.preventDefault();
|
||||
|
||||
return;
|
||||
}
|
||||
|
||||
if (index !== this.d_activeStep) {
|
||||
this.updateActiveStep(event, index);
|
||||
updateValue(newValue) {
|
||||
if (this.d_value !== newValue) {
|
||||
this.d_value = newValue;
|
||||
this.$emit('update:value', newValue);
|
||||
}
|
||||
},
|
||||
isItemDisabled(index) {
|
||||
return this.linear && !this.isStepActive(index);
|
||||
isStepActive(value) {
|
||||
return this.d_value === value;
|
||||
},
|
||||
prevCallback(event, index) {
|
||||
if (index !== 0) {
|
||||
this.updateActiveStep(event, index - 1);
|
||||
}
|
||||
},
|
||||
nextCallback(event, index) {
|
||||
if (index !== this.stepperpanels.length - 1) {
|
||||
this.updateActiveStep(event, index + 1);
|
||||
}
|
||||
isStepDisabled() {
|
||||
return this.linear;
|
||||
}
|
||||
},
|
||||
computed: {
|
||||
stepperpanels() {
|
||||
return this.$slots.default().reduce((stepperpanels, child) => {
|
||||
if (this.isStep(child)) {
|
||||
stepperpanels.push(child);
|
||||
} else if (child.children && child.children instanceof Array) {
|
||||
child.children.forEach((nestedChild) => {
|
||||
if (this.isStep(nestedChild)) {
|
||||
stepperpanels.push(nestedChild);
|
||||
}
|
||||
});
|
||||
}
|
||||
|
||||
return stepperpanels;
|
||||
}, []);
|
||||
}
|
||||
},
|
||||
components: {
|
||||
StepperContent,
|
||||
StepperHeader,
|
||||
StepperSeparator
|
||||
}
|
||||
};
|
||||
</script>
|
||||
|
|
|
@ -1,47 +0,0 @@
|
|||
<template>
|
||||
<div
|
||||
:id="id"
|
||||
:class="cx('stepper.panelContent', { stepperpanel, index })"
|
||||
role="tabpanel"
|
||||
:aria-labelledby="ariaLabelledby"
|
||||
v-bind="{ ...getStepPT(stepperpanel, 'root', index), ...getStepPT(stepperpanel, 'panelContent', index) }"
|
||||
data-pc-name="stepperpanel"
|
||||
:data-pc-index="index"
|
||||
:data-p-active="active"
|
||||
>
|
||||
<component
|
||||
v-if="template"
|
||||
:is="template"
|
||||
:index="index"
|
||||
:active="active"
|
||||
:highlighted="highlighted"
|
||||
:clickCallback="(event) => onItemClick(event, index)"
|
||||
:prevCallback="(event) => prevCallback(event, index)"
|
||||
:nextCallback="(event) => nextCallback(event, index)"
|
||||
></component>
|
||||
<component v-else :is="stepperpanel"></component>
|
||||
</div>
|
||||
</template>
|
||||
|
||||
<script>
|
||||
import BaseComponent from 'primevue/basecomponent';
|
||||
|
||||
export default {
|
||||
name: 'StepperContent',
|
||||
hostName: 'Stepper',
|
||||
extends: BaseComponent,
|
||||
props: {
|
||||
id: null,
|
||||
template: null,
|
||||
ariaLabelledby: null,
|
||||
stepperpanel: null,
|
||||
index: null,
|
||||
active: null,
|
||||
highlighted: null,
|
||||
clickCallback: null,
|
||||
prevCallback: null,
|
||||
nextCallback: null,
|
||||
getStepPT: null
|
||||
}
|
||||
};
|
||||
</script>
|
|
@ -1,41 +0,0 @@
|
|||
<template>
|
||||
<component
|
||||
v-if="template"
|
||||
:is="template"
|
||||
:index="index"
|
||||
:active="active"
|
||||
:highlighted="highlighted"
|
||||
:class="cx('stepper.itemHeader')"
|
||||
:headerClass="cx('stepper.itemHeader')"
|
||||
:numberClass="cx('stepper.itemNumber')"
|
||||
:titleClass="cx('stepper.itemTitle')"
|
||||
:clickCallback="(event) => clickCallback(event, index)"
|
||||
/>
|
||||
<button v-else :id="id" :class="cx('stepper.itemHeader')" role="tab" :tabindex="disabled ? -1 : undefined" :aria-controls="ariaControls" @click="clickCallback($event, index)" v-bind="getStepPT(stepperpanel, 'itemHeader', index)">
|
||||
<span :class="cx('stepper.itemNumber')" v-bind="getStepPT(stepperpanel, 'itemNumber', index)">{{ index + 1 }}</span>
|
||||
<span :class="cx('stepper.itemTitle')" v-bind="getStepPT(stepperpanel, 'itemTitle', index)">{{ getStepProp(stepperpanel, 'header') }}</span>
|
||||
</button>
|
||||
</template>
|
||||
|
||||
<script>
|
||||
import BaseComponent from 'primevue/basecomponent';
|
||||
|
||||
export default {
|
||||
name: 'StepperHeader',
|
||||
hostName: 'Stepper',
|
||||
extends: BaseComponent,
|
||||
props: {
|
||||
id: null,
|
||||
template: null,
|
||||
stepperpanel: null,
|
||||
index: null,
|
||||
disabled: null,
|
||||
active: null,
|
||||
highlighted: null,
|
||||
ariaControls: null,
|
||||
clickCallback: null,
|
||||
getStepPT: null,
|
||||
getStepProp: null
|
||||
}
|
||||
};
|
||||
</script>
|
|
@ -1,6 +1,5 @@
|
|||
<template>
|
||||
<component v-if="template" :is="template" :class="separatorClass" :index="index" :active="active" :highlighted="highlighted" />
|
||||
<span v-else :class="separatorClass" aria-hidden="true" v-bind="getStepPT" />
|
||||
<span :class="cx('separator')" v-bind="ptm('separator')" />
|
||||
</template>
|
||||
|
||||
<script>
|
||||
|
@ -9,15 +8,6 @@ import BaseComponent from 'primevue/basecomponent';
|
|||
export default {
|
||||
name: 'StepperSeparator',
|
||||
hostName: 'Stepper',
|
||||
extends: BaseComponent,
|
||||
props: {
|
||||
template: null,
|
||||
separatorClass: null,
|
||||
stepperpanel: null,
|
||||
index: null,
|
||||
active: null,
|
||||
highlighted: null,
|
||||
getStepPT: null
|
||||
}
|
||||
extends: BaseComponent
|
||||
};
|
||||
</script>
|
||||
|
|
|
@ -15,45 +15,9 @@ export enum StepperClasses {
|
|||
*/
|
||||
root = 'p-stepper',
|
||||
/**
|
||||
* Class name of the list element
|
||||
* Class name of the separator element
|
||||
*/
|
||||
list = 'p-stepper-list',
|
||||
/**
|
||||
* Class name of the stepper item element
|
||||
*/
|
||||
stepperItem = 'p-stepper-item',
|
||||
/**
|
||||
* Class name of the stepper item header element
|
||||
*/
|
||||
stepperItemHeader = 'p-stepper-item-header',
|
||||
/**
|
||||
* Class name of the stepper item number element
|
||||
*/
|
||||
stepperItemNumber = 'p-stepper-item-number',
|
||||
/**
|
||||
* Class name of the stepper item title element
|
||||
*/
|
||||
stepperItemTitle = 'p-stepper-item-title',
|
||||
/**
|
||||
* Class name of the stepper separator element
|
||||
*/
|
||||
stepperSeparator = 'p-stepper-separator',
|
||||
/**
|
||||
* Class name of the stepper panel content container element
|
||||
*/
|
||||
stepperPanelContentContainer = 'p-stepper-panel-content-container',
|
||||
/**
|
||||
* Class name of the stepper panel content element
|
||||
*/
|
||||
stepperPanelContent = 'p-stepper-panel-content',
|
||||
/**
|
||||
* Class name of the panels element
|
||||
*/
|
||||
panels = 'p-stepper-panels',
|
||||
/**
|
||||
* Class name of the panel element
|
||||
*/
|
||||
panel = 'p-stepper-panel'
|
||||
separator = 'p-stepper-separator'
|
||||
}
|
||||
|
||||
export interface StepperStyle extends BaseStyle {}
|
||||
|
|
|
@ -1,7 +1,7 @@
|
|||
import BaseStyle from 'primevue/base/style';
|
||||
|
||||
const theme = ({ dt }) => `
|
||||
.p-stepper-list {
|
||||
.p-steplist {
|
||||
position: relative;
|
||||
display: flex;
|
||||
justify-content: space-between;
|
||||
|
@ -12,20 +12,20 @@ const theme = ({ dt }) => `
|
|||
overflow-x: auto;
|
||||
}
|
||||
|
||||
.p-stepper-item {
|
||||
.p-step {
|
||||
position: relative;
|
||||
display: flex;
|
||||
flex: 1 1 auto;
|
||||
align-items: center;
|
||||
gap: ${dt('stepper.item.gap')};
|
||||
padding: ${dt('stepper.item.padding')};
|
||||
gap: ${dt('stepper.step.gap')};
|
||||
padding: ${dt('stepper.step.padding')};
|
||||
}
|
||||
|
||||
.p-stepper-item:last-of-type {
|
||||
.p-step:last-of-type {
|
||||
flex: initial;
|
||||
}
|
||||
|
||||
.p-stepper-item-header {
|
||||
.p-step-header {
|
||||
border: 0 none;
|
||||
display: inline-flex;
|
||||
align-items: center;
|
||||
|
@ -34,82 +34,86 @@ const theme = ({ dt }) => `
|
|||
transition: background ${dt('stepper.transition.duration')}, color ${dt('stepper.transition.duration')}, border-color ${dt('stepper.transition.duration')}, outline-color ${dt('stepper.transition.duration')}, box-shadow ${dt(
|
||||
'stepper.transition.duration'
|
||||
)};
|
||||
border-radius: ${dt('stepper.item.header.border.radius')};
|
||||
border-radius: ${dt('stepper.step.header.border.radius')};
|
||||
outline-color: transparent;
|
||||
background: transparent;
|
||||
padding: ${dt('stepper.item.header.padding')};
|
||||
gap: ${dt('stepper.item.header.gap')};
|
||||
padding: ${dt('stepper.step.header.padding')};
|
||||
gap: ${dt('stepper.step.header.gap')};
|
||||
}
|
||||
|
||||
.p-stepper-item-header:focus-visible {
|
||||
box-shadow: ${dt('stepper.item.header.focus.ring.shadow')};
|
||||
outline: ${dt('stepper.item.header.focus.ring.width')} ${dt('stepper.item.header.focus.ring.style')} ${dt('stepper.item.header.focus.ring.color')};
|
||||
outline-offset: ${dt('stepper.item.header.focus.ring.offset')};
|
||||
.p-step-header:focus-visible {
|
||||
box-shadow: ${dt('stepper.step.header.focus.ring.shadow')};
|
||||
outline: ${dt('stepper.step.header.focus.ring.width')} ${dt('stepper.step.header.focus.ring.style')} ${dt('stepper.step.header.focus.ring.color')};
|
||||
outline-offset: ${dt('stepper.step.header.focus.ring.offset')};
|
||||
}
|
||||
|
||||
.p-stepper.p-stepper-readonly .p-stepper-item {
|
||||
.p-stepper.p-stepper-readonly .p-step {
|
||||
cursor: auto;
|
||||
}
|
||||
|
||||
.p-stepper-item-title {
|
||||
.p-step-title {
|
||||
display: block;
|
||||
white-space: nowrap;
|
||||
overflow: hidden;
|
||||
text-overflow: ellipsis;
|
||||
max-width: 100%;
|
||||
color: ${dt('stepper.item.title.color')};
|
||||
font-weight: ${dt('stepper.item.title.font.weight')};
|
||||
color: ${dt('stepper.step.title.color')};
|
||||
font-weight: ${dt('stepper.step.title.font.weight')};
|
||||
transition: background ${dt('stepper.transition.duration')}, color ${dt('stepper.transition.duration')}, border-color ${dt('stepper.transition.duration')}, box-shadow ${dt('stepper.transition.duration')}, outline-color ${dt(
|
||||
'stepper.transition.duration'
|
||||
)};
|
||||
}
|
||||
|
||||
.p-stepper-item-number {
|
||||
.p-step-number {
|
||||
display: flex;
|
||||
align-items: center;
|
||||
justify-content: center;
|
||||
color: ${dt('stepper.item.number.color')};
|
||||
border: 2px solid ${dt('stepper.item.number.border.color')};
|
||||
background: ${dt('stepper.item.number.background')};
|
||||
min-width: ${dt('stepper.item.number.size')};
|
||||
height: ${dt('stepper.item.number.size')};
|
||||
line-height: ${dt('stepper.item.number.size')};
|
||||
font-size: ${dt('stepper.item.number.font.size')};
|
||||
color: ${dt('stepper.step.number.color')};
|
||||
border: 2px solid ${dt('stepper.step.number.border.color')};
|
||||
background: ${dt('stepper.step.number.background')};
|
||||
min-width: ${dt('stepper.step.number.size')};
|
||||
height: ${dt('stepper.step.number.size')};
|
||||
line-height: ${dt('stepper.step.number.size')};
|
||||
font-size: ${dt('stepper.step.number.font.size')};
|
||||
z-index: 1;
|
||||
border-radius: ${dt('stepper.item.number.border.radius')};
|
||||
border-radius: ${dt('stepper.step.number.border.radius')};
|
||||
position: relative;
|
||||
font-weight: ${dt('stepper.item.number.font.weight')};
|
||||
font-weight: ${dt('stepper.step.number.font.weight')};
|
||||
}
|
||||
|
||||
.p-stepper-item-number::after {
|
||||
.p-step-number::after {
|
||||
content: " ";
|
||||
position: absolute;
|
||||
width: 100%;
|
||||
height: 100%;
|
||||
border-radius: ${dt('stepper.item.number.border.radius')};
|
||||
box-shadow: ${dt('stepper.item.number.shadow')};
|
||||
border-radius: ${dt('stepper.step.number.border.radius')};
|
||||
box-shadow: ${dt('stepper.step.number.shadow')};
|
||||
}
|
||||
|
||||
.p-stepper-item-active .p-stepper-item-header {
|
||||
.p-step-active .p-step-header {
|
||||
cursor: default;
|
||||
}
|
||||
|
||||
.p-stepper-item-active .p-stepper-item-number {
|
||||
background: ${dt('stepper.item.number.active.background')};
|
||||
border-color: ${dt('stepper.item.number.active.border.color')};
|
||||
color: ${dt('stepper.item.number.active.color')};
|
||||
.p-step-active .p-step-number {
|
||||
background: ${dt('stepper.step.number.active.background')};
|
||||
border-color: ${dt('stepper.step.number.active.border.color')};
|
||||
color: ${dt('stepper.step.number.active.color')};
|
||||
}
|
||||
|
||||
.p-stepper-item-active .p-stepper-item-title {
|
||||
color: ${dt('stepper.item.title.active.color')};
|
||||
.p-step-active .p-step-title {
|
||||
color: ${dt('stepper.step.title.active.color')};
|
||||
}
|
||||
|
||||
.p-stepper-item:not(.p-disabled):focus-visible {
|
||||
.p-step:not(.p-disabled):focus-visible {
|
||||
outline: ${dt('focus.ring.width')} ${dt('focus.ring.style')} ${dt('focus.ring.color')};
|
||||
outline-offset: ${dt('focus.ring.offset')};
|
||||
}
|
||||
|
||||
.p-stepper-item:has(~ .p-stepper-item-active) .p-stepper-separator {
|
||||
.p-step:has(~ .p-step-active) .p-stepper-separator {
|
||||
background: ${dt('stepper.separator.active.background')};
|
||||
}
|
||||
|
||||
.p-step:has(~ .p-step-active) .p-stepper-separator {
|
||||
background: ${dt('stepper.separator.active.background')};
|
||||
}
|
||||
|
||||
|
@ -123,63 +127,59 @@ const theme = ({ dt }) => `
|
|||
)};
|
||||
}
|
||||
|
||||
.p-stepper-panels {
|
||||
padding: ${dt('stepper.panel.content.orientation.horizontal.padding')};
|
||||
.p-steppanels {
|
||||
padding: ${dt('stepper.steppanels.padding')};
|
||||
}
|
||||
|
||||
.p-stepper-panel-content {
|
||||
background: ${dt('stepper.panel.content.background')};
|
||||
color: ${dt('stepper.panel.content.color')};
|
||||
.p-steppanel {
|
||||
background: ${dt('stepper.steppanel.content.background')};
|
||||
color: ${dt('stepper.steppanel.content.color')};
|
||||
}
|
||||
|
||||
.p-stepper-vertical .p-stepper-list {
|
||||
flex-direction: column;
|
||||
}
|
||||
|
||||
.p-stepper-vertical {
|
||||
.p-stepper:has(.p-stepitem) {
|
||||
display: flex;
|
||||
flex-direction: column;
|
||||
}
|
||||
|
||||
.p-stepper-vertical .p-stepper-panel-content-container {
|
||||
display: flex;
|
||||
flex: 1 1 auto;
|
||||
}
|
||||
|
||||
.p-stepper-vertical .p-stepper-panel {
|
||||
.p-stepitem {
|
||||
display: flex;
|
||||
flex-direction: column;
|
||||
flex: initial;
|
||||
}
|
||||
|
||||
.p-stepper-vertical .p-stepper-panel.p-stepper-panel-active {
|
||||
.p-stepitem.p-stepitem-active {
|
||||
flex: 1 1 auto;
|
||||
}
|
||||
|
||||
.p-stepper-vertical .p-stepper-panel .p-stepper-item {
|
||||
.p-stepitem .p-step {
|
||||
flex: initial;
|
||||
}
|
||||
|
||||
.p-stepper-vertical .p-stepper-panel .p-stepper-panel-content {
|
||||
.p-stepitem .p-steppanel-content {
|
||||
width: 100%;
|
||||
padding: ${dt('stepper.panel.content.orientation.vertical.padding')};
|
||||
padding: ${dt('stepper.steppanel.padding')};
|
||||
}
|
||||
|
||||
.p-stepper-vertical .p-stepper-panel .p-stepper-separator {
|
||||
.p-stepitem .p-steppanel {
|
||||
display: flex;
|
||||
flex: 1 1 auto;
|
||||
}
|
||||
|
||||
.p-stepitem .p-stepper-separator {
|
||||
flex: 0 0 auto;
|
||||
width: ${dt('stepper.separator.size')};
|
||||
height: auto;
|
||||
margin: ${dt('stepper.separator.orientation.vertical.margin')};
|
||||
margin: ${dt('stepper.separator.margin')};
|
||||
position: relative;
|
||||
left: calc(-1 * ${dt('stepper.separator.size')} / 2);
|
||||
left: calc(-1 * ${dt('stepper.separator.size')});
|
||||
}
|
||||
|
||||
.p-stepper-vertical .p-stepper-panel:has(~ .p-stepper-panel-active) .p-stepper-separator {
|
||||
background: ${dt('stepper.connector.active.background')};
|
||||
.p-stepitem:has(~ .p-stepitem-active) .p-stepper-separator {
|
||||
background: ${dt('stepper.separator.active.background')};
|
||||
}
|
||||
|
||||
.p-stepper-vertical .p-stepper-panel:last-of-type .p-stepper-panel-content {
|
||||
padding: ${dt('stepper.panel.content.orientation.vertical.last.padding')};
|
||||
.p-stepitem:last-of-type .p-steppanel {
|
||||
padding: ${dt('stepper.steppanel.last.padding')};
|
||||
}
|
||||
`;
|
||||
|
||||
|
@ -187,34 +187,10 @@ const classes = {
|
|||
root: ({ props }) => [
|
||||
'p-stepper p-component',
|
||||
{
|
||||
'p-stepper-horizontal': props.orientation === 'horizontal',
|
||||
'p-stepper-vertical': props.orientation === 'vertical',
|
||||
'p-readonly': props.linear
|
||||
}
|
||||
],
|
||||
list: 'p-stepper-list',
|
||||
stepper: {
|
||||
item: ({ instance, step, index }) => [
|
||||
'p-stepper-item',
|
||||
{
|
||||
'p-stepper-item-active': instance.isStepActive(index),
|
||||
'p-disabled': instance.isItemDisabled(index)
|
||||
}
|
||||
],
|
||||
itemHeader: 'p-stepper-item-header',
|
||||
itemNumber: 'p-stepper-item-number',
|
||||
itemTitle: 'p-stepper-item-title',
|
||||
separator: 'p-stepper-separator',
|
||||
panelContentContainer: 'p-stepper-panel-content-container',
|
||||
panelContent: 'p-stepper-panel-content'
|
||||
},
|
||||
panels: 'p-stepper-panels',
|
||||
panel: ({ instance, props, index }) => [
|
||||
'p-stepper-panel',
|
||||
{
|
||||
'p-stepper-panel-active': props.orientation === 'vertical' && instance.isStepActive(index)
|
||||
}
|
||||
]
|
||||
separator: 'p-stepper-separator'
|
||||
};
|
||||
|
||||
export default BaseStyle.extend({
|
||||
|
|
|
@ -266,6 +266,8 @@ export interface StepperPanelEmitsOptions {}
|
|||
export declare type StepperPanelEmits = EmitFn<StepperPanelEmitsOptions>;
|
||||
|
||||
/**
|
||||
* @deprecated since v4. Use the new structure of Stepper instead.
|
||||
*
|
||||
* **PrimeVue - StepperPanel**
|
||||
*
|
||||
* _StepperPanel is a helper component for Stepper component._
|
||||
|
|
|
@ -7,6 +7,9 @@ import BaseStepperPanel from './BaseStepperPanel.vue';
|
|||
|
||||
export default {
|
||||
name: 'StepperPanel',
|
||||
extends: BaseStepperPanel
|
||||
extends: BaseStepperPanel,
|
||||
mounted() {
|
||||
console.warn('Deprecated since v4. Use the new structure of Stepper instead.');
|
||||
}
|
||||
};
|
||||
</script>
|
||||
|
|
|
@ -5,18 +5,14 @@ export default {
|
|||
separator: {
|
||||
background: '{content.border.color}',
|
||||
activeBackground: '{primary.color}',
|
||||
orientation: {
|
||||
vertical: {
|
||||
margin: '0 0 0 1.625rem'
|
||||
}
|
||||
},
|
||||
margin: '0 0 0 1.625rem',
|
||||
size: '2px'
|
||||
},
|
||||
item: {
|
||||
step: {
|
||||
padding: '0.5rem',
|
||||
gap: '1rem'
|
||||
},
|
||||
itemHeader: {
|
||||
stepHeader: {
|
||||
padding: '0',
|
||||
borderRadius: '{content.border.radius}',
|
||||
focusRing: {
|
||||
|
@ -28,12 +24,12 @@ export default {
|
|||
},
|
||||
gap: '0.5rem'
|
||||
},
|
||||
itemTitle: {
|
||||
stepTitle: {
|
||||
color: '{text.muted.color}',
|
||||
activeColor: '{primary.color}',
|
||||
fontWeight: '500'
|
||||
},
|
||||
itemNumber: {
|
||||
stepNumber: {
|
||||
background: '{content.background}',
|
||||
activeBackground: '{content.background}',
|
||||
borderColor: '{content.border.color}',
|
||||
|
@ -46,22 +42,15 @@ export default {
|
|||
borderRadius: '50%',
|
||||
shadow: '0px 0.5px 0px 0px rgba(0, 0, 0, 0.06), 0px 1px 1px 0px rgba(0, 0, 0, 0.12)'
|
||||
},
|
||||
verticalPanelContainer: {
|
||||
paddingLeft: '1rem'
|
||||
steppanels: {
|
||||
padding: '0.875rem 0.5rem 1.125rem 0.5rem'
|
||||
},
|
||||
panelContent: {
|
||||
steppanel: {
|
||||
background: '{content.background}',
|
||||
color: '{content.color}',
|
||||
orientation: {
|
||||
horizontal: {
|
||||
padding: '0.875rem 0.5rem 1.125rem 0.5rem'
|
||||
},
|
||||
vertical: {
|
||||
padding: '0 0 0 1rem',
|
||||
last: {
|
||||
padding: '0 0 0 3rem'
|
||||
}
|
||||
}
|
||||
padding: '0 0 0 1rem',
|
||||
last: {
|
||||
padding: '0 0 0 1.625rem'
|
||||
}
|
||||
}
|
||||
};
|
||||
|
|
|
@ -5,18 +5,14 @@ export default {
|
|||
separator: {
|
||||
background: '{content.border.color}',
|
||||
activeBackground: '{primary.color}',
|
||||
orientation: {
|
||||
vertical: {
|
||||
margin: '0 0 0 1.625rem'
|
||||
}
|
||||
},
|
||||
margin: '0 0 0 1.625rem',
|
||||
size: '2px'
|
||||
},
|
||||
item: {
|
||||
step: {
|
||||
padding: '0.5rem',
|
||||
gap: '1rem'
|
||||
},
|
||||
itemHeader: {
|
||||
stepHeader: {
|
||||
padding: '0',
|
||||
borderRadius: '{content.border.radius}',
|
||||
focusRing: {
|
||||
|
@ -28,12 +24,12 @@ export default {
|
|||
},
|
||||
gap: '0.5rem'
|
||||
},
|
||||
itemTitle: {
|
||||
stepTitle: {
|
||||
color: '{text.muted.color}',
|
||||
activeColor: '{primary.color}',
|
||||
fontWeight: '500'
|
||||
},
|
||||
itemNumber: {
|
||||
stepNumber: {
|
||||
background: '{content.background}',
|
||||
activeBackground: '{primary.color}',
|
||||
borderColor: '{content.border.color}',
|
||||
|
@ -46,22 +42,15 @@ export default {
|
|||
borderRadius: '50%',
|
||||
shadow: 'none'
|
||||
},
|
||||
verticalPanelContainer: {
|
||||
paddingLeft: '1rem'
|
||||
steppanels: {
|
||||
padding: '0.875rem 0.5rem 1.125rem 0.5rem'
|
||||
},
|
||||
panelContent: {
|
||||
steppanel: {
|
||||
background: '{content.background}',
|
||||
color: '{content.color}',
|
||||
orientation: {
|
||||
horizontal: {
|
||||
padding: '0.875rem 0.5rem 1.125rem 0.5rem'
|
||||
},
|
||||
vertical: {
|
||||
padding: '0 0 0 1rem',
|
||||
last: {
|
||||
padding: '0 0 0 3rem'
|
||||
}
|
||||
}
|
||||
padding: '0 0 0 1rem',
|
||||
last: {
|
||||
padding: '0 0 0 1.625rem'
|
||||
}
|
||||
}
|
||||
};
|
||||
|
|
|
@ -5,18 +5,14 @@ export default {
|
|||
separator: {
|
||||
background: '{content.border.color}',
|
||||
activeBackground: '{primary.color}',
|
||||
orientation: {
|
||||
vertical: {
|
||||
margin: '0 0 0 1.625rem'
|
||||
}
|
||||
},
|
||||
margin: '0 0 0 1.625rem',
|
||||
size: '2px'
|
||||
},
|
||||
item: {
|
||||
step: {
|
||||
padding: '0.5rem',
|
||||
gap: '1rem'
|
||||
},
|
||||
itemHeader: {
|
||||
stepHeader: {
|
||||
padding: '0',
|
||||
borderRadius: '{content.border.radius}',
|
||||
focusRing: {
|
||||
|
@ -28,12 +24,12 @@ export default {
|
|||
},
|
||||
gap: '0.5rem'
|
||||
},
|
||||
itemTitle: {
|
||||
stepTitle: {
|
||||
color: '{text.muted.color}',
|
||||
activeColor: '{primary.color}',
|
||||
fontWeight: '700'
|
||||
},
|
||||
itemNumber: {
|
||||
stepNumber: {
|
||||
background: '{content.background}',
|
||||
activeBackground: '{primary.color}',
|
||||
borderColor: '{content.border.color}',
|
||||
|
@ -46,22 +42,15 @@ export default {
|
|||
borderRadius: '50%',
|
||||
shadow: 'none'
|
||||
},
|
||||
verticalPanelContainer: {
|
||||
paddingLeft: '1rem'
|
||||
steppanels: {
|
||||
padding: '0.875rem 0.5rem 1.125rem 0.5rem'
|
||||
},
|
||||
panelContent: {
|
||||
steppanel: {
|
||||
background: '{content.background}',
|
||||
color: '{content.color}',
|
||||
orientation: {
|
||||
horizontal: {
|
||||
padding: '0.875rem 0.5rem 1.125rem 0.5rem'
|
||||
},
|
||||
vertical: {
|
||||
padding: '0 0 0 1rem',
|
||||
last: {
|
||||
padding: '0 0 0 3rem'
|
||||
}
|
||||
}
|
||||
padding: '0 0 0 1rem',
|
||||
last: {
|
||||
padding: '0 0 0 1.625rem'
|
||||
}
|
||||
}
|
||||
};
|
||||
|
|
|
@ -39,21 +39,11 @@ export interface StepperDesignTokens extends ColorSchemeDesignToken<StepperDesig
|
|||
*/
|
||||
activeBackground?: string;
|
||||
/**
|
||||
* Orientation of separator
|
||||
* Margin of separator
|
||||
*
|
||||
* @designToken stepper.separator.margin
|
||||
*/
|
||||
orientation?: {
|
||||
/**
|
||||
* Orientation vertical of separator
|
||||
*/
|
||||
vertical?: {
|
||||
/**
|
||||
* Orientation vertical margin of separator
|
||||
*
|
||||
* @designToken stepper.separator.orientation.vertical.margin
|
||||
*/
|
||||
margin?: string;
|
||||
};
|
||||
};
|
||||
margin?: string;
|
||||
/**
|
||||
* Size of separator
|
||||
*
|
||||
|
@ -62,238 +52,217 @@ export interface StepperDesignTokens extends ColorSchemeDesignToken<StepperDesig
|
|||
size?: string;
|
||||
};
|
||||
/**
|
||||
* Used to pass tokens of the item section
|
||||
* Used to pass tokens of the step section
|
||||
*/
|
||||
item?: {
|
||||
step?: {
|
||||
/**
|
||||
* Padding of item
|
||||
* Padding of step
|
||||
*
|
||||
* @designToken stepper.item.padding
|
||||
* @designToken stepper.step.padding
|
||||
*/
|
||||
padding?: string;
|
||||
/**
|
||||
* Gap of item
|
||||
* Gap of step
|
||||
*
|
||||
* @designToken stepper.item.gap
|
||||
* @designToken stepper.step.gap
|
||||
*/
|
||||
gap?: string;
|
||||
};
|
||||
/**
|
||||
* Used to pass tokens of the item header section
|
||||
* Used to pass tokens of the step header section
|
||||
*/
|
||||
itemHeader?: {
|
||||
stepHeader?: {
|
||||
/**
|
||||
* Padding of item header
|
||||
* Padding of step header
|
||||
*
|
||||
* @designToken stepper.item.header.padding
|
||||
* @designToken stepper.step.header.padding
|
||||
*/
|
||||
padding?: string;
|
||||
/**
|
||||
* Border radius of item header
|
||||
* Border radius of step header
|
||||
*
|
||||
* @designToken stepper.item.header.border.radius
|
||||
* @designToken stepper.step.header.border.radius
|
||||
*/
|
||||
borderRadius?: string;
|
||||
/**
|
||||
* Focus ring of item header
|
||||
* Focus ring of step header
|
||||
*/
|
||||
focusRing?: {
|
||||
/**
|
||||
* Focus ring width of item header
|
||||
* Focus ring width of step header
|
||||
*
|
||||
* @designToken stepper.item.header.focus.ring.width
|
||||
* @designToken stepper.step.header.focus.ring.width
|
||||
*/
|
||||
width?: string;
|
||||
/**
|
||||
* Focus ring style of item header
|
||||
* Focus ring style of step header
|
||||
*
|
||||
* @designToken stepper.item.header.focus.ring.style
|
||||
* @designToken stepper.step.header.focus.ring.style
|
||||
*/
|
||||
style?: string;
|
||||
/**
|
||||
* Focus ring color of item header
|
||||
* Focus ring color of step header
|
||||
*
|
||||
* @designToken stepper.item.header.focus.ring.color
|
||||
* @designToken stepper.step.header.focus.ring.color
|
||||
*/
|
||||
color?: string;
|
||||
/**
|
||||
* Focus ring offset of item header
|
||||
* Focus ring offset of step header
|
||||
*
|
||||
* @designToken stepper.item.header.focus.ring.offset
|
||||
* @designToken stepper.step.header.focus.ring.offset
|
||||
*/
|
||||
offset?: string;
|
||||
/**
|
||||
* Focus ring shadow of item header
|
||||
* Focus ring shadow of step header
|
||||
*
|
||||
* @designToken stepper.item.header.focus.ring.shadow
|
||||
* @designToken stepper.step.header.focus.ring.shadow
|
||||
*/
|
||||
shadow?: string;
|
||||
};
|
||||
/**
|
||||
* Gap of item header
|
||||
* Gap of step header
|
||||
*
|
||||
* @designToken stepper.item.header.gap
|
||||
* @designToken stepper.step.header.gap
|
||||
*/
|
||||
gap?: string;
|
||||
};
|
||||
/**
|
||||
* Used to pass tokens of the item title section
|
||||
* Used to pass tokens of the step title section
|
||||
*/
|
||||
itemTitle?: {
|
||||
stepTitle?: {
|
||||
/**
|
||||
* Color of item title
|
||||
* Color of step title
|
||||
*
|
||||
* @designToken stepper.item.title.color
|
||||
* @designToken stepper.step.title.color
|
||||
*/
|
||||
color?: string;
|
||||
/**
|
||||
* Active color of item title
|
||||
* Active color of step title
|
||||
*
|
||||
* @designToken stepper.item.title.active.color
|
||||
* @designToken stepper.step.title.active.color
|
||||
*/
|
||||
activeColor?: string;
|
||||
/**
|
||||
* Font weight of item title
|
||||
* Font weight of step title
|
||||
*
|
||||
* @designToken stepper.item.title.font.weight
|
||||
* @designToken stepper.step.title.font.weight
|
||||
*/
|
||||
fontWeight?: string;
|
||||
};
|
||||
/**
|
||||
* Used to pass tokens of the item number section
|
||||
* Used to pass tokens of the step number section
|
||||
*/
|
||||
itemNumber?: {
|
||||
stepNumber?: {
|
||||
/**
|
||||
* Background of item number
|
||||
* Background of step number
|
||||
*
|
||||
* @designToken stepper.item.number.background
|
||||
* @designToken stepper.step.number.background
|
||||
*/
|
||||
background?: string;
|
||||
/**
|
||||
* Active background of item number
|
||||
* Active background of step number
|
||||
*
|
||||
* @designToken stepper.item.number.active.background
|
||||
* @designToken stepper.step.number.active.background
|
||||
*/
|
||||
activeBackground?: string;
|
||||
/**
|
||||
* Border color of item number
|
||||
* Border color of step number
|
||||
*
|
||||
* @designToken stepper.item.number.border.color
|
||||
* @designToken stepper.step.number.border.color
|
||||
*/
|
||||
borderColor?: string;
|
||||
/**
|
||||
* Active border color of item number
|
||||
* Active border color of step number
|
||||
*
|
||||
* @designToken stepper.item.number.active.border.color
|
||||
* @designToken stepper.step.number.active.border.color
|
||||
*/
|
||||
activeBorderColor?: string;
|
||||
/**
|
||||
* Color of item number
|
||||
* Color of step number
|
||||
*
|
||||
* @designToken stepper.item.number.color
|
||||
* @designToken stepper.step.number.color
|
||||
*/
|
||||
color?: string;
|
||||
/**
|
||||
* Active color of item number
|
||||
* Active color of step number
|
||||
*
|
||||
* @designToken stepper.item.number.active.color
|
||||
* @designToken stepper.step.number.active.color
|
||||
*/
|
||||
activeColor?: string;
|
||||
/**
|
||||
* Size of item number
|
||||
* Size of step number
|
||||
*
|
||||
* @designToken stepper.item.number.size
|
||||
* @designToken stepper.step.number.size
|
||||
*/
|
||||
size?: string;
|
||||
/**
|
||||
* Font size of item number
|
||||
* Font size of step number
|
||||
*
|
||||
* @designToken stepper.item.number.font.size
|
||||
* @designToken stepper.step.number.font.size
|
||||
*/
|
||||
fontSize?: string;
|
||||
/**
|
||||
* Font weight of item number
|
||||
* Font weight of step number
|
||||
*
|
||||
* @designToken stepper.item.number.font.weight
|
||||
* @designToken stepper.step.number.font.weight
|
||||
*/
|
||||
fontWeight?: string;
|
||||
/**
|
||||
* Border radius of item number
|
||||
* Border radius of step number
|
||||
*
|
||||
* @designToken stepper.item.number.border.radius
|
||||
* @designToken stepper.step.number.border.radius
|
||||
*/
|
||||
borderRadius?: string;
|
||||
/**
|
||||
* Shadow of item number
|
||||
* Shadow of step number
|
||||
*
|
||||
* @designToken stepper.item.number.shadow
|
||||
* @designToken stepper.step.number.shadow
|
||||
*/
|
||||
shadow?: string;
|
||||
};
|
||||
/**
|
||||
* Used to pass tokens of the vertical panel container section
|
||||
* Used to pass tokens of the steppanels section
|
||||
*/
|
||||
verticalPanelContainer?: {
|
||||
steppanels?: {
|
||||
/**
|
||||
* Padding left of vertical panel container
|
||||
* Padding of steppanels
|
||||
*
|
||||
* @designToken stepper.vertical.panel.container.padding.left
|
||||
* @designToken stepper.steppanels.padding
|
||||
*/
|
||||
paddingLeft?: string;
|
||||
padding?: string;
|
||||
};
|
||||
/**
|
||||
* Used to pass tokens of the panel content section
|
||||
* Used to pass tokens of the steppanel section
|
||||
*/
|
||||
panelContent?: {
|
||||
steppanel?: {
|
||||
/**
|
||||
* Background of panel content
|
||||
* Background of steppanel
|
||||
*
|
||||
* @designToken stepper.panel.content.background
|
||||
* @designToken stepper.steppanel.background
|
||||
*/
|
||||
background?: string;
|
||||
/**
|
||||
* Color of panel content
|
||||
* Color of steppanel
|
||||
*
|
||||
* @designToken stepper.panel.content.color
|
||||
* @designToken stepper.steppanel.color
|
||||
*/
|
||||
color?: string;
|
||||
/**
|
||||
* Orientation of panel content
|
||||
* Padding of steppanel
|
||||
*
|
||||
* @designToken stepper.steppanel.padding
|
||||
*/
|
||||
orientation?: {
|
||||
padding?: string;
|
||||
/**
|
||||
* Last of steppanel
|
||||
*/
|
||||
last?: {
|
||||
/**
|
||||
* Orientation horizontal of panel content
|
||||
* Last padding of steppanel
|
||||
*
|
||||
* @designToken stepper.steppanel.last.padding
|
||||
*/
|
||||
horizontal?: {
|
||||
/**
|
||||
* Orientation horizontal padding of panel content
|
||||
*
|
||||
* @designToken stepper.panel.content.orientation.horizontal.padding
|
||||
*/
|
||||
padding?: string;
|
||||
};
|
||||
/**
|
||||
* Orientation vertical of panel content
|
||||
*/
|
||||
vertical?: {
|
||||
/**
|
||||
* Orientation vertical padding of panel content
|
||||
*
|
||||
* @designToken stepper.panel.content.orientation.vertical.padding
|
||||
*/
|
||||
padding?: string;
|
||||
/**
|
||||
* Orientation vertical last of panel content
|
||||
*/
|
||||
last?: {
|
||||
/**
|
||||
* Orientation vertical last padding of panel content
|
||||
*
|
||||
* @designToken stepper.panel.content.orientation.vertical.last.padding
|
||||
*/
|
||||
padding?: string;
|
||||
};
|
||||
};
|
||||
padding?: string;
|
||||
};
|
||||
};
|
||||
}
|
||||
|
|
|
@ -52,6 +52,11 @@ const panel = [
|
|||
'ScrollPanel',
|
||||
'Splitter',
|
||||
'SplitterPanel',
|
||||
'Step',
|
||||
'StepItem',
|
||||
'StepList',
|
||||
'StepPanel',
|
||||
'StepPanels',
|
||||
'Stepper',
|
||||
'StepperPanel',
|
||||
'TabView',
|
||||
|
|
|
@ -102,9 +102,14 @@ const STYLE_ALIAS = {
|
|||
'primevue/splitbutton/style': path.resolve(__dirname, './components/lib/splitbutton/style/SplitButtonStyle.js'),
|
||||
'primevue/splitter/style': path.resolve(__dirname, './components/lib/splitter/style/SplitterStyle.js'),
|
||||
'primevue/splitterpanel/style': path.resolve(__dirname, './components/lib/splitterpanel/style/SplitterPanelStyle.js'),
|
||||
'primevue/steps/style': path.resolve(__dirname, './components/lib/steps/style/StepsStyle.js'),
|
||||
'primevue/step/style': path.resolve(__dirname, './components/lib/step/style/StepStyle.js'),
|
||||
'primevue/stepitem/style': path.resolve(__dirname, './components/lib/stepitem/style/StepItemStyle.js'),
|
||||
'primevue/steplist/style': path.resolve(__dirname, './components/lib/steplist/style/StepListStyle.js'),
|
||||
'primevue/steppanel/style': path.resolve(__dirname, './components/lib/steppanel/style/StepPanelStyle.js'),
|
||||
'primevue/steppanels/style': path.resolve(__dirname, './components/lib/steppanels/style/StepPanelsStyle.js'),
|
||||
'primevue/stepper/style': path.resolve(__dirname, './components/lib/stepper/style/StepperStyle.js'),
|
||||
'primevue/stepperpanel/style': path.resolve(__dirname, './components/lib/stepperpanel/style/StepperPanelStyle.js'),
|
||||
'primevue/steps/style': path.resolve(__dirname, './components/lib/steps/style/StepsStyle.js'),
|
||||
'primevue/styleclass/style': path.resolve(__dirname, './components/lib/styleclass/style/StyleClassStyle.js'),
|
||||
'primevue/tabmenu/style': path.resolve(__dirname, './components/lib/tabmenu/style/TabMenuStyle.js'),
|
||||
'primevue/tabs/style': path.resolve(__dirname, './components/lib/tabs/style/TabsStyle.js'),
|
||||
|
|
Loading…
Reference in New Issue