Refactor #5175 - IconField component added
parent
355bbebe7e
commit
0f2aa807bd
|
@ -0,0 +1,21 @@
|
|||
<script>
|
||||
import BaseComponent from 'primevue/basecomponent';
|
||||
import IconFieldStyle from 'primevue/iconfield/style';
|
||||
|
||||
export default {
|
||||
name: 'BaseIconField',
|
||||
extends: BaseComponent,
|
||||
props: {
|
||||
iconPosition: {
|
||||
type: String,
|
||||
default: 'right'
|
||||
}
|
||||
},
|
||||
style: IconFieldStyle,
|
||||
provide() {
|
||||
return {
|
||||
$parentInstance: this
|
||||
};
|
||||
}
|
||||
};
|
||||
</script>
|
|
@ -0,0 +1,127 @@
|
|||
/**
|
||||
*
|
||||
* IconField is used to select a boolean value.
|
||||
*
|
||||
* [Live Demo](https://www.primevue.org/inputtext/)
|
||||
*
|
||||
* @module iconfield
|
||||
*
|
||||
*/
|
||||
import { InputHTMLAttributes, VNode } from 'vue';
|
||||
import { ComponentHooks } from '../basecomponent';
|
||||
import { PassThroughOptions } from '../passthrough';
|
||||
import { ClassComponent, GlobalComponentConstructor, PassThrough } from '../ts-helpers';
|
||||
|
||||
export declare type IconFieldPassThroughOptionType = IconFieldPassThroughAttributes | ((options: IconFieldPassThroughMethodOptions) => IconFieldPassThroughAttributes | string) | string | null | undefined;
|
||||
|
||||
/**
|
||||
* Custom passthrough(pt) option method.
|
||||
*/
|
||||
export interface IconFieldPassThroughMethodOptions {
|
||||
/**
|
||||
* Defines instance.
|
||||
*/
|
||||
instance: any;
|
||||
/**
|
||||
* Defines valid properties.
|
||||
*/
|
||||
props: IconFieldProps;
|
||||
/**
|
||||
* Defines valid attributes.
|
||||
*/
|
||||
attrs: any;
|
||||
/**
|
||||
* Defines parent options.
|
||||
*/
|
||||
parent: any;
|
||||
/**
|
||||
* Defines passthrough(pt) options in global config.
|
||||
*/
|
||||
global: object | undefined;
|
||||
}
|
||||
|
||||
/**
|
||||
* Custom passthrough(pt) options.
|
||||
* @see {@link IconFieldProps.pt}
|
||||
*/
|
||||
export interface IconFieldPassThroughOptions {
|
||||
/**
|
||||
* Used to pass attributes to the root's DOM element.
|
||||
*/
|
||||
root?: IconFieldPassThroughOptionType;
|
||||
/**
|
||||
* Used to manage all lifecycle hooks.
|
||||
* @see {@link BaseComponent.ComponentHooks}
|
||||
*/
|
||||
hooks?: ComponentHooks;
|
||||
}
|
||||
|
||||
/**
|
||||
* Custom passthrough attributes for each DOM elements
|
||||
*/
|
||||
export interface IconFieldPassThroughAttributes {
|
||||
[key: string]: any;
|
||||
}
|
||||
|
||||
/**
|
||||
* Defines valid properties in IconField component.
|
||||
*/
|
||||
export interface IconFieldProps {
|
||||
/**
|
||||
* Position of the icon
|
||||
* @defaultValue right
|
||||
*/
|
||||
iconPosition?: 'left' | 'right' | undefined;
|
||||
/**
|
||||
* Used to pass attributes to DOM elements inside the component.
|
||||
* @type {IconFieldPassThroughOptions}
|
||||
*/
|
||||
pt?: PassThrough<IconFieldPassThroughOptions>;
|
||||
/**
|
||||
* Used to configure passthrough(pt) options of the component.
|
||||
* @type {PassThroughOptions}
|
||||
*/
|
||||
ptOptions?: PassThroughOptions;
|
||||
/**
|
||||
* When enabled, it removes component related styles in the core.
|
||||
* @defaultValue false
|
||||
*/
|
||||
unstyled?: boolean;
|
||||
}
|
||||
|
||||
/**
|
||||
* Defines valid slots in IconField component.
|
||||
*/
|
||||
export interface IconFieldSlots {
|
||||
/**
|
||||
* Default slot for content.
|
||||
*/
|
||||
default(): VNode[];
|
||||
}
|
||||
|
||||
/**
|
||||
* Defines valid emits in IconField component.
|
||||
*/
|
||||
export interface IconFieldEmits {}
|
||||
|
||||
/**
|
||||
* **PrimeVue - IconField**
|
||||
*
|
||||
* _IconField is used to select a boolean value._
|
||||
*
|
||||
* [Live Demo](https://www.primevue.org/inputtext/)
|
||||
* --- ---
|
||||
* 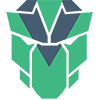
|
||||
*
|
||||
* @group Component
|
||||
*
|
||||
*/
|
||||
declare class IconField extends ClassComponent<IconFieldProps, IconFieldSlots, IconFieldEmits> {}
|
||||
|
||||
declare module '@vue/runtime-core' {
|
||||
interface GlobalComponents {
|
||||
iconfield: GlobalComponentConstructor<IconField>;
|
||||
}
|
||||
}
|
||||
|
||||
export default IconField;
|
|
@ -0,0 +1,14 @@
|
|||
<template>
|
||||
<div :class="cx('root')" v-bind="ptm('root')" data-pc-name="iconfield">
|
||||
<slot />
|
||||
</div>
|
||||
</template>
|
||||
|
||||
<script>
|
||||
import BaseIconFeild from './BaseIconField.vue';
|
||||
|
||||
export default {
|
||||
name: 'IconField',
|
||||
extends: BaseIconFeild
|
||||
};
|
||||
</script>
|
|
@ -0,0 +1,9 @@
|
|||
{
|
||||
"main": "./iconfield.cjs.js",
|
||||
"module": "./iconfield.esm.js",
|
||||
"unpkg": "./iconfield.min.js",
|
||||
"types": "./IconField.d.ts",
|
||||
"browser": {
|
||||
"./sfc": "./IconField.vue"
|
||||
}
|
||||
}
|
|
@ -0,0 +1,3 @@
|
|||
import { BaseStyle } from '../../base/style';
|
||||
|
||||
export interface IconFieldStyle extends BaseStyle {}
|
|
@ -0,0 +1,41 @@
|
|||
import BaseStyle from 'primevue/base/style';
|
||||
|
||||
const css = `
|
||||
@layer primevue {
|
||||
.p-input-icon-left,
|
||||
.p-input-icon-right {
|
||||
position: relative;
|
||||
display: inline-block;
|
||||
}
|
||||
|
||||
.p-input-icon-left > i,
|
||||
.p-input-icon-left > svg,
|
||||
.p-input-icon-right > i,
|
||||
.p-input-icon-right > svg {
|
||||
position: absolute;
|
||||
top: 50%;
|
||||
margin-top: -.5rem;
|
||||
}
|
||||
|
||||
.p-fluid .p-input-icon-left,
|
||||
.p-fluid .p-input-icon-right {
|
||||
display: block;
|
||||
width: 100%;
|
||||
}
|
||||
}
|
||||
`;
|
||||
|
||||
const classes = {
|
||||
root: ({ props }) => [
|
||||
{
|
||||
'p-input-icon-right': props.iconPosition === 'right',
|
||||
'p-input-icon-left': props.iconPosition === 'left'
|
||||
}
|
||||
]
|
||||
};
|
||||
|
||||
export default BaseStyle.extend({
|
||||
name: 'iconfield',
|
||||
css,
|
||||
classes
|
||||
});
|
|
@ -0,0 +1,6 @@
|
|||
{
|
||||
"main": "./iconfieldstyle.cjs.js",
|
||||
"module": "./iconfieldstyle.esm.js",
|
||||
"unpkg": "./iconfieldstyle.min.js",
|
||||
"types": "./IconFieldStyle.d.ts"
|
||||
}
|
Loading…
Reference in New Issue